- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Debug TypeScript: Fix Common Coding Errors
Learn effective debugging techniques for cleaner code.
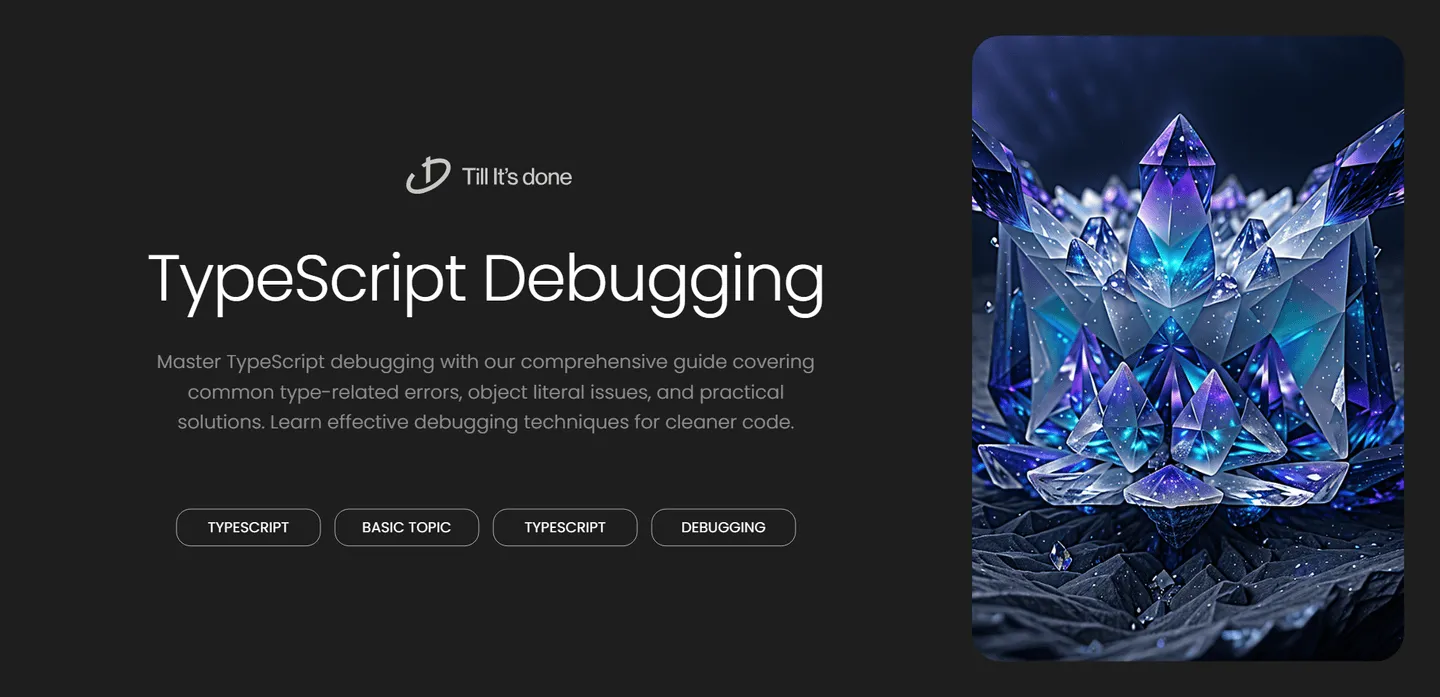
Debugging TypeScript Code: Common Errors and Solutions
As TypeScript continues to gain popularity among developers, understanding how to effectively debug your code becomes increasingly important. In this guide, we’ll explore common TypeScript errors you might encounter and provide practical solutions to help you resolve them quickly.
Understanding Type-Related Errors
One of the most frequent challenges developers face is dealing with type-related errors. These usually occur when TypeScript’s type system detects inconsistencies in your code that could lead to runtime errors.
Type ‘undefined’ is not assignable
This error typically appears when you’re trying to use a variable that might be undefined. Here’s how to handle it:
// Incorrect approachfunction getFirstElement(arr: number[]) { const firstElement = arr[0]; // Error: might be undefined return firstElement.toString();}
// Correct approachfunction getFirstElement(arr: number[]) { if (arr.length > 0) { return arr[0].toString(); } return "Array is empty";}
Object Literal Type Errors
Another common stumbling block is dealing with object literal types. These errors often occur when you’re trying to assign an object to a type with specific requirements.
interface User { name: string; age: number;}
// TypeScript will catch this errorconst user: User = { name: "John", age: "25" // Type 'string' is not assignable to type 'number'}
Working with Generics
Generics can be particularly tricky when you’re first getting started with TypeScript. Here’s an example of how to properly use generics to create type-safe functions:
function wrapInArray\<T\>(value: T): T[] { return [value];}
const numberArray = wrapInArray(42); // type is number[]const stringArray = wrapInArray("Hello"); // type is string[]
Pro Tips for Effective Debugging
- Enable strict mode in your
tsconfig.json
to catch more potential errors early:
{ "compilerOptions": { "strict": true }}
-
Use the
--noEmitOnError
flag to prevent TypeScript from compiling when there are errors. -
Take advantage of TypeScript’s built-in utility types like
Partial\<T\>
,Required\<T\>
, andPick\<T\>
to handle complex type transformations.
Remember, TypeScript’s type system is your friend, not your enemy. While it might seem strict at first, it helps catch errors before they make it to production, saving you valuable debugging time later.
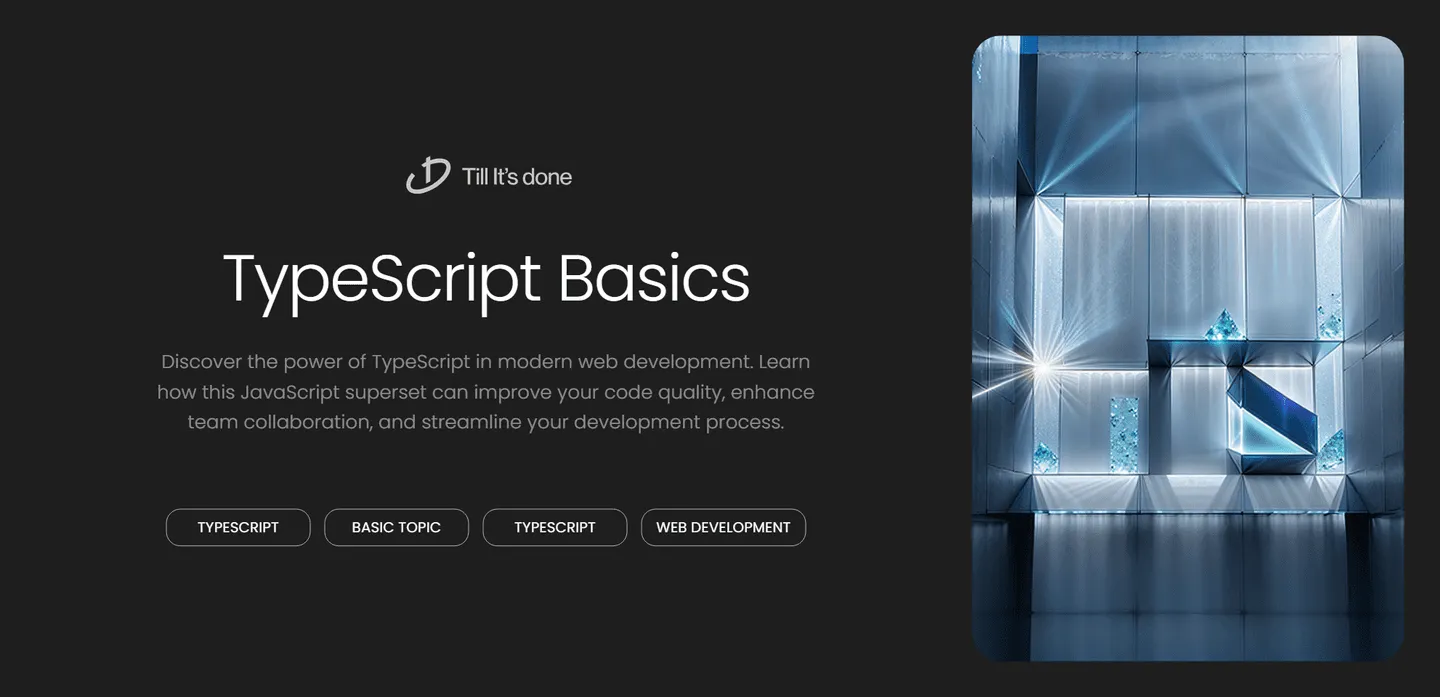
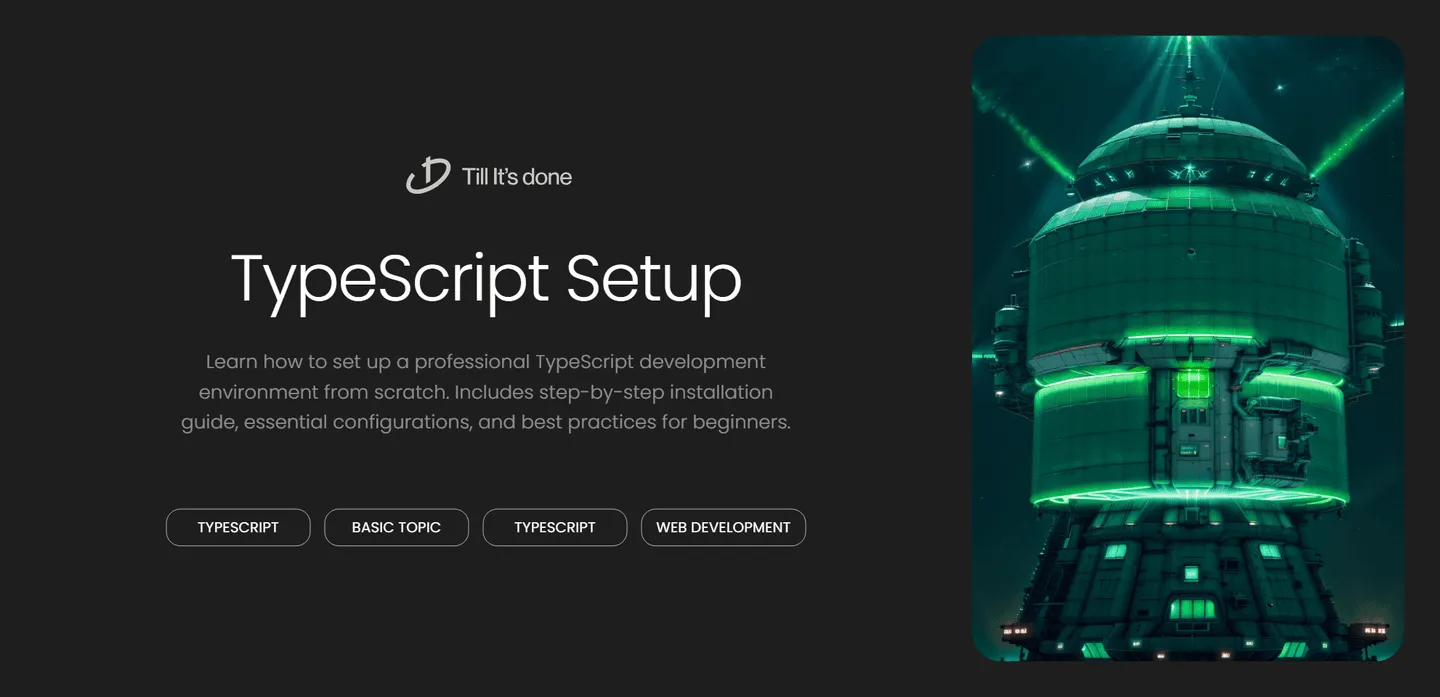
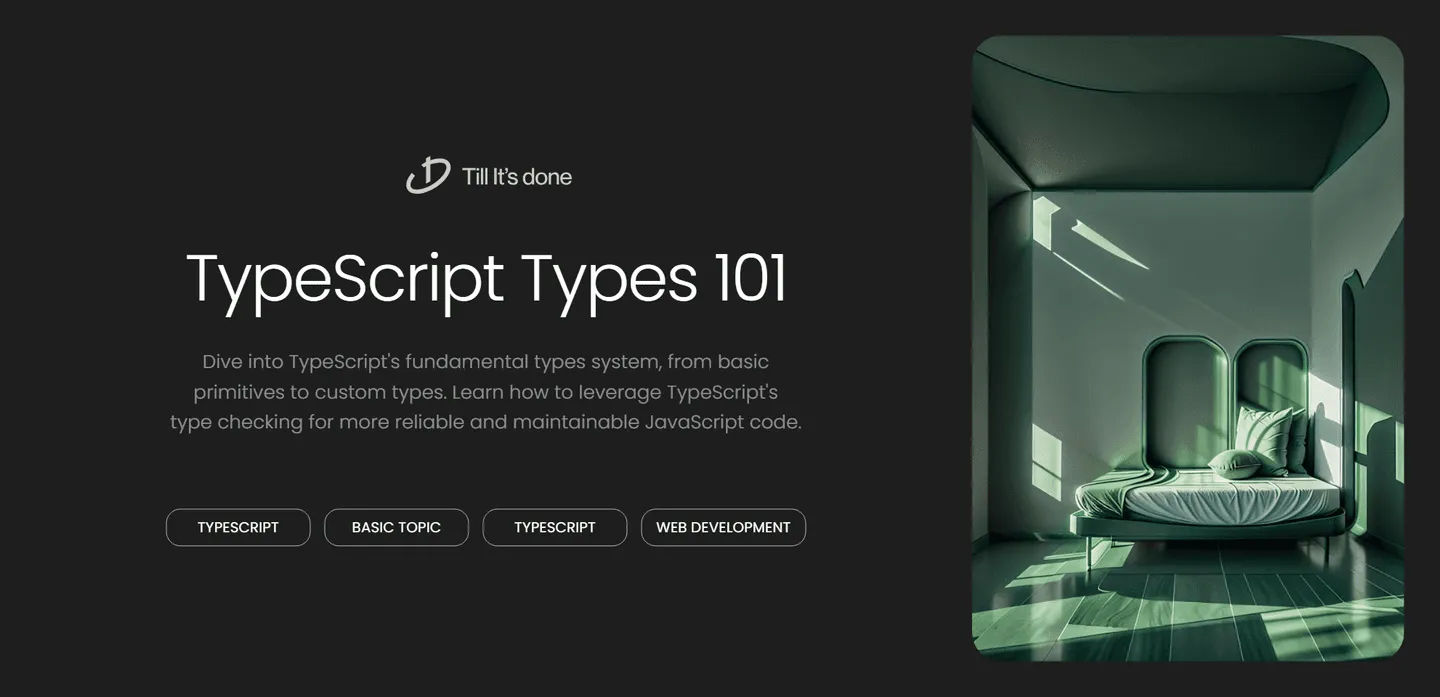
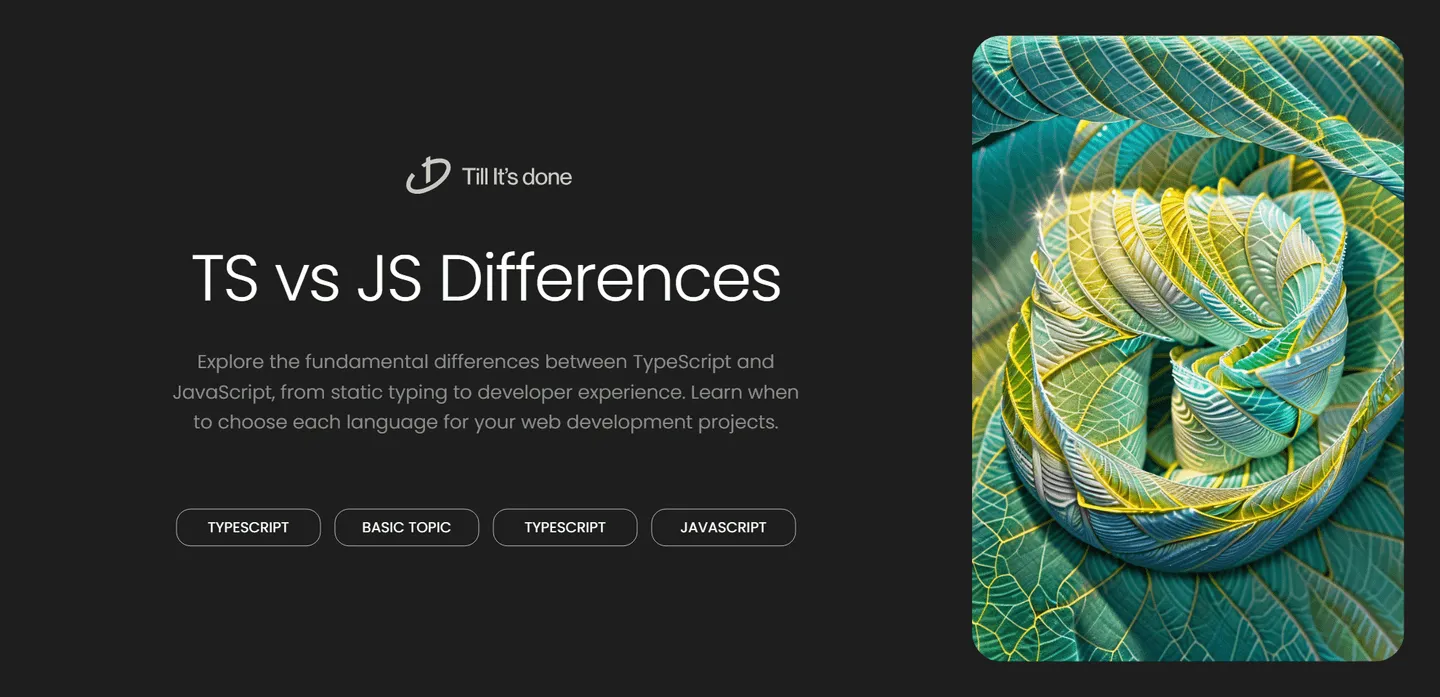
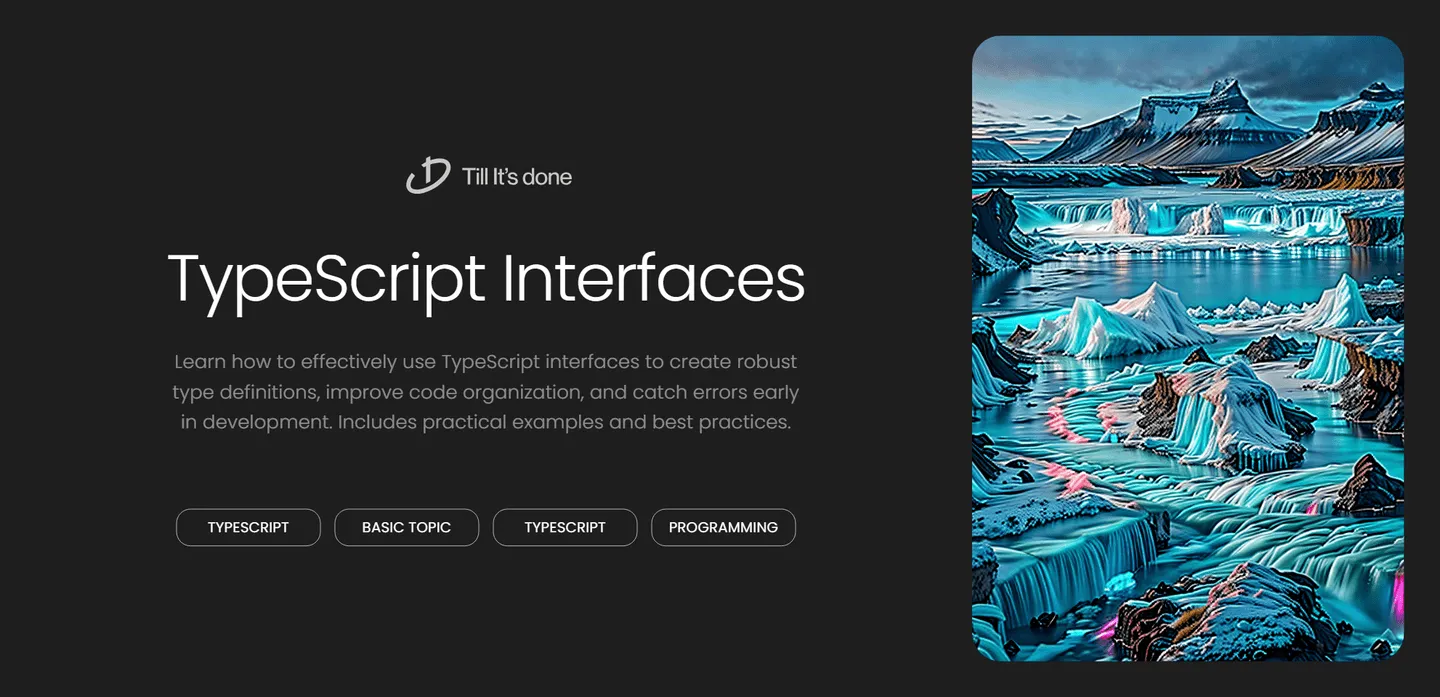
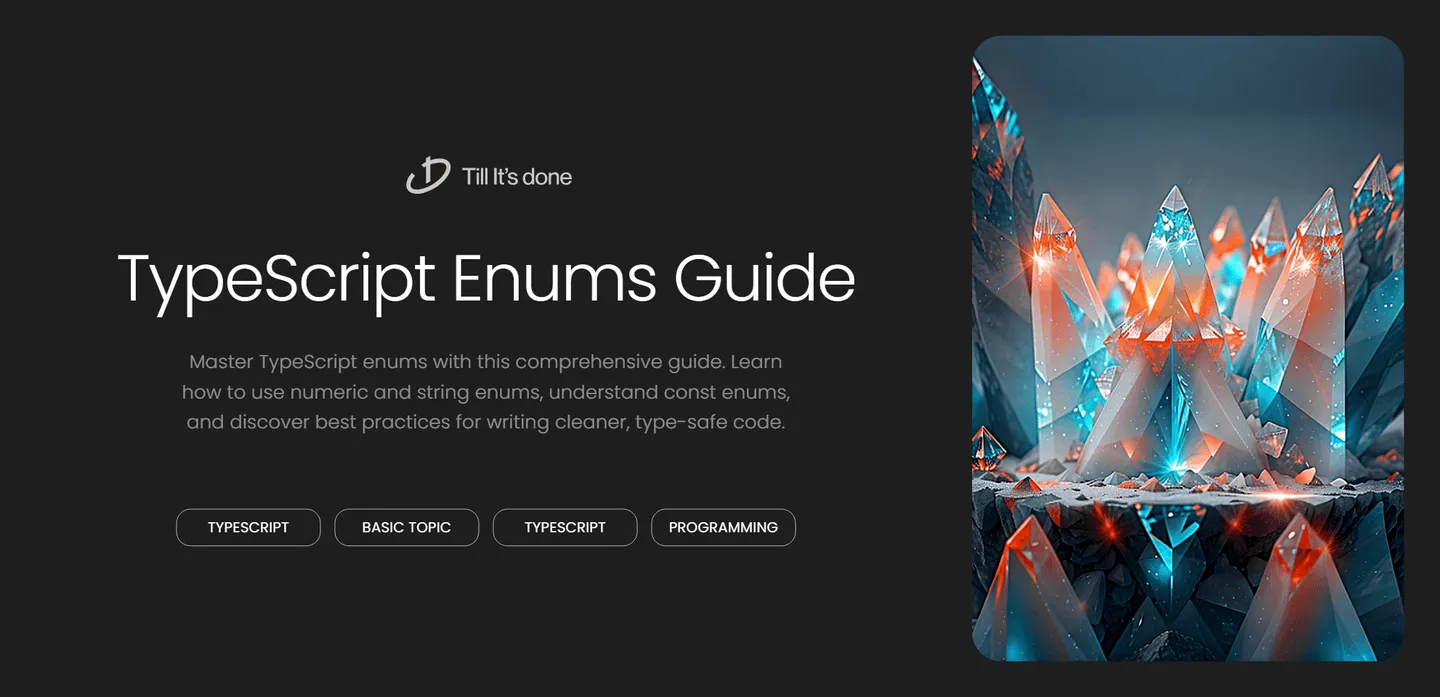
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.