- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Custom Hooks for Framer Motion: Reusable Animations
Discover practical examples and best practices for building maintainable animated applications.
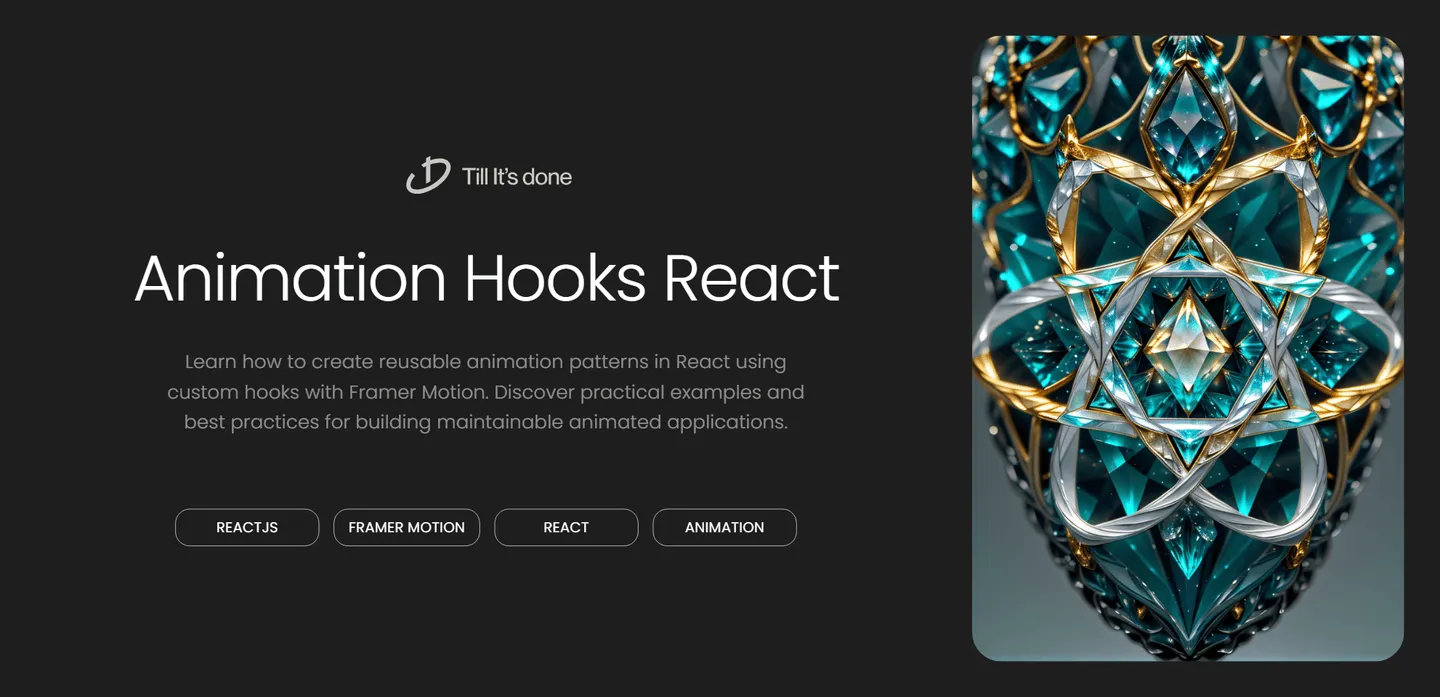
Custom Hooks for Framer Motion: Reusability in Animated React Apps
Building engaging animations in React applications has never been more enjoyable than with Framer Motion. However, as your projects grow, you might find yourself repeating animation logic across different components. This is where custom hooks come in – they’re your secret weapon for creating reusable, maintainable animation code.
Why Custom Hooks for Animations?
Think of custom hooks as your animation recipe book. Instead of writing the same animation logic repeatedly, you can package it into a neat, reusable function. This approach not only keeps your code DRY but also makes it more maintainable and easier to update across your entire application.
Creating Your First Animation Hook
Let’s dive into a practical example. Here’s a custom hook that handles a fade-in animation – something you might use across multiple components:
import { useAnimation } from "framer-motion";import { useInView } from "react-intersection-observer";import { useEffect } from "react";
export const useFadeInAnimation = () => { const controls = useAnimation(); const [ref, inView] = useInView({ threshold: 0.2, triggerOnce: true });
useEffect(() => { if (inView) { controls.start({ opacity: 1, y: 0, transition: { duration: 0.5 } }); } }, [controls, inView]);
return { ref, animate: controls, initial: { opacity: 0, y: 20 } };};
Real-World Applications
The beauty of custom hooks lies in their versatility. Let’s explore another powerful example – a hook for creating smooth hover animations:
export const useHoverAnimation = () => { const [hover, setHover] = useState(false);
const animation = { scale: hover ? 1.05 : 1, transition: { type: "spring", stiffness: 300 } };
return { animation, onHoverStart: () => setHover(true), onHoverEnd: () => setHover(false) };};
Best Practices for Animation Hooks
- Keep them focused: Each hook should handle one specific animation pattern
- Make them configurable: Allow customization through parameters
- Consider performance: Use
useMemo
for complex calculations - Handle cleanup: Don’t forget to clean up any subscriptions or listeners
Implementation Tips
When implementing these hooks in your components, remember to keep your animation values consistent across your application. Here’s how you might use our fade-in hook:
const MyComponent = () => { const fadeAnimation = useFadeInAnimation();
return ( <motion.div ref={fadeAnimation.ref} initial={fadeAnimation.initial} animate={fadeAnimation.animate} > <h2>Content that fades in</h2> </motion.div> );};
Conclusion
Custom hooks are your pathway to creating more maintainable and scalable animated React applications. They encapsulate animation logic in a reusable way, making your codebase cleaner and more efficient. Start small, experiment with different patterns, and gradually build up your library of animation hooks.
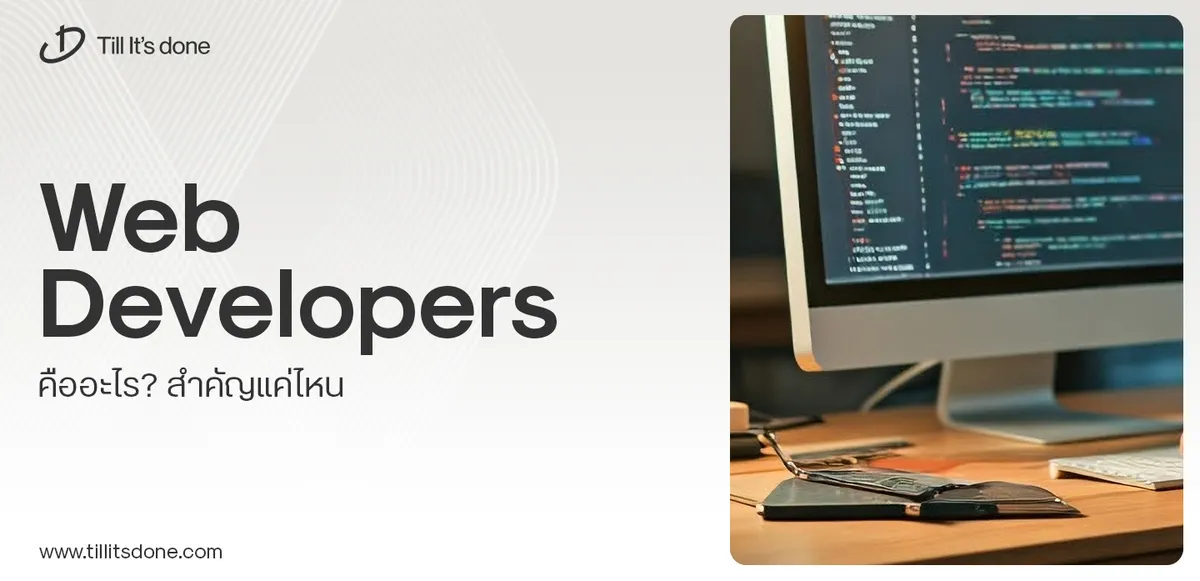
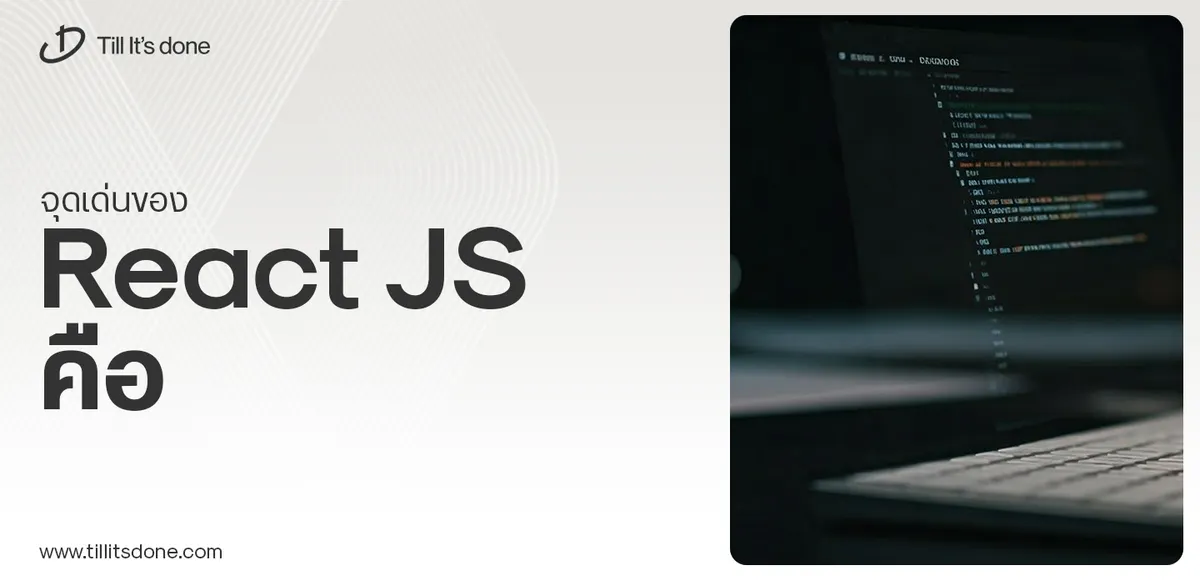
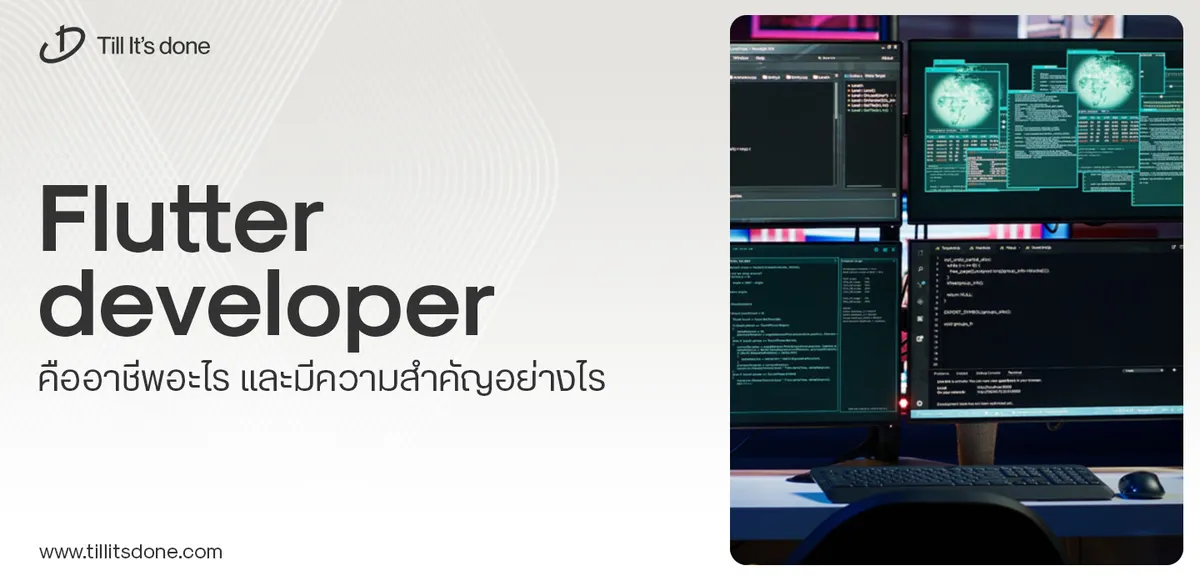
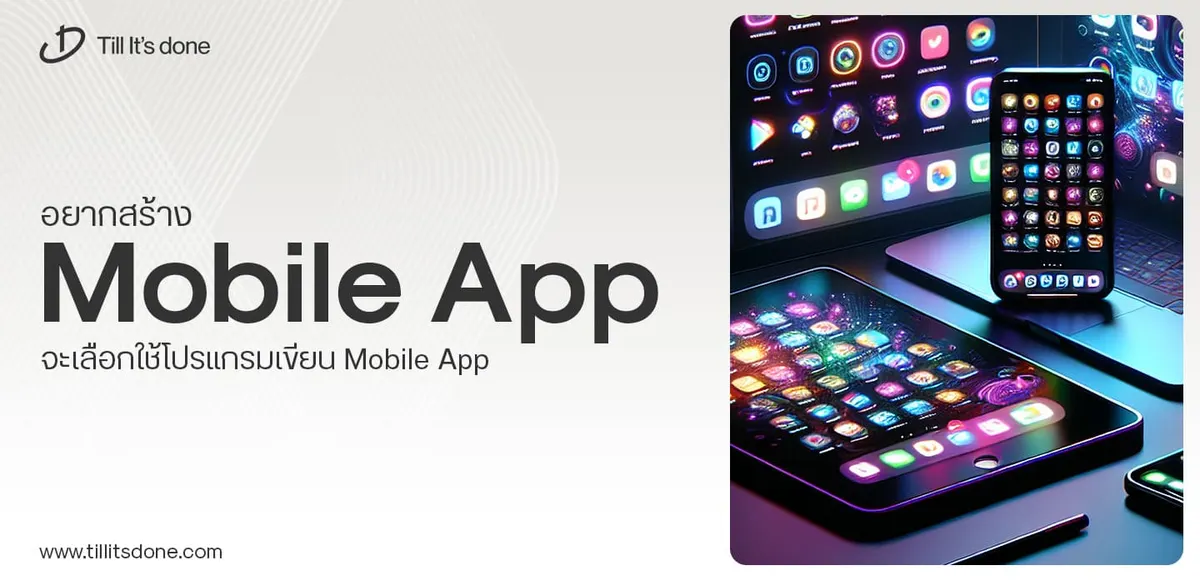
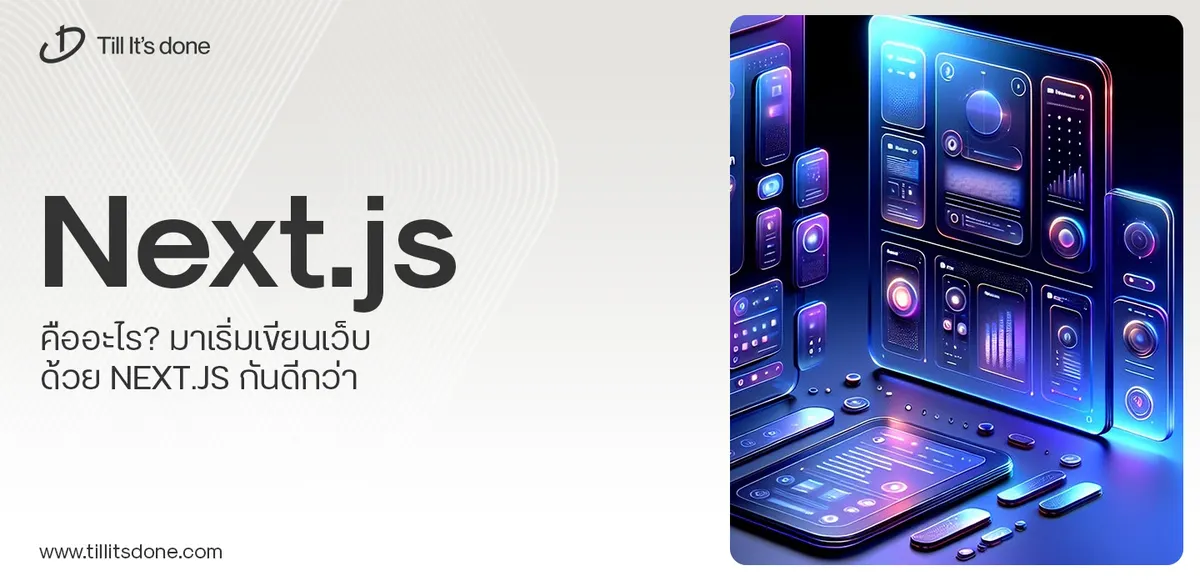
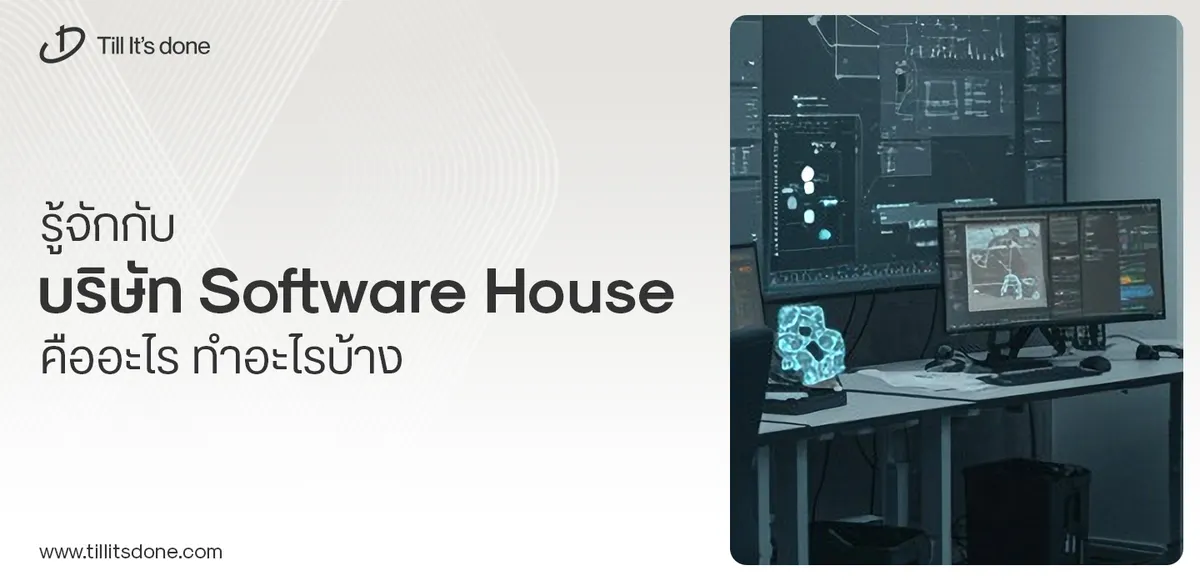
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.