- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Flutter Test: Write Better Testable Code Now
Discover dependency injection, testing patterns, and practical strategies to create maintainable and robust applications.
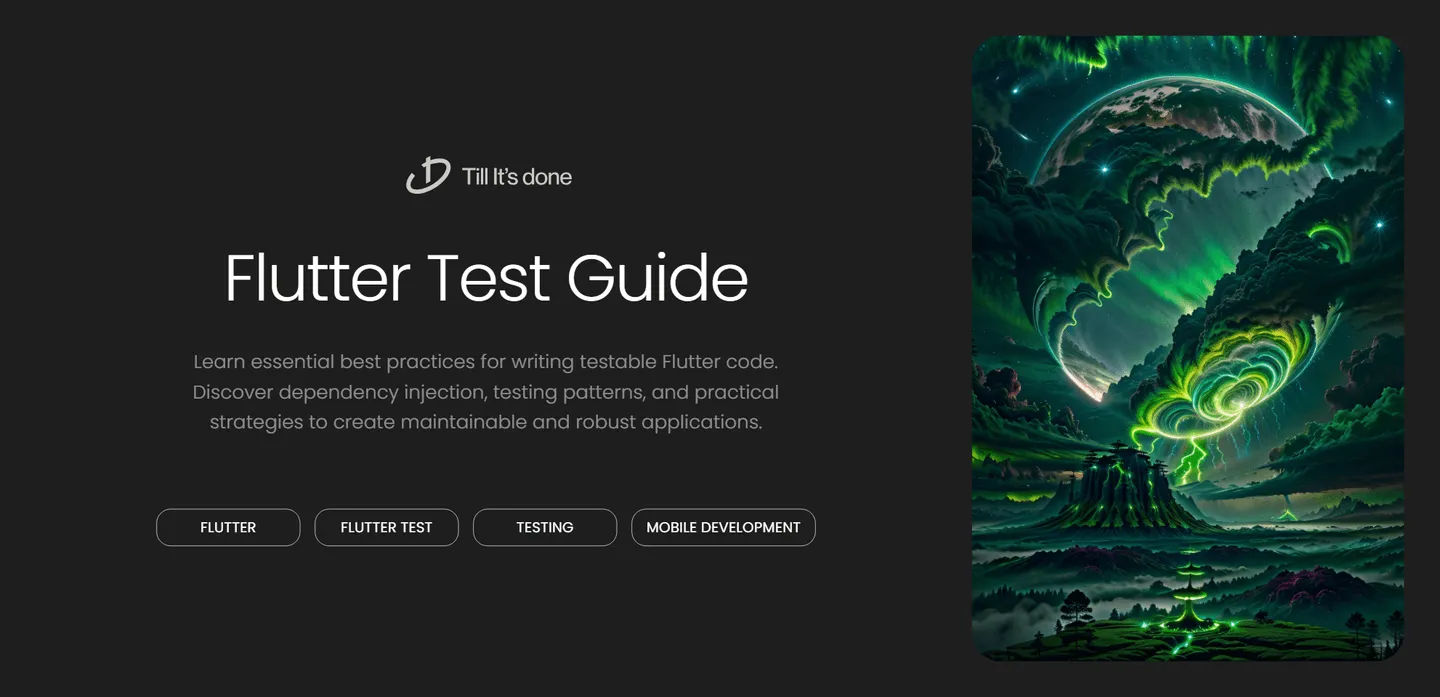
Flutter Test: Best Practices for Writing Testable Code
Writing testable code in Flutter is crucial for maintaining a robust and reliable application. As your Flutter project grows, having a solid testing strategy becomes increasingly important. In this guide, we’ll explore best practices for writing testable Flutter code that will help you build more maintainable applications.
Understanding the Importance of Testing in Flutter
Testing isn’t just about finding bugs – it’s about building confidence in your code. Flutter provides excellent testing utilities that make it easier to write and maintain tests. The key is to structure your code in a way that makes testing natural and straightforward.
Key Principles for Writing Testable Flutter Code
1. Dependency Injection
One of the most important principles for testable code is dependency injection. Instead of creating dependencies inside your widgets or classes, pass them as parameters. This makes it easier to substitute real implementations with mock objects during testing.
// Bad Practiceclass UserProfile { final _api = ApiService();}
// Good Practiceclass UserProfile { final ApiService api; UserProfile(this.api);}
2. Single Responsibility Principle
Keep your widgets and classes focused on a single responsibility. This makes them easier to test and maintain. Break down complex widgets into smaller, more manageable components.
3. State Management Separation
Separate your business logic from your UI code. Using state management solutions like Provider, Riverpod, or BLoC makes your code more testable by creating clear boundaries between different layers of your application.
Best Practices for Flutter Tests
1. Write Different Types of Tests
- Unit Tests: Test individual units of code in isolation
- Widget Tests: Test widget behavior and rendering
- Integration Tests: Test how different parts work together
2. Use Test Doubles Effectively
// Example of using a mockclass MockApiService extends Mock implements ApiService { @override Future<UserData> getUserData() async { return UserData(name: 'Test User'); }}
3. Golden Tests for UI Consistency
Golden tests are perfect for catching unintended visual changes in your UI. They create snapshots of your widgets and compare them against future changes.
4. Test Coverage Strategy
- Focus on testing business-critical features first
- Aim for high coverage in your business logic
- Don’t obsess over 100% coverage – focus on meaningful tests
Common Testing Patterns
1. Builder Pattern for Test Data
class UserBuilder { String name = 'Default Name'; String email = 'default@email.com';
UserBuilder withName(String name) { this.name = name; return this; }
User build() { return User(name: name, email: email); }}
2. Robot Pattern for Integration Tests
The robot pattern helps create more maintainable integration tests by encapsulating common test actions and verifications.
3. Repository Pattern
Implement repositories to abstract data sources, making it easier to swap implementations during testing.
Conclusion
Writing testable Flutter code is an investment in your application’s future. By following these best practices, you can create more reliable, maintainable, and robust applications that are easier to test and debug.
Remember, the goal isn’t just to write tests, but to write code that’s naturally testable. This approach leads to better architecture and more maintainable applications in the long run.
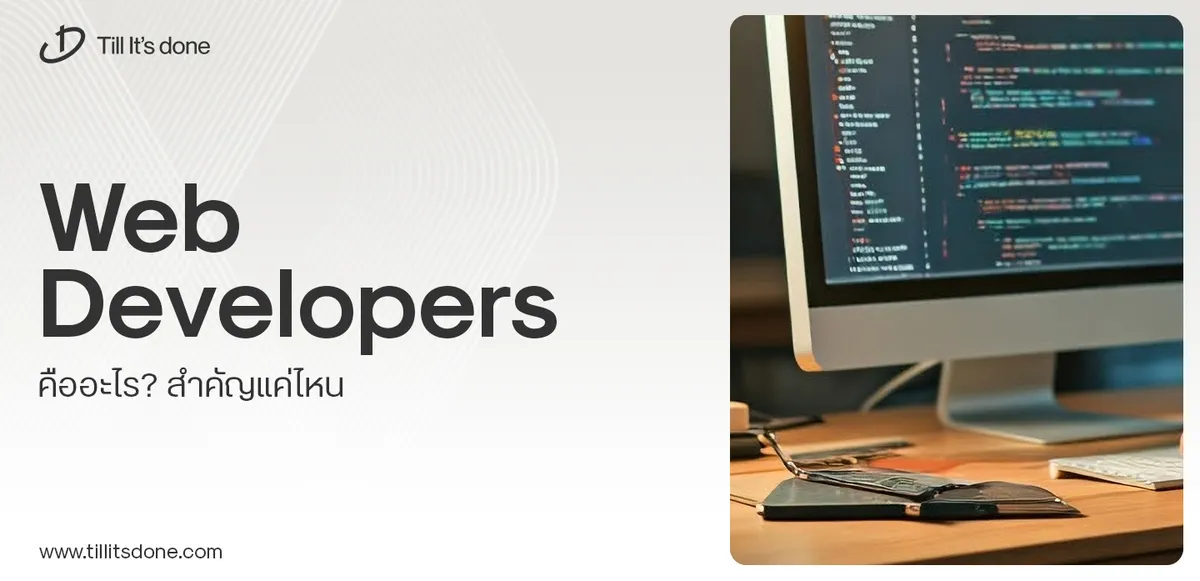
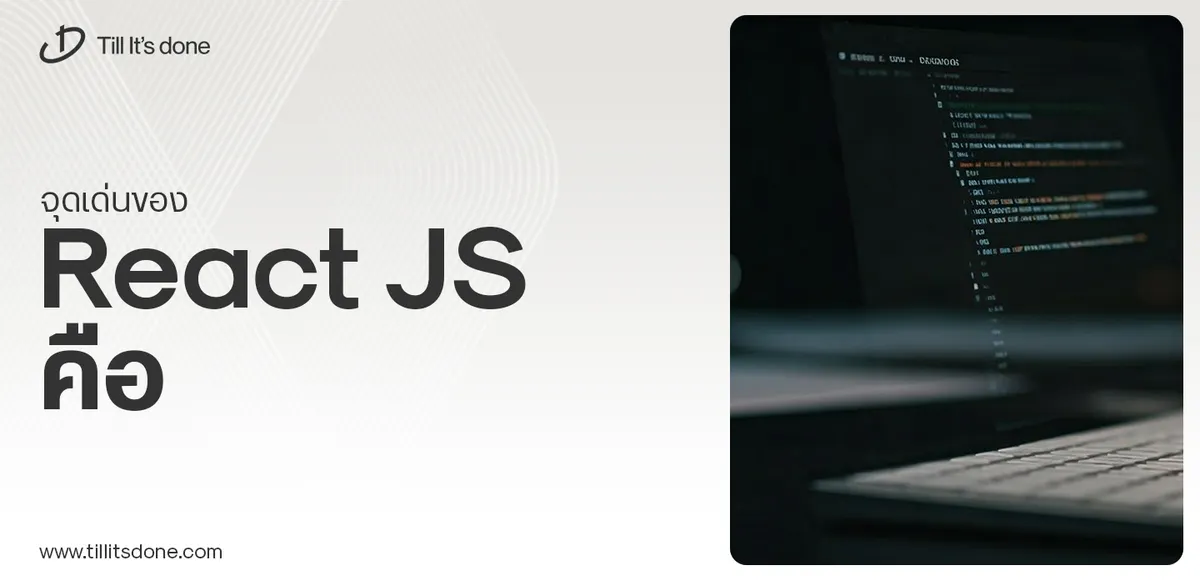
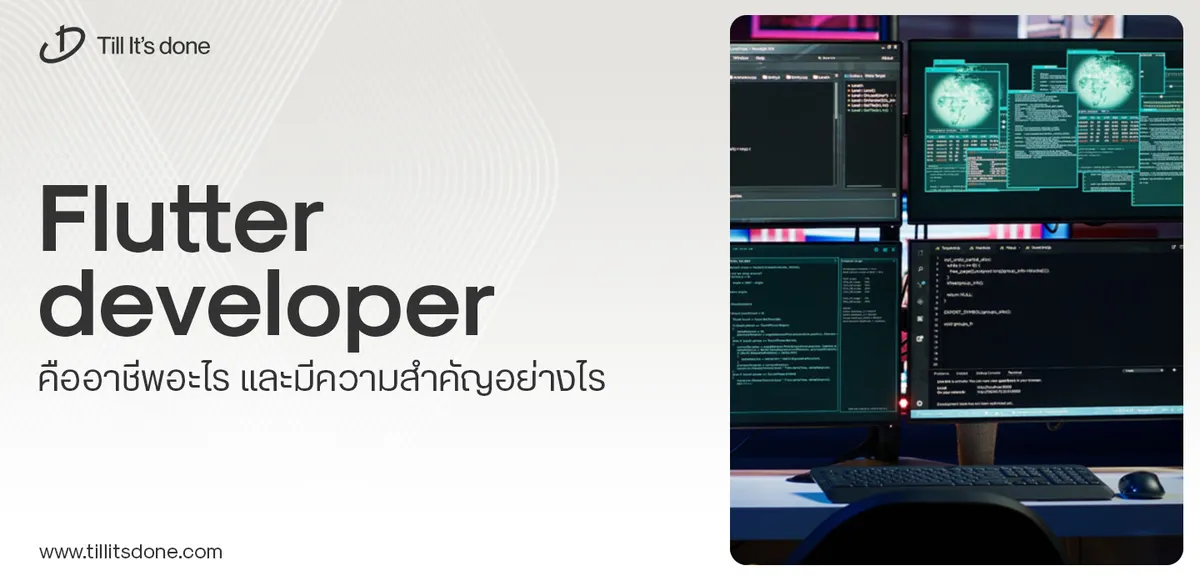
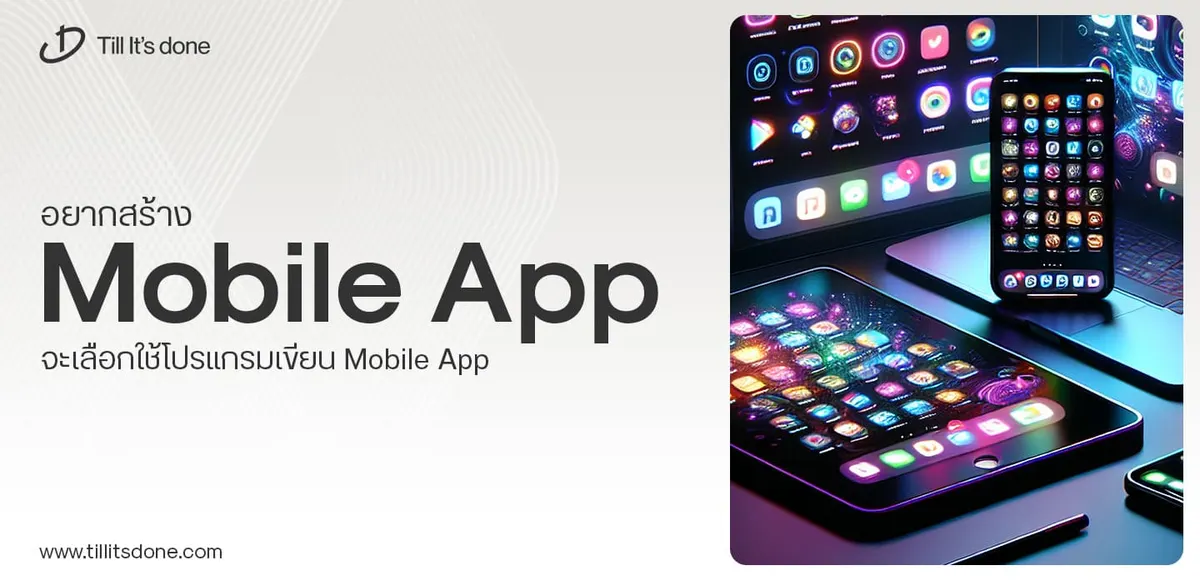
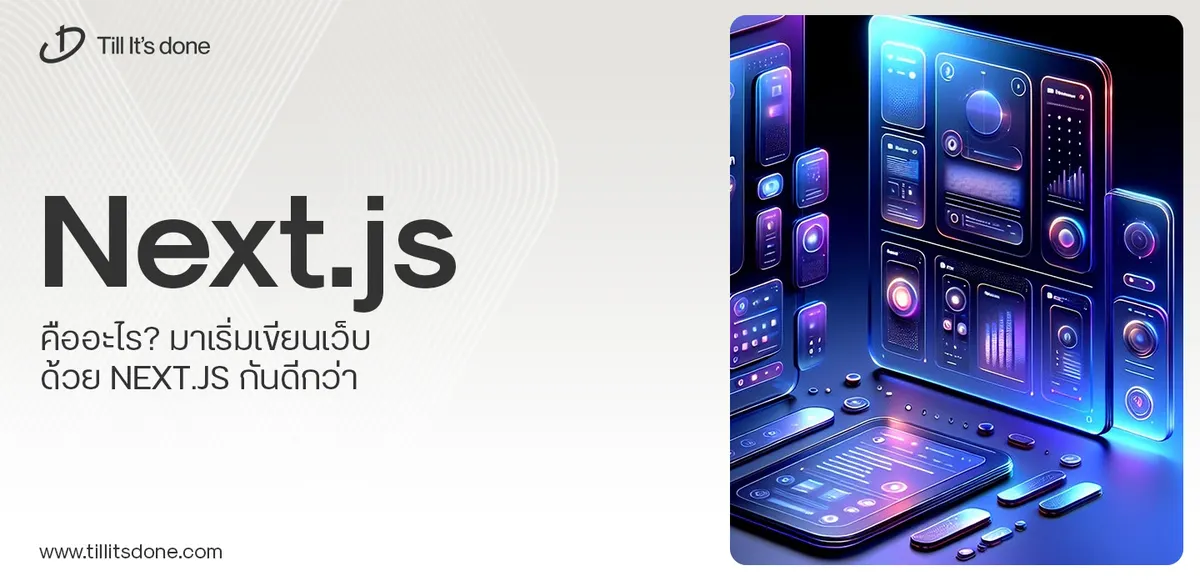
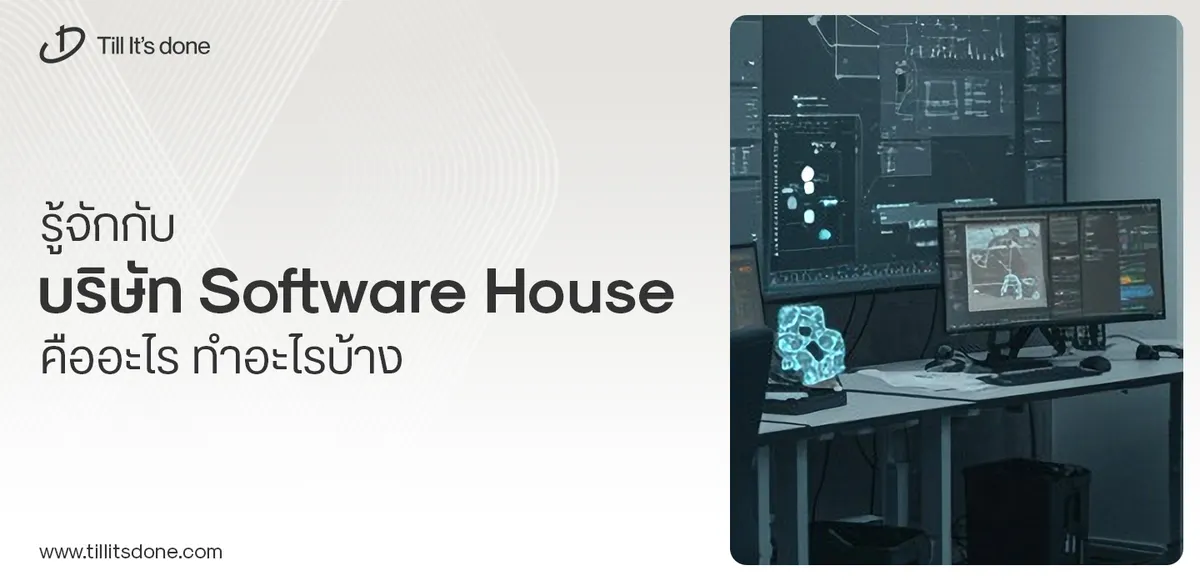
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.