- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Building Offline Apps with Flutter & Firebase
Master data persistence, smart caching strategies, and best practices for offline-first development.
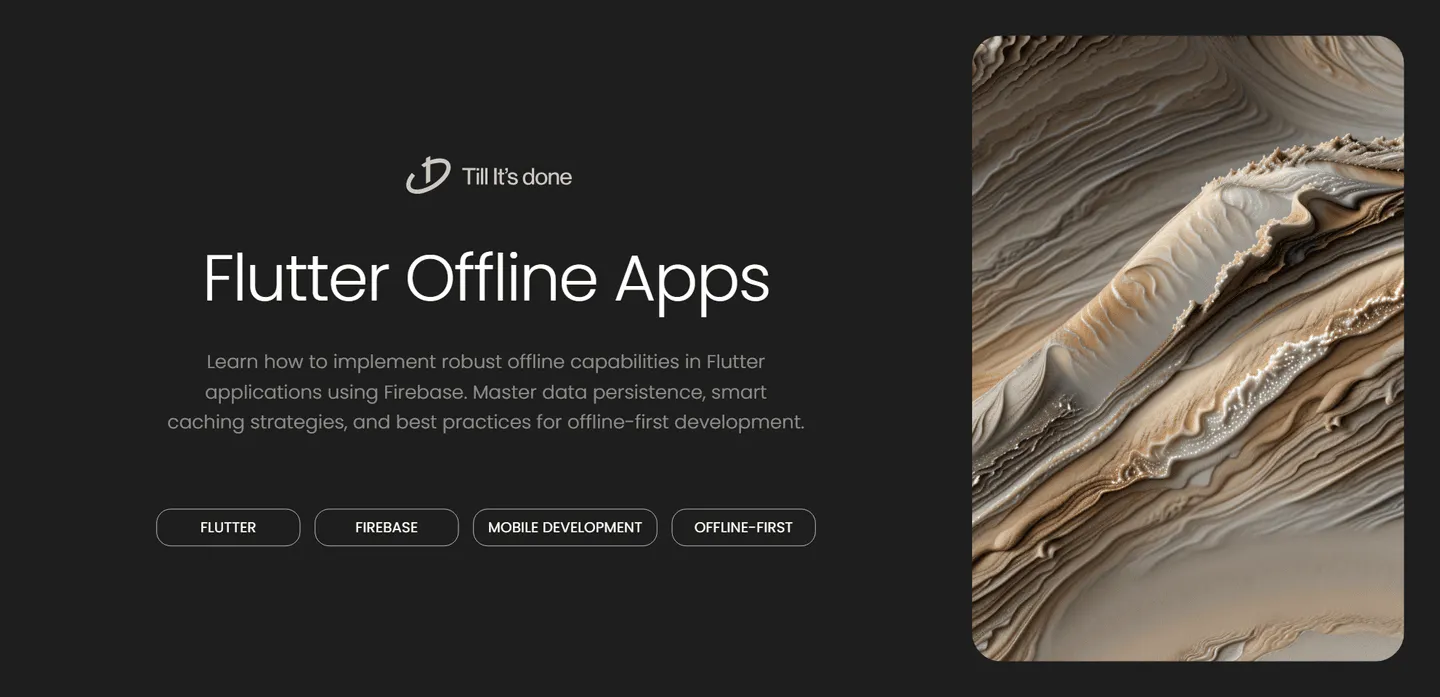
Building Robust Offline Capabilities in Flutter Using Firebase
In today’s fast-paced mobile world, users expect apps to work seamlessly - with or without an internet connection. Let’s dive into how we can achieve this using Flutter and Firebase, creating apps that work flawlessly even when offline.
Understanding Offline Persistence
Firebase provides powerful offline capabilities out of the box, but knowing how to implement them effectively can make the difference between a good app and a great one. When properly configured, your app can maintain its functionality even when users lose connectivity, ensuring a smooth user experience.
Setting Up Offline Persistence
The first step in enabling offline capabilities is to configure Firebase to persist data locally. In Flutter, this is surprisingly straightforward:
void main() async { await Firebase.initializeApp(); FirebaseFirestore.instance.settings = Settings(cacheSizeBytes: Settings.CACHE_SIZE_UNLIMITED);}
Implementing Smart Caching Strategies
Think of offline caching as your app’s safety net. Here are some key strategies to implement:
- Cache Critical Data
await FirebaseFirestore.instance .collection('important_data') .get() .then((querySnapshot) { // Data is now cached locally});
- Handle Offline Writes
try { await FirebaseFirestore.instance .collection('users') .doc('user123') .update({'lastSeen': DateTime.now()});} catch (e) { // Handle offline scenario}
Best Practices for Offline-First Development
- Always validate data locally before attempting to sync
- Implement retry mechanisms for failed operations
- Use optimistic UI updates to maintain responsiveness
- Monitor network status and adapt accordingly
Real-World Applications
Consider an e-commerce app that needs to handle product browsing and cart management offline. Here’s how you might approach it:
class OfflineFirstCart { Future<void> addToCart(Product product) async { // Save locally first await LocalDatabase.saveCartItem(product);
// Then sync with Firebase when online try { await FirebaseFirestore.instance .collection('carts') .doc(userId) .update({ 'items': FieldValue.arrayUnion([product.toMap()]) }); } catch (e) { // Queue for later sync await SyncQueue.add(product); } }}
Testing and Monitoring
Remember to thoroughly test your offline capabilities:
- Use airplane mode to simulate network loss
- Test various network conditions
- Monitor sync conflicts and resolution
- Verify data consistency after reconnection
Conclusion
Building offline-first applications with Flutter and Firebase isn’t just about handling network failures - it’s about creating resilient apps that users can rely on, regardless of their connection status.
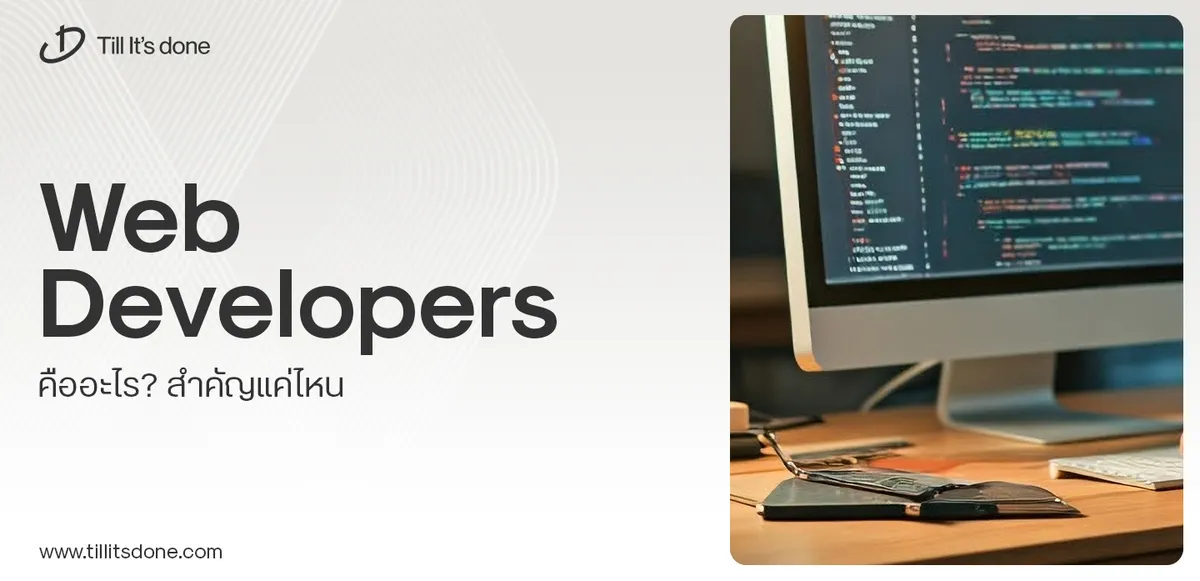
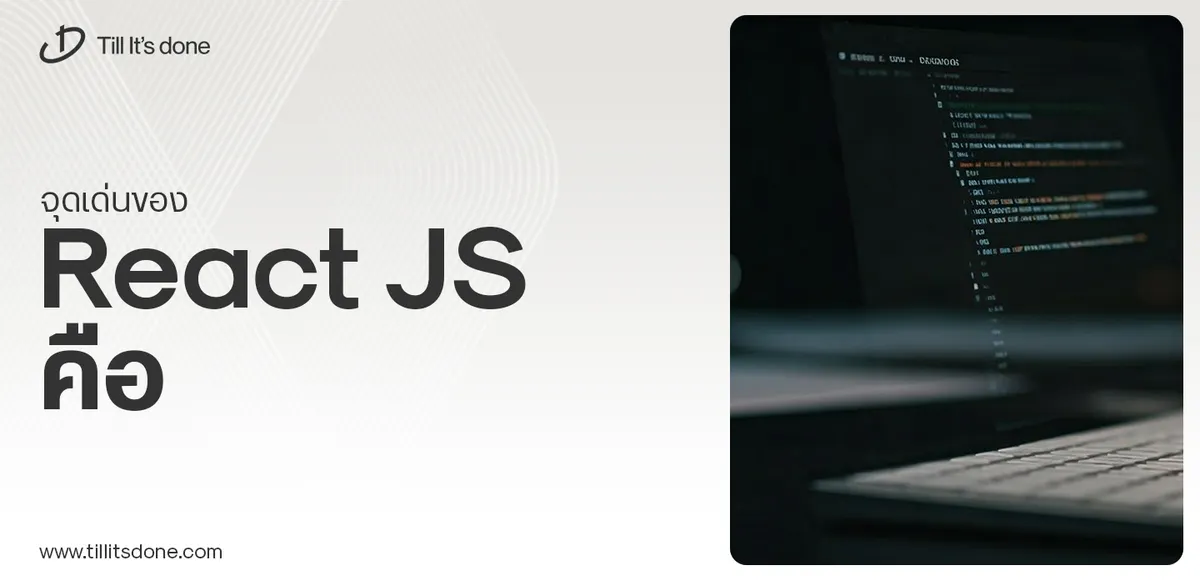
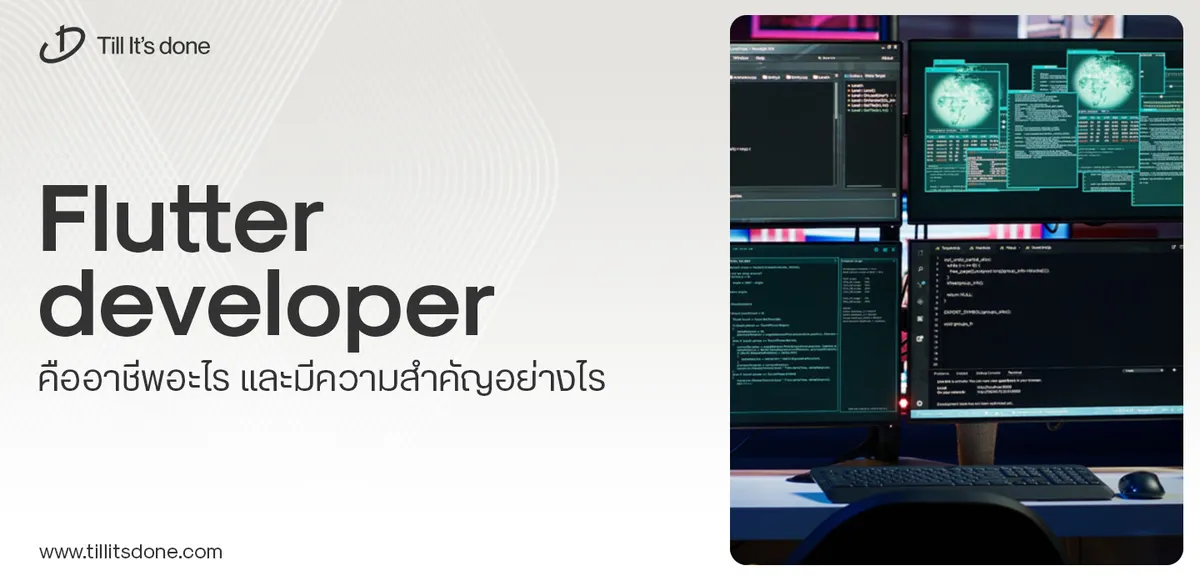
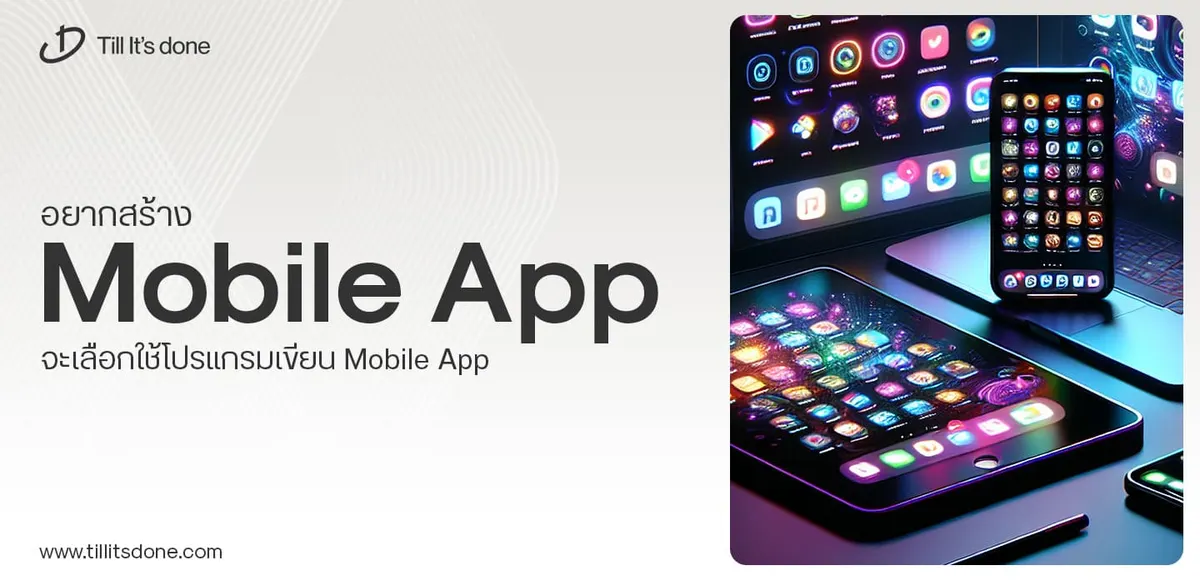
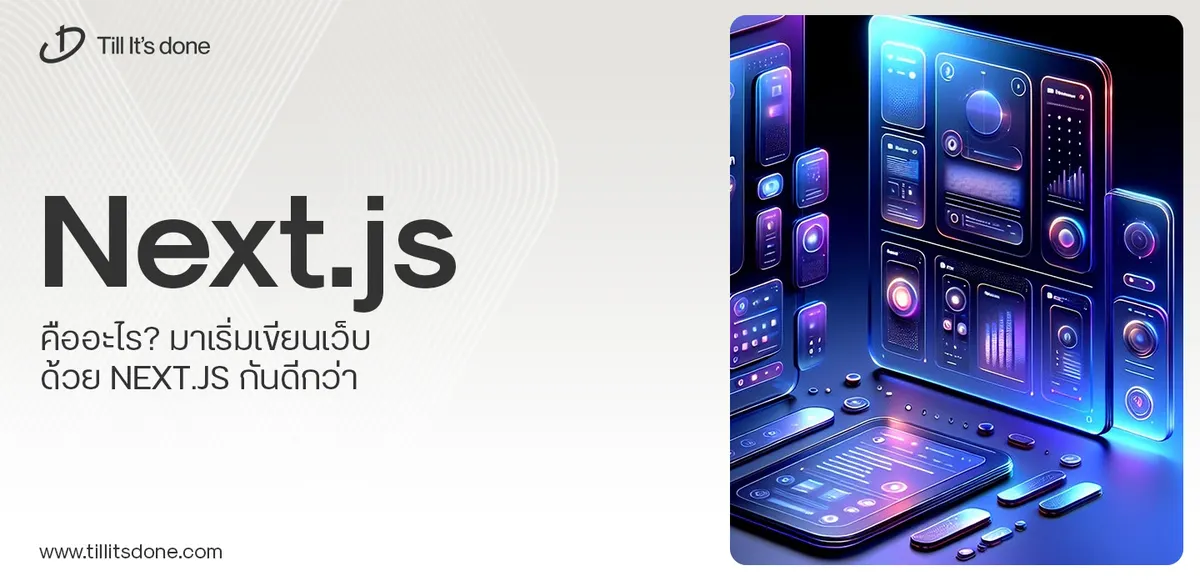
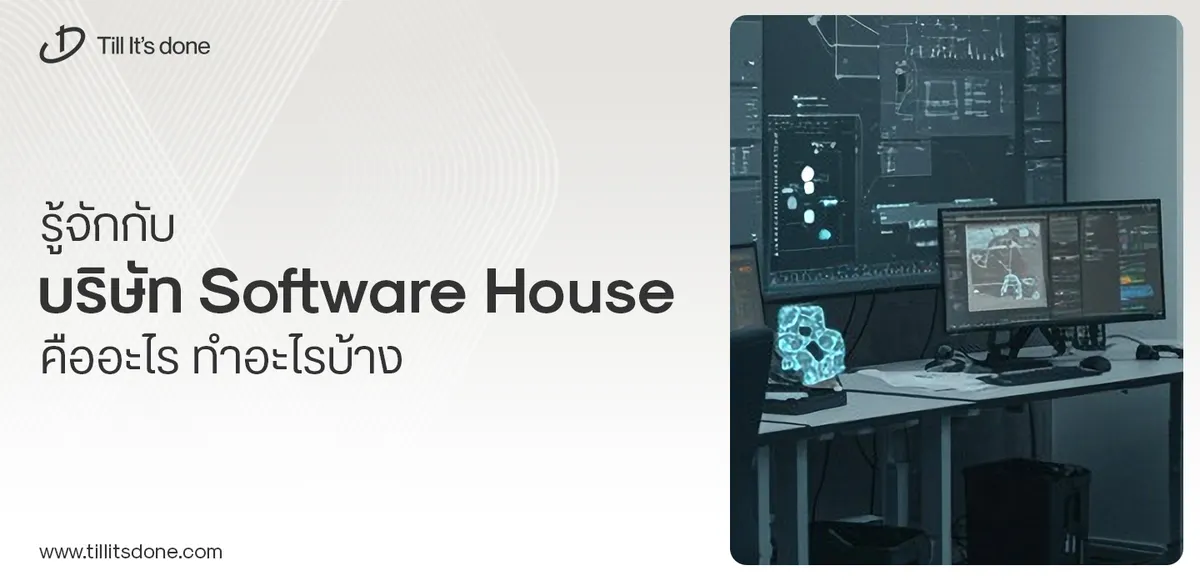
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.