- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Error Handling in Go: Using the 'error' Type
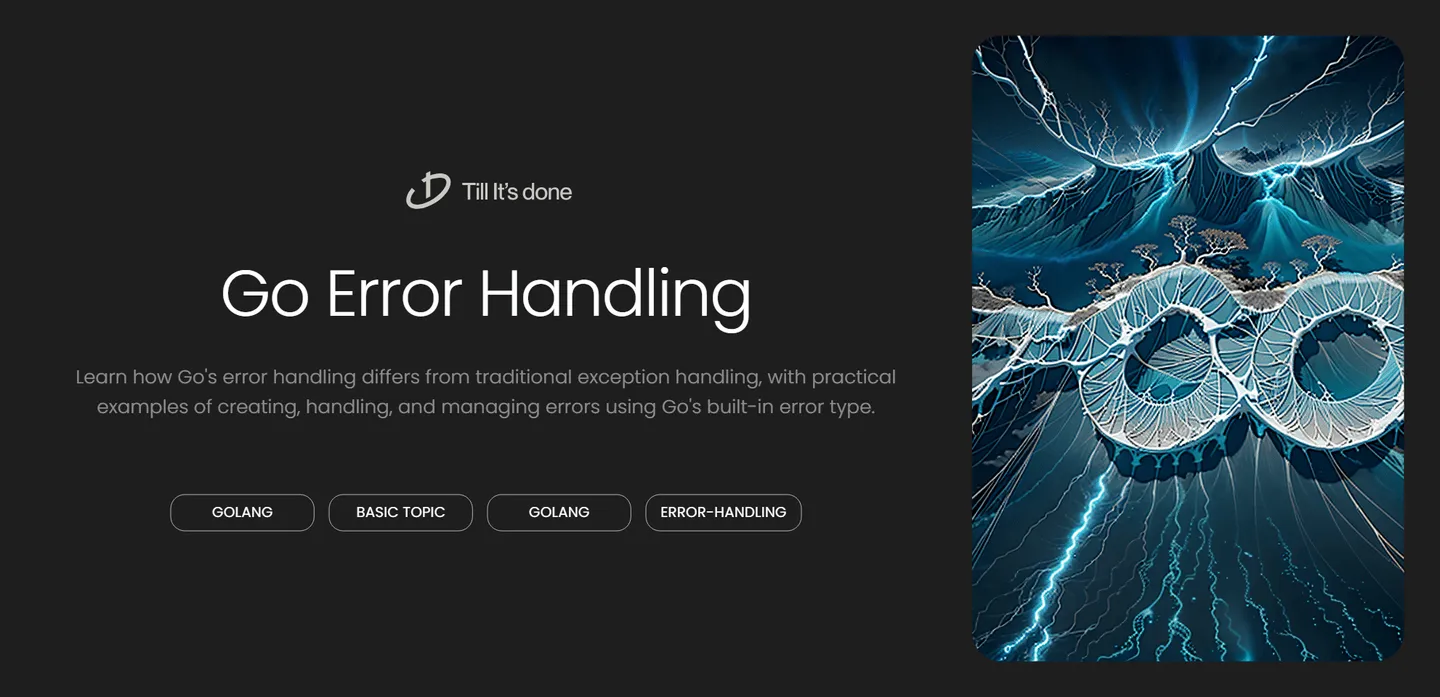
Error Handling in Go: How to Use the ‘error’ Type
One of Go’s most distinctive features is its approach to error handling. Unlike many other programming languages that use exceptions, Go takes a more straightforward path with its error
type. Today, let’s dive into how Go handles errors and why this approach might be simpler than you think.
Understanding Go’s Error Philosophy
In Go, errors are values. This simple concept is fundamental to understanding how Go handles errors. Instead of throwing exceptions and using try-catch blocks, Go functions typically return an error value that you can check and handle directly.
Let’s look at a basic example:
func divide(a, b float64) (float64, error) { if b == 0 { return 0, errors.New("division by zero") } return a / b, nil}
Working with Errors in Practice
When calling functions that might return errors, we handle them using an if statement check. This might seem verbose at first, but it makes error handling explicit and clear:
result, err := divide(10, 0)if err != nil { fmt.Println("An error occurred:", err) return}fmt.Printf("Result: %v\n", result)
Creating Custom Errors
Sometimes, you’ll want to create custom errors to provide more context. Go offers several ways to do this:
// Using errors.Newerr := errors.New("invalid input")
// Using fmt.Errorferr := fmt.Errorf("processing failed: %v", underlying)
// Custom error typetype ValidationError struct { Field string Message string}
func (e *ValidationError) Error() string { return fmt.Sprintf("%s: %s", e.Field, e.Message)}
Best Practices for Error Handling
- Always check returned errors
- Keep error messages clear and actionable
- Add context when wrapping errors
- Use custom error types for specific error cases
- Don’t ignore errors silently
Remember, good error handling isn’t just about catching problems – it’s about making your code more reliable and maintainable.
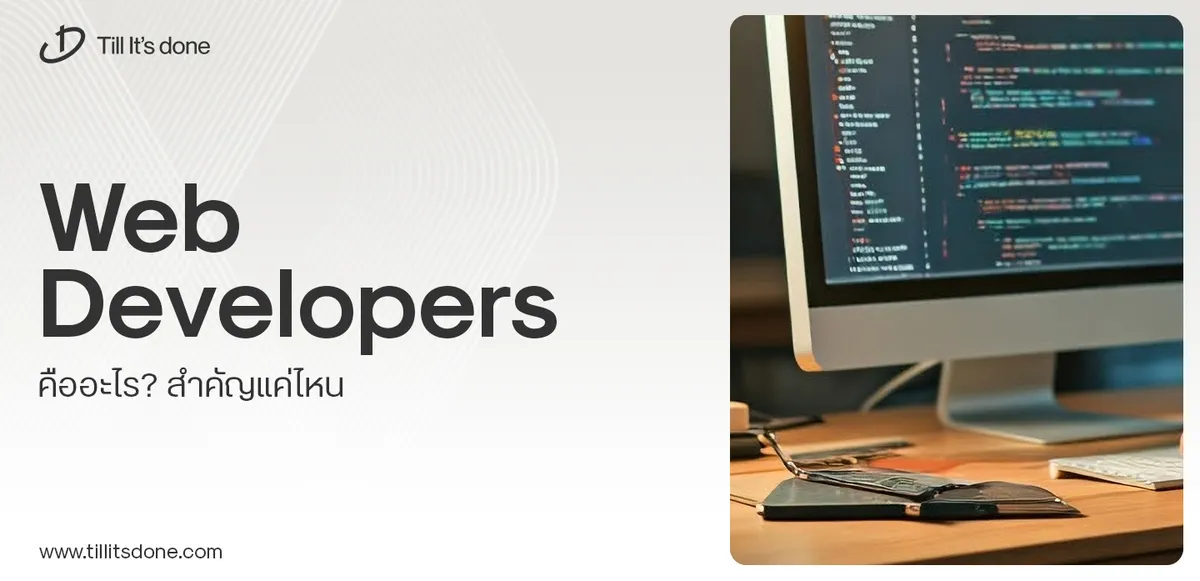
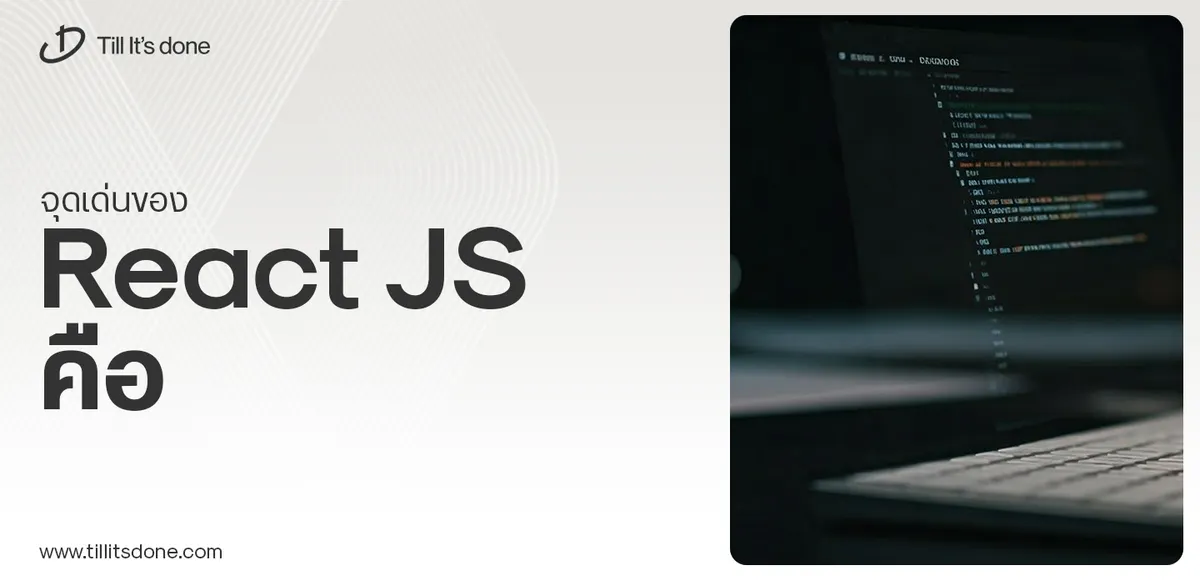
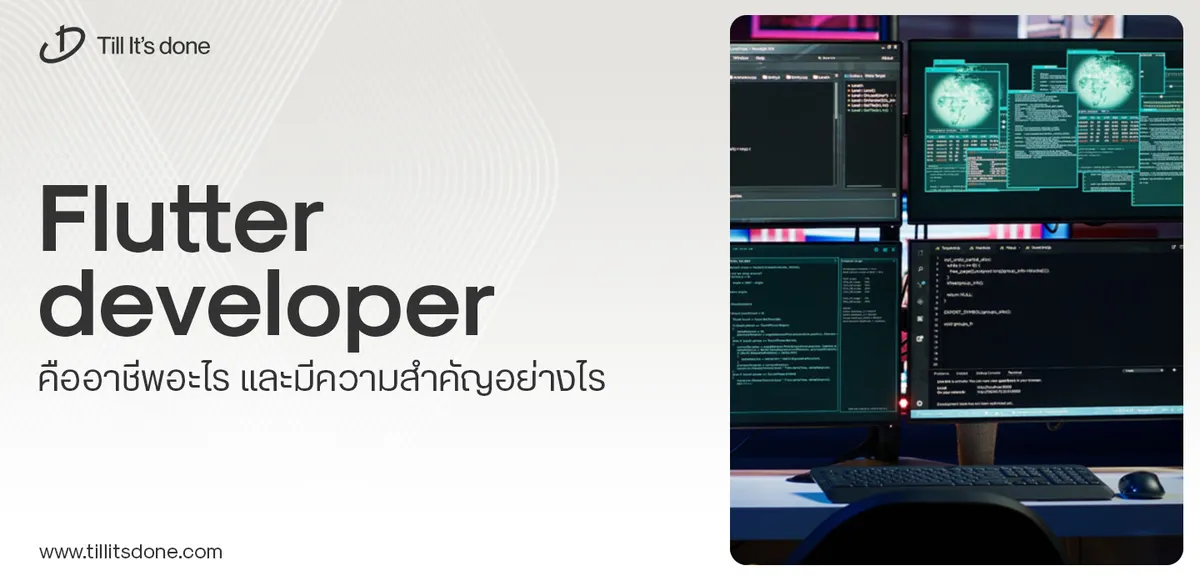
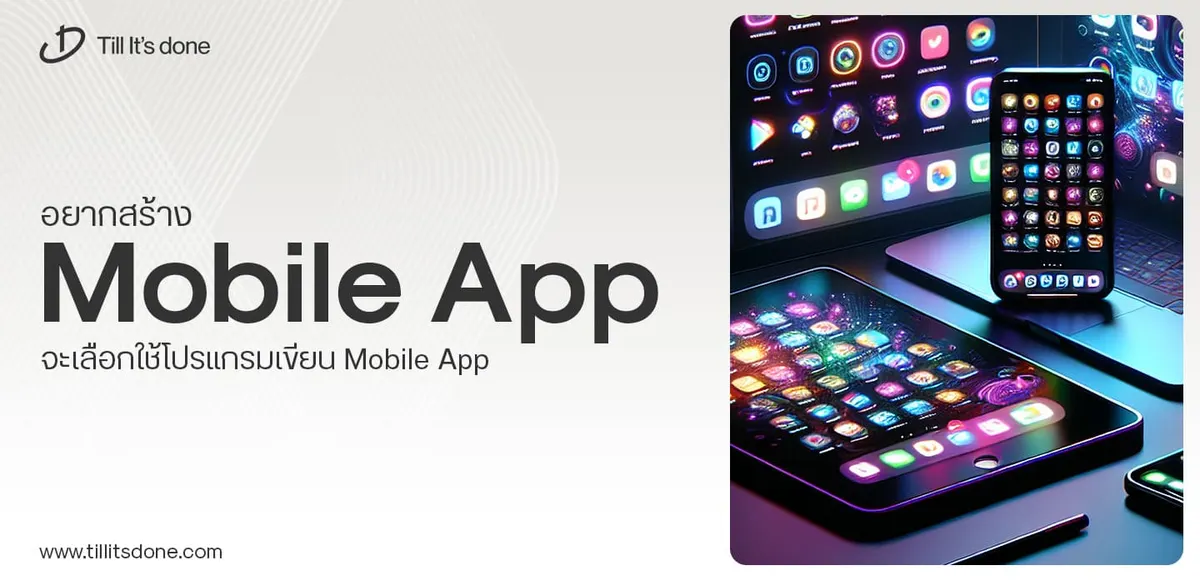
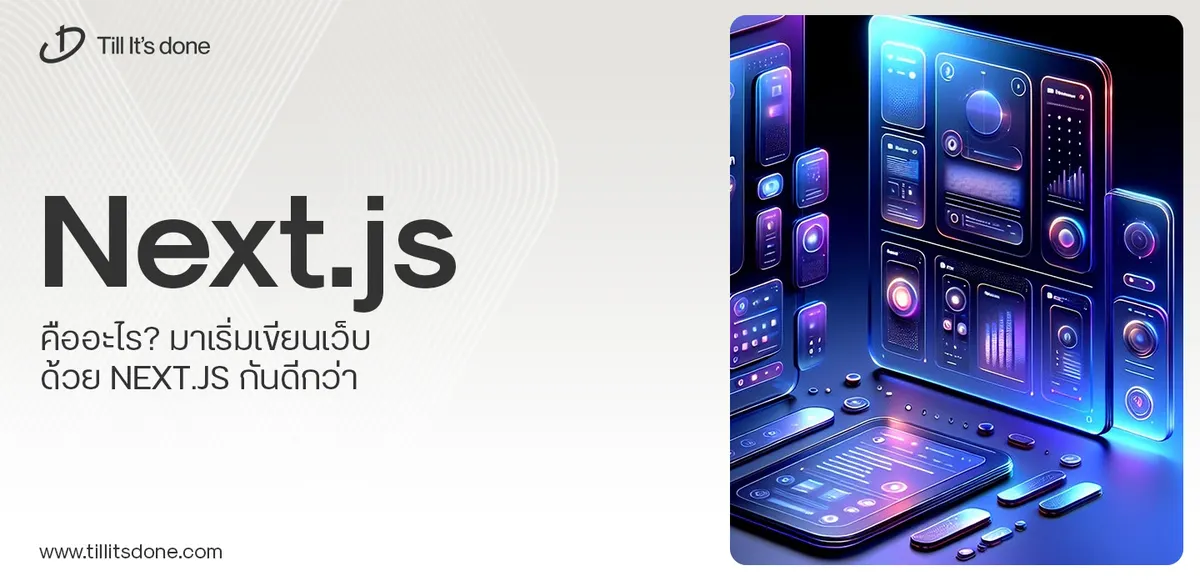
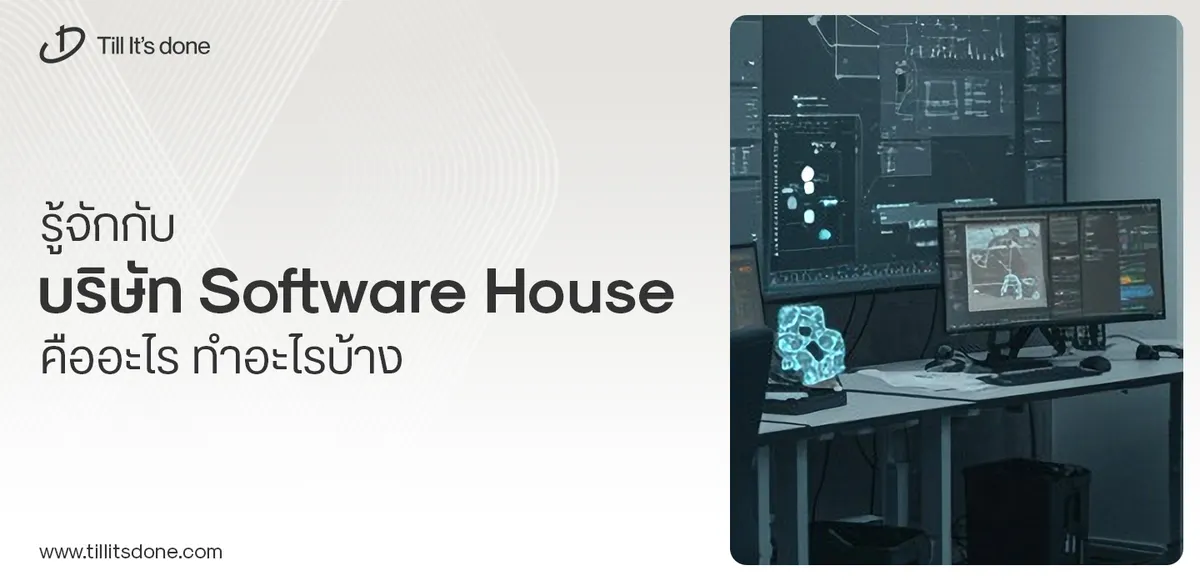
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.