- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Building a RESTful API with Express.js & Node.js
This guide covers setup, routing, middleware, security best practices, and testing for building production-ready APIs.
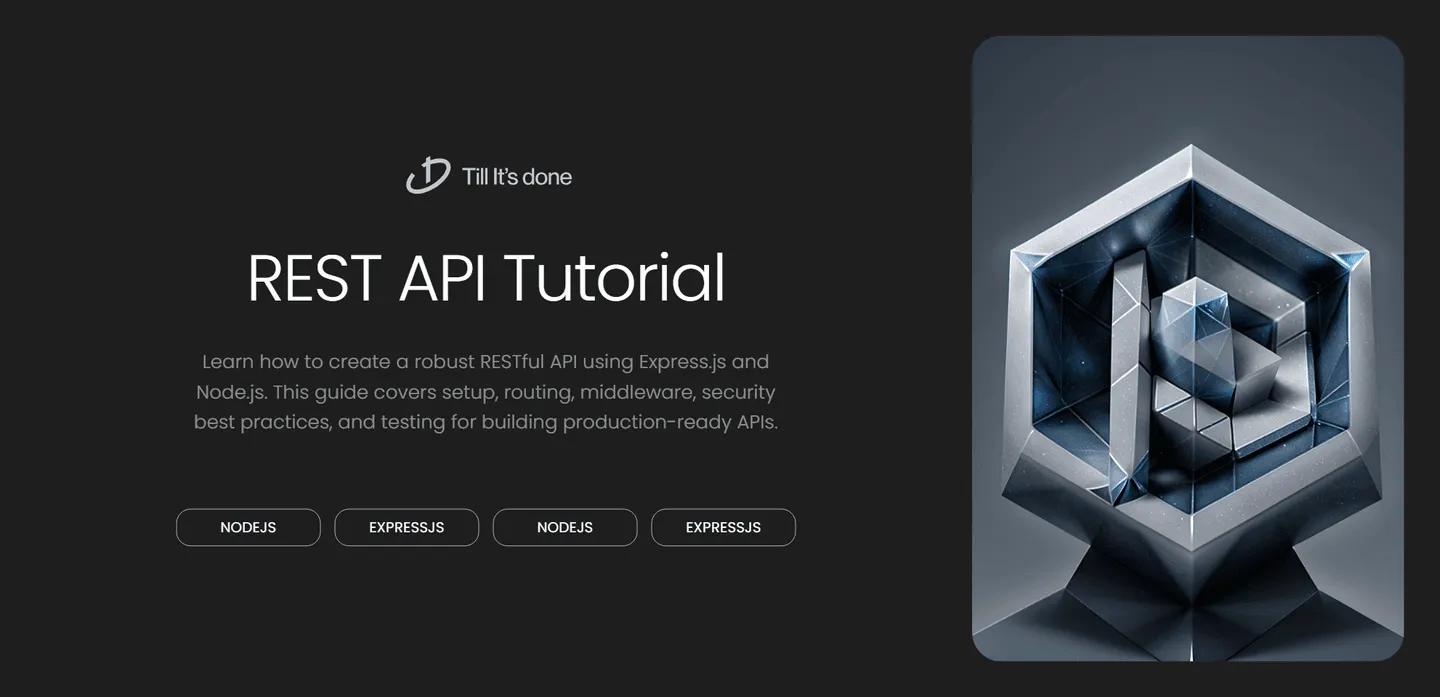
Building a RESTful API with Express.js and Node.js
In today’s digital landscape, RESTful APIs have become the backbone of modern web applications. Let’s dive into creating a robust API using Express.js and Node.js, two powerhouses of backend development.
Getting Started
Before we jump into coding, let’s understand what makes Express.js such a popular choice. Its minimal, flexible approach combined with Node.js’s event-driven architecture creates a perfect environment for building scalable APIs.
Setting Up Your Project
First, you’ll need Node.js installed on your system. Create a new project directory and initialize it:
mkdir express-apicd express-apinpm init -ynpm install express body-parser cors
Creating Your First API Endpoint
The beauty of Express lies in its straightforward approach to handling routes:
const express = require('express');const app = express();const port = 3000;
app.use(express.json());
app.get('/api/users', (req, res) => { res.json({ message: 'Users retrieved successfully' });});
app.listen(port, () => { console.log(`Server running on port ${port}`);});
Best Practices for API Design
- Use appropriate HTTP methods (GET, POST, PUT, DELETE)
- Implement proper error handling
- Validate input data
- Use middleware for common tasks
- Structure your routes logically
Here’s an example of a more structured approach:
// Route handlerapp.post('/api/users', validateUser, async (req, res) => { try { const user = await createUser(req.body); res.status(201).json(user); } catch (error) { res.status(500).json({ error: error.message }); }});
Middleware Magic
Middleware functions are the secret sauce of Express. They can:
- Handle authentication
- Log requests
- Parse request bodies
- Handle CORS
- Validate data
Testing Your API
Always test your endpoints thoroughly. Tools like Postman or curl make this process straightforward:
curl -X GET http://localhost:3000/api/users
Security Considerations
Remember to:
- Implement rate limiting
- Use helmet for security headers
- Validate and sanitize inputs
- Handle errors gracefully
- Use environment variables for sensitive data
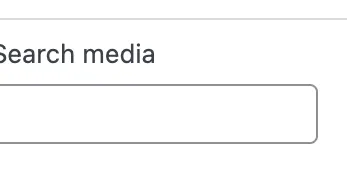





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.