- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Understanding Flutter's Integration Testing Framework
Discover best practices and common testing scenarios for robust apps.
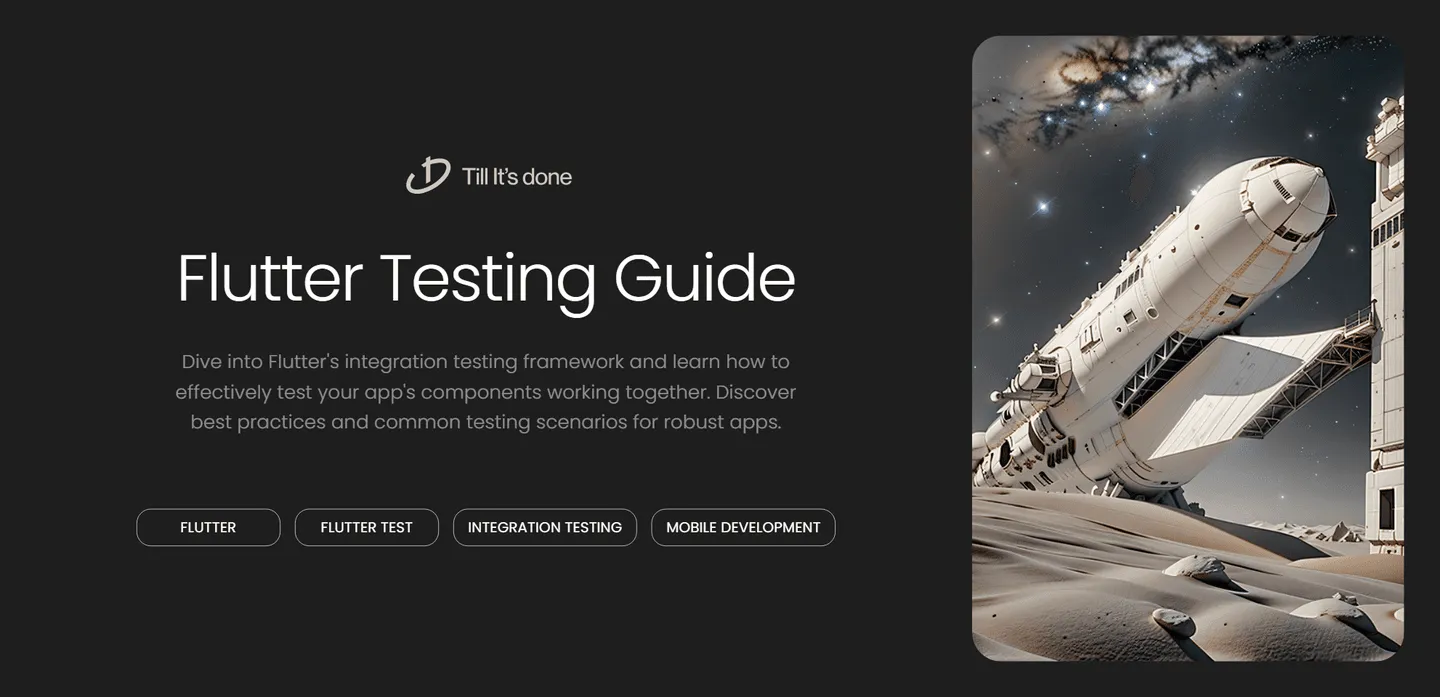
Understanding Flutter’s Integration Testing Framework
Integration testing is a crucial aspect of mobile app development that ensures your application works seamlessly across different components. Flutter’s integration testing framework provides a robust solution for testing your app’s functionality from end to end. Let’s dive deep into understanding how to leverage this powerful testing framework effectively.
What is Integration Testing?
Integration testing is the phase where individual software modules are combined and tested as a group. Unlike unit tests that focus on isolated components, integration tests verify that different parts of your application work together as expected. In Flutter, this means testing how your widgets, services, and state management solutions interact in real-world scenarios.
Setting Up Integration Tests in Flutter
Before writing your first integration test, you’ll need to set up your testing environment. First, add the integration_test package to your pubspec.yaml file’s dev_dependencies:
dev_dependencies: integration_test: sdk: flutter flutter_test: sdk: flutter
Create a new directory called integration_test
in your project root and add your test files there. This is where all your integration tests will live.
Writing Your First Integration Test
Integration tests in Flutter typically follow a pattern of arranging the test environment, performing actions, and verifying the results. Here’s a basic structure:
void main() { IntegrationTestWidgetsFlutterBinding.ensureInitialized();
testWidgets('Counter increments smoke test', (WidgetTester tester) async { await tester.pumpWidget(MyApp()); await tester.pumpAndSettle();
// Find and verify initial state expect(find.text('0'), findsOneWidget);
// Perform test actions await tester.tap(find.byIcon(Icons.add)); await tester.pumpAndSettle();
// Verify results expect(find.text('1'), findsOneWidget); });}
Best Practices for Integration Testing
-
Test Real User Flows: Focus on testing complete user journeys rather than isolated features. This ensures your app works as expected from a user’s perspective.
-
Maintain Test Independence: Each test should be independent and not rely on the state from previous tests. This makes tests more reliable and easier to debug.
-
Handle Asynchronous Operations: Use
pumpAndSettle()
orpump()
appropriately to handle animations and async operations in your tests. -
Group Related Tests: Use
group()
to organize related tests together, making your test suite more maintainable.
Common Integration Testing Scenarios
Navigation Testing
testWidgets('Test navigation flow', (tester) async { await tester.pumpWidget(MyApp()); await tester.tap(find.byType(ElevatedButton)); await tester.pumpAndSettle(); expect(find.text('Second Screen'), findsOneWidget);});
Form Submission Testing
testWidgets('Test form submission', (tester) async { await tester.pumpWidget(MyApp()); await tester.enterText(find.byType(TextField), 'test@example.com'); await tester.tap(find.byType(ElevatedButton)); await tester.pumpAndSettle(); expect(find.text('Success'), findsOneWidget);});
Running Integration Tests
To run your integration tests, use the following command:
flutter test integration_test
You can also run tests on specific devices or simulators:
flutter test integration_test/app_test.dart -d <device-id>
Remember that integration tests are an essential part of your testing strategy, but they shouldn’t be your only testing approach. Combine them with unit tests and widget tests for comprehensive test coverage. Happy testing!
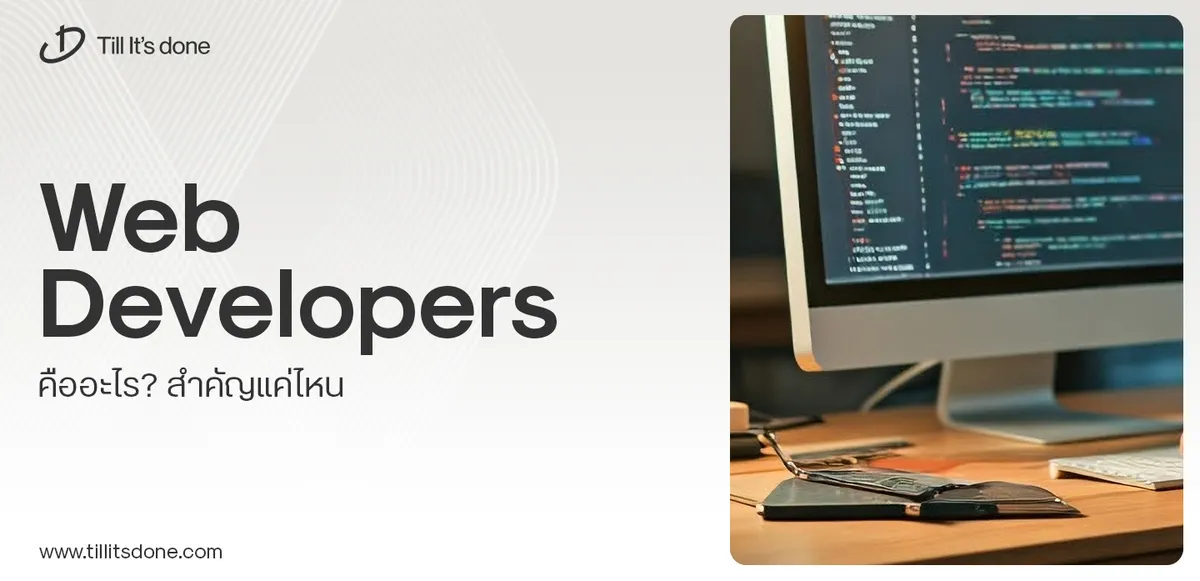
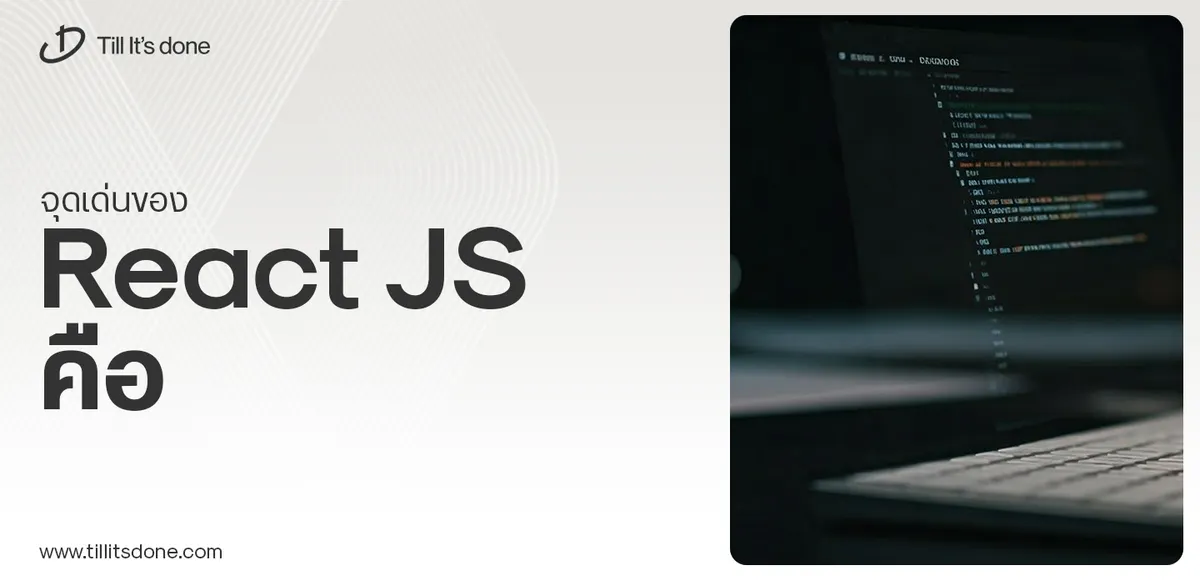
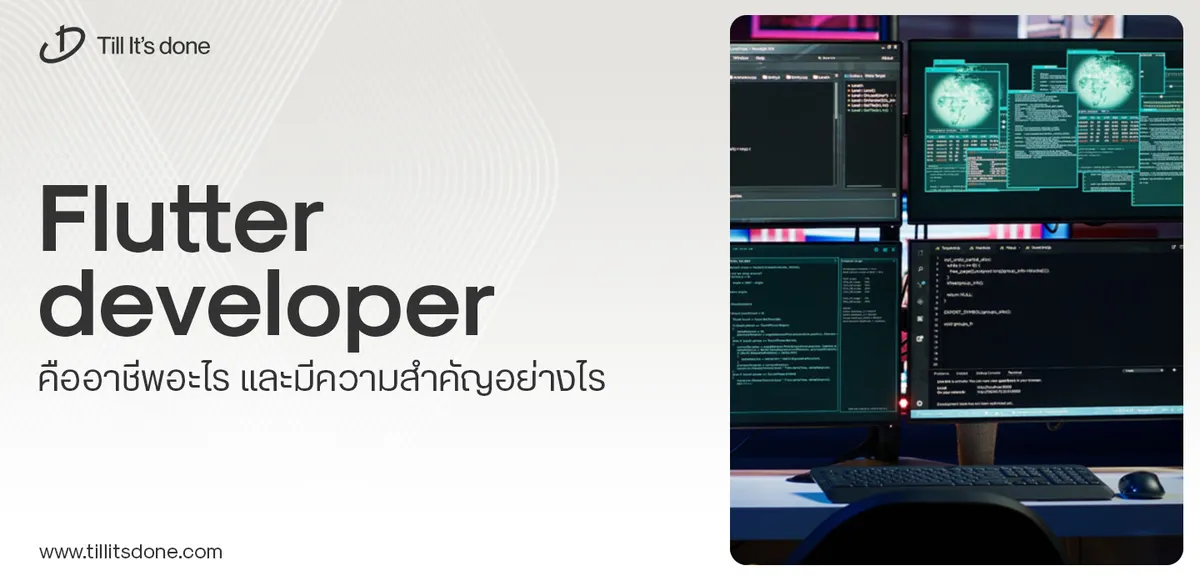
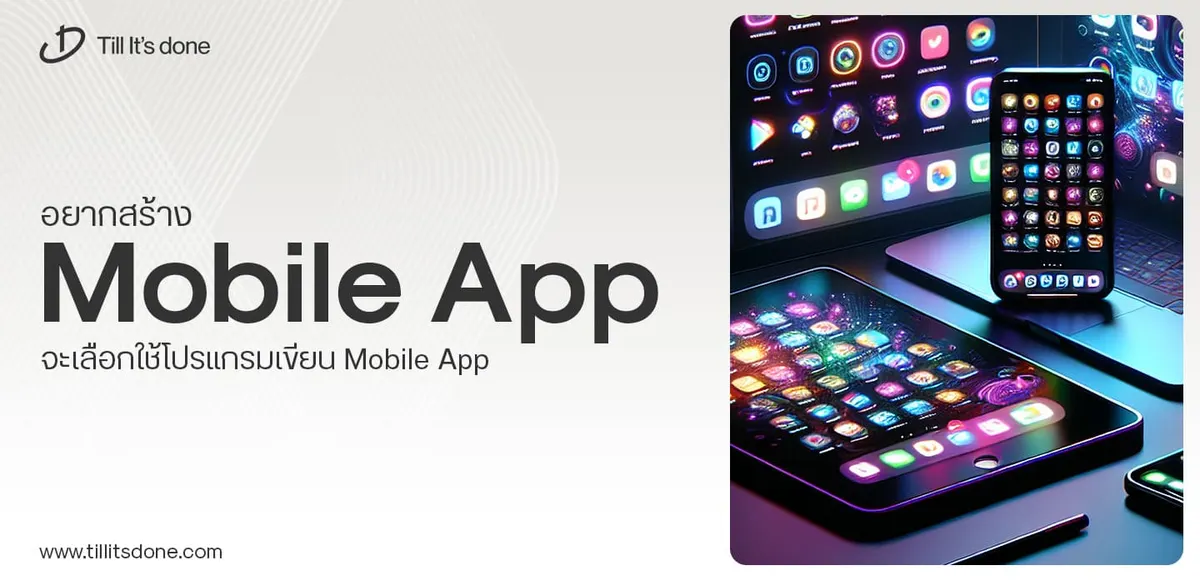
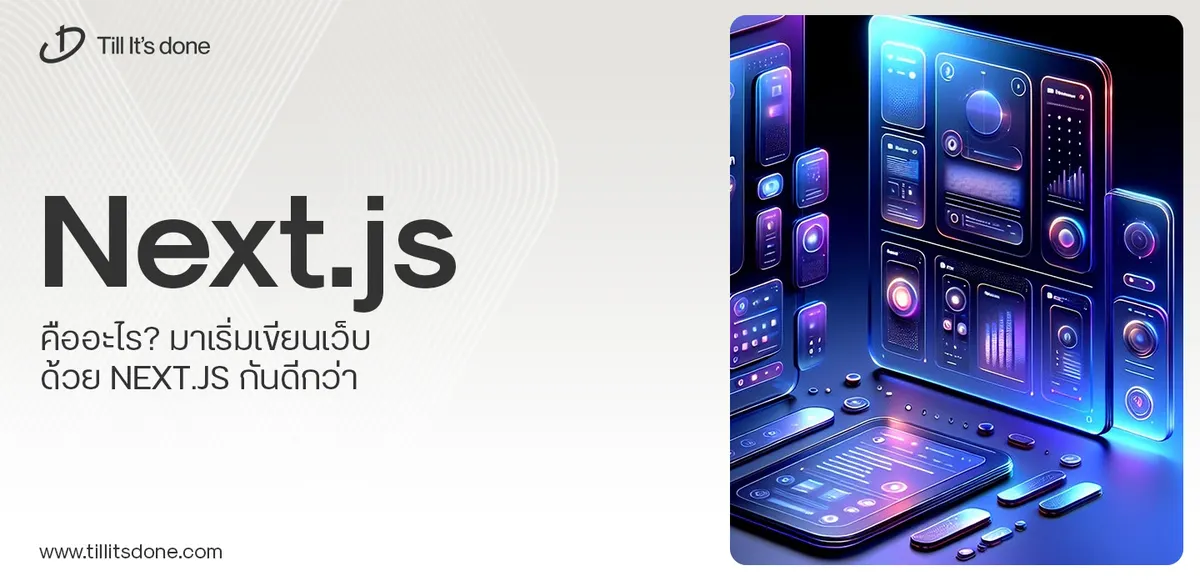
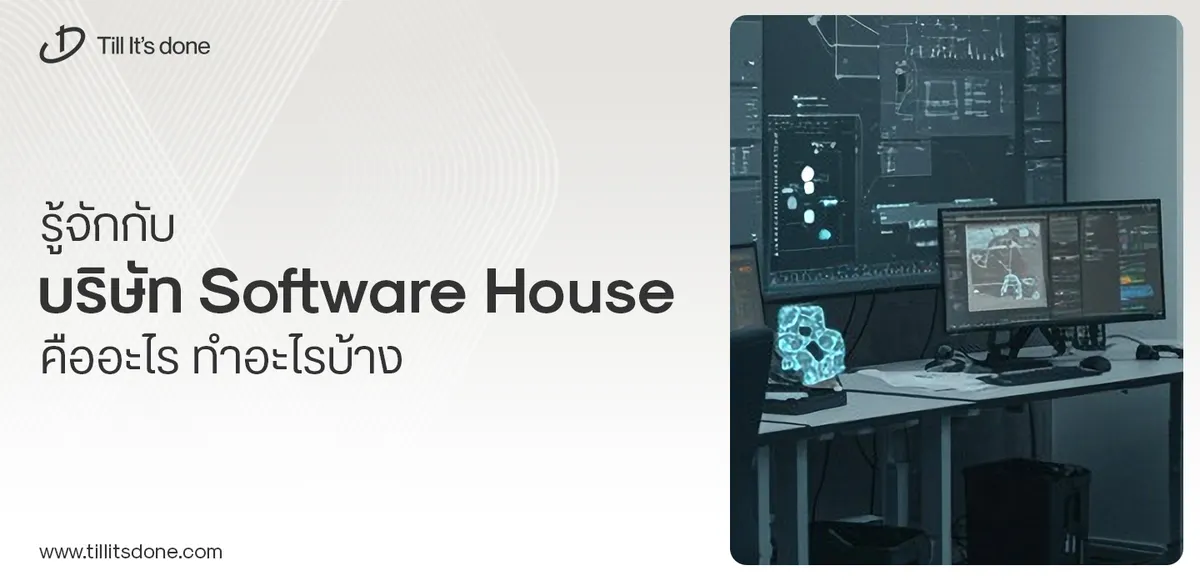
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.