- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Managing Nested State in Flutter Using Provider
Discover best practices, implementation patterns, and advanced techniques for scalable state management.
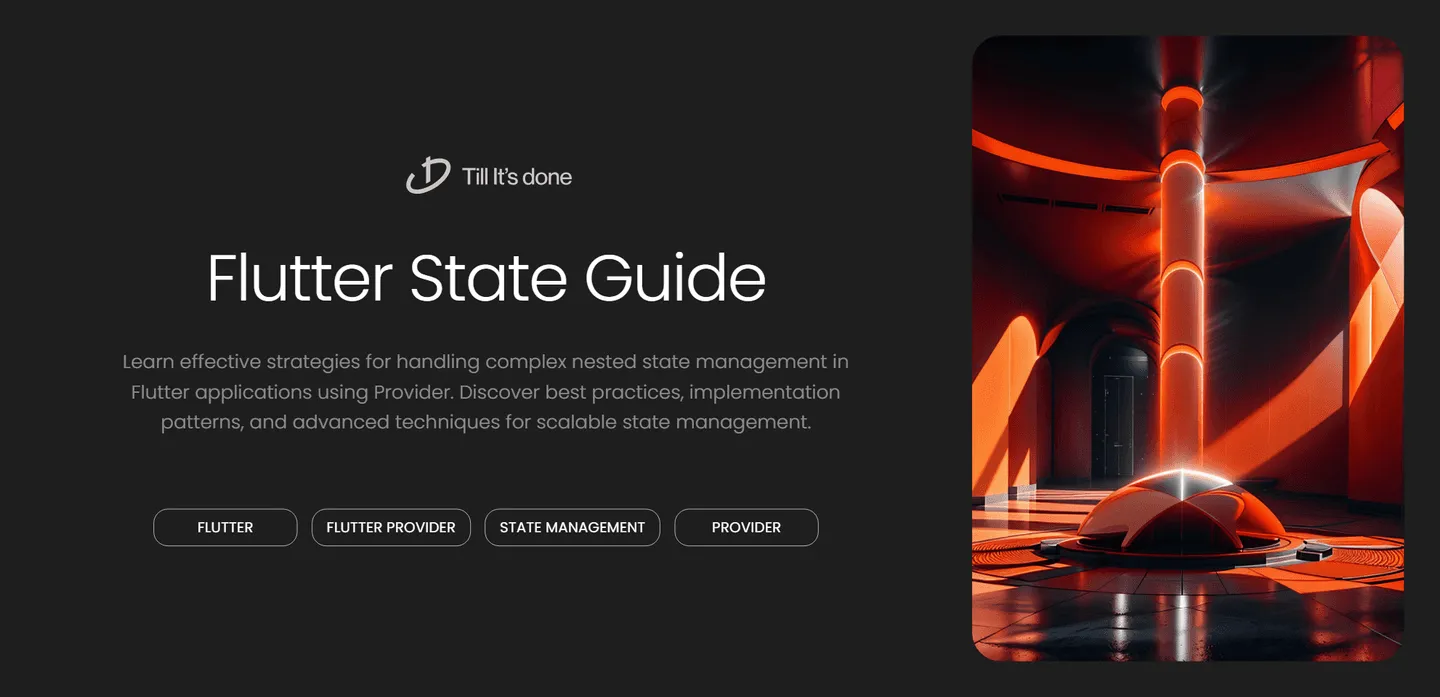
Managing state in Flutter applications becomes increasingly complex as your app grows. While Provider is a popular state management solution, handling nested state can be particularly challenging. Today, let’s explore effective strategies for managing nested state using Provider in Flutter applications.
Understanding Nested State
Imagine you’re building an e-commerce app where you need to track the shopping cart, user preferences, and product categories simultaneously. Each of these could have their own nested states – your shopping cart contains items, each with quantities and variations, while product categories might have subcategories and filters.
Best Practices for Nested Providers
1. Provider Hierarchy
One of the most effective approaches is to structure your Providers in a hierarchy that mirrors your data structure. Rather than creating one massive Provider that handles everything, break it down into logical, nested components.
MultiProvider( providers: [ ChangeNotifierProvider(create: (_) => CartProvider()), ChangeNotifierProvider(create: (_) => UserPreferencesProvider()), ChangeNotifierProxyProvider<CartProvider, OrderProvider>( create: (_) => OrderProvider(), update: (_, cart, previous) => previous!..updateCart(cart), ), ], child: MyApp(),)
2. Implementing Sub-Providers
When dealing with complex nested state, create dedicated Providers for each major feature area. This approach improves maintainability and makes your code more modular.
3. State Updates and Communication
Remember to implement proper update mechanisms between your nested Providers. Use ProxyProvider when a child Provider needs to react to changes in its parent:
class CategoryProvider extends ChangeNotifier { List<Category> _categories = []; List<SubCategory> _subCategories = [];
void updateCategories() { // Update logic here notifyListeners(); }}
Advanced Techniques
Selective Rebuilds
One common pitfall with nested state is unnecessary rebuilds. Use Consumer widgets strategically to rebuild only the parts of your UI that depend on specific pieces of state:
Consumer<CartProvider>( builder: (context, cart, child) { return Consumer<ProductProvider>( builder: (context, products, child) { return ProductGrid( cart: cart, products: products, ); }, ); },)
Managing Side Effects
When working with nested state, side effects can become tricky. Consider using ProxyProvider to manage dependencies between different parts of your state tree effectively.
Conclusion
Managing nested state in Flutter using Provider doesn’t have to be overwhelming. By following these patterns and best practices, you can create maintainable and scalable state management solutions for your Flutter applications.
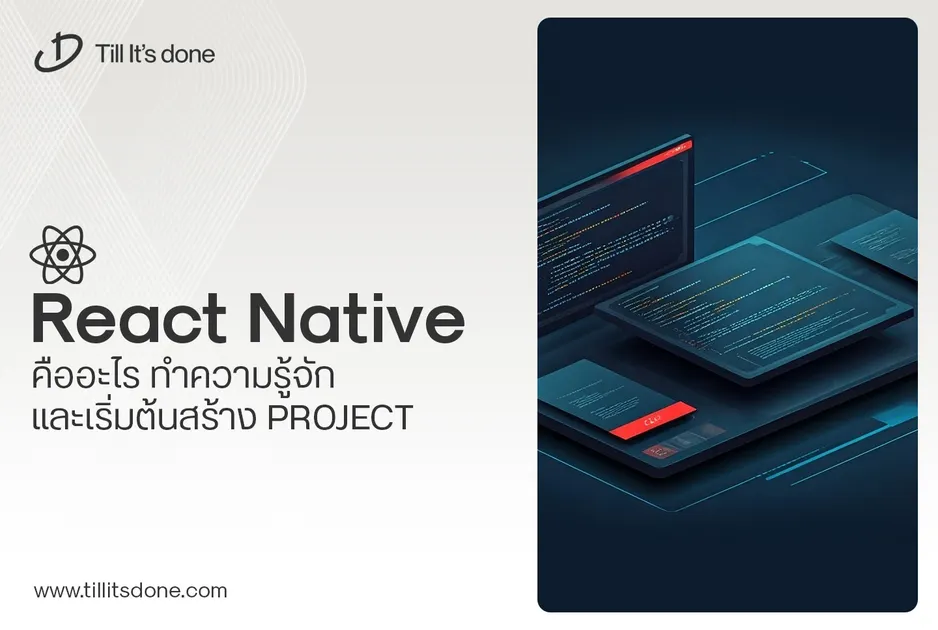
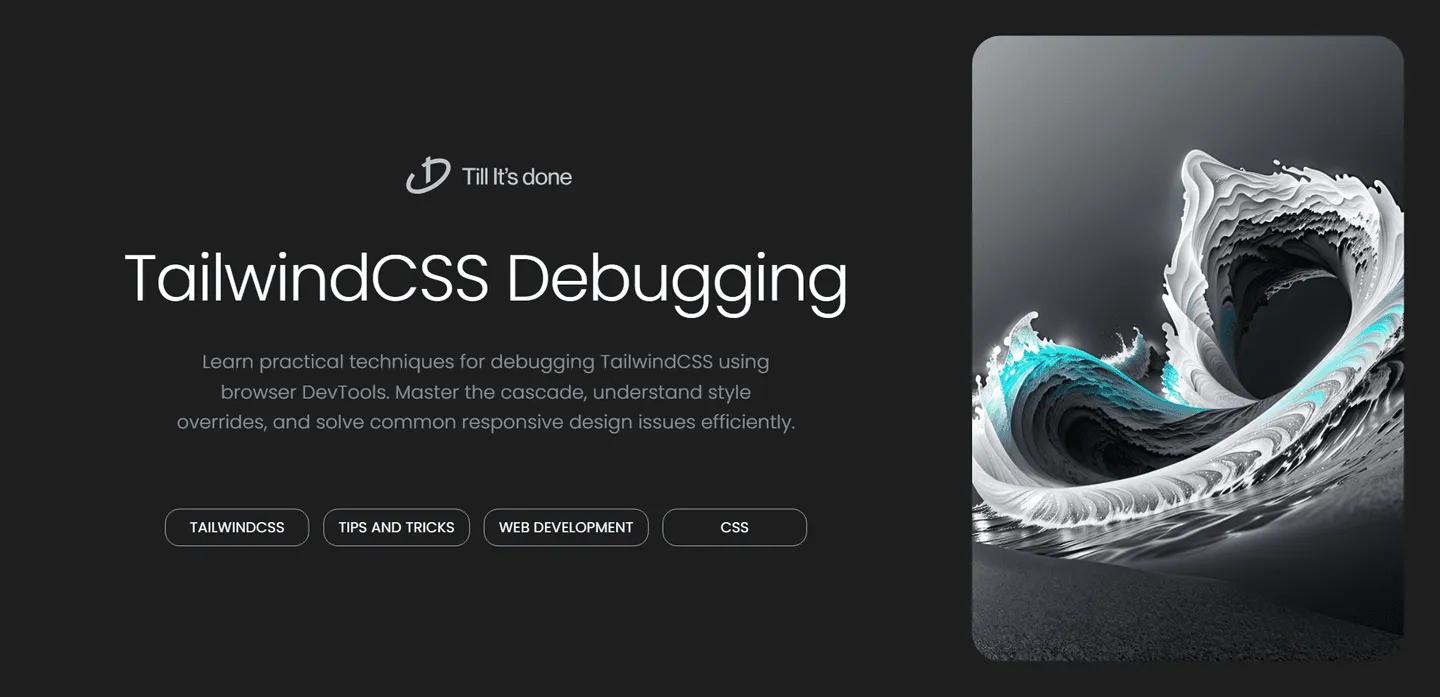
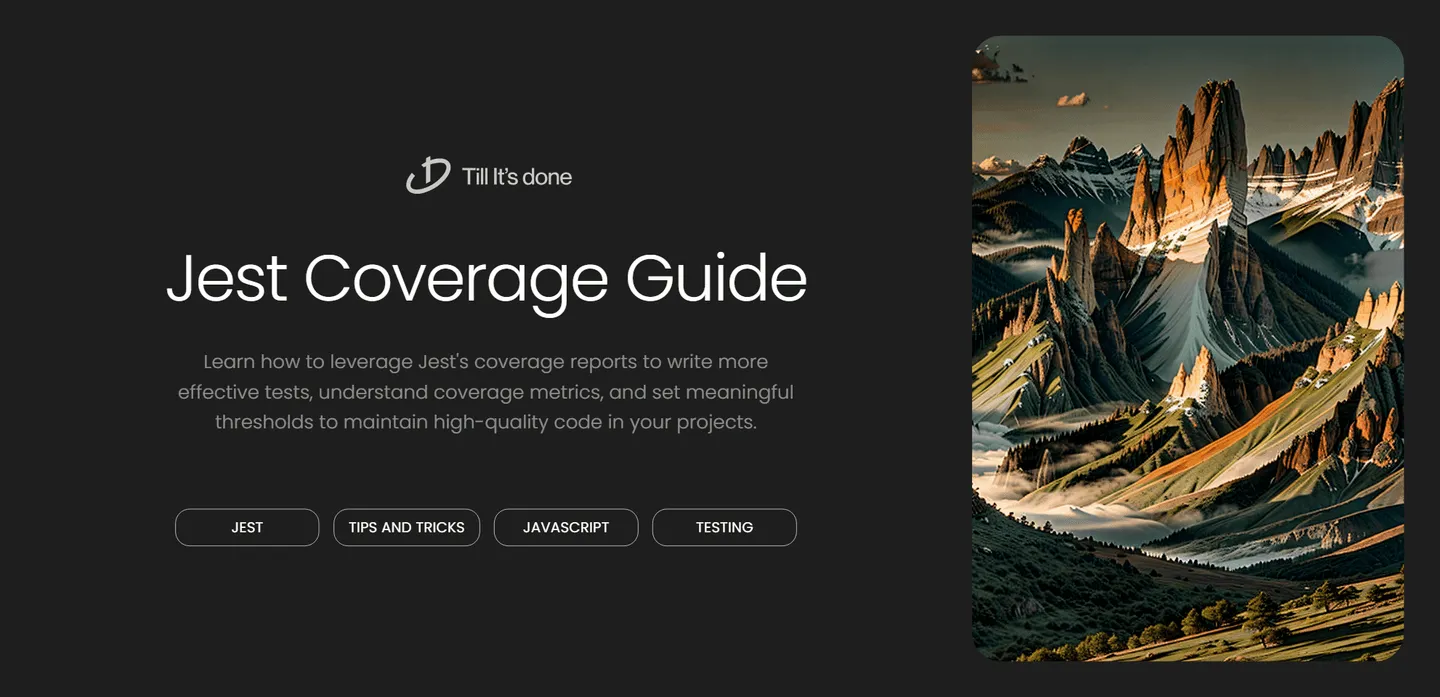
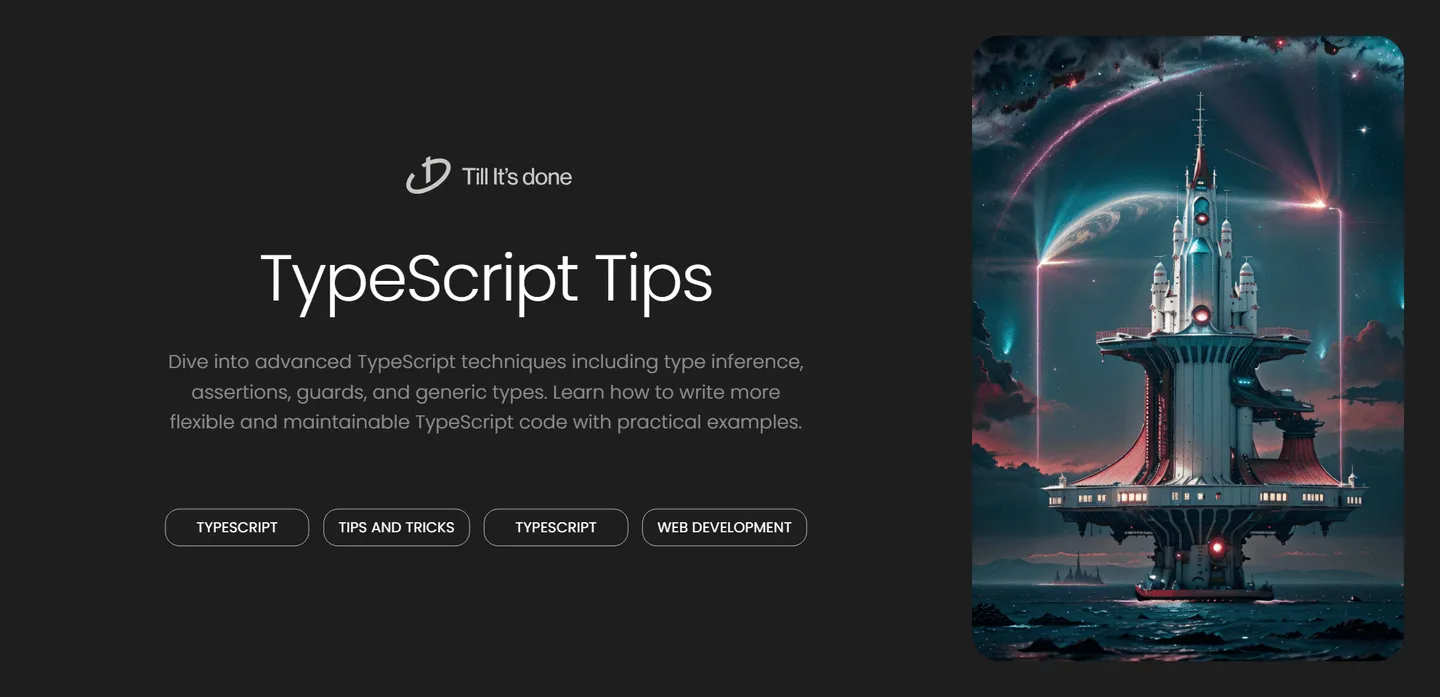
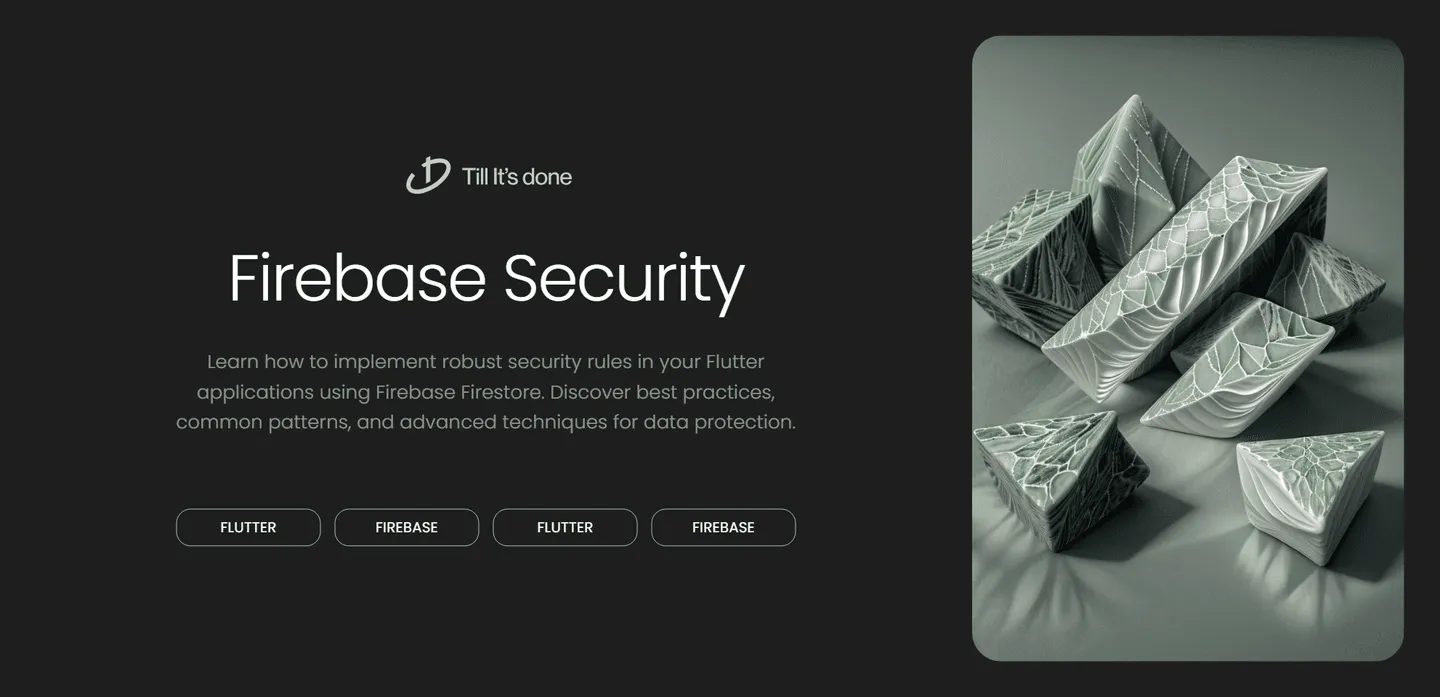
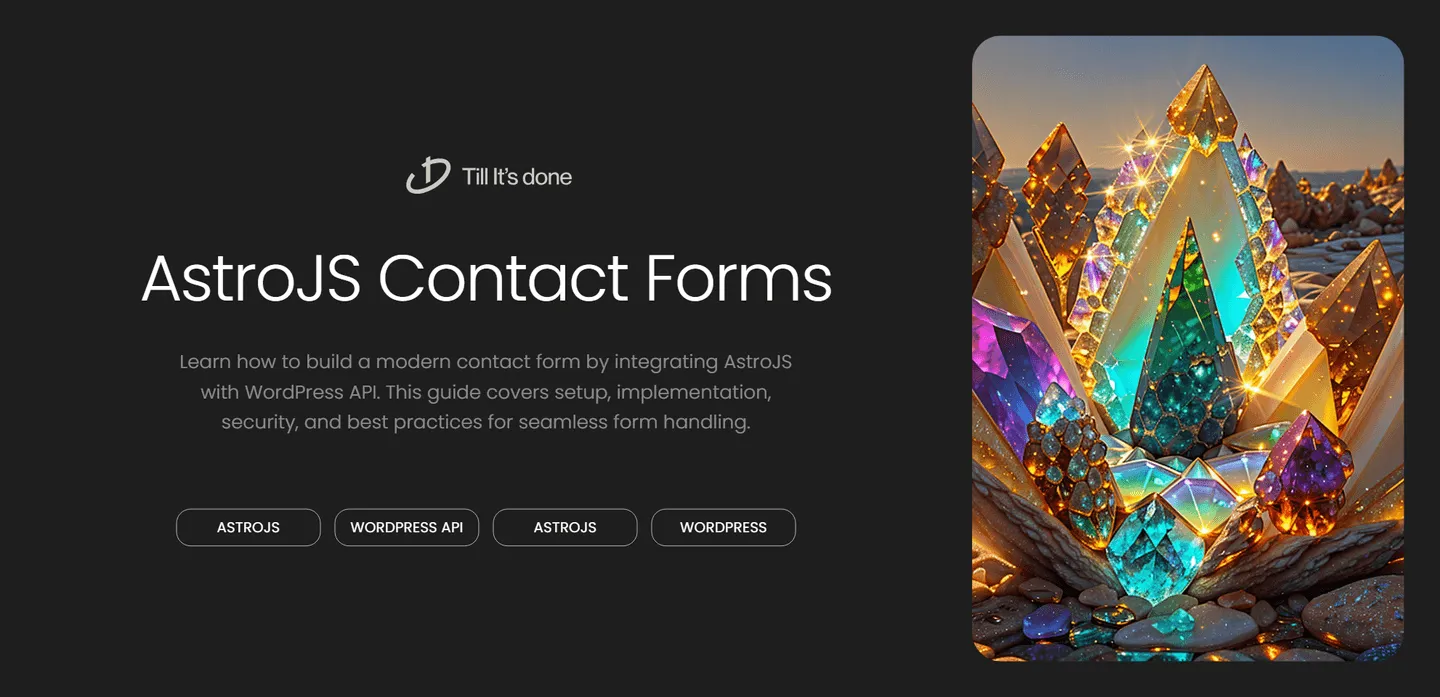
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.