- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Implement Dio's Retry for Failed Requests

Implementing Dio’s Retry Mechanism for Failed Requests in Flutter
In the world of mobile app development, handling network requests efficiently is crucial for delivering a smooth user experience. One common challenge we face is dealing with failed API requests. Network issues, server timeouts, or temporary outages can all lead to failed requests. Today, we’ll explore how to implement a robust retry mechanism using Dio in Flutter to handle such scenarios gracefully.
Understanding the Need for Retry Logic
When making API calls, things don’t always go as planned. Maybe the user’s internet connection dropped for a split second, or the server experienced a momentary hiccup. Instead of immediately showing an error to users, implementing a retry mechanism can help maintain a seamless experience by attempting to recover from these temporary failures.
Setting Up the Retry Interceptor
Let’s create a custom interceptor that will handle the retry logic for our Dio client. We’ll make it configurable so you can adjust the retry attempts and delay between retries based on your needs.
Here’s how we can implement our retry mechanism:
class RetryInterceptor extends Interceptor { final Dio dio; final int maxRetries; final Duration retryDelay;
RetryInterceptor({ required this.dio, this.maxRetries = 3, this.retryDelay = const Duration(seconds: 1), });
@override Future onError(DioException err, ErrorInterceptorHandler handler) async { int retryCount = 0;
if (_shouldRetry(err)) { while (retryCount < maxRetries) { try { retryCount++;
await Future.delayed(retryDelay * retryCount);
final response = await dio.request( err.requestOptions.path, options: Options( method: err.requestOptions.method, headers: err.requestOptions.headers, ), data: err.requestOptions.data, queryParameters: err.requestOptions.queryParameters, );
return handler.resolve(response); } on DioException catch (e) { if (retryCount >= maxRetries) { return handler.next(e); } } } }
return handler.next(err); }
bool _shouldRetry(DioException error) { return error.type == DioExceptionType.connectionTimeout || error.type == DioExceptionType.sendTimeout || error.type == DioExceptionType.receiveTimeout || error.type == DioExceptionType.connectionError; }}
Implementing the Retry Mechanism
Now that we have our interceptor ready, let’s see how to integrate it into our Dio client:
Dio createDioWithRetry() { final dio = Dio();
dio.interceptors.add( RetryInterceptor( dio: dio, maxRetries: 3, retryDelay: const Duration(seconds: 2), ), );
return dio;}
This setup will automatically retry failed requests up to three times, with an exponential delay between attempts. The delay starts at 2 seconds and doubles with each retry, giving servers time to recover and preventing request flooding.
Best Practices and Considerations
-
Choose Appropriate Retry Conditions: Not all errors should trigger retries. Focus on transient failures like network timeouts rather than server errors (400s, 500s).
-
Implement Exponential Backoff: Increasing the delay between retries helps prevent overwhelming the server and gives it time to recover.
-
Set Reasonable Limits: Don’t set too many retry attempts, as this could lead to poor user experience. Three to five retries is usually sufficient.
-
Monitor and Log: Keep track of retry attempts and success rates to help identify recurring issues that might need addressing at the server level.
Conclusion
Implementing a retry mechanism in your Flutter app using Dio is a powerful way to handle network instabilities and improve user experience. By following these patterns and best practices, you can create more resilient applications that gracefully handle temporary failures and maintain seamless functionality for your users.


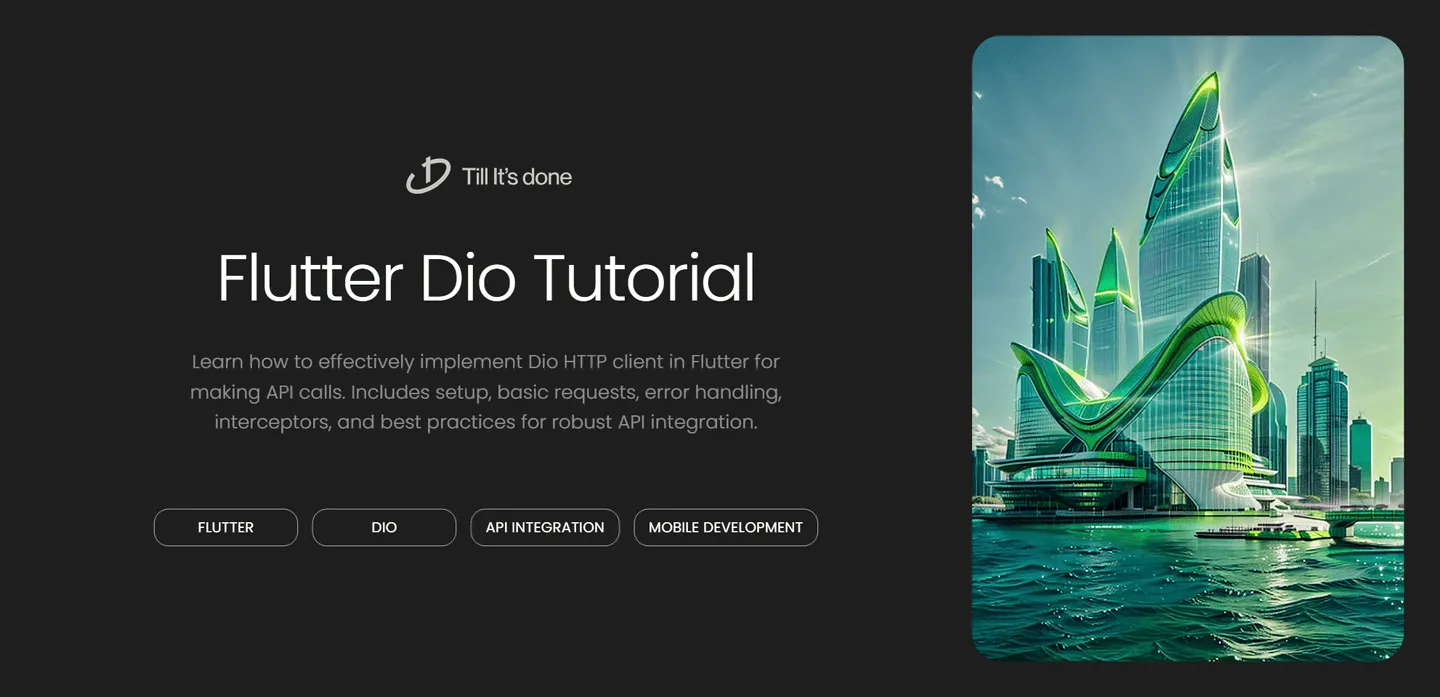



Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.