- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
10 Essential TypeScript Tips for Beginners
Learn how to write safer, more maintainable code from day one.
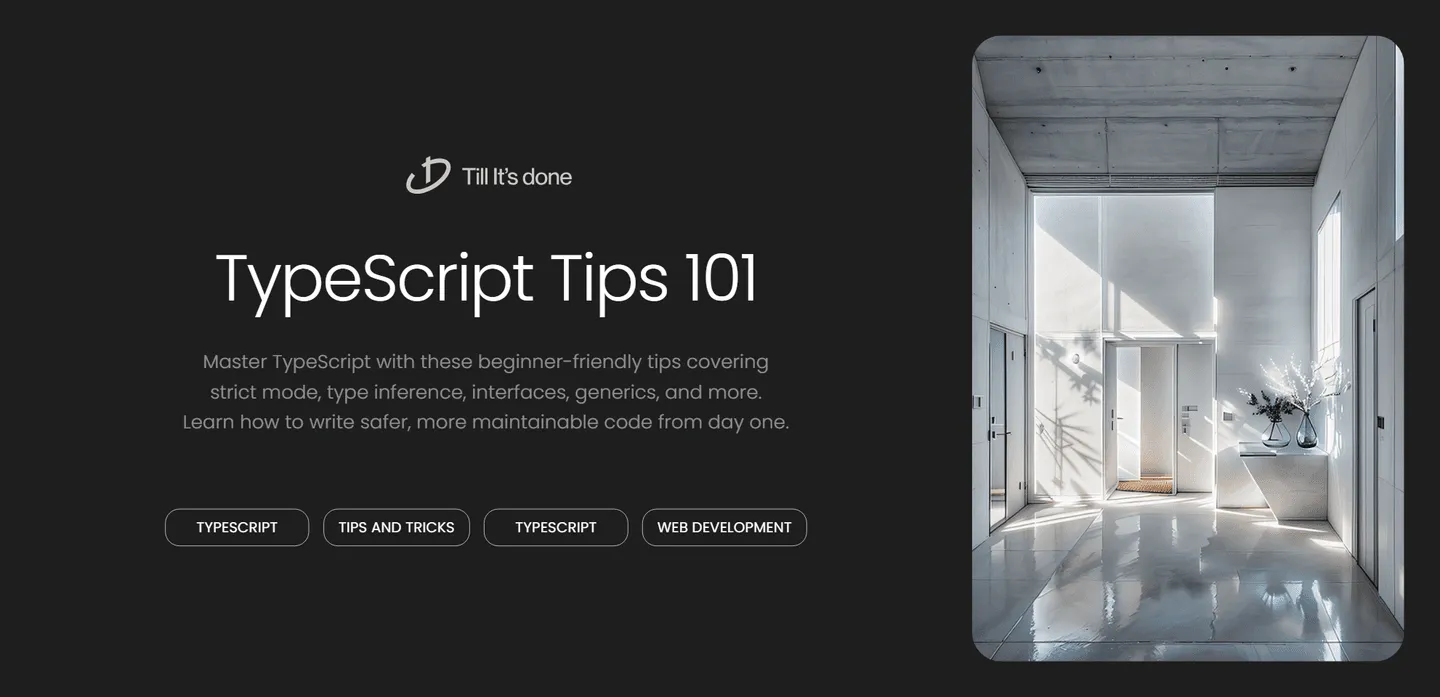
10 Essential TypeScript Tips for Beginners
As someone who’s spent countless hours working with TypeScript, I’ve gathered some invaluable tips that I wish I’d known when starting out. Let’s dive into ten essential TypeScript tips that will help you write better, safer code from day one.
1. Enable Strict Mode from the Start
One of the best decisions you can make when beginning with TypeScript is to enable strict mode in your tsconfig.json
. While it might seem intimidating at first, it’s like having a strict but helpful mentor watching over your code:
{ "compilerOptions": { "strict": true }}
This single setting helps catch common mistakes and enforces better typing practices. Trust me, your future self will thank you!
2. Type Inference Is Your Friend
Instead of explicitly typing everything, let TypeScript’s powerful type inference do the heavy lifting:
// Instead of thisconst message: string = "Hello";
// Do thisconst message = "Hello"; // TypeScript knows it's a string!
3. Understanding the ‘any’ Type Trap
Think of ‘any’ as an escape hatch - it’s tempting to use it when you’re stuck, but it defeats the purpose of using TypeScript. Instead, consider using ‘unknown’ for better type safety:
// Avoidfunction processData(data: any) { // This is unsafe!}
// Betterfunction processData(data: unknown) { if (typeof data === "string") { // TypeScript knows it's safe now return data.toUpperCase(); }}
4. Interface vs. Type: Making the Right Choice
Interfaces and types are similar, but each has its sweet spot. Interfaces are great for defining object shapes and can be extended, while types are perfect for unions and complex types:
// Interface for objectsinterface User { name: string; age: number;}
// Type for unionstype Status = "pending" | "approved" | "rejected";
5. Mastering Optional Properties
Use the ?
operator for optional properties instead of allowing undefined:
interface Profile { name: string; bio?: string; // Optional}
6. Leveraging Union Types
Union types are incredibly powerful for handling multiple possible types:
function handleResponse(response: string | number) { if (typeof response === "string") { console.log(response.toUpperCase()); } else { console.log(response.toFixed(2)); }}
7. Type Assertions When Necessary
Sometimes you know more about a type than TypeScript does. Use type assertions wisely:
const canvas = document.getElementById("myCanvas") as HTMLCanvasElement;
8. Generics for Reusable Code
Generics might seem complex, but they’re worth learning for creating flexible, reusable code:
function firstElement\<T\>(arr: T[]): T | undefined { return arr[0];}
9. Readonly for Immutability
Use readonly
to prevent accidental modifications:
interface Config { readonly apiKey: string; readonly baseUrl: string;}
10. Utilize Built-in Utility Types
TypeScript comes with powerful utility types that can save you time:
type UserPartial = Partial<User>; // All properties become optionaltype UserReadonly = Readonly<User>; // All properties become readonly
Remember, TypeScript is here to help, not to complicate your development process. Start with these fundamentals, and you’ll be writing safer, more maintainable code in no time!
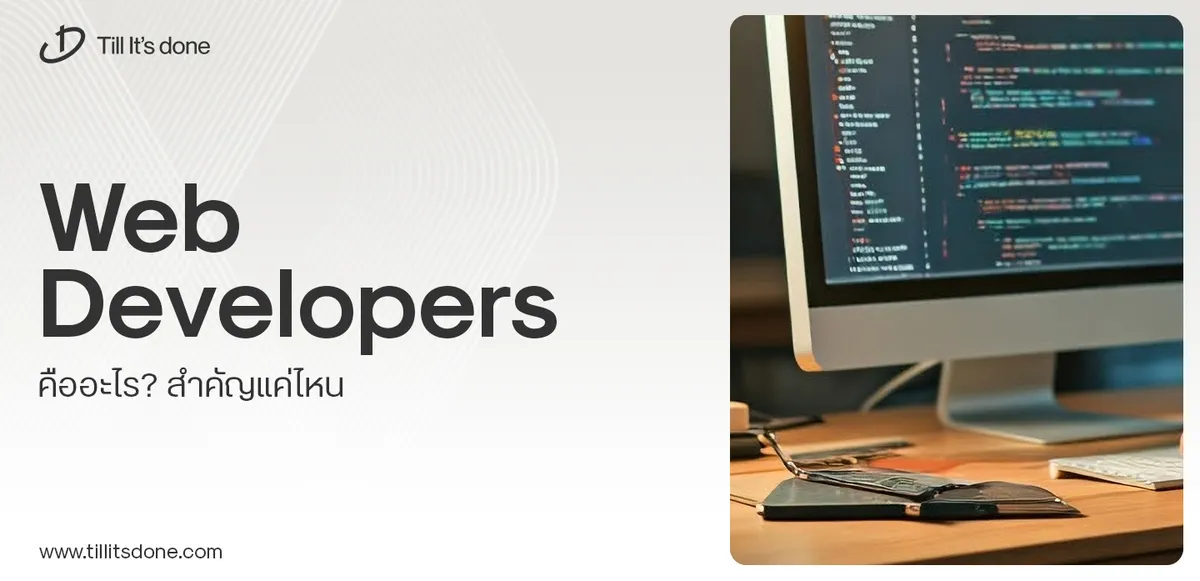
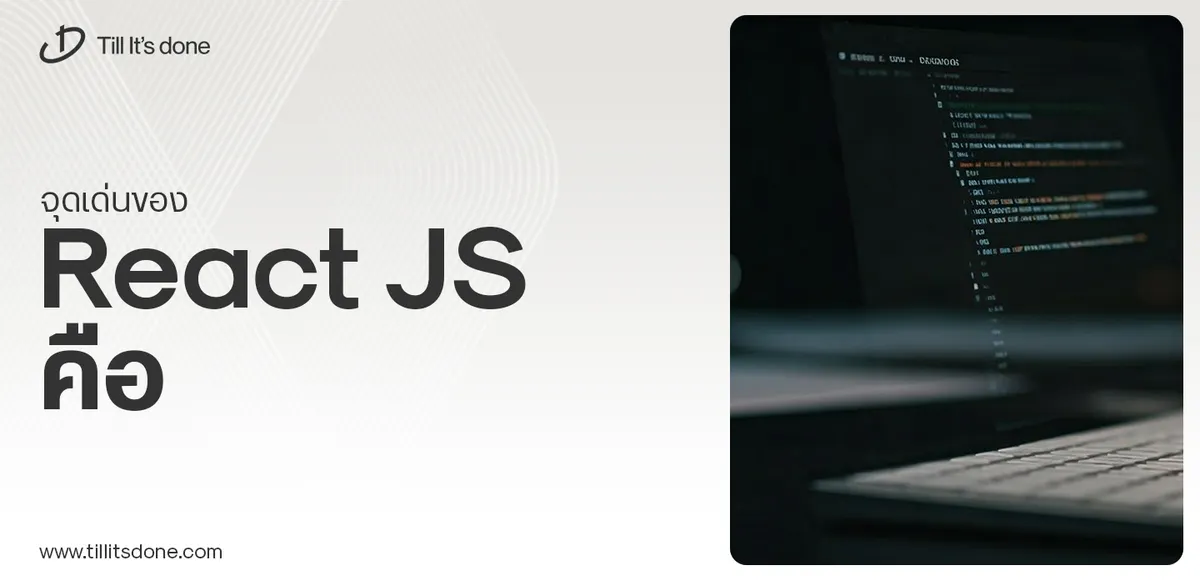
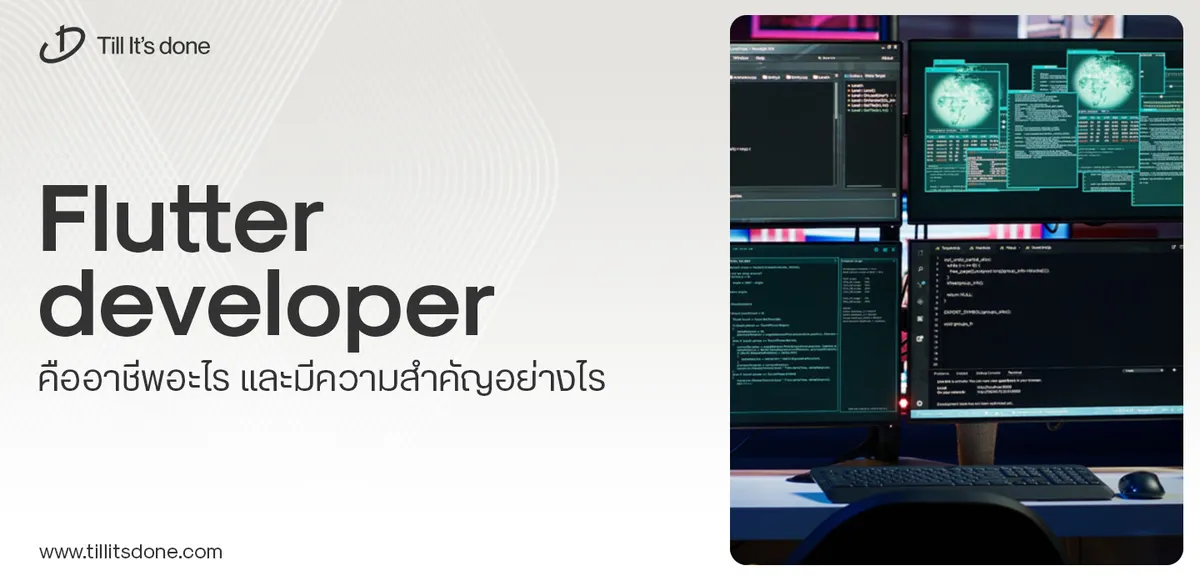
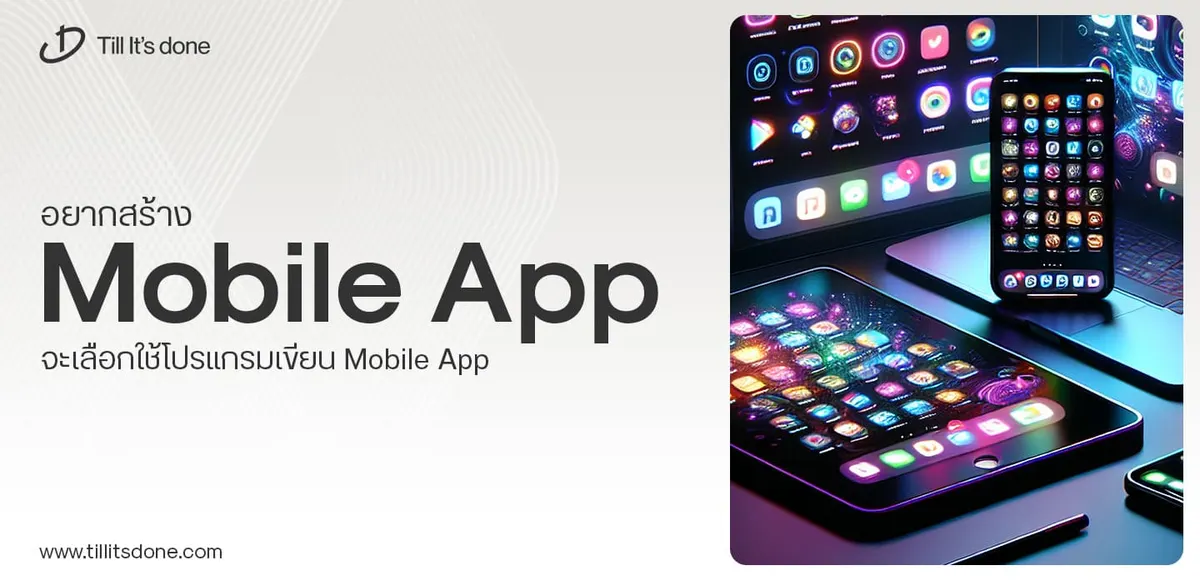
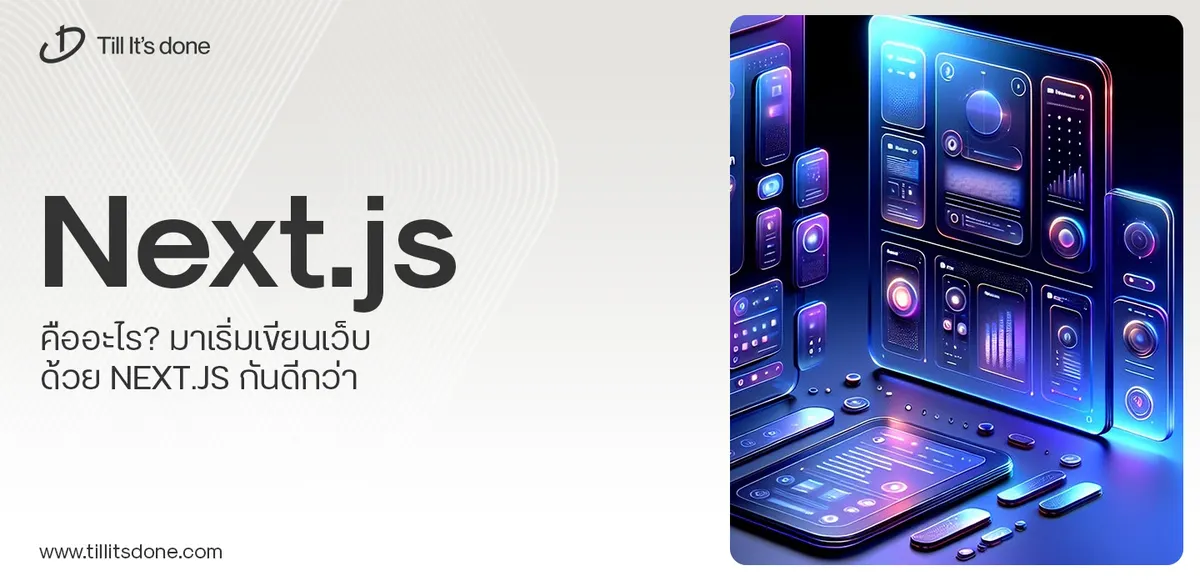
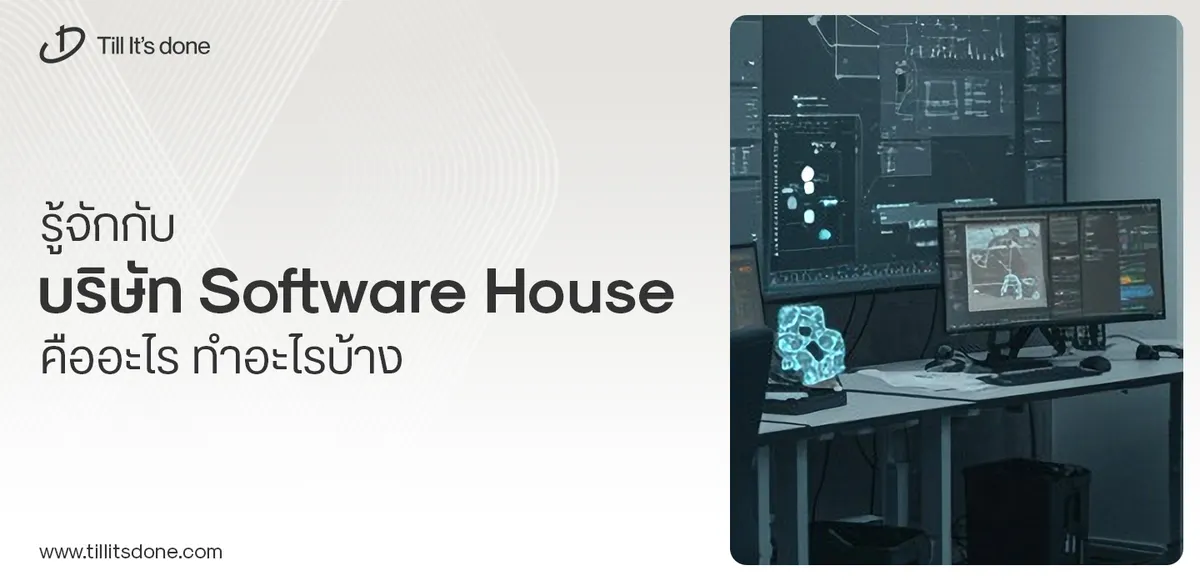
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.