- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Provider for Async Data Handling in Flutter
Discover best practices for handling loading states, error management, and implementing robust data workflows.
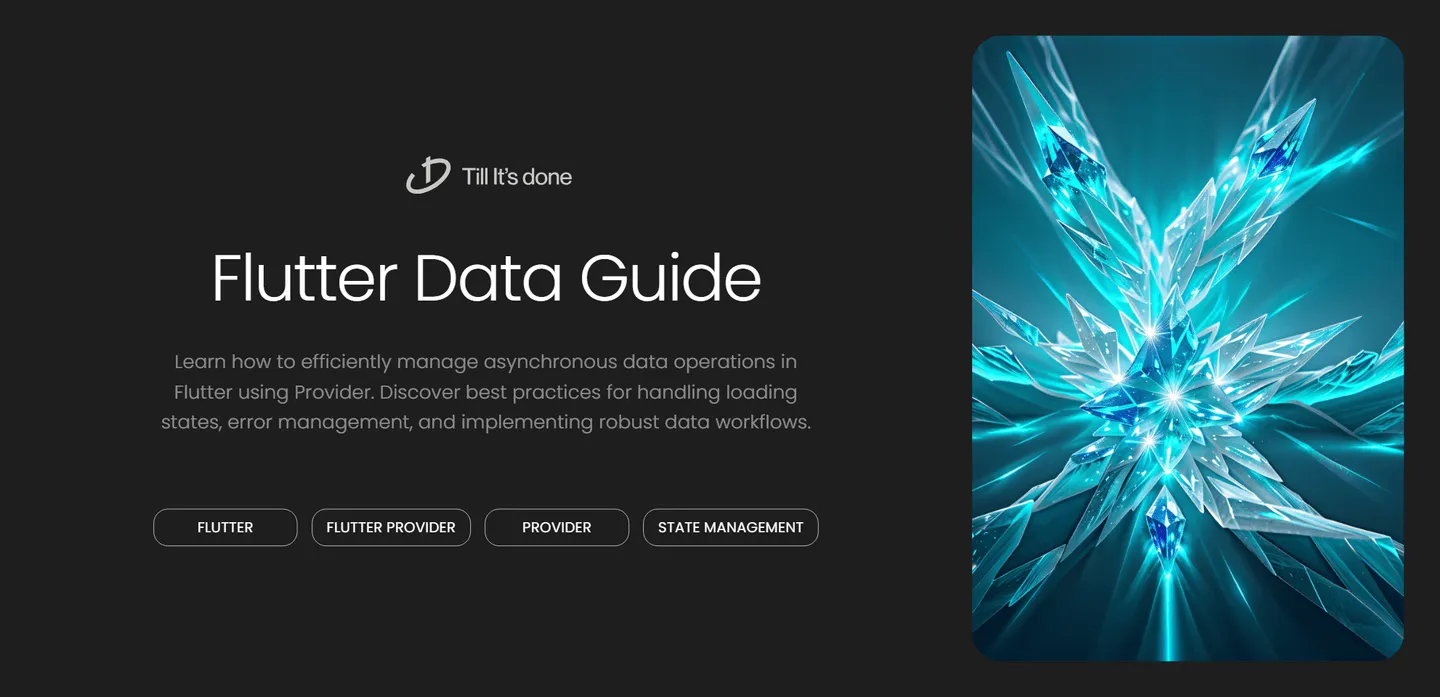
Using Provider for Asynchronous Data Handling in Flutter
Managing asynchronous data efficiently is crucial for building responsive Flutter applications. Provider, combined with Flutter’s async capabilities, offers an elegant solution for handling data operations without compromising your app’s performance. Let’s dive into how you can leverage Provider for managing async operations effectively.
Understanding the Basics
Before we delve into implementation details, it’s essential to grasp what makes Provider such a powerful tool for state management. Provider acts as a wrapper around your data models, making them accessible throughout your widget tree while maintaining clean separation of concerns.
Setting Up the Provider
The first step is creating a provider class that will handle your async operations. This class should extend ChangeNotifier to inform widgets when data changes:
class DataProvider with ChangeNotifier { List<Item> _items = []; bool _isLoading = false; String? _error;
List<Item> get items => _items; bool get isLoading => _isLoading; String? get error => _error;
Future<void> fetchData() async { _isLoading = true; _error = null; notifyListeners();
try { final response = await apiService.fetchItems(); _items = response; _isLoading = false; notifyListeners(); } catch (e) { _error = e.toString(); _isLoading = false; notifyListeners(); } }}
Implementing Loading States
One of the key aspects of handling async data is managing loading states effectively. Provider makes this straightforward by allowing you to track and expose loading states to your UI:
Consumer<DataProvider>( builder: (context, provider, child) { if (provider.isLoading) { return CircularProgressIndicator(); }
if (provider.error != null) { return ErrorWidget(provider.error!); }
return ListView.builder( itemCount: provider.items.length, itemBuilder: (context, index) { return ItemWidget(provider.items[index]); }, ); },)
Best Practices
- Always handle errors gracefully
- Implement proper loading indicators
- Use debouncing for frequent updates
- Cache data when appropriate
- Implement retry mechanisms for failed requests
Future-Proofing Your Implementation
Consider implementing a repository pattern between your Provider and API service. This additional layer of abstraction makes your code more maintainable and testable:
class Repository { final ApiService _apiService;
Future<List<Item>> fetchItems() async { try { return await _apiService.fetchItems(); } catch (e) { throw DataException('Failed to fetch items'); } }}
By following these patterns, you create a robust foundation for handling async operations in your Flutter applications. Remember to dispose of your providers properly to prevent memory leaks and always consider the user experience when implementing loading and error states.
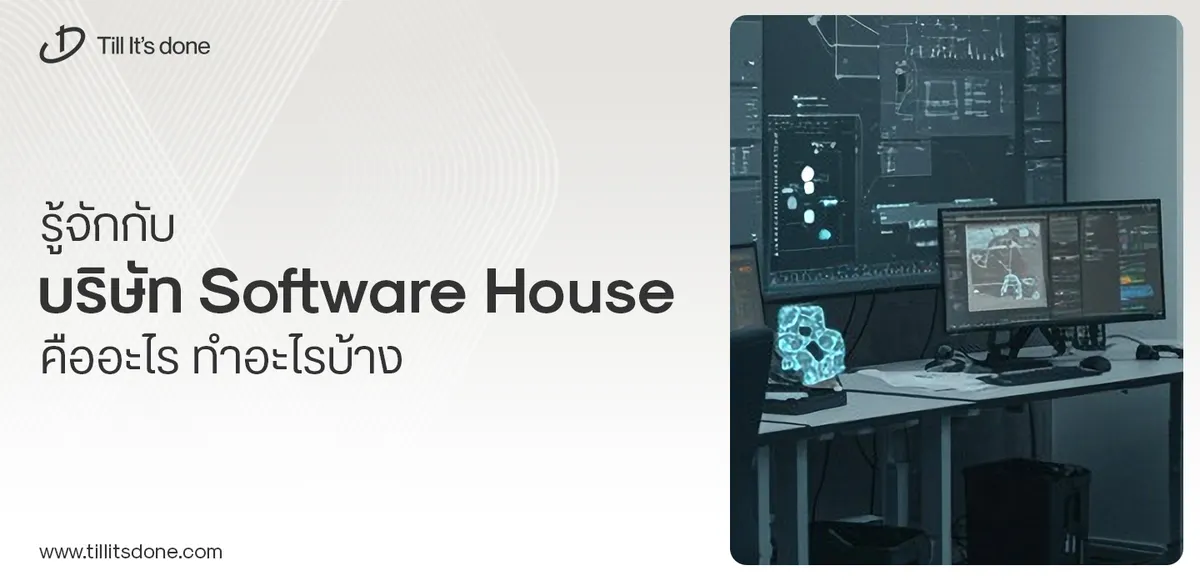
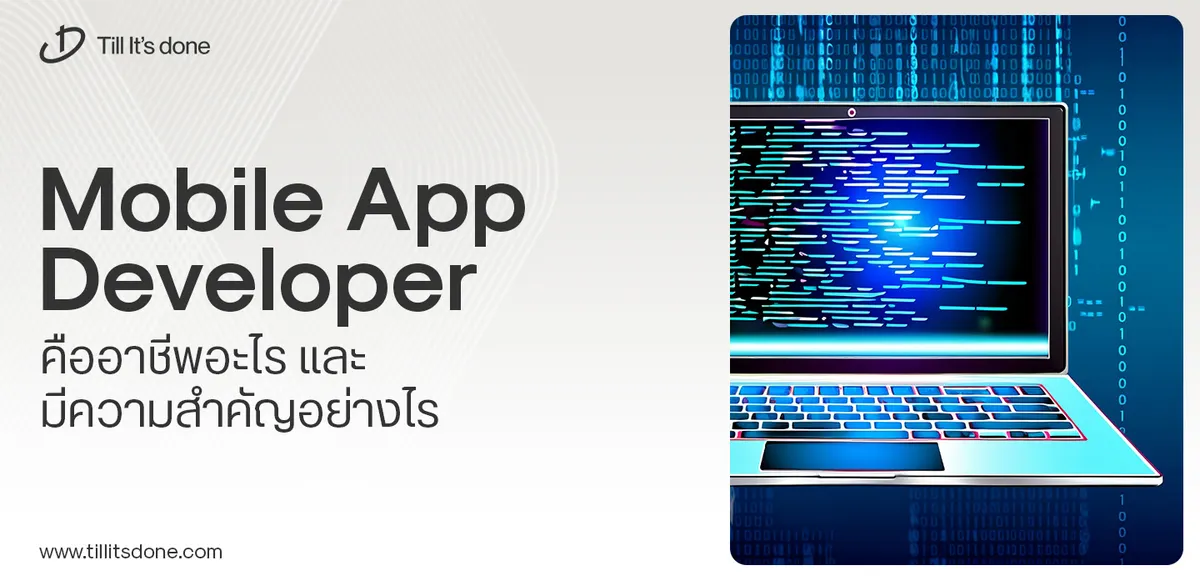
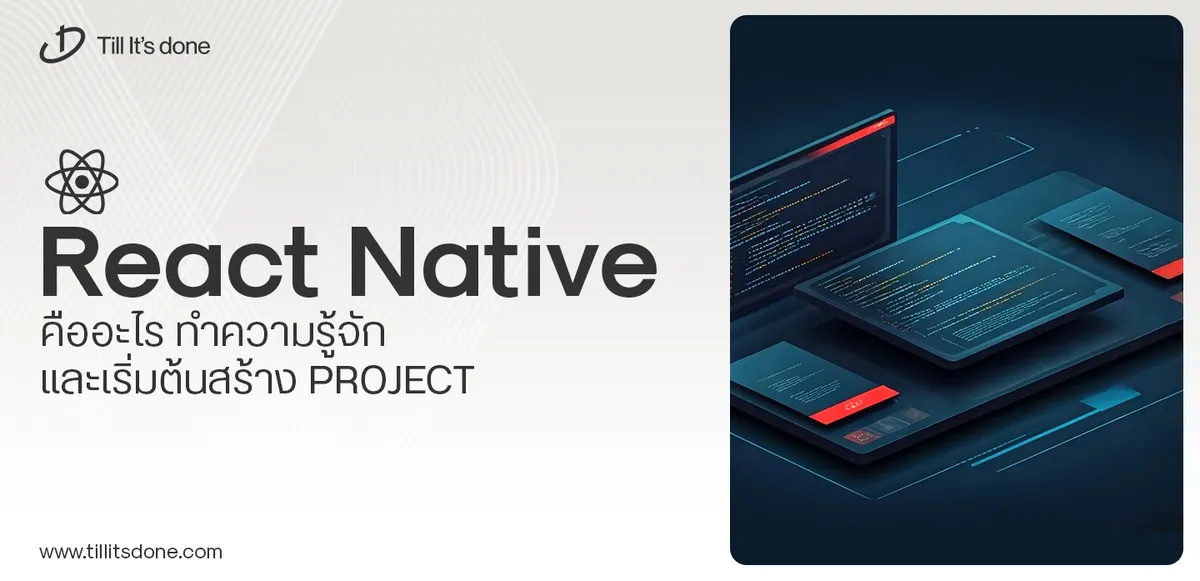
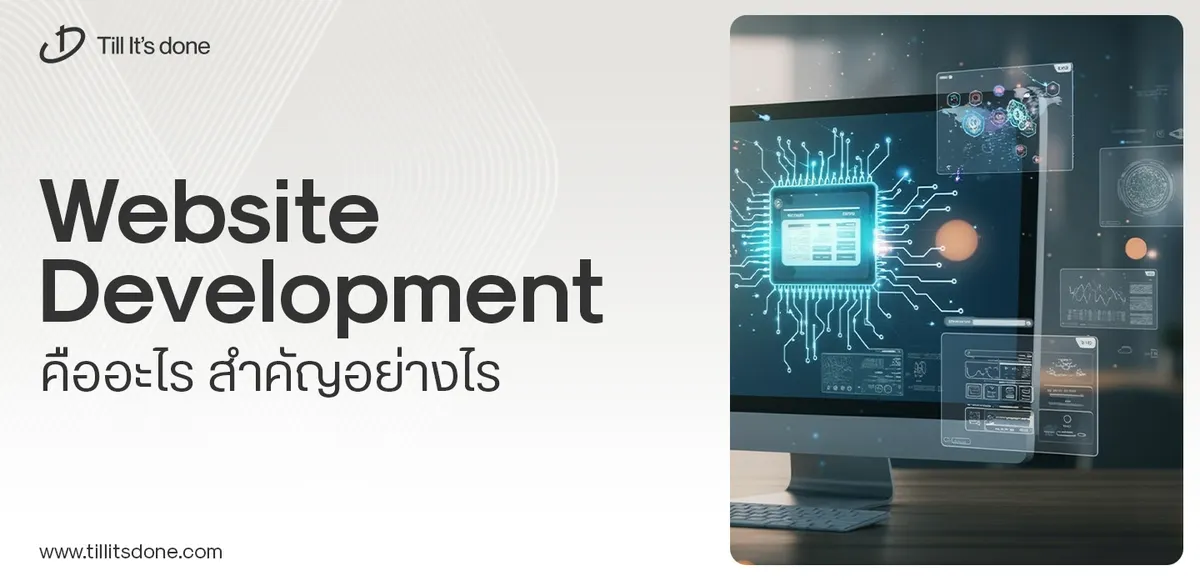
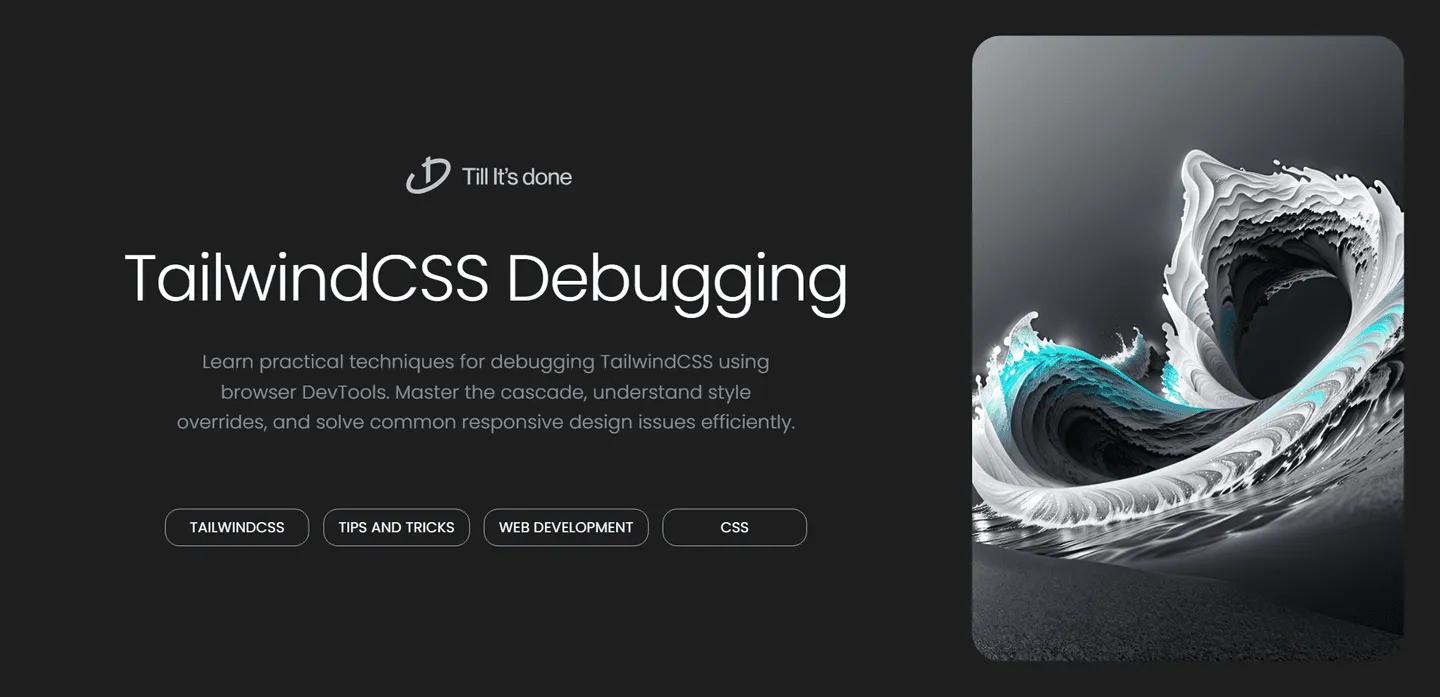
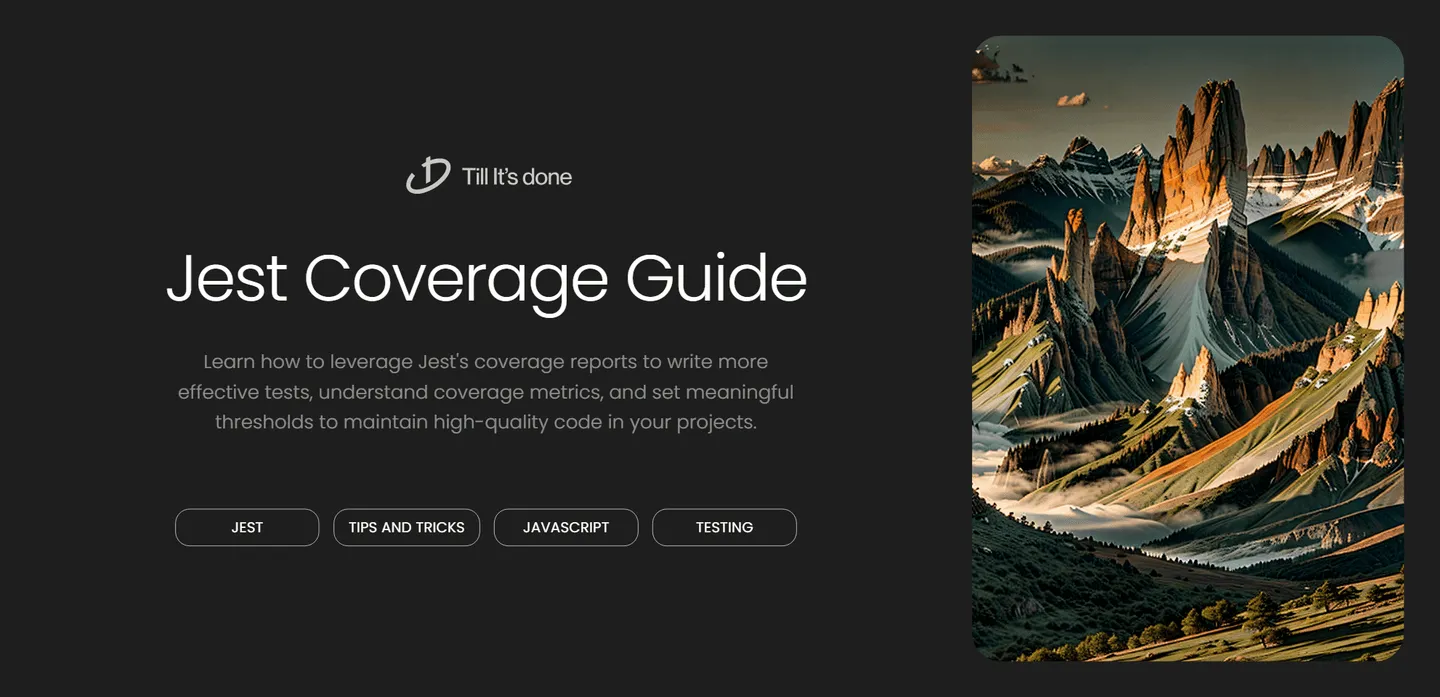
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.