- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Building Custom Validation Rules with Zod in Node.js
Discover type-safe validation patterns, async validation, and best practices for data validation.
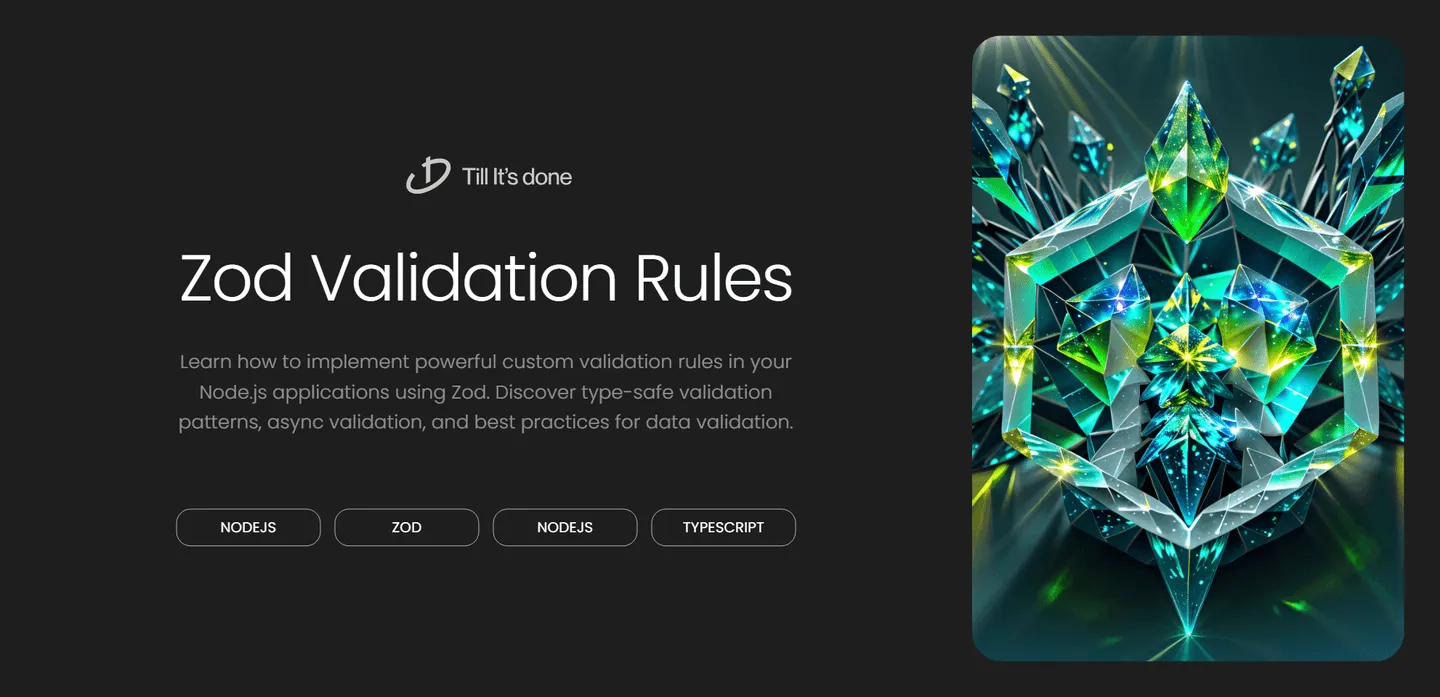
Building Custom Validation Rules with Zod in Node.js
In the ever-evolving landscape of Node.js development, data validation remains a crucial aspect of building robust applications. Enter Zod – a TypeScript-first schema validation library that’s revolutionizing how we handle data validation in our Node.js applications. Today, we’ll dive deep into creating custom validation rules with Zod and explore how it can make our validation logic both powerful and maintainable.
Understanding Zod’s Fundamentals
At its core, Zod provides an elegant way to define schemas and validate data against them. Think of it as your data’s bouncer – checking IDs and ensuring only the right data gets through to your application. What sets Zod apart is its exceptional TypeScript integration and intuitive API.
Building Your First Custom Validator
Let’s start with a practical example. Imagine you’re building an e-commerce platform where product SKUs must follow a specific pattern. Here’s how you might create a custom validator for that:
import { z } from "zod";
const ProductSchema = z.object({ sku: z.string().refine( (val) => /^PRD-[A-Z]{2}-\d{4}$/.test(val), { message: "SKU must follow pattern: PRD-XX-0000" } ), name: z.string().min(3), price: z.number().positive()});
Advanced Custom Validation Techniques
As your application grows, you’ll need more sophisticated validation rules. Zod shines in these scenarios by allowing you to chain multiple refinements and create reusable validation patterns.
Async Validation Rules
Sometimes, we need to validate data against external sources, like checking if a username is unique in our database:
const UserSchema = z.object({ username: z.string().refine( async (username) => { // Simulate database check const isUnique = await checkUsernameInDatabase(username); return isUnique; }, { message: "Username already taken" } )});
Best Practices and Tips
- Keep your validation rules modular and reusable
- Use meaningful error messages that guide users
- Combine multiple refinements for complex validations
- Leverage TypeScript’s type inference with Zod
- Consider performance implications for async validators
Error Handling and Custom Messages
Zod provides extensive error handling capabilities. You can customize error messages and create structured error responses that make sense for your application:
const validateData = (data: unknown) => { try { const result = ProductSchema.parse(data); return { success: true, data: result }; } catch (error) { if (error instanceof z.ZodError) { return { success: false, errors: error.errors.map(err => ({ path: err.path.join('.'), message: err.message })) }; } throw error; }};
Conclusion
Custom validation with Zod brings type safety, flexibility, and maintainability to your Node.js applications. By leveraging its powerful features, you can create robust validation rules that grow with your application’s needs.
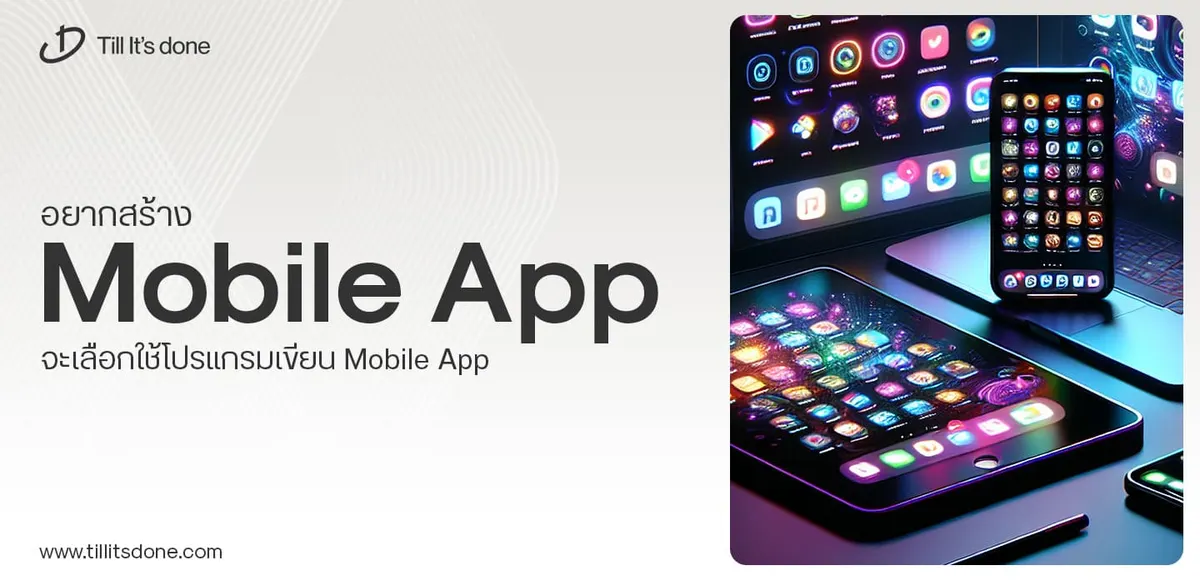
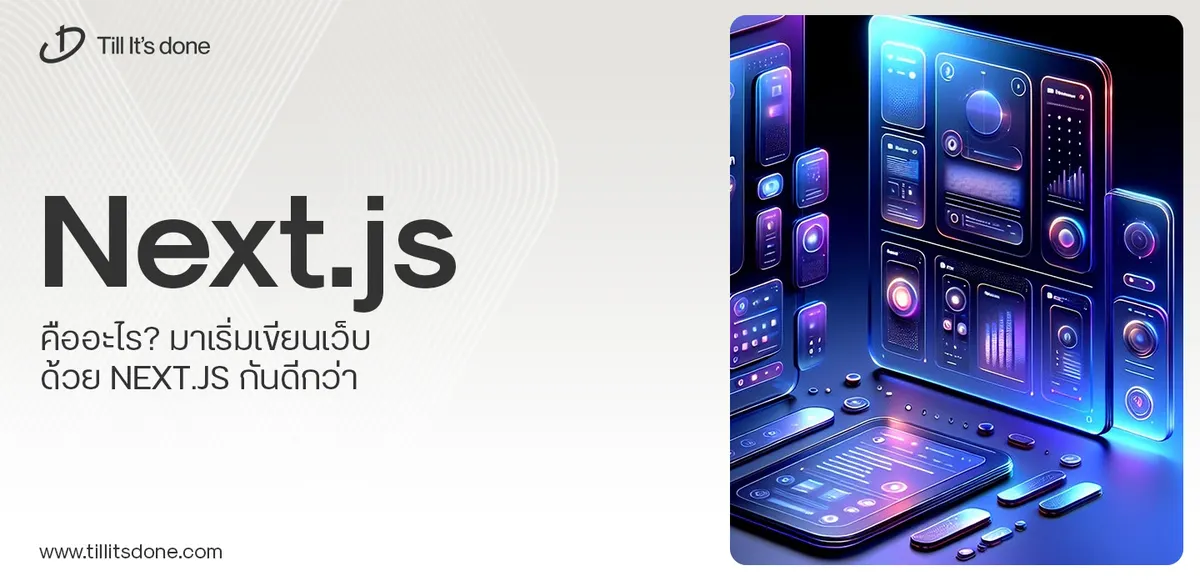
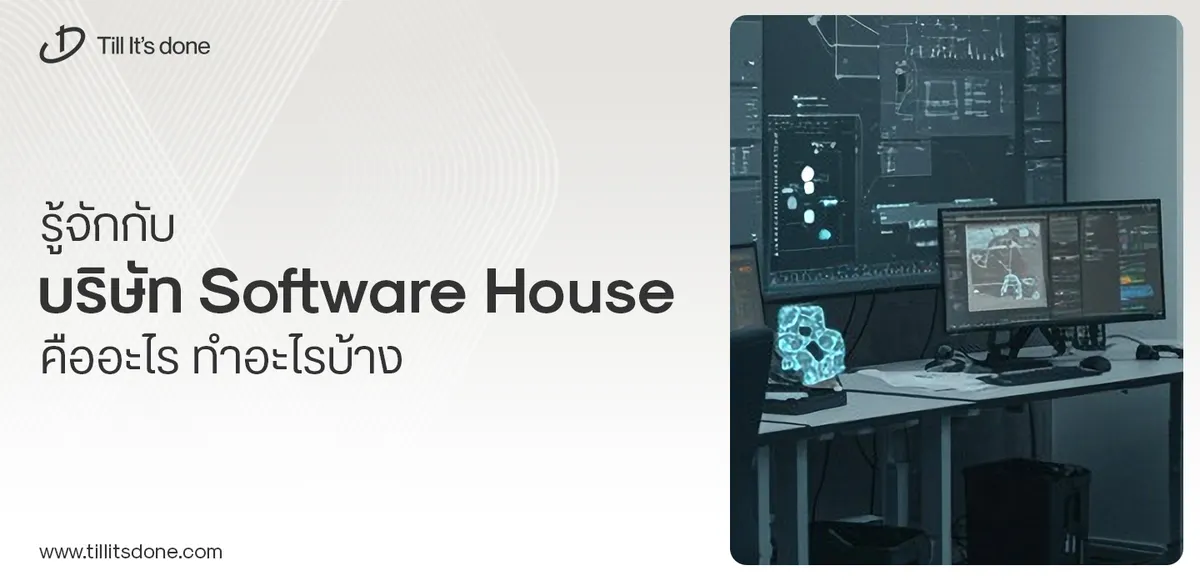
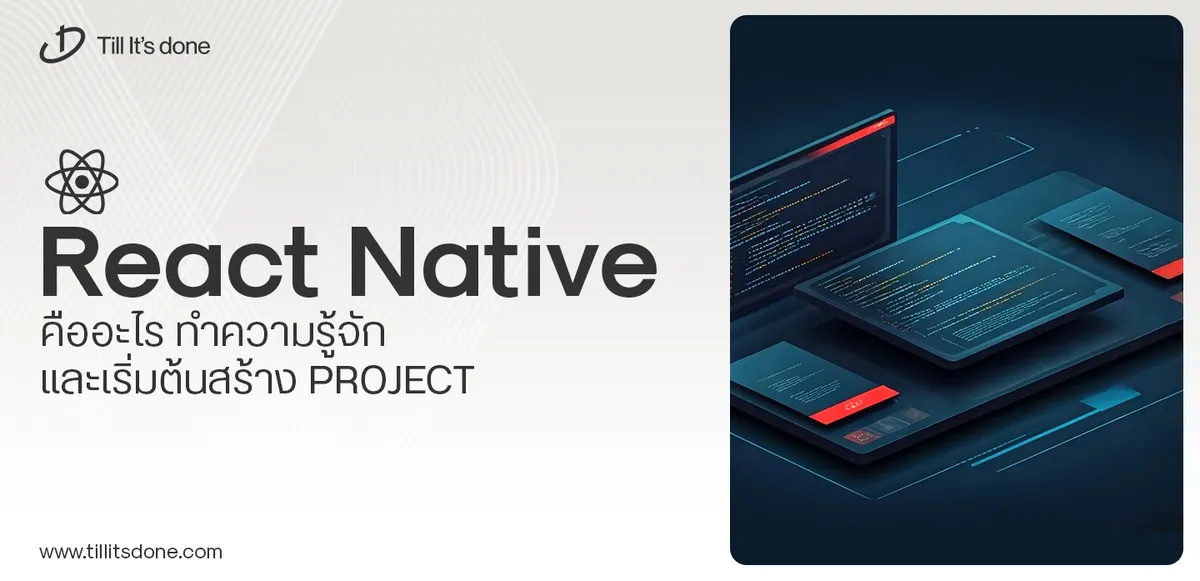
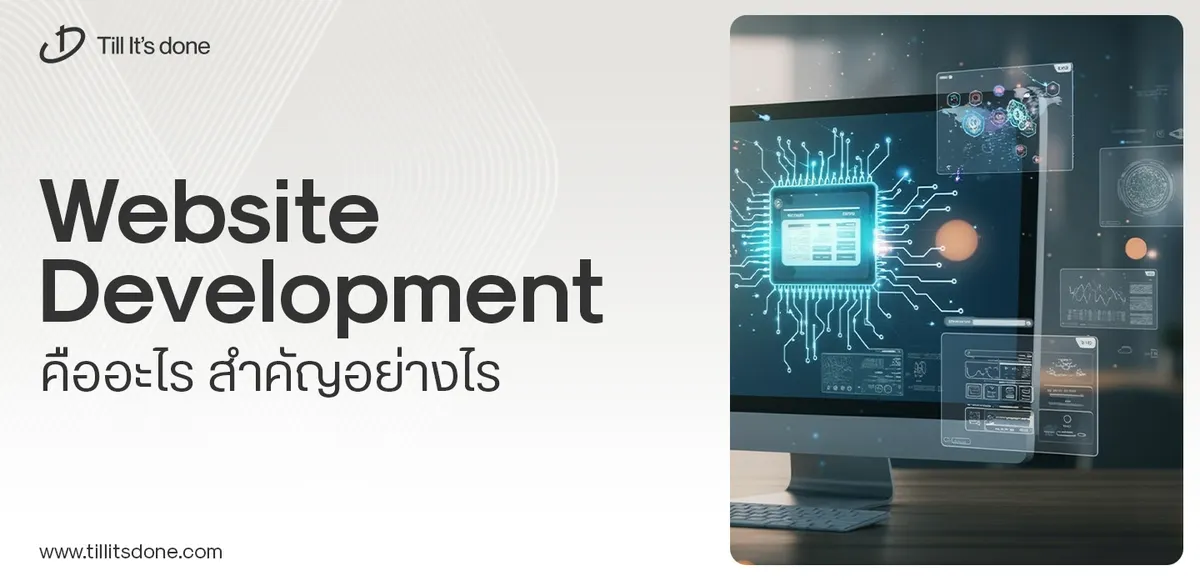
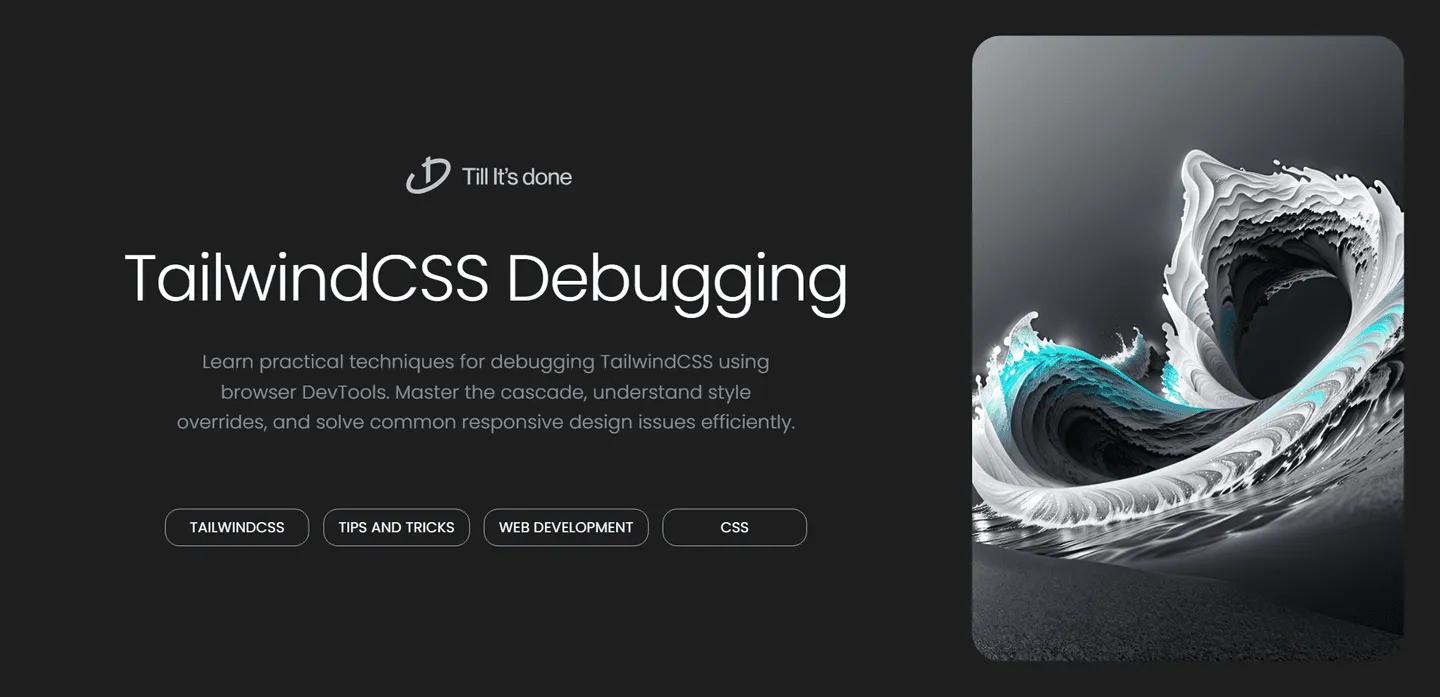
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.