- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Introduction to CSS grid-auto-flow Enhance Grid Layouts
Explore options like row, column, dense, row dense, and column dense.
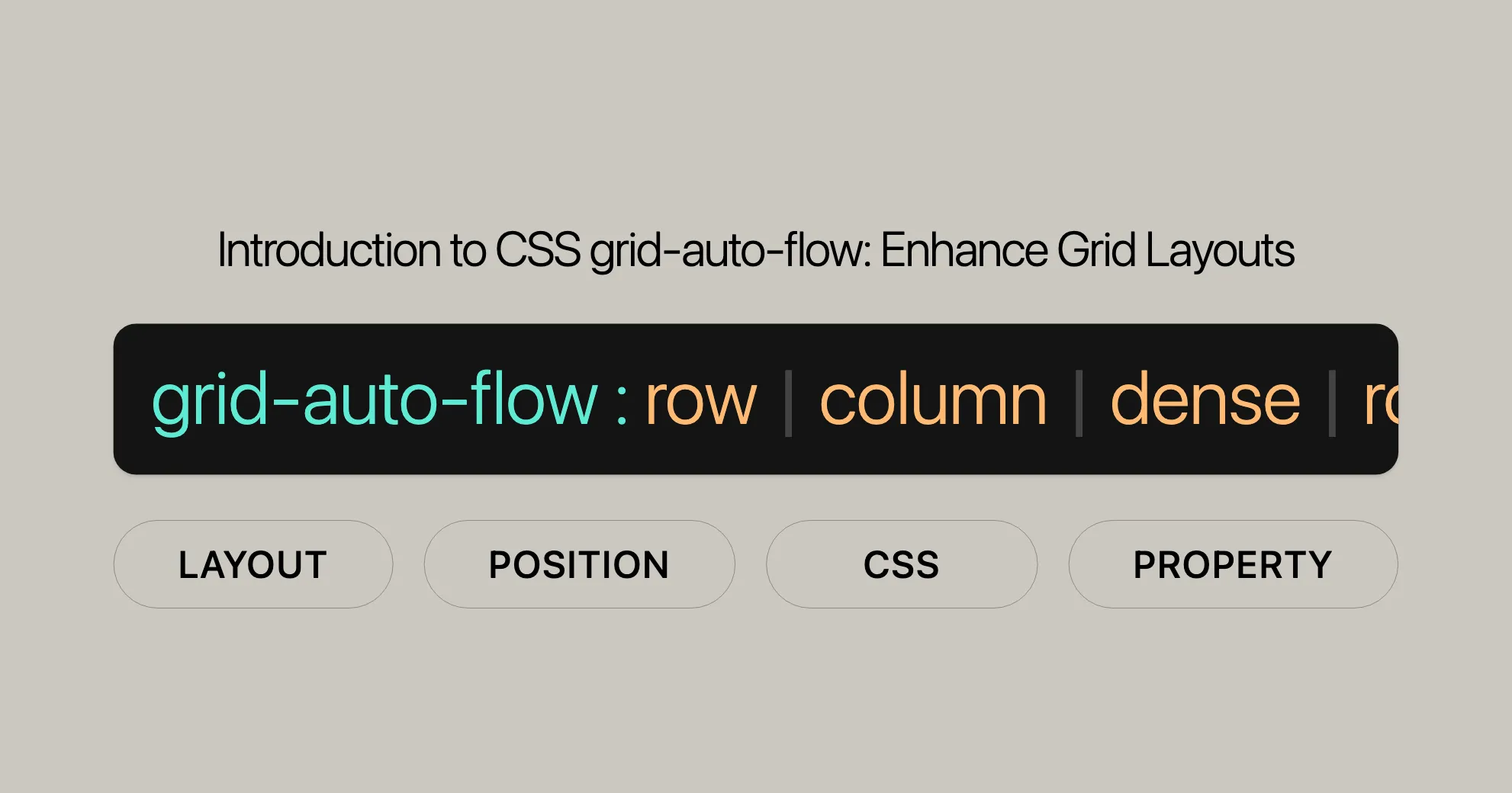
Introduction to grid-auto-flow
The grid-auto-flow
property in CSS is a handy tool for controlling how items are placed within a grid layout. It helps you manage the auto-placement algorithm efficiently, making your web development and design more flexible.
By default, grid items are placed in a row-based flow, moving from left to right. But grid-auto-flow
offers additional options like column
, row dense
, and column dense
, giving you more flexibility in arranging items.
In this article, we’ll explore the various aspects of the grid-auto-flow
property, including its syntax, values, and practical examples. By the end, you’ll have a solid understanding of how to use this property to create responsive and visually appealing grid layouts.
Baseline Widely Available
The grid-auto-flow
property is well-established and works across many devices and browser versions. It has been available since July 2020, ensuring compatibility across a wide range of browsers. This means you can confidently use grid-auto-flow
in your web development projects without worrying about browser support issues.
For more detailed information on compatibility, you can refer to the following resources:
This wide availability makes grid-auto-flow
a reliable choice for enhancing your grid layouts and improving your web design projects.
Syntax and Values
The grid-auto-flow
property in CSS controls how auto-placed items are arranged within a grid. Understanding the syntax and values will help you effectively use this property in your web development projects.
Syntax
The grid-auto-flow
property can be set using the following syntax:
grid-auto-flow: row | column | dense | row dense | column dense;
Values
Here are the possible values for the grid-auto-flow
property:
- row: Items are placed by filling each row in turn, adding new rows as necessary. This is the default value if neither
row
norcolumn
is specified. - column: Items are placed by filling each column in turn, adding new columns as necessary.
- dense: The “dense” packing algorithm attempts to fill in holes earlier in the grid if smaller items come up later. This may cause items to appear out of order to fill gaps left by larger items.
- row dense: Items are placed by filling each row in turn, and the “dense” algorithm is used to fill in any holes.
- column dense: Items are placed by filling each column in turn, and the “dense” algorithm is used to fill in any holes.
Example
Here’s a basic example to demonstrate the use of the grid-auto-flow
property:
HTML
<div id="grid"> <div id="item1"></div> <div id="item2"></div> <div id="item3"></div> <div id="item4"></div> <div id="item5"></div></div><select id="direction"> <option value="column">column</option> <option value="row">row</option></select><input id="dense" type="checkbox" /><label for="dense">dense</label>
CSS
#grid { height: 200px; width: 200px; display: grid; gap: 10px; grid-template: repeat(4, 1fr) / repeat(2, 1fr); grid-auto-flow: column; /* or 'row', 'row dense', 'column dense' */}
#item1 { background-color: lime; grid-row-start: 3;}
#item2 { background-color: yellow;}
#item3 { background-color: blue;}
#item4 { grid-column-start: 2; background-color: red;}
#item5 { background-color: aqua;}
JavaScript
function changeGridAutoFlow() { const grid = document.getElementById("grid"); const direction = document.getElementById("direction"); const dense = document.getElementById("dense"); let gridAutoFlow = direction.value === "row" ? "row" : "column";
if (dense.checked) { gridAutoFlow += " dense"; }
grid.style.gridAutoFlow = gridAutoFlow;}
const selectElem = document.querySelector("select");const inputElem = document.querySelector("input");selectElem.addEventListener("change", changeGridAutoFlow);inputElem.addEventListener("change", changeGridAutoFlow);
By using the grid-auto-flow
property, you can create dynamic and flexible grid layouts that adapt to different screen sizes and content requirements.
Formal Definition
The grid-auto-flow
property in CSS is formally defined to control the auto-placement algorithm for grid items. This property determines how items are automatically placed within a grid container when their positions are not explicitly specified.
Initial Value
The initial value for the grid-auto-flow
property is row
. This means that, by default, items will flow in rows, filling each row in turn and adding new rows as necessary.
Applies To
The grid-auto-flow
property applies to grid containers. These are the elements that have their display
property set to grid
.
Inherited
The grid-auto-flow
property is not inherited. This means that the value set for a grid container does not automatically apply to its child elements unless explicitly specified.
Computed Value
The computed value for grid-auto-flow
is the same as the specified value. There is no additional computation or interpretation applied to the value.
Animation Type
The animation type for grid-auto-flow
is discrete. This means that the property does not support smooth transitions between values; changes occur instantly.
Formal Syntax
The formal syntax for the grid-auto-flow
property is as follows:
grid-auto-flow = [ row | column ] || dense
This syntax indicates that the property can take one or two keyword values. The first keyword specifies the direction (row
or column
), and the second keyword specifies the packing algorithm (dense
).
Summary
Understanding the formal definition of the grid-auto-flow
property helps in effectively controlling the layout of grid items. By specifying the direction and packing algorithm, you can create complex and responsive grid layouts that adapt to various content and screen sizes. This makes grid-auto-flow
a powerful tool for web developers and designers aiming to enhance their grid-based layouts.
Examples of grid-auto-flow
To better understand how the grid-auto-flow
property works in practice, let’s explore some examples that demonstrate its various values and their effects on grid layouts.
Setting Grid Auto-Placement
In this example, we will create a simple grid container with five items. We will use a dropdown menu to change the grid-auto-flow
value between row
and column
, and a checkbox to toggle the dense
packing algorithm.
Result
In this example, you can see how changing the grid-auto-flow
value affects the layout of the grid items. When the value is set to column
, items are placed top to bottom, then left to right. When the value is set to row
, items are placed left to right, then top to bottom. The dense
packing algorithm can be toggled to fill in any holes in the grid, potentially causing items to appear out of order.
Additional Examples
Example 1: Grid Auto-Flow Column
In this example, the grid-auto-flow
property is set to column
. Items are packed top to bottom, then left to right.
HTML
<div class="grid-container"> <div>1</div> <div>2</div> <div>3</div> <div>4</div> <div>5</div> <div>6</div> <div>7</div> <div>8</div> <div>9</div> <div>10</div></div>
CSS
.grid-container { background-color: steelblue; padding: 10px; display: grid; grid-gap: 10px; grid-template-rows: auto auto auto; grid-auto-flow: column;}
.grid-container > div { background-color: aliceblue; text-align: center; padding: 15px 5px; font-size: 18px;}
Example 2: Grid Auto-Flow Row Dense
In this example, the grid-auto-flow
property is set to row dense
. Items are placed left to right, then top to bottom, and any holes in the grid are filled using the dense packing algorithm.
HTML
<div class="grid-container-dense"> <div>1</div> <div>2</div> <div>3</div> <div>4</div> <div>5</div> <div>6</div> <div>7</div> <div>8</div> <div>9</div> <div>10</div></div>
CSS
.grid-container-dense { background-color: steelblue; padding: 10px; display: grid; grid-gap: 10px; grid-template-rows: auto auto auto; grid-auto-flow: row dense;}
.grid-container-dense > div { background-color: aliceblue; text-align: center; padding: 15px 5px; font-size: 18px;}
Conclusion
The grid-auto-flow
property provides a powerful way to control the placement of items within a grid layout. By experimenting with the various values and packing algorithms, you can create dynamic and responsive grid layouts that adapt to different content and screen sizes. Whether you choose row
, column
, or a dense packing algorithm, grid-auto-flow
offers the flexibility and control needed to enhance your web development and design projects.
Specifications
The grid-auto-flow
property is part of the CSS Grid Layout Module Level 2 specification. This specification outlines the rules and behaviors for grid layouts in CSS, including how auto-placed items are managed within a grid container.
Specification Details
- Specification Name: CSS Grid Layout Module Level 2
- Property:
grid-auto-flow
- Link: CSS Grid Layout Module Level 2 - grid-auto-flow property
This specification provides a comprehensive overview of the grid-auto-flow
property, including its syntax, values, and how it interacts with other grid properties. It ensures that web developers have a standardized way to control the auto-placement of grid items, enhancing the consistency and predictability of grid layouts across different browsers and devices.
Importance of Specifications
Adhering to specifications is crucial for web developers as it ensures that their code will work consistently across various browsers and platforms. The CSS Grid Layout Module Level 2 specification for grid-auto-flow
guarantees that the property behaves as expected, allowing developers to create reliable and functional grid layouts without worrying about cross-browser compatibility issues.
Future-Proofing Your Code
By following the specifications, you can future-proof your code. As browsers evolve, they are more likely to align with the standards defined in these specifications. This means that your grid layouts will remain functional and compatible even as browsers update and change over time.
Staying Updated
It’s essential to stay updated with the latest specifications to take full advantage of new features and improvements. The CSS Grid Layout Module Level 2 specification is continuously updated to reflect the latest best practices and advancements in grid layout design.
Conclusion
The grid-auto-flow
property is a powerful tool for controlling the placement of items within a grid layout. By understanding its syntax, values, and practical examples, you can create dynamic and responsive grid layouts that adapt to different content and screen sizes. Whether you choose row
, column
, or a dense packing algorithm, grid-auto-flow
offers the flexibility and control needed to enhance your web development and design projects.
Browser Compatibility
The grid-auto-flow
property is widely supported across many browsers, making it a reliable choice for web development projects. Here’s what you need to know about its compatibility:
Compatibility Overview
The grid-auto-flow
property has been available across browsers since July 2020. This wide availability ensures that you can use this property with confidence, knowing that it will work consistently across various devices and browser versions.
Browser Support Details
Here is a summary of when grid-auto-flow
support started for each major browser:
- Chrome: Supported since version 57 (March 2017)
- Firefox: Supported since version 52 (March 2017)
- IE/Edge: Supported since version 16 (September 2017)
- Opera: Supported since version 44 (March 2017)
- Safari: Supported since version 10 (September 2016)
Checking Compatibility
To view detailed compatibility information, you can refer to the following resources:
Importance of Browser Compatibility
Browser compatibility is crucial for ensuring that your web designs work consistently across different platforms. Understanding the support for grid-auto-flow
in various browsers allows you to create robust and reliable grid layouts that enhance user experience.
Future-Proofing Your Code
By staying informed about browser compatibility, you can future-proof your code. As browsers evolve, they continue to support standardized properties like grid-auto-flow
. This means that your grid layouts will remain functional and compatible even as browsers update and change over time.
Testing Your Layouts
It’s always a good practice to test your grid layouts across different browsers to ensure they work as expected. Tools like BrowserStack or manual testing on various devices can help you identify any compatibility issues and make necessary adjustments.
Conclusion
The grid-auto-flow
property’s wide browser support makes it a valuable tool for web developers and designers. By understanding and ensuring compatibility, you can create dynamic, responsive, and visually appealing grid layouts that work seamlessly across different platforms.
For more detailed information on browser compatibility, refer to the resources linked above. This will help you stay updated and ensure that your grid layouts are robust and reliable.
See Also
To further enhance your understanding and use of the grid-auto-flow
property, you might find the following resources helpful:
grid-auto-rows
: Learn about specifying the size of automatically generated rows in a grid.grid-auto-columns
: Explore how to define the size of automatically generated columns in a grid.grid
: Get an overview of the CSS Grid Layout system.- Auto-placement in grid layout: Understand the auto-placement algorithm in grid layouts.
- Introducing grid auto-placement and order: Watch a video tutorial on grid auto-placement and order.
These resources provide additional insights and practical examples that can help you master the use of grid-auto-flow
and other related properties in your web development projects.
For any feedback or to report issues, you can use the following links:
By using these resources, you can ensure that your grid layouts are not only visually appealing but also functional and reliable across different platforms.
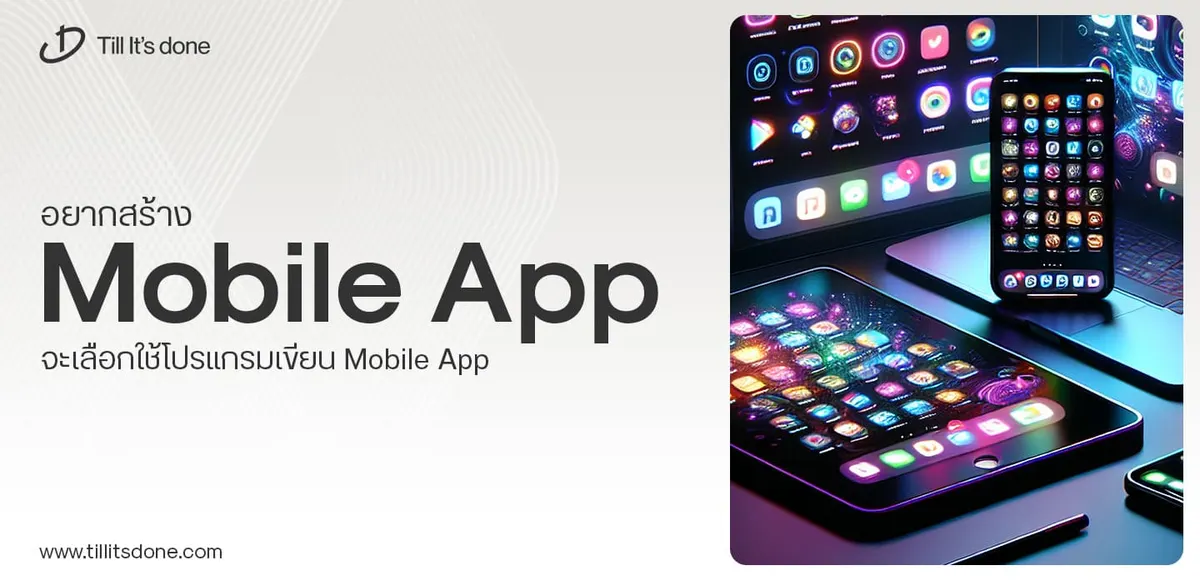
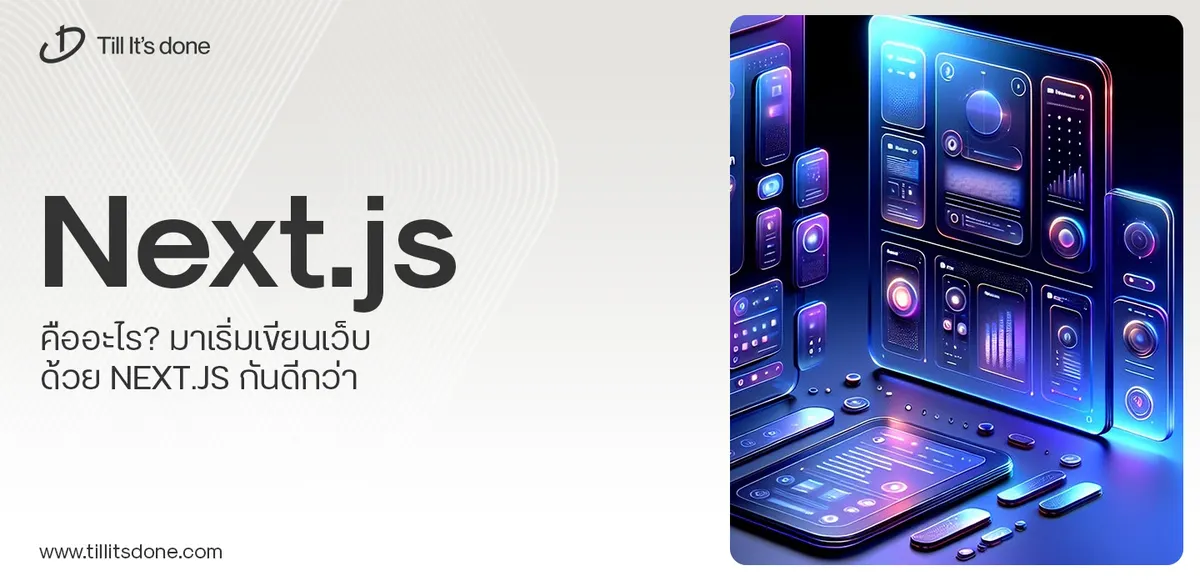
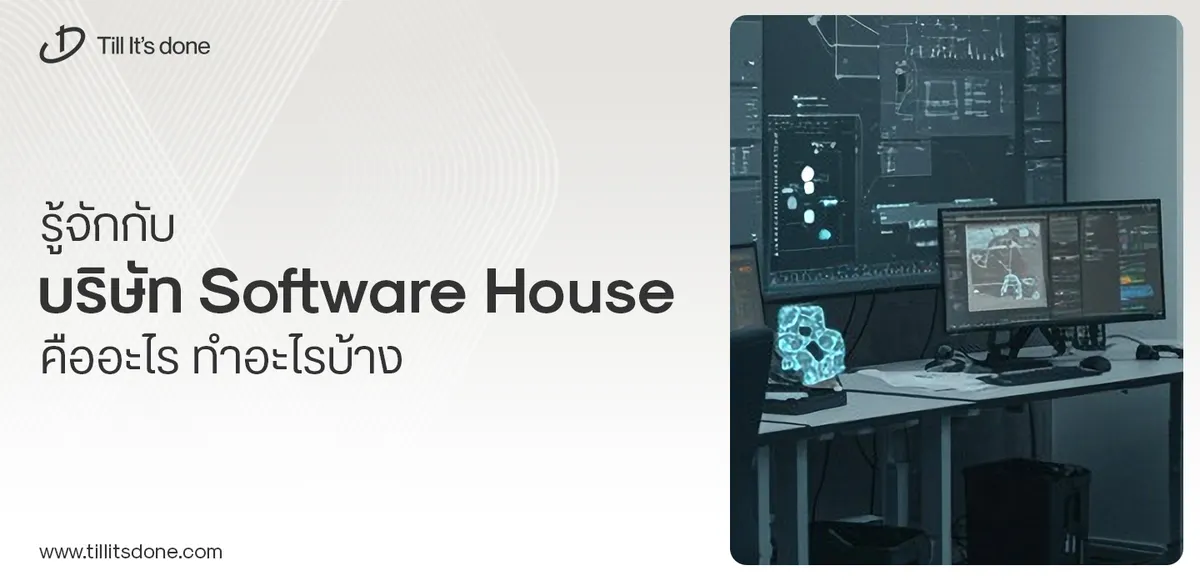
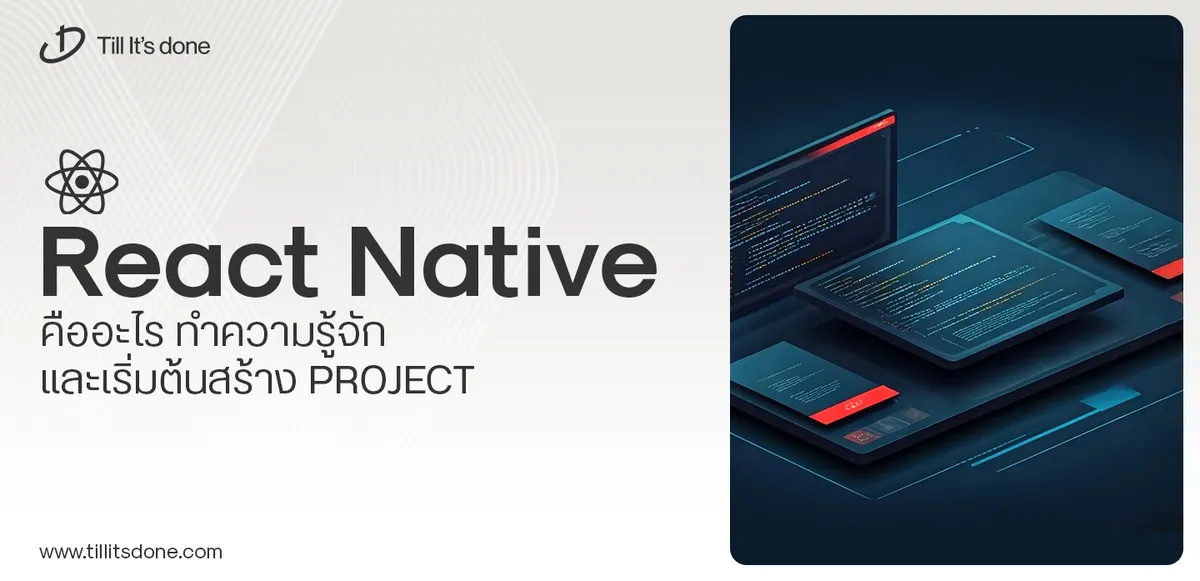
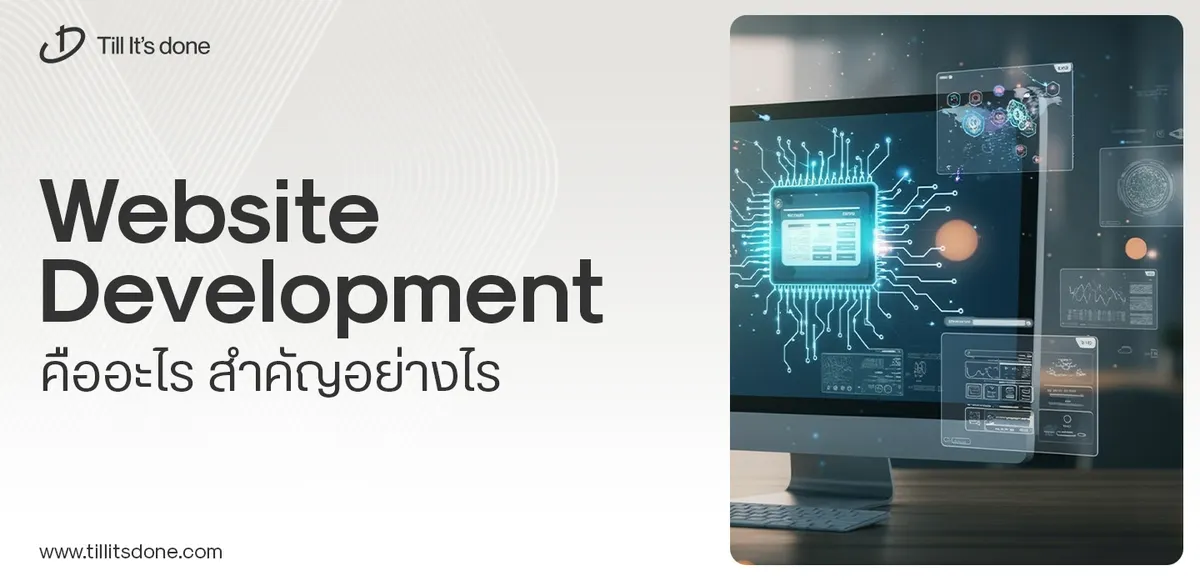
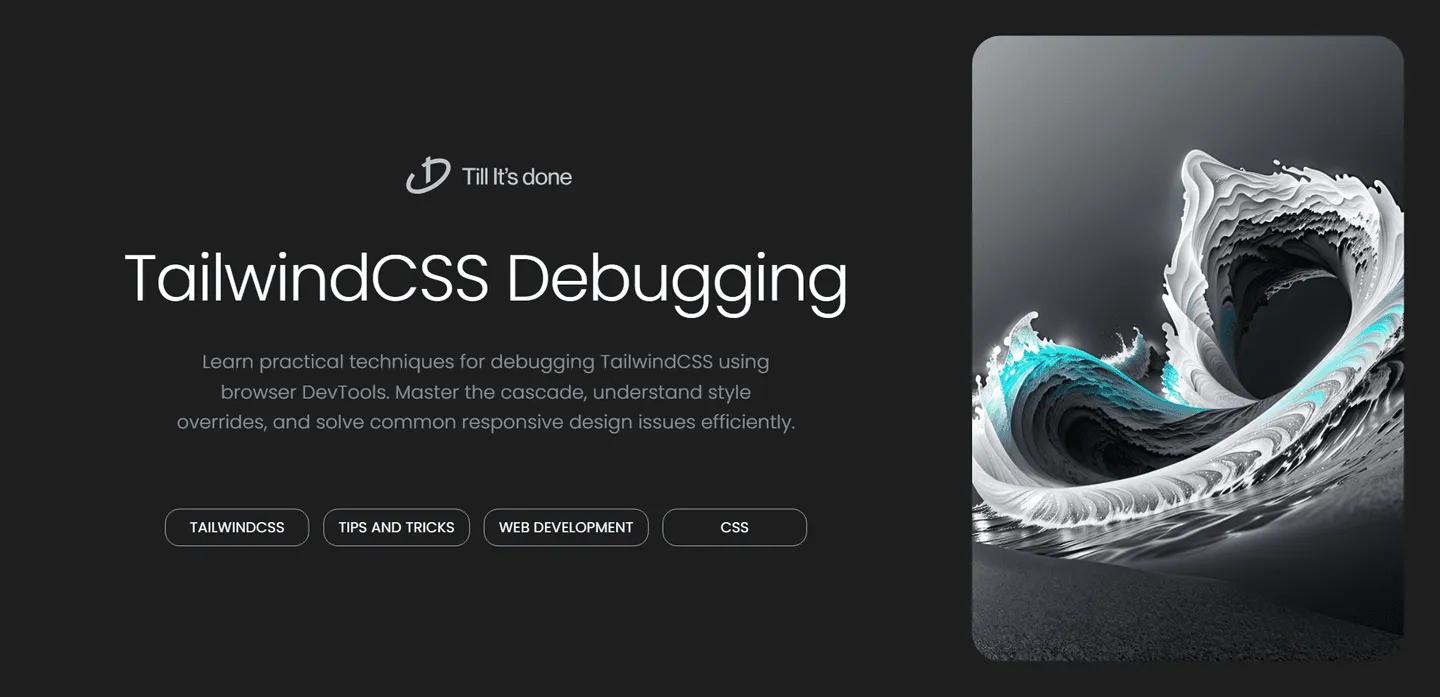
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.