- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
How to Set Up and Use Provider in Flutter App
This guide covers setup, basic concepts, and best practices for using Provider effectively in your Flutter projects.
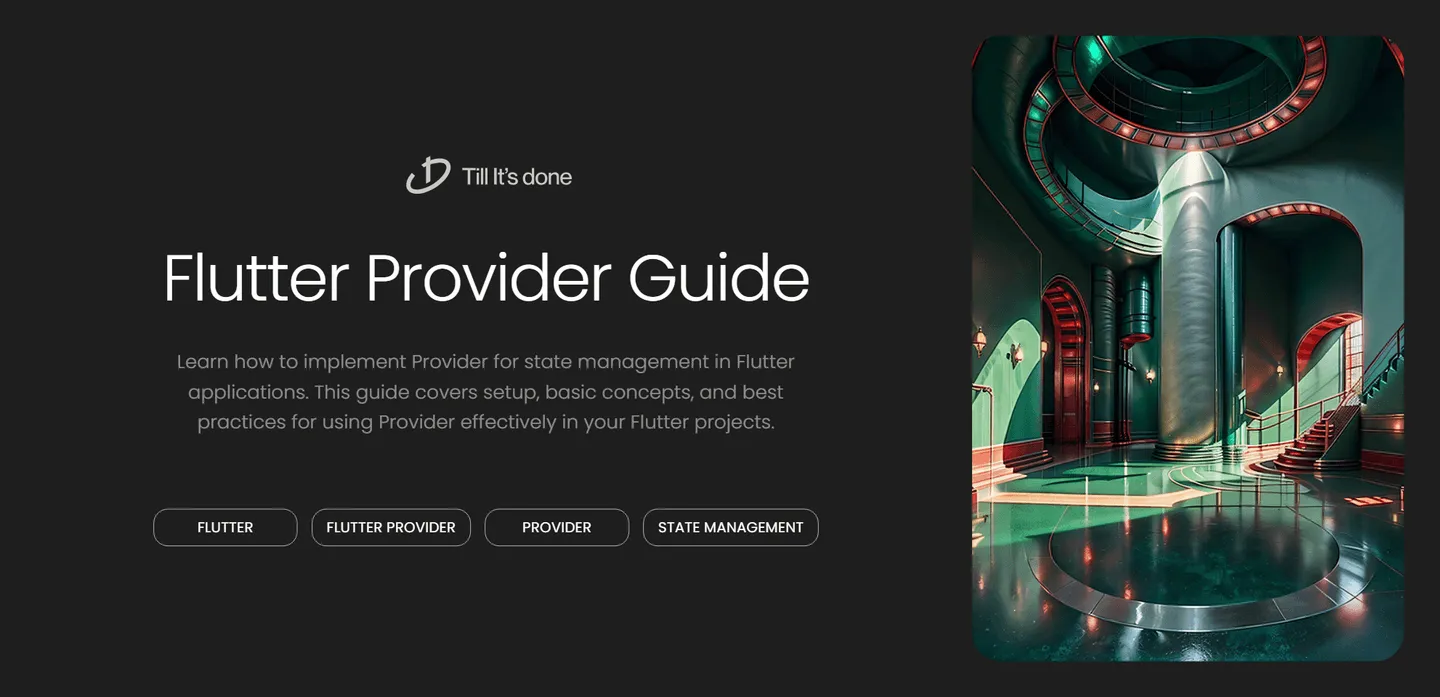
How to Set Up and Use Provider in a Flutter App
State management in Flutter can be tricky, but Provider makes it surprisingly straightforward. In this guide, we’ll walk through setting up Provider in your Flutter app and explore how to use it effectively for state management. Whether you’re building a small app or a complex project, Provider offers a clean and efficient solution that you’ll love.
Getting Started with Provider
First things first, let’s add Provider to our project. Open your pubspec.yaml
file and add the following dependency:
dependencies: provider: ^6.0.5
Run flutter pub get
, and you’re ready to roll! Provider is now available in your project.
Understanding Provider Basics
Think of Provider as a delivery service for your data. Just like how a delivery person brings packages to specific addresses, Provider delivers data to different parts of your app. It’s that simple!
Creating Your First Provider
Let’s create a simple counter example to see Provider in action. First, we’ll create a class to manage our state:
class CounterProvider extends ChangeNotifier { int _count = 0; int get count => _count;
void increment() { _count++; notifyListeners(); }}
Setting Up the Provider
Now, let’s wrap our app with a Provider. This makes our state available throughout the widget tree:
void main() { runApp( ChangeNotifierProvider( create: (_) => CounterProvider(), child: MyApp(), ), );}
Using the Provider in Widgets
Accessing your state is super easy. Here’s how you can use it in your widgets:
class CounterWidget extends StatelessWidget { @override Widget build(BuildContext context) { return Consumer<CounterProvider>( builder: (context, counter, child) { return Text('Count: ${counter.count}'); }, ); }}
Best Practices and Tips
- Always dispose of providers when they’re no longer needed
- Use
Consumer
widgets only where necessary to avoid unnecessary rebuilds - Consider using
Provider.of
for accessing data without rebuilding - Keep your provider classes focused and single-responsibility
When to Use Provider
Provider shines in many scenarios:
- Managing form data
- Handling user authentication state
- Managing shopping cart data
- Controlling theme settings
- Managing app configuration
Provider isn’t just a state management solution; it’s a powerful tool that makes your code cleaner and more maintainable. Start small, experiment, and you’ll quickly see why it’s such a popular choice in the Flutter community.
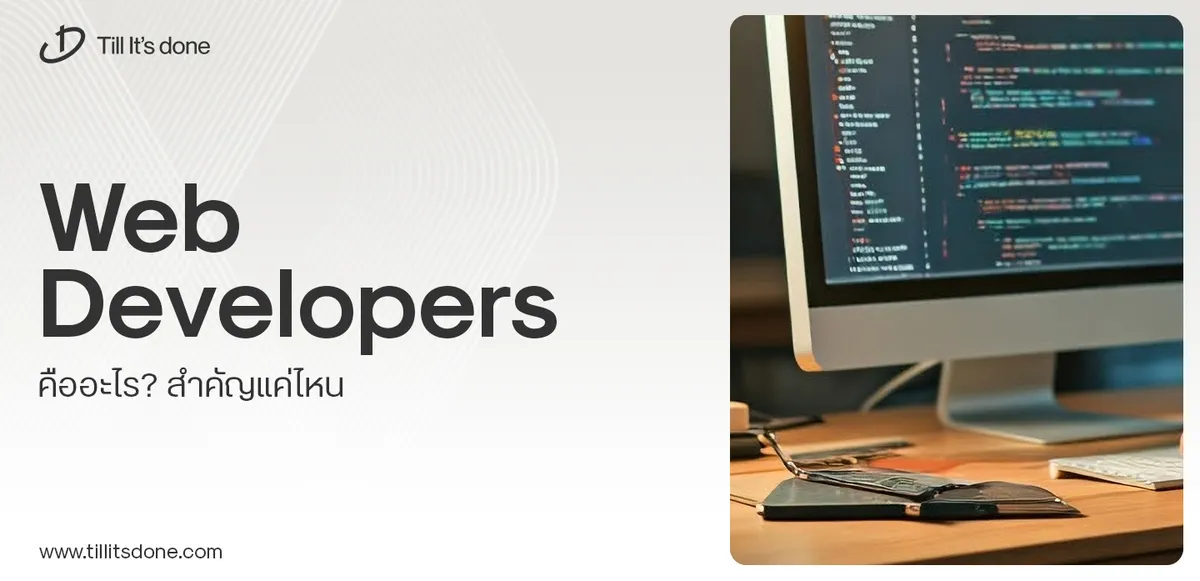
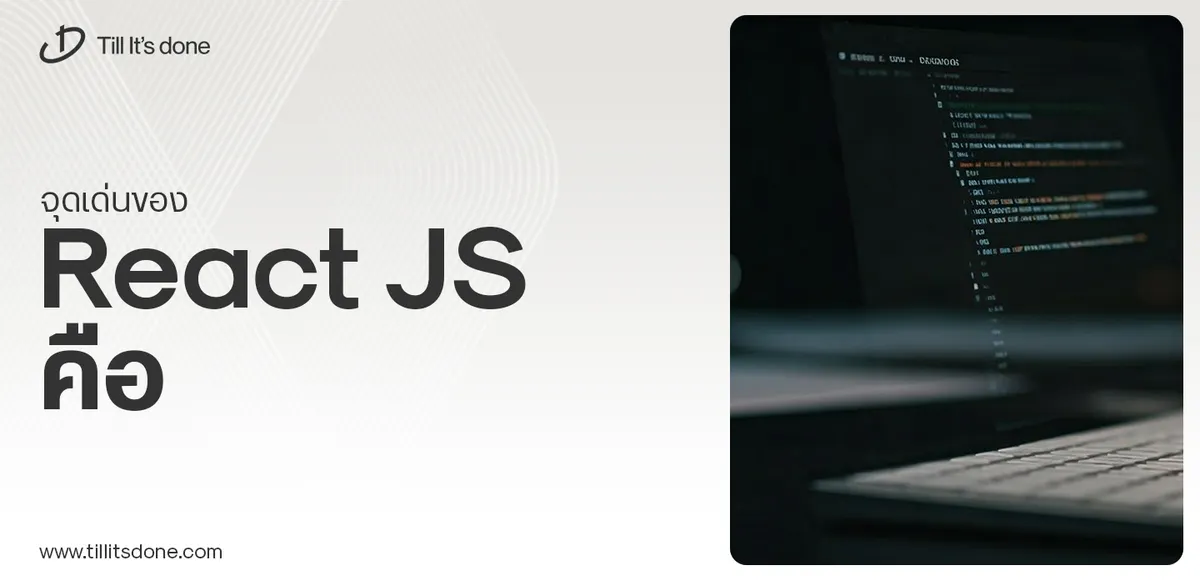
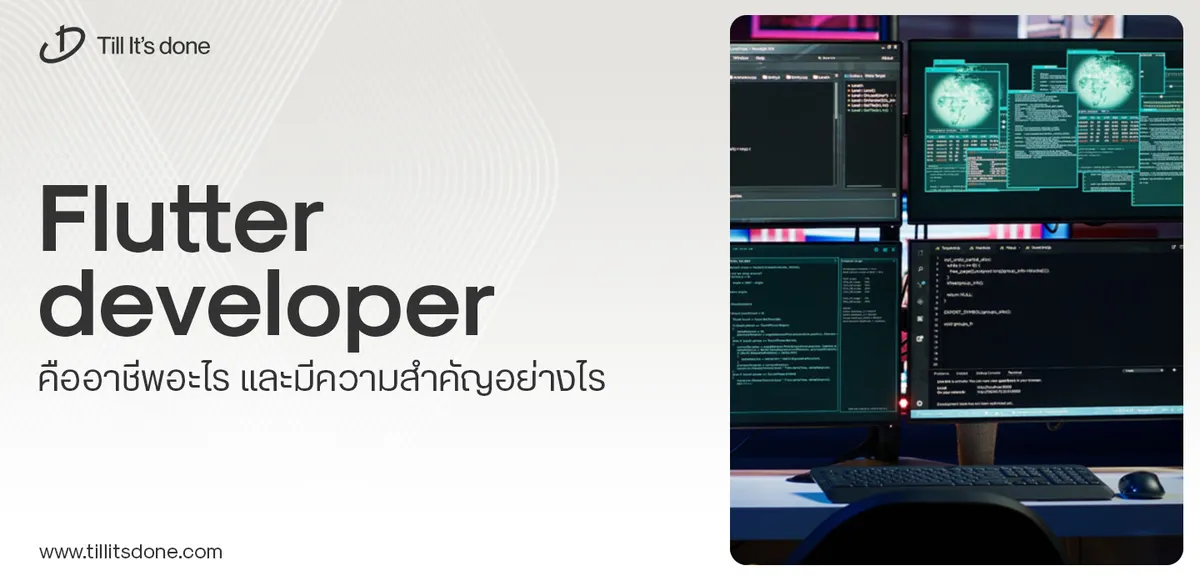
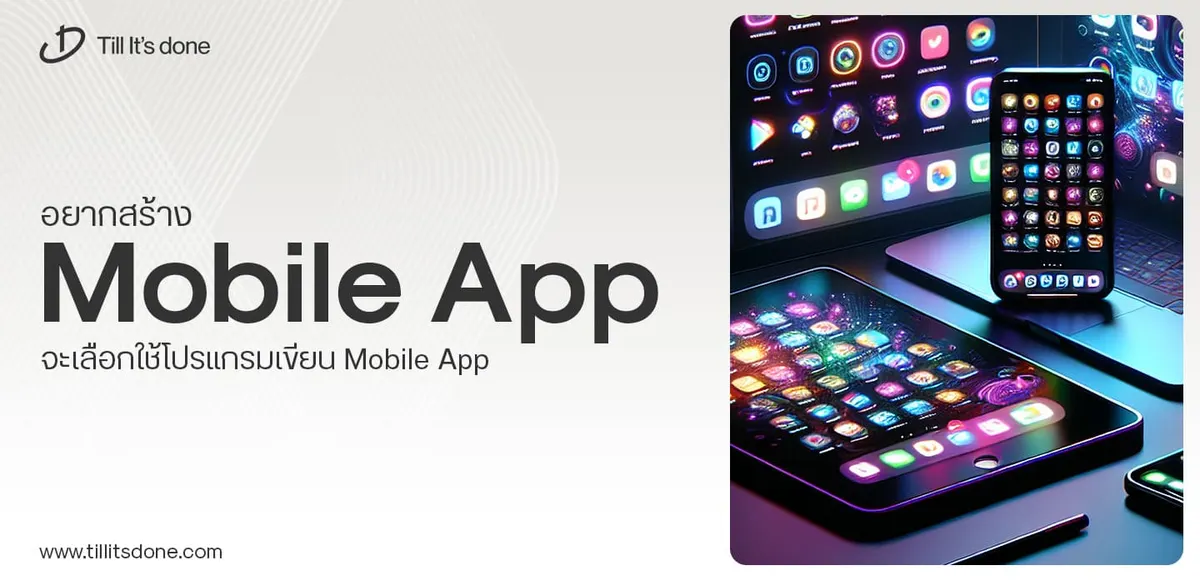
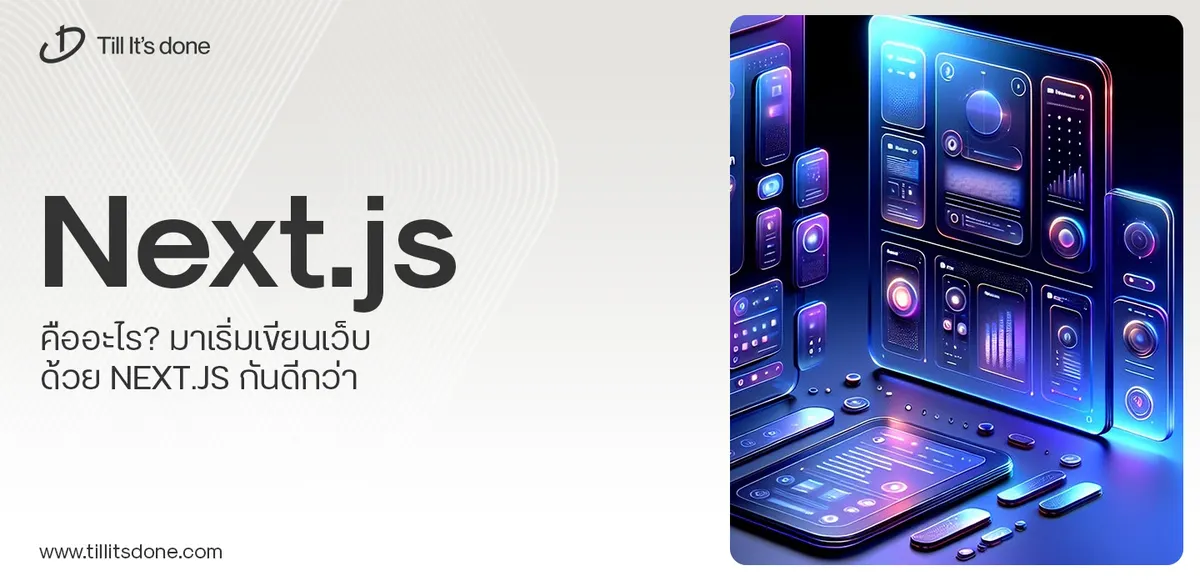
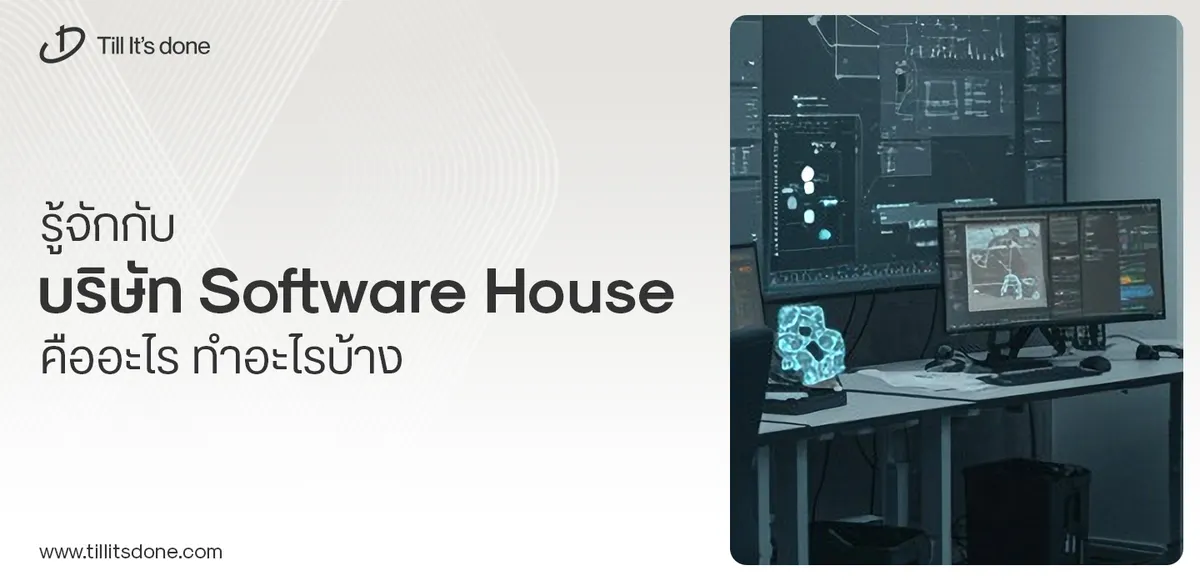
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.