- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Flutter Widget Testing: Best Practices Guide 2024
Improve your app's quality assurance process.

Flutter Widget Testing: Best Practices for Quality Assurance
Widget testing is a crucial aspect of Flutter app development that ensures your UI components behave correctly and consistently. In this comprehensive guide, we’ll explore best practices for implementing effective widget tests in your Flutter projects.
Understanding Widget Testing in Flutter
Widget testing in Flutter allows developers to verify that UI components render correctly and respond appropriately to user interactions. Unlike unit tests that focus on individual functions, widget tests examine how various UI elements work together within your application.
Key Benefits of Widget Testing
Testing your widgets brings several advantages to your development process:
- Early bug detection and prevention
- Improved code reliability and maintainability
- Faster development cycles with confident refactoring
- Better documentation of widget behavior
- Reduced QA testing time
Essential Best Practices
1. Keep Tests Focused and Independent
Each test should focus on validating a single aspect of your widget. Avoid testing multiple behaviors in one test case. This approach makes tests easier to maintain and debug when issues arise.
testWidgets('Counter increments smoke test', (WidgetTester tester) async { await tester.pumpWidget(MyApp()); expect(find.text('0'), findsOneWidget); await tester.tap(find.byIcon(Icons.add)); await tester.pump(); expect(find.text('1'), findsOneWidget);});
2. Use Meaningful Test Descriptions
Write clear, descriptive test names that explain the expected behavior. This helps other developers understand the purpose of each test and makes debugging easier.
3. Implement the Given-When-Then Pattern
Structure your tests using the Given-When-Then pattern to make them more readable and maintainable:
testWidgets('Button changes color when pressed', (WidgetTester tester) async { // Given await tester.pumpWidget(MyButton()); final button = find.byType(ElevatedButton);
// When await tester.tap(button); await tester.pump();
// Then expect(tester.widget<ElevatedButton>(button).style?.backgroundColor, equals(Colors.blue));});
4. Mock Dependencies Effectively
Use mocking to isolate widget tests from external dependencies. This ensures your tests remain reliable and fast:
class MockDataService extends Mock implements DataService {}
testWidgets('Widget displays data from service', (WidgetTester tester) async { final mockService = MockDataService(); when(mockService.getData()).thenReturn(['item1', 'item2']);
await tester.pumpWidget(MyWidget(service: mockService)); expect(find.text('item1'), findsOneWidget);});
5. Test Edge Cases
Don’t forget to test how your widgets handle edge cases:
- Empty states
- Loading states
- Error states
- Different screen sizes
- Various device orientations
6. Use Test Helpers
Create helper functions for common testing patterns to reduce code duplication and improve maintenance:
Future<void> pumpWidgetWithProviders( WidgetTester tester, Widget widget,) async { await tester.pumpWidget( MaterialApp( home: MultiProvider( providers: [ ChangeNotifierProvider(create: (_) => ThemeProvider()), // Add other providers ], child: widget, ), ), );}
Common Pitfalls to Avoid
- Not waiting for animations to complete
- Forgetting to pump the widget tree after state changes
- Testing implementation details instead of behavior
- Not testing user interactions thoroughly
- Overcomplicating test setup
Conclusion
Implementing these widget testing best practices will help you build more reliable Flutter applications. Remember that testing is an investment in your app’s quality and maintainability. Start small, focus on critical components first, and gradually expand your test coverage as your application grows.


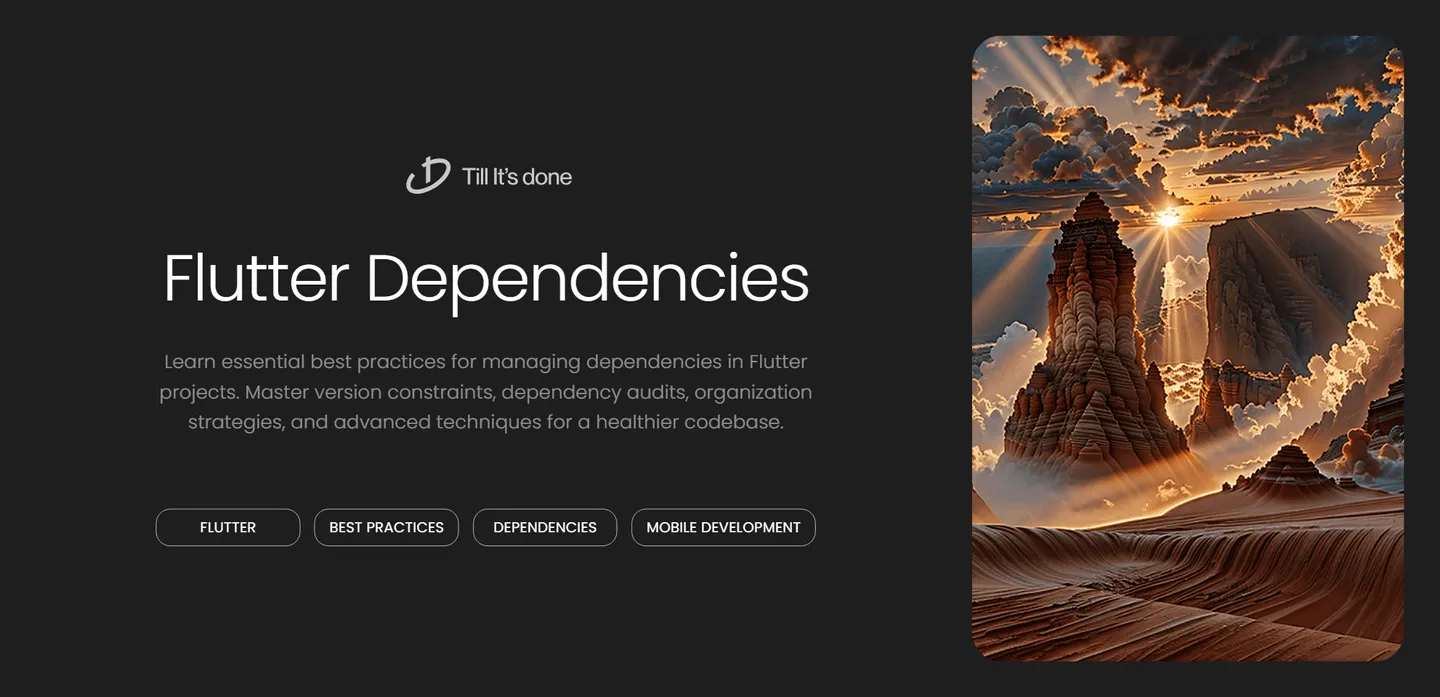



Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.