- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Best Practices for Structuring Express.js Apps
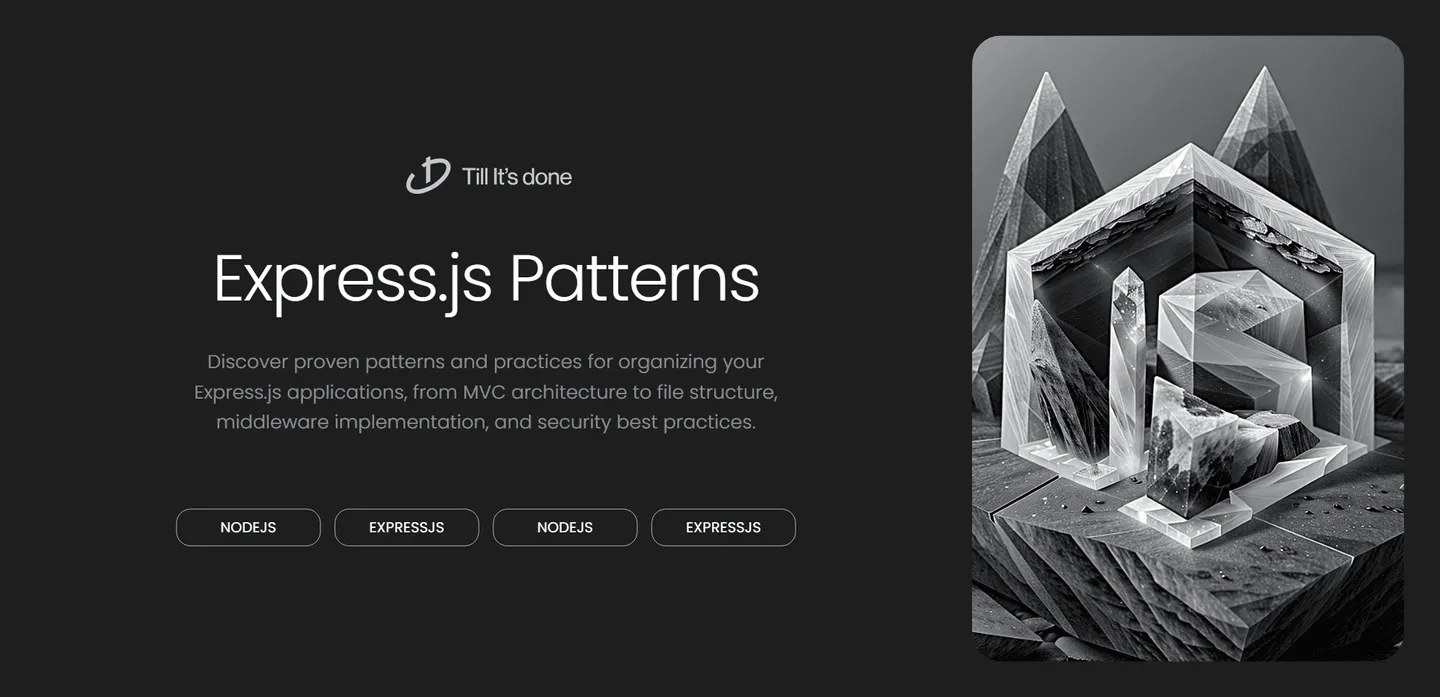
Best Practices for Structuring Express.js Applications
Over the years of building Node.js applications with Express.js, I’ve learned that a well-structured project can make the difference between a maintenance nightmare and a scalable, easy-to-manage codebase. Let’s dive into some battle-tested practices that can help you structure your Express.js applications better.
1. Follow the MVC Pattern
The Model-View-Controller (MVC) pattern has stood the test of time for a reason. In Express.js applications, this translates to:
- Models: Handle data logic and interact with your database
- Views: Handle the presentation layer (templates, JSON responses)
- Controllers: Process incoming requests and return appropriate responses
2. Organize Files by Feature
Instead of grouping files by technical role (all controllers in one folder, all models in another), consider organizing them by feature. Here’s what this might look like:
src/ features/ auth/ auth.controller.js auth.model.js auth.routes.js auth.middleware.js users/ users.controller.js users.model.js users.routes.js products/ products.controller.js products.model.js products.routes.js
3. Implement Middleware Effectively
Middleware is one of Express.js’s superpowers. Use it wisely for:
- Authentication and authorization
- Request logging
- Error handling
- Request parsing
- CORS handling
4. Centralize Error Handling
Don’t scatter try-catch blocks throughout your code. Instead, create a central error handling middleware:
const errorHandler = (err, req, res, next) => { const statusCode = err.statusCode || 500; res.status(statusCode).json({ status: 'error', message: err.message });};
5. Use Environment Configuration
Keep your configuration separate from your code using environment variables:
- Use
dotenv
for local development - Store configuration in environment variables for production
- Create a configuration module to manage all config values
6. Implement API Versioning
Future-proof your API by implementing versioning from the start:
src/ v1/ features/ users/ products/ v2/ features/ users/ products/
7. Write Clean, Testable Code
- Keep functions small and focused
- Use dependency injection
- Separate business logic from Express.js routing logic
- Write unit tests for your business logic
- Use integration tests for your API endpoints
8. Security Best Practices
- Use Helmet.js for security headers
- Implement rate limiting
- Validate input data
- Use CORS properly
- Keep dependencies updated
9. Performance Optimization
- Implement caching strategies
- Use compression middleware
- Optimize database queries
- Consider using clustering
Remember, these practices should be adapted to your specific needs. The key is maintaining consistency throughout your project and making it easy for team members to understand and contribute to the codebase.
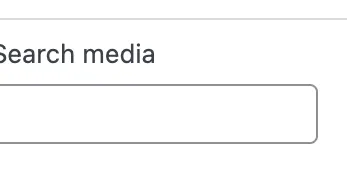





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.