- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Flutter Async Programming: Future and Stream
Learn how to handle time-consuming tasks, manage data flows, and create responsive applications with practical examples.

Understanding Flutter’s Asynchronous Programming with Future and Stream
Have you ever wondered how Flutter handles tasks that take time to complete? Think about fetching data from an API, reading files, or processing large amounts of information. This is where asynchronous programming comes into play, and Flutter has two powerful tools for handling it: Future and Stream.
Understanding Future
Imagine you’re at a coffee shop. When you order a coffee, you don’t stand at the counter waiting - you get an order number (a promise) and can do other things while your coffee is being prepared. This is exactly how Future works in Flutter!
A Future represents a value that will be available at some point in the future. It’s like a container that will eventually hold your data. Here’s what makes Future special:
- It helps your app stay responsive while performing time-consuming tasks
- Perfect for one-time operations like API calls or file operations
- Can handle both success and error scenarios gracefully
When working with Future, we typically use async/await syntax, which makes our code clean and easy to read. For example, fetching user data becomes as simple as waiting for your coffee order:
Future<String> fetchUserData() async { await Future.delayed(Duration(seconds: 2)); // Simulating network delay return 'User data loaded successfully';}
Diving into Stream
While Future is great for single values, Stream is perfect for handling multiple values over time. Think of it like a river of data - values keep flowing through it. This makes Stream perfect for:
- Real-time data updates
- Location tracking
- Chat applications
- Live sensor readings
Here’s how you might use a Stream to listen to real-time updates:
Stream<int> countDownStream() async* { for (int i = 5; i > 0; i--) { await Future.delayed(Duration(seconds: 1)); yield i; }}
Best Practices
When working with asynchronous programming in Flutter, keep these tips in mind:
- Always handle errors in your async operations
- Use StreamController when you need to create custom streams
- Don’t forget to dispose of your StreamSubscriptions
- Consider using StreamBuilder for UI updates
- Keep your async operations as focused and simple as possible
Wrapping Up
Asynchronous programming might seem daunting at first, but with Flutter’s Future and Stream, you have powerful tools at your disposal. Practice with simple examples first, and gradually work your way up to more complex scenarios. Remember, good async code makes your app more responsive and user-friendly!
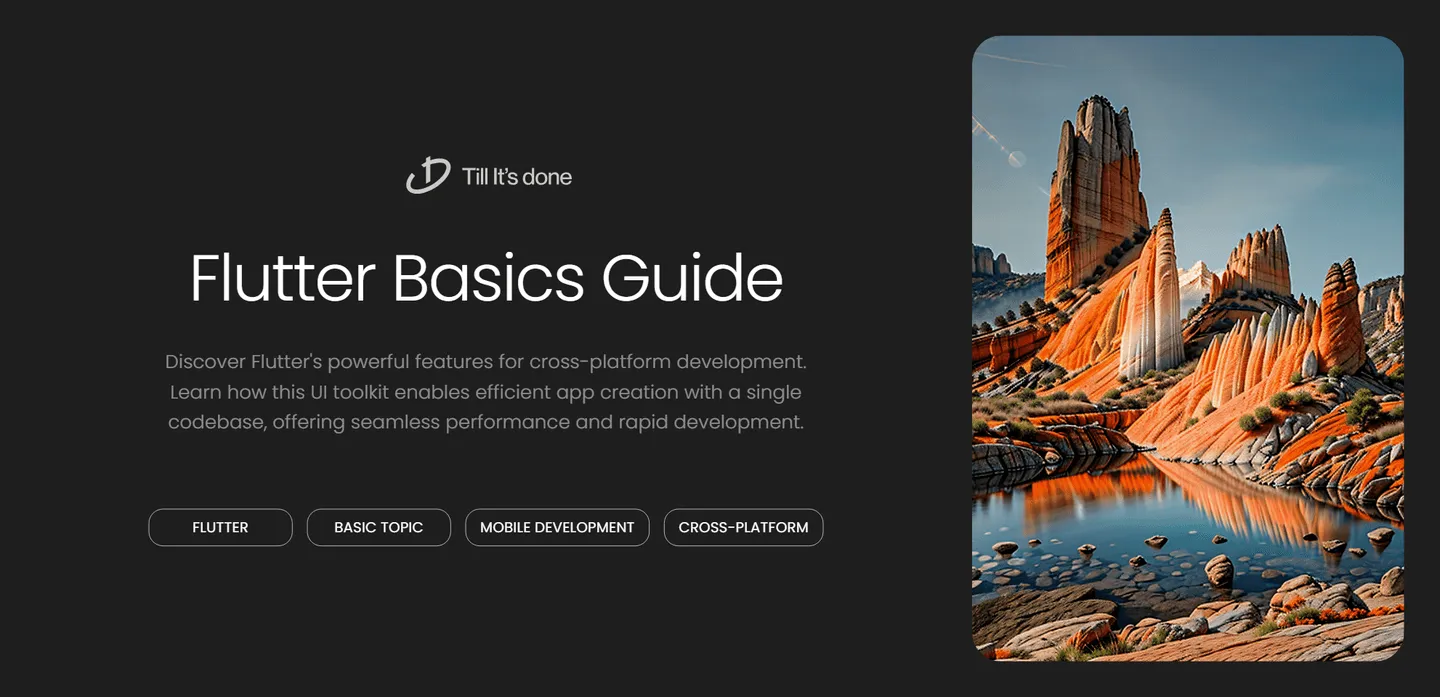
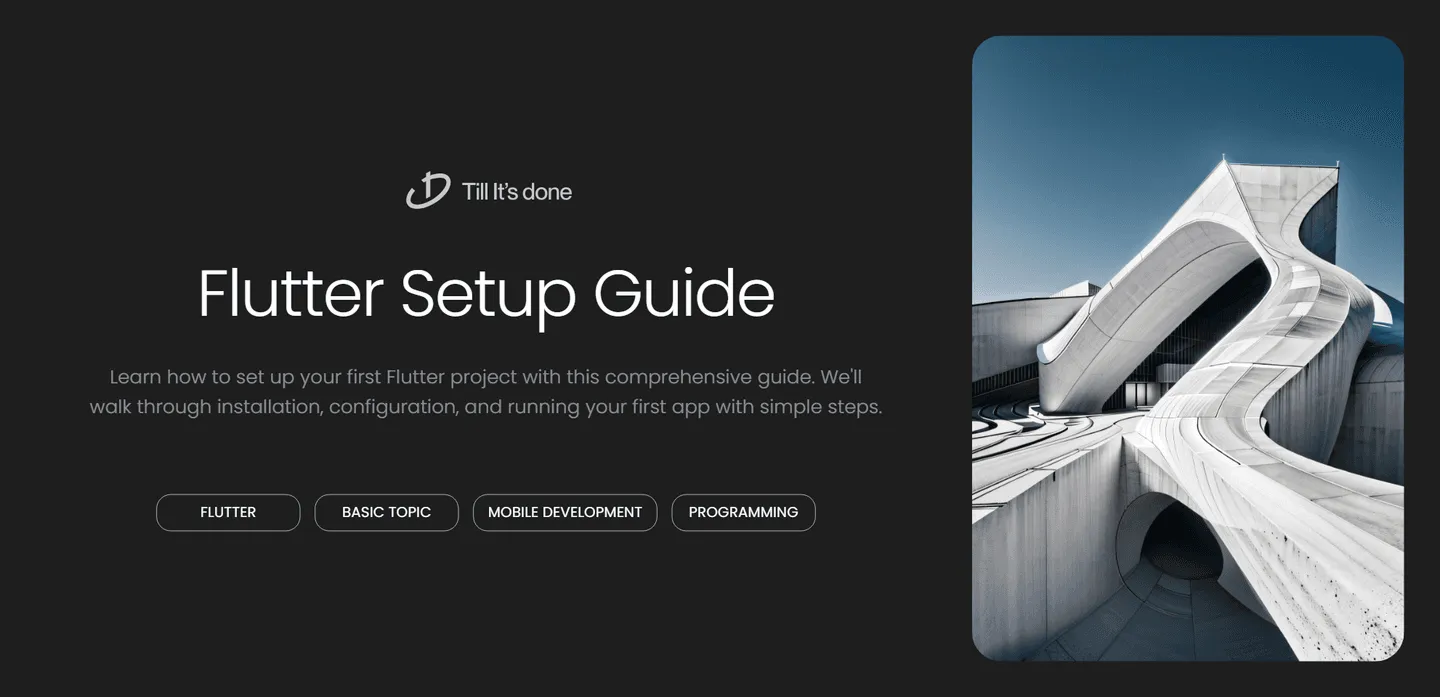
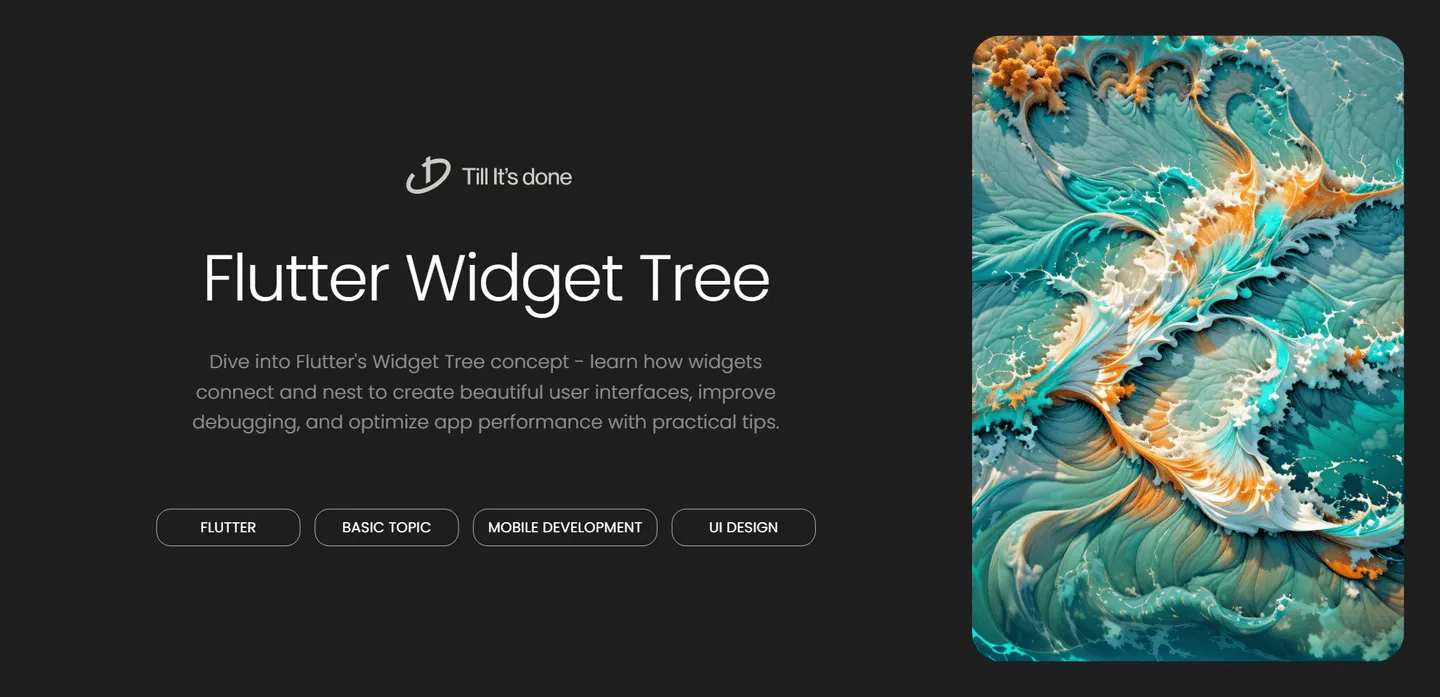
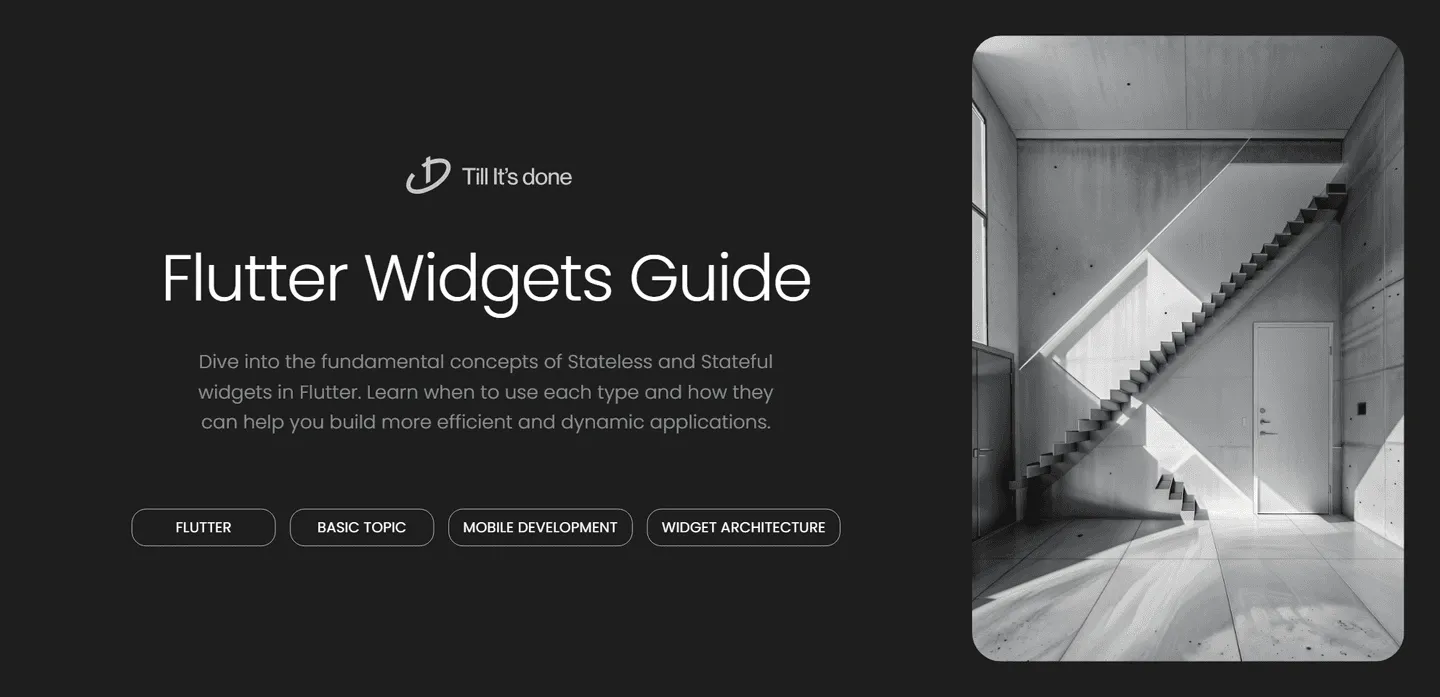
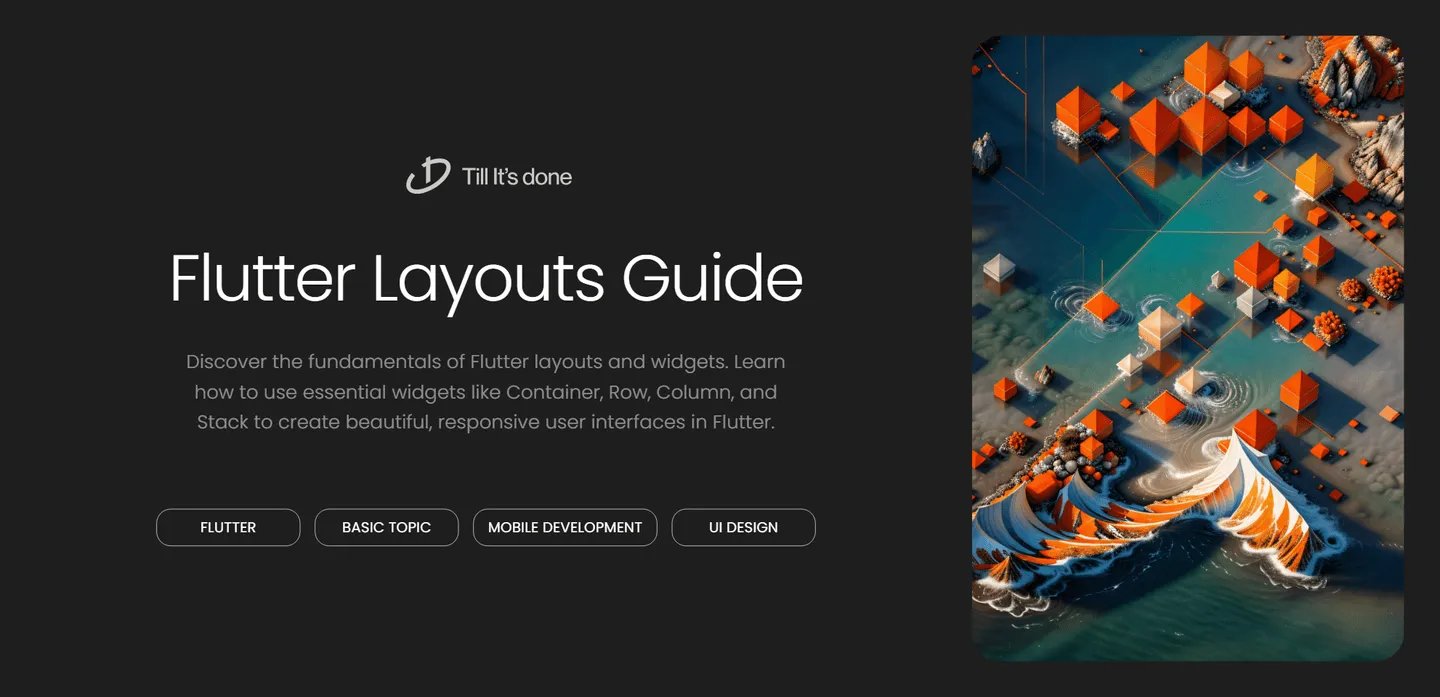
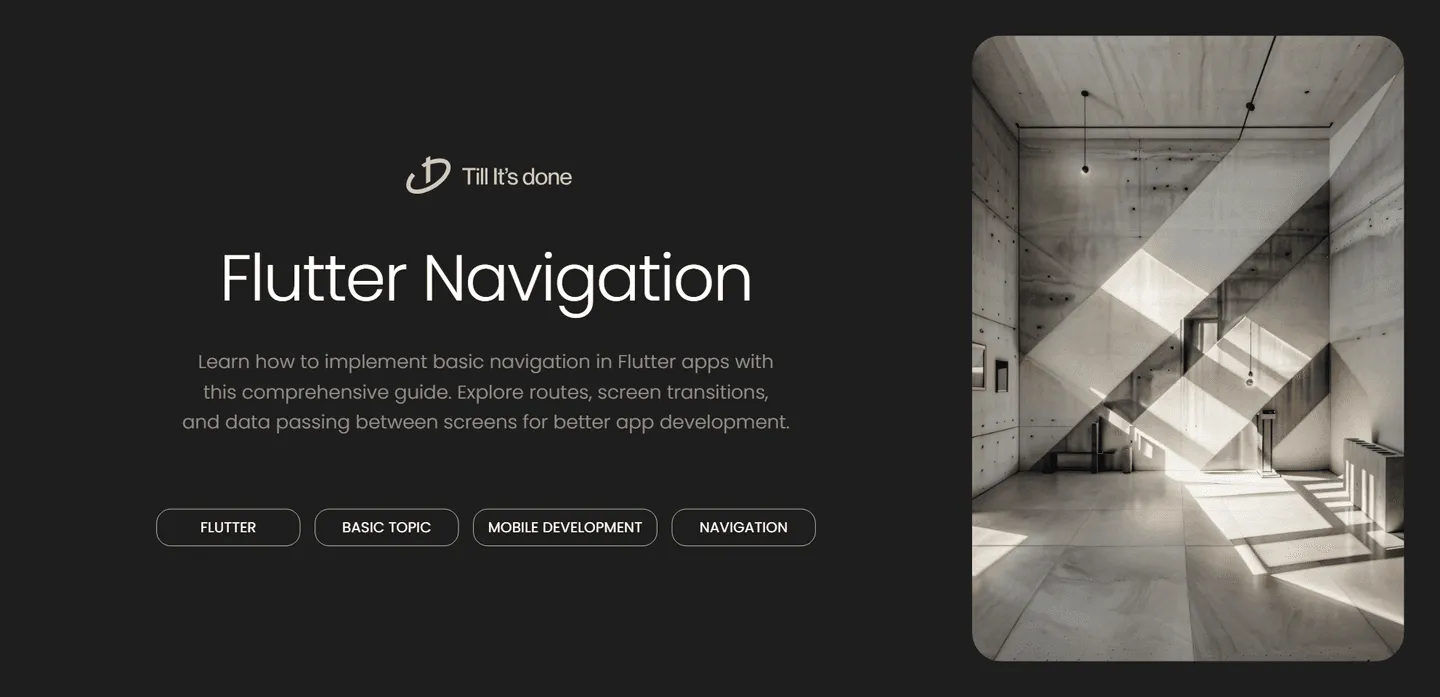
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.