- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Handle Dependency Injection with GetX Flutter
Discover practical examples and best practices for better app architecture and code organization.
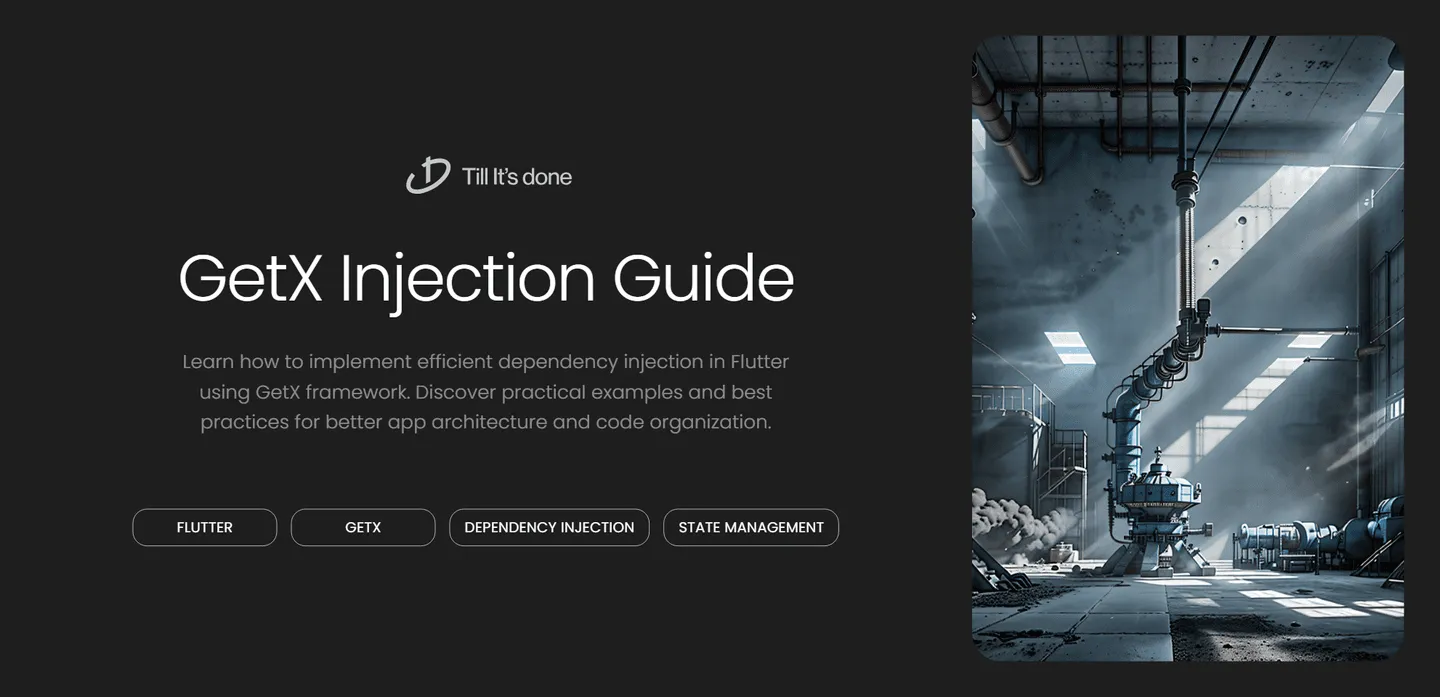
How to Handle Dependency Injection with GetX in Flutter
If you’ve been developing Flutter applications, you’ve probably encountered the need for state management and dependency injection. GetX is a powerful solution that not only handles state management but also provides an elegant way to manage dependencies in your Flutter apps. Let’s dive into how you can leverage GetX for dependency injection to write cleaner, more maintainable code.
Understanding Dependency Injection in GetX
Think of dependency injection like a smart coffee machine that knows exactly what type of coffee beans and water it needs without you having to manually fill it every time. GetX works similarly - it manages your app’s dependencies automatically, making sure each part of your app gets exactly what it needs.
Getting Started with GetX Dependency Injection
The first step in implementing dependency injection with GetX is understanding its core methods. GetX provides several ways to inject dependencies:
- Get.put(): Instantiates your dependency and makes it available for use
- Get.lazyPut(): Creates your dependency only when it’s actually needed
- Get.putAsync(): Perfect for dependencies that require async initialization
- Get.create(): Creates a new instance every time you call Get.find()
Let’s look at a practical example of how these methods work in real-world scenarios.
Practical Implementation
Imagine we’re building a weather app that needs to manage API services and controllers. Here’s how we can structure our dependency injection:
// First, define your serviceclass WeatherService { final apiKey = 'your-api-key';
Future<String> getWeatherData() async { // API call implementation return 'Weather data'; }}
// Create your controllerclass WeatherController extends GetxController { final WeatherService weatherService;
WeatherController({required this.weatherService});
// Controller logic here}
// Initialize dependenciesvoid initializeDependencies() { // Inject the service Get.put(WeatherService());
// Inject the controller with its dependency Get.put(WeatherController(weatherService: Get.find()));}
Best Practices and Tips
- Always initialize your dependencies early in the app lifecycle
- Use
lazyPut
for dependencies that aren’t needed immediately - Implement proper dependency disposal when they’re no longer needed
- Keep your dependency initialization organized in a separate file
- Consider using bindings for better code organization
Conclusion
GetX’s dependency injection system provides a clean and efficient way to manage dependencies in your Flutter applications. By following these patterns and best practices, you can create more maintainable and scalable applications while reducing boilerplate code.
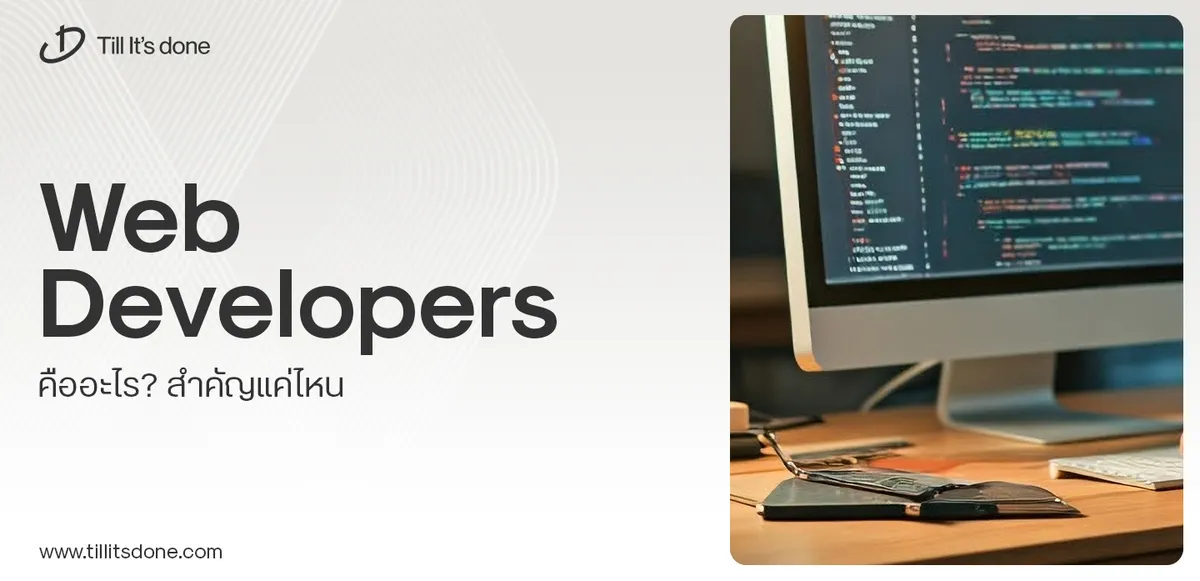
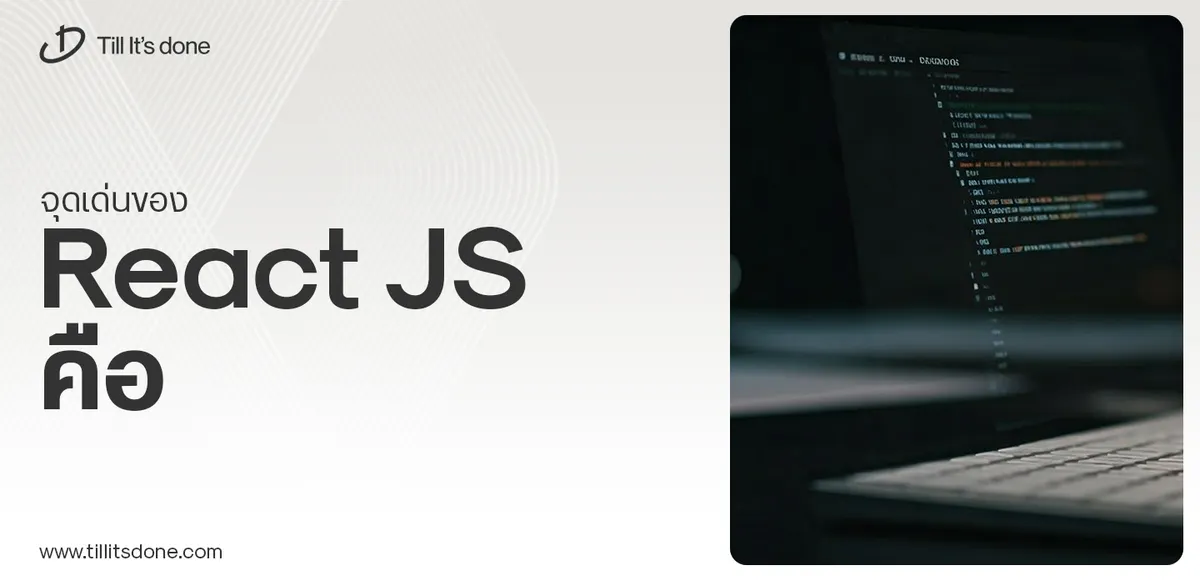
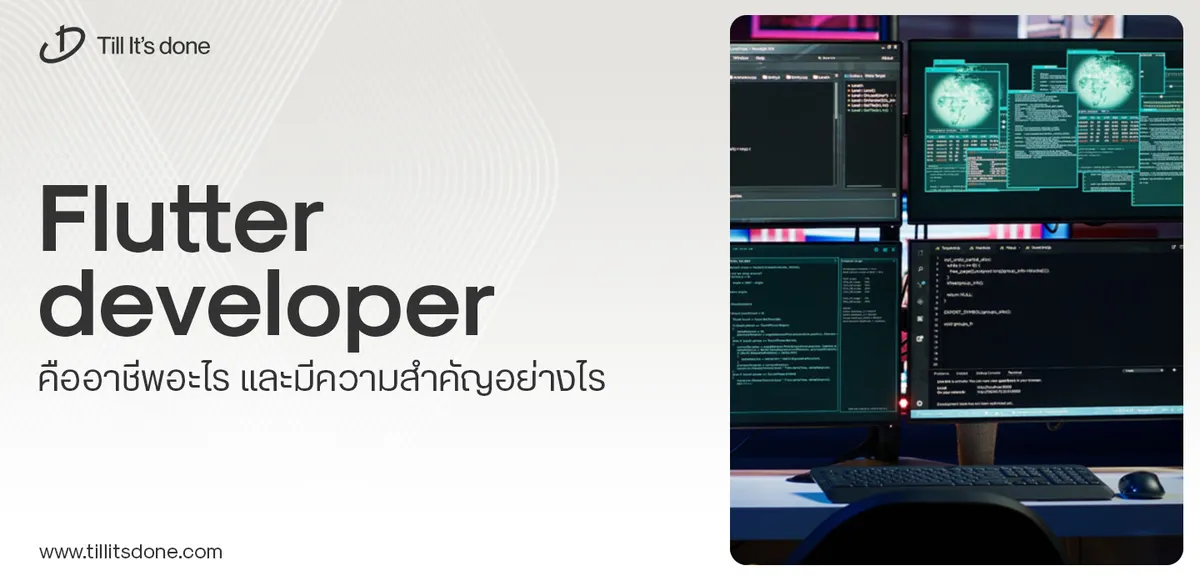
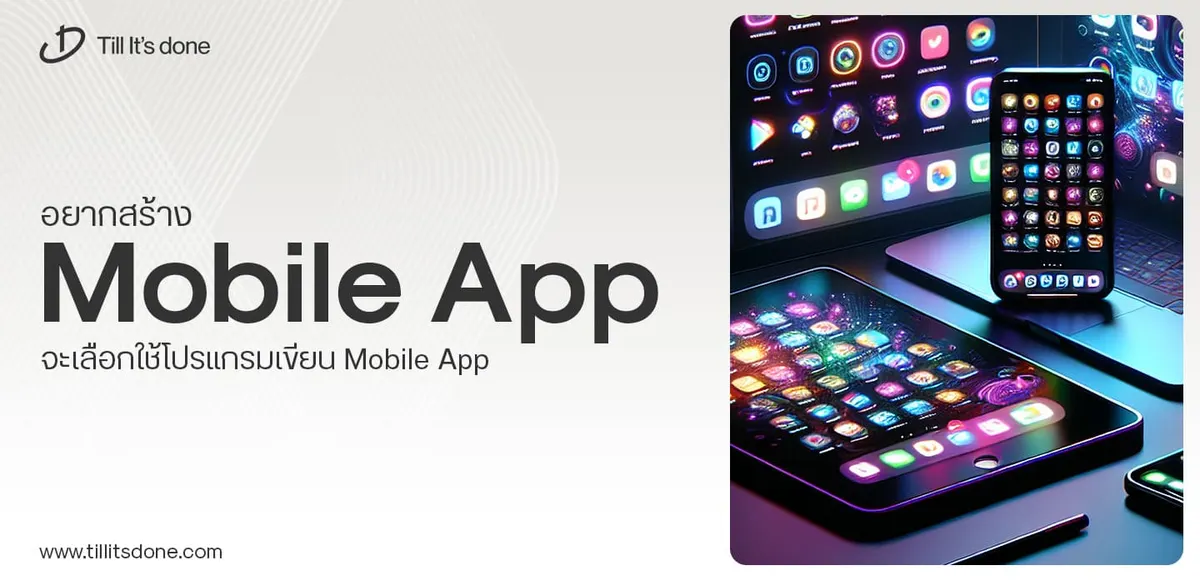
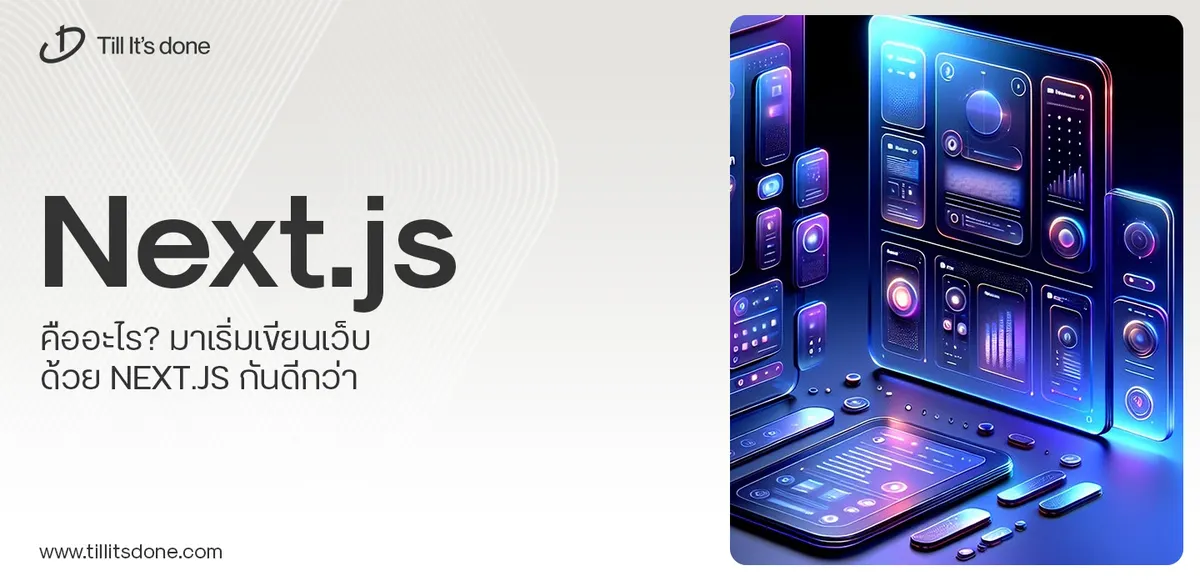
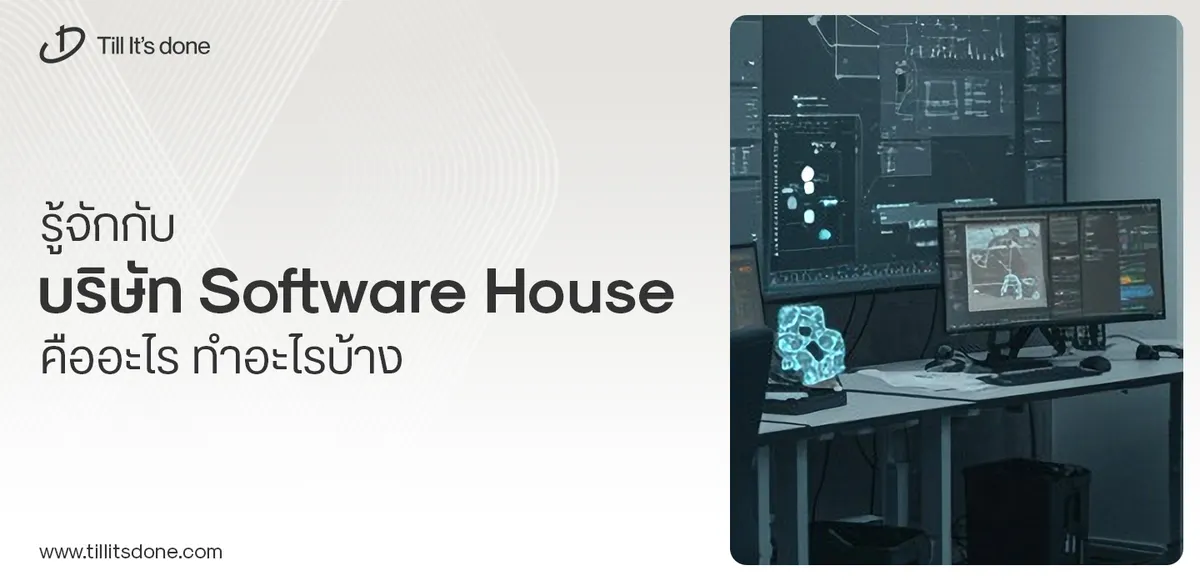
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.