- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Best Practices: Dates & Times in Node.js with Day.js
Learn essential best practices, avoid common pitfalls, and implement production-ready solutions for robust temporal operations.

Best Practices for Handling Dates and Times in Node.js with Day.js
Working with dates and times in JavaScript has always been a bit tricky. While the native Date object gets the job done, it’s not the most developer-friendly solution out there. That’s where Day.js comes in – a lightweight alternative to Moment.js that makes handling dates and times in Node.js applications a breeze.
Why Choose Day.js?
When I first started working with dates in Node.js, I was using the native Date object. It worked, but I often found myself writing extra code for basic operations. Day.js changed all that. At just 2KB minified and gzipped, it’s incredibly lightweight yet powerful enough to handle most date-time manipulation needs.
Essential Best Practices
1. Always Parse Dates with a Format String
Instead of leaving date parsing to chance, always specify the expected format:
// Good practiceconst date = dayjs('2024-03-15', 'YYYY-MM-DD')
// Avoidconst date = dayjs('2024-03-15')
2. Use UTC for Consistency
When dealing with users across different time zones, UTC is your friend:
const utcDate = dayjs.utc()
3. Leverage Immutability
One of the best features of Day.js is that it’s immutable by default. Each operation returns a new instance:
const today = dayjs()const tomorrow = today.add(1, 'day')// today remains unchanged
4. Plugin Management
Keep your bundle size small by only importing the plugins you need:
import utc from 'dayjs/plugin/utc'import timezone from 'dayjs/plugin/timezone'dayjs.extend(utc)dayjs.extend(timezone)
Common Pitfalls to Avoid
- Don’t mix different date libraries in the same project. Stick to Day.js throughout your codebase for consistency.
- Always validate dates before operations:
const userInput = '2024-13-45' // invalid dateconst isValid = dayjs(userInput).isValid() // check before using
- Remember that months are zero-indexed when manually creating dates:
// December is 11, not 12const december = dayjs('2024-12-25')
Advanced Tips for Production
For production applications, consider these advanced practices:
- Use relative time calculations for better user experience:
dayjs().from(dayjs('2024-01-01')) // "2 months ago"
- Implement proper error handling for date operations:
try { const date = dayjs(userInput) if (!date.isValid()) { throw new Error('Invalid date') }} catch (error) { // Handle the error appropriately}
- Cache frequently used date calculations to improve performance.
Remember, the key to successful date handling is consistency and validation. By following these practices, you’ll avoid the common pitfalls that come with date manipulation in Node.js applications.

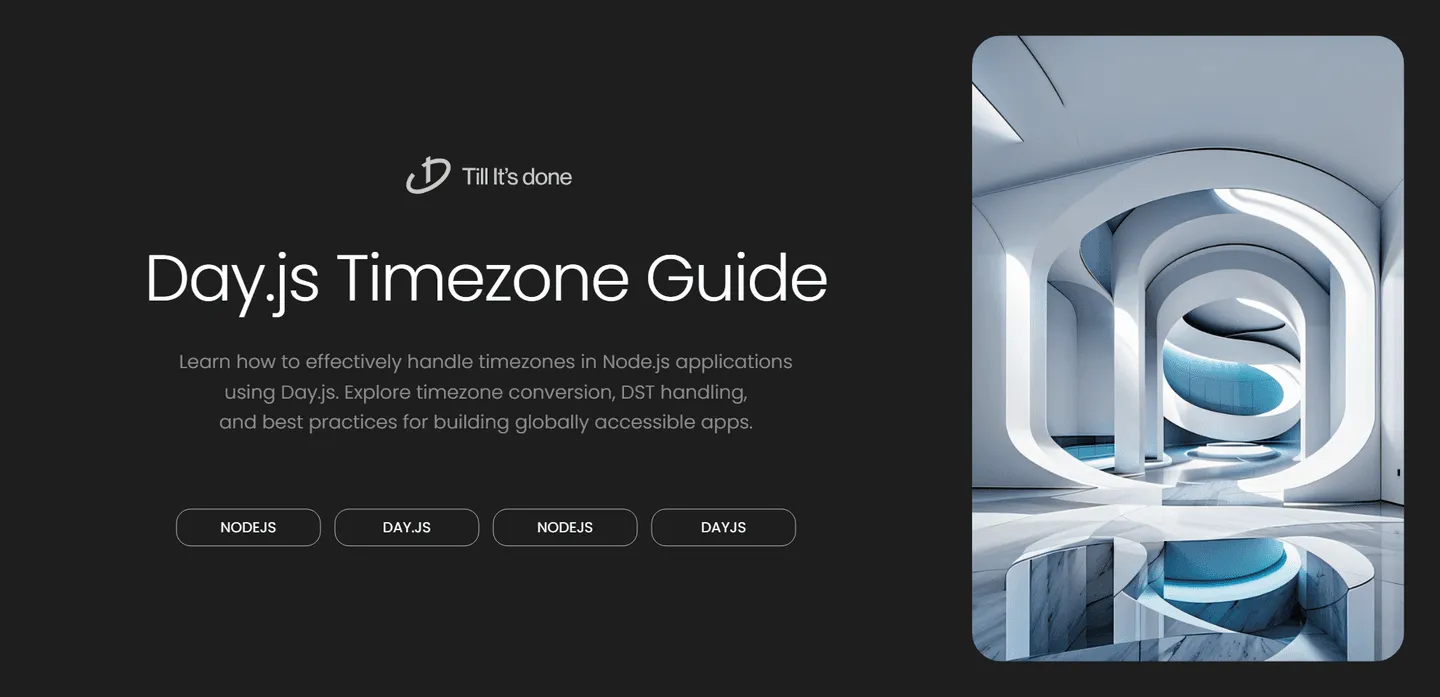




Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.