- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Mastering CSS Flex-Flow for Responsive Layouts
This property combines flex-direction and flex-wrap, offering options like row, column, wrap, and more.
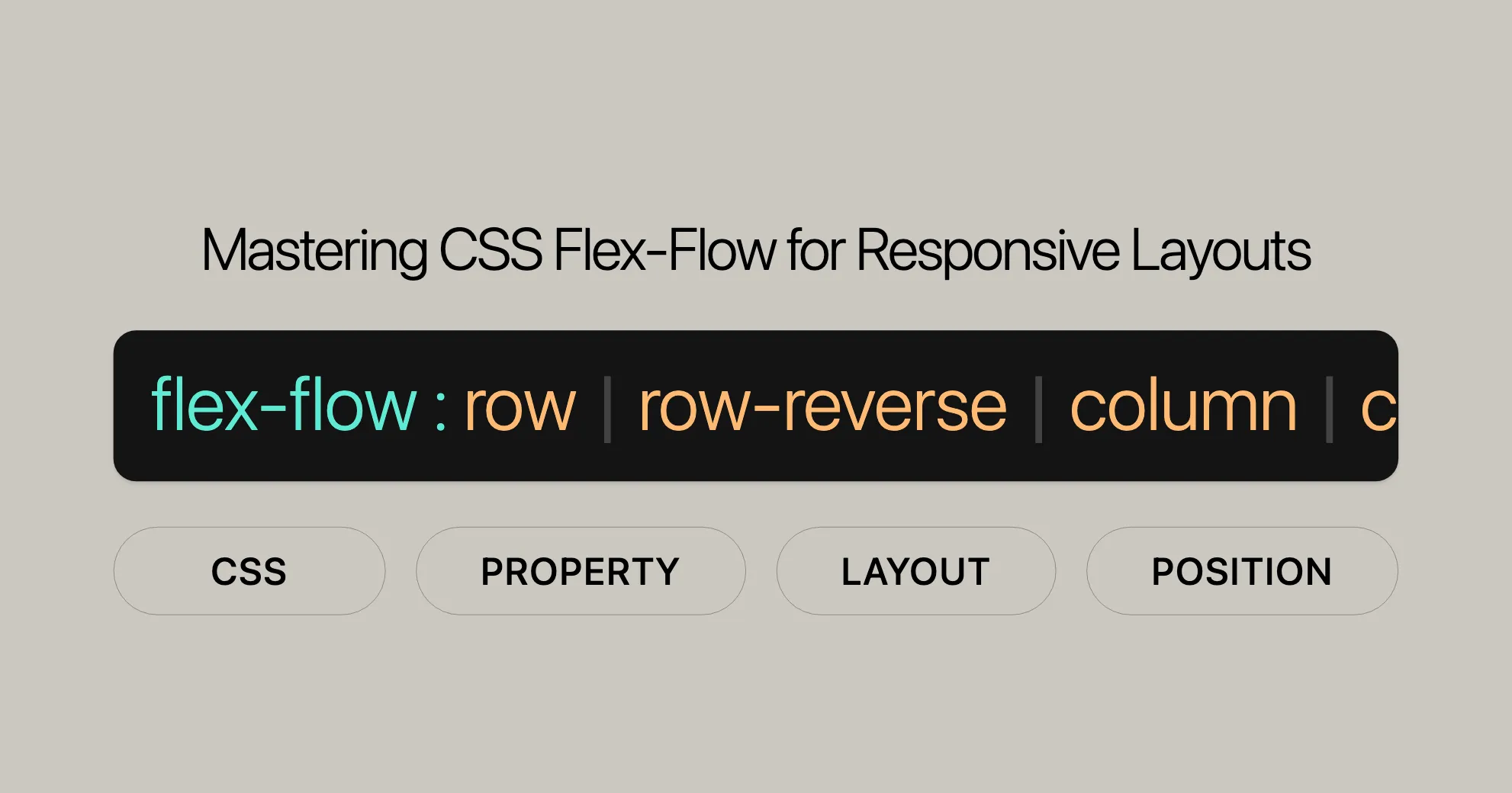
Introduction
The flex-flow
property in CSS is a shorthand that combines flex-direction
and flex-wrap
. It helps control the direction and wrapping of flex items within a flex container. This property has been widely supported across browsers since September 2015.
Using flex-flow
, you can manage the layout of your flex items more easily, whether you want them in rows, columns, or to wrap when they run out of space. Let’s dive into the specifics to help you use it effectively in your projects.
Specification
The flex-flow
property is defined in the CSS Flexible Box Layout Module Level 1 specification. This specification ensures consistency across different browsers and platforms.
The specification for flex-flow
can be found here:
Description
The flex-flow
property in CSS is a shorthand that sets both flex-direction
and flex-wrap
in one go. This makes it easier to control the layout and wrapping behavior of flex items within a flex container.
By combining these properties, flex-flow
allows you to:
- Set the direction of the flex items (
row
,row-reverse
,column
,column-reverse
). - Define the wrapping behavior of the flex items (
nowrap
,wrap
,wrap-reverse
).
Using flex-flow
simplifies your CSS and makes your code more maintainable.
Constituent Properties
The flex-flow
property combines two essential CSS properties for managing flex containers: flex-direction
and flex-wrap
.
flex-direction
row
: Places the flex items in a row, following the text direction.row-reverse
: Places the flex items in a row, but in the opposite direction of the text.column
: Stacks the flex items vertically, from top to bottom.column-reverse
: Stacks the flex items vertically, but from bottom to top.
flex-wrap
nowrap
: Prevents the flex items from wrapping onto multiple lines, keeping them on a single line.wrap
: Allows the flex items to wrap onto multiple lines if necessary.wrap-reverse
: Allows the flex items to wrap onto multiple lines, but in the reverse order.
Syntax
The syntax for flex-flow
is straightforward:
flex-flow: flex-direction flex-wrap;
Example Syntax
/* Setting flex-direction only */flex-flow: row;flex-flow: row-reverse;flex-flow: column;flex-flow: column-reverse;
/* Setting flex-wrap only */flex-flow: nowrap;flex-flow: wrap;flex-flow: wrap-reverse;
/* Setting both flex-direction and flex-wrap */flex-flow: row nowrap;flex-flow: column wrap;flex-flow: column-reverse wrap-reverse;
/* Global values */flex-flow: inherit;flex-flow: initial;flex-flow: revert;flex-flow: revert-layer;flex-flow: unset;
Values
The flex-flow
property accepts values from flex-direction
and flex-wrap
.
flex-direction
Values
row
: Arranges the flex items in a row, following the text direction.row-reverse
: Arranges the flex items in a row, but in the opposite direction of the text.column
: Stacks the flex items vertically, from top to bottom.column-reverse
: Stacks the flex items vertically, but from bottom to top.
flex-wrap
Values
nowrap
: Prevents the flex items from wrapping onto multiple lines.wrap
: Allows the flex items to wrap onto multiple lines if necessary.wrap-reverse
: Allows the flex items to wrap onto multiple lines, but in the reverse order.
Example
Here is an example that demonstrates the use of the flex-flow
property:
element { flex-flow: column-reverse wrap;}
In this example:
column-reverse
: The flex items are arranged from bottom to top.wrap
: The flex items are allowed to wrap, creating new lines if needed.
Browser Compatibility
The flex-flow
property is widely supported across browsers, ensuring compatibility across various devices and browser versions.
Examples
Example 1: Row Direction with No Wrap
In this example, items are arranged in a row and do not wrap onto multiple lines.
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>flex-flow Example</title> <style> #main { width: 400px; height: 300px; border: 2px solid black; display: flex; flex-flow: row nowrap; }
#main div { width: 100px; height: 50px; }
h1, h3 { margin-left: 50px; } </style></head><body> <h1>Website</h1> <h3>The flex-flow: row nowrap</h3> <div id="main"> <div style="background-color:#009900;">1</div> <div style="background-color:#00cc99;">2</div> <div style="background-color:#0066ff;">3</div> <div style="background-color:#66ffff;">4</div> <div style="background-color:#660066;">5</div> <div style="background-color:#663300;">6</div> </div></body></html>
Example 2: Column Direction with Wrap
In this example, items are arranged in a column and can wrap onto multiple lines if needed.
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>flex-flow Example</title> <style> #main { width: 400px; height: 300px; border: 2px solid black; display: flex; flex-flow: column wrap; }
#main div { width: 100px; height: 50px; }
h1, h3 { margin-left: 50px; } </style></head><body> <h1>Website</h1> <h3>The flex-flow: column wrap</h3> <div id="main"> <div style="background-color:#009900;">1</div> <div style="background-color:#00cc99;">2</div> <div style="background-color:#0066ff;">3</div> <div style="background-color:#66ffff;">4</div> <div style="background-color:#660066;">5</div> <div style="background-color:#663300;">6</div> </div></body></html>
Example 3: Row-Reverse Direction with Wrap-Reverse
In this example, items are arranged in a row in reverse direction and can wrap onto multiple lines in reverse order.
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>flex-flow Example</title> <style> #main { width: 400px; height: 300px; border: 2px solid black; display: flex; flex-flow: row-reverse wrap-reverse; }
#main div { width: 100px; height: 50px; }
h1, h3 { margin-left: 50px; } </style></head><body> <h1>Website</h1> <h3>The flex-flow: row-reverse wrap-reverse</h3> <div id="main"> <div style="background-color:#009900;">1</div> <div style="background-color:#00cc99;">2</div> <div style="background-color:#0066ff;">3</div> <div style="background-color:#66ffff;">4</div> <div style="background-color:#660066;">5</div> <div style="background-color:#663300;">6</div> </div></body></html>
Example 4: Column-Reverse Direction with No Wrap
In this example, items are arranged in a column in reverse direction and do not wrap onto multiple lines.
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>flex-flow Example</title> <style> #main { width: 400px; height: 300px; border: 2px solid black; display: flex; flex-flow: column-reverse nowrap; }
#main div { width: 100px; height: 50px; }
h1, h3 { margin-left: 50px; } </style></head><body> <h1>Website</h1> <h3>The flex-flow: column-reverse nowrap</h3> <div id="main"> <div style="background-color:#009900;">1</div> <div style="background-color:#00cc99;">2</div> <div style="background-color:#0066ff;">3</div> <div style="background-color:#66ffff;">4</div> <div style="background-color:#660066;">5</div> <div style="background-color:#663300;">6</div> </div></body></html>
Example 5: Row Direction with Wrap-Reverse
In this example, the flex items are arranged in a row, and they wrap onto multiple lines in the reverse order if necessary.
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>flex-flow Example</title> <style> #main { width: 400px; height: 300px; border: 2px solid black; display: flex; flex-flow: row wrap-reverse; }
#main div { width: 100px; height: 50px; }
h1 { color: #009900; font-size: 42px; margin-left: 50px; }
h3 { margin-top: -20px; margin-left: 50px; } </style></head><body> <h1>Website</h1> <h3>The flex-flow: row wrap-reverse</h3> <div id="main"> <div style="background-color:#009900;">1</div> <div style="background-color:#00cc99;">2</div> <div style="background-color:#0066ff;">3</div> <div style="background-color:#66ffff;">4</div> <div style="background-color:#660066;">5</div> <div style="background-color:#663300;">6</div> </div></body></html>
Example 6: Column Direction with No Wrap
In this example, the flex items are arranged in a column and do not wrap onto multiple lines.
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>flex-flow Example</title> <style> #main { width: 400px; height: 300px; border: 2px solid black; display: flex; flex-flow: column nowrap; }
#main div { width: 100px; height: 50px; }
h1 { color: #009900; font-size: 42px; margin-left: 50px; }
h3 { margin-top: -20px; margin-left: 50px; } </style></head><body> <h1>Website</h1> <h3>The flex-flow: column nowrap</h3> <div id="main"> <div style="background-color:#009900;">1</div> <div style="background-color:#00cc99;">2</div> <div style="background-color:#0066ff;">3</div> <div style="background-color:#66ffff;">4</div> <div style="background-color:#660066;">5</div> <div style="background-color:#663300;">6</div> </div></body></html>
Example 7: Row-Reverse Direction with Wrap
In this example, the flex items are arranged in a row in the reverse direction and wrap onto multiple lines if necessary.
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>flex-flow Example</title> <style> #main { width: 400px; height: 300px; border: 2px solid black; display: flex; flex-flow: row-reverse wrap; }
#main div { width: 100px; height: 50px; }
h1 { color: #009900; font-size: 42px; margin-left: 50px; }
h3 { margin-top: -20px; margin-left: 50px; } </style></head><body> <h1>Website</h1> <h3>The flex-flow: row-reverse wrap</h3> <div id="main"> <div style="background-color:#009900;">1</div> <div style="background-color:#00cc99;">2</div> <div style="background-color:#0066ff;">3</div> <div style="background-color:#66ffff;">4</div> <div style="background-color:#660066;">5</div> <div style="background-color:#663300;">6</div> </div></body></html>
Example 8: Column-Reverse Direction with Wrap
In this example, the flex items are arranged in a column in the reverse direction and wrap onto multiple lines if necessary.
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>flex-flow Example</title> <style> #main { width: 400px; height: 300px; border: 2px solid black; display: flex; flex-flow: column-reverse wrap; }
#main div { width: 100px; height: 50px; }
h1 { color: #009900; font-size: 42px; margin-left: 50px; }
h3 { margin-top: -20px; margin-left: 50px; } </style></head><body> <h1>Website</h1> <h3>The flex-flow: column-reverse wrap</h3> <div id="main"> <div style="background-color:#009900;">1</div> <div style="background-color:#00cc99;">2</div> <div style="background-color:#0066ff;">3</div> <div style="background-color:#66ffff;">4</div> <div style="background-color:#660066;">5</div> <div style="background-color:#663300;">6</div> </div></body></html>
Browser Compatibility
The flex-flow
property is widely supported across modern web browsers:
- Google Chrome: Since version 29.0 (August 2013)
- Mozilla Firefox: Since version 28.0 (March 2014)
- Internet Explorer: Since version 11.0 (October 2013)
- Safari: Since version 9.0 (September 2015)
- Opera: Since version 17.0 (August 2013)
For mobile browsers:
- Android Browser: Since version 4.4 (October 2013)
- iOS Safari: Since version 9.0 (September 2015)
- Opera Mobile: Since version 12.0 (April 2013)
- Chrome for Android: Since version 29.0 (August 2013)
- Firefox for Android: Since version 28.0 (March 2014)
For the most accurate and up-to-date information on browser compatibility, you can refer to the MDN Web Docs Browser Compatibility Data.
Frequently Asked Questions
What is the flex-flow
property?
The flex-flow
property combines flex-direction
and flex-wrap
into one declaration, making it easier to manage flex container layouts.
How do I use flex-flow
to set a row direction with wrapping?
flex-flow: row wrap;
This arranges items in a row and allows them to wrap onto new lines if needed.
What values can flex-flow
accept?
flex-flow
accepts values from flex-direction
(row
, row-reverse
, column
, column-reverse
) and flex-wrap
(nowrap
, wrap
, wrap-reverse
).
What is the default value of flex-flow
?
The default value is row nowrap
.
Can I set only one value with flex-flow
?
Yes, if you set only one value, the other property takes its default value. For example:
flex-flow: column;
This sets flex-direction
to column
and keeps flex-wrap
as nowrap
.
How does flex-flow
affect the layout of flex items?
flex-flow
controls the direction and wrapping behavior of flex items. The flex-direction
part specifies row or column layout, and the flex-wrap
part determines if items wrap onto multiple lines.
Is flex-flow
widely supported across browsers?
Yes, flex-flow
is widely supported in modern browsers.
How can I use flex-flow
to create a responsive layout?
Combine different flex-direction
and flex-wrap
values to create layouts that adapt to different screen sizes.
What is the difference between flex-flow
and using flex-direction
and flex-wrap
separately?
Using flex-flow
is more concise and combines both properties into one declaration, reducing the amount of code.
Can flex-flow
be used with other flexbox properties?
Yes, flex-flow
can be used with properties like justify-content
, align-items
, and flex-grow
to create complex layouts.
By leveraging the flex-flow
property, you can create flexible and dynamic layouts that work seamlessly across various devices and browsers.
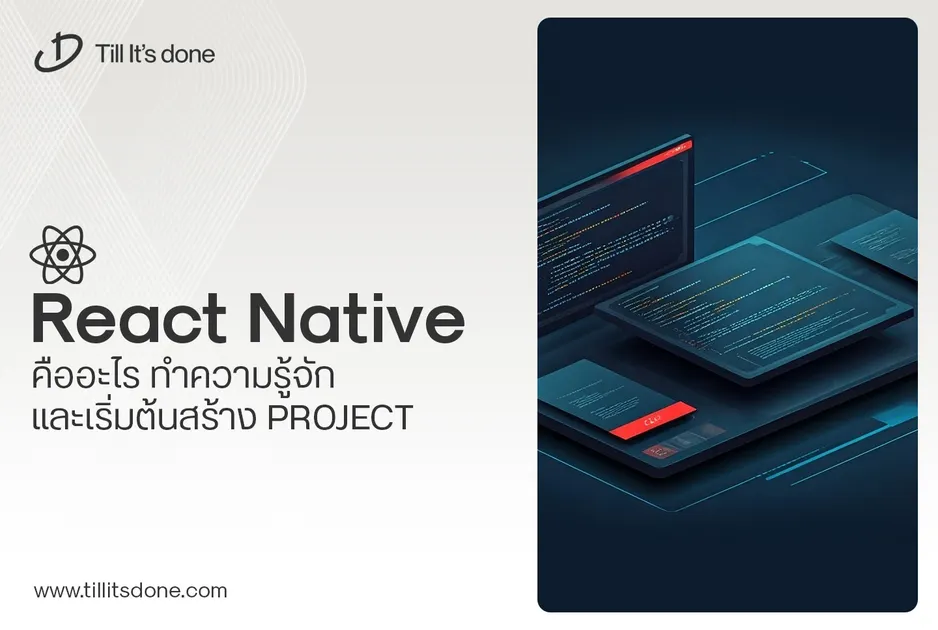
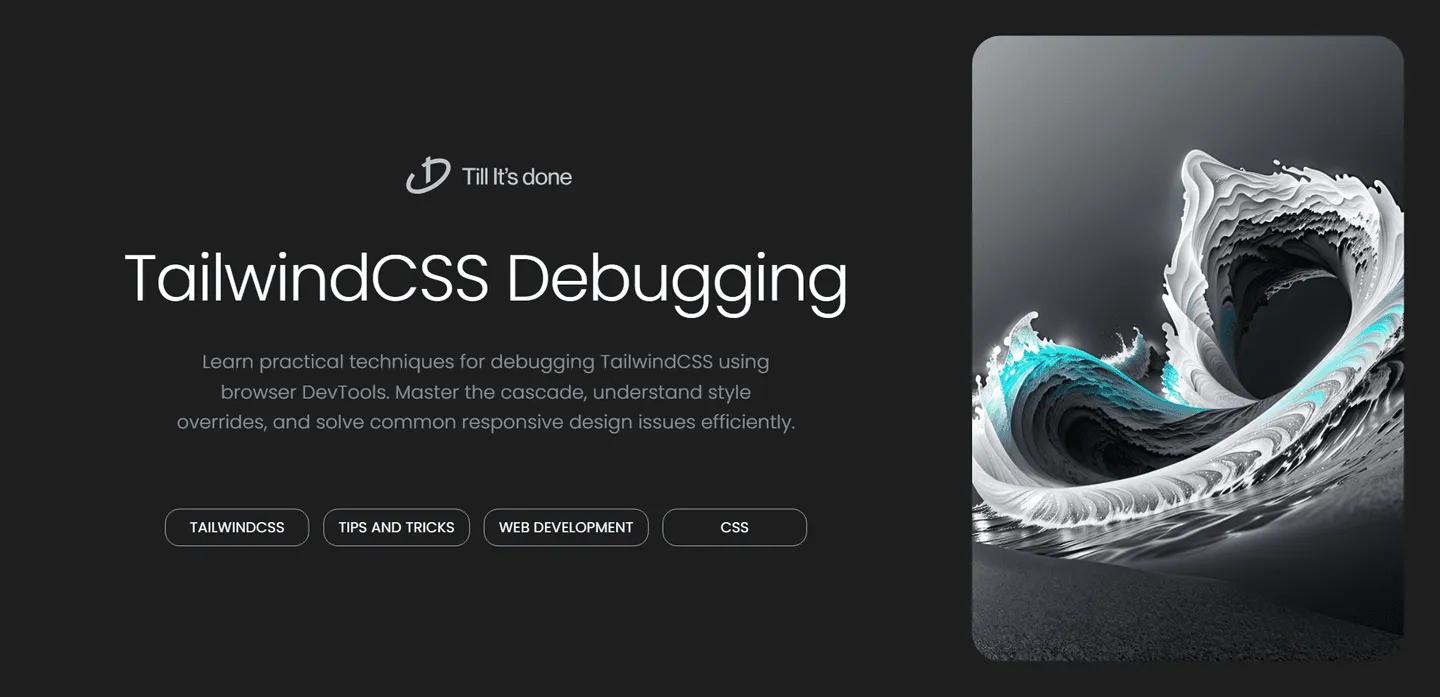
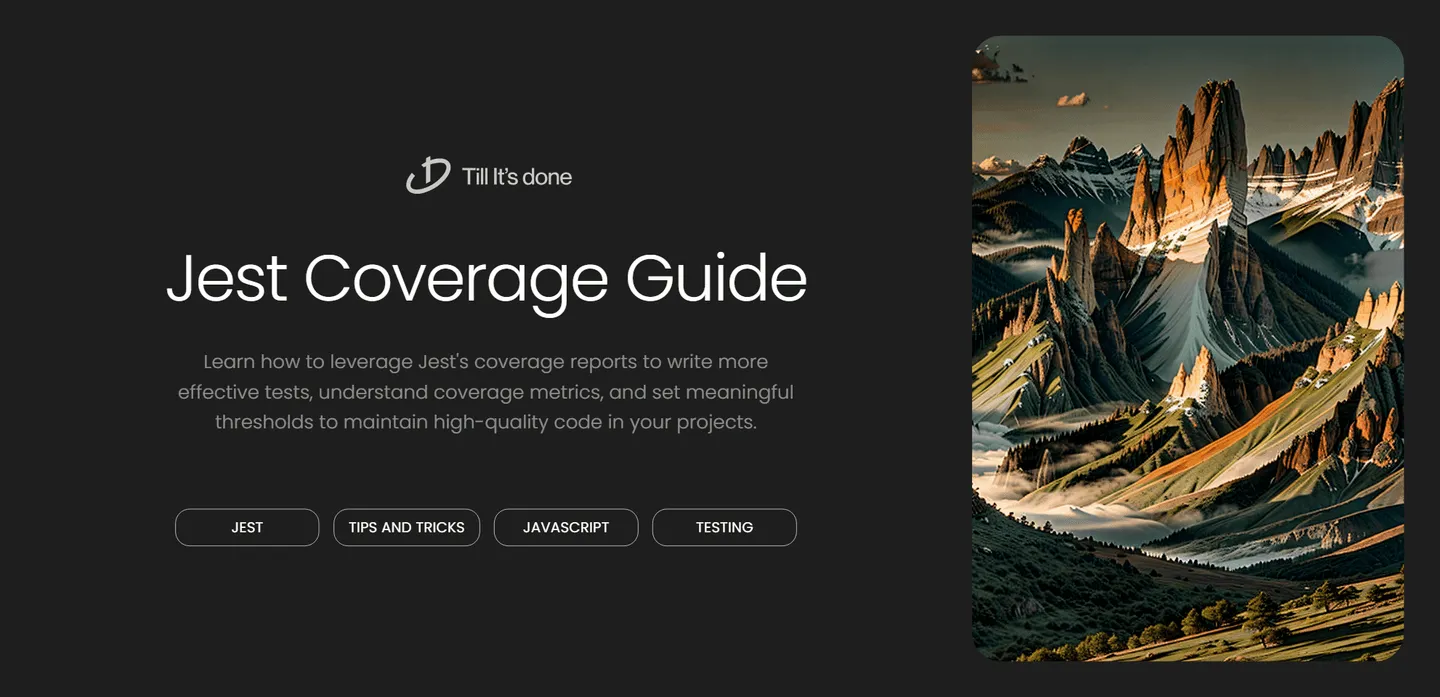
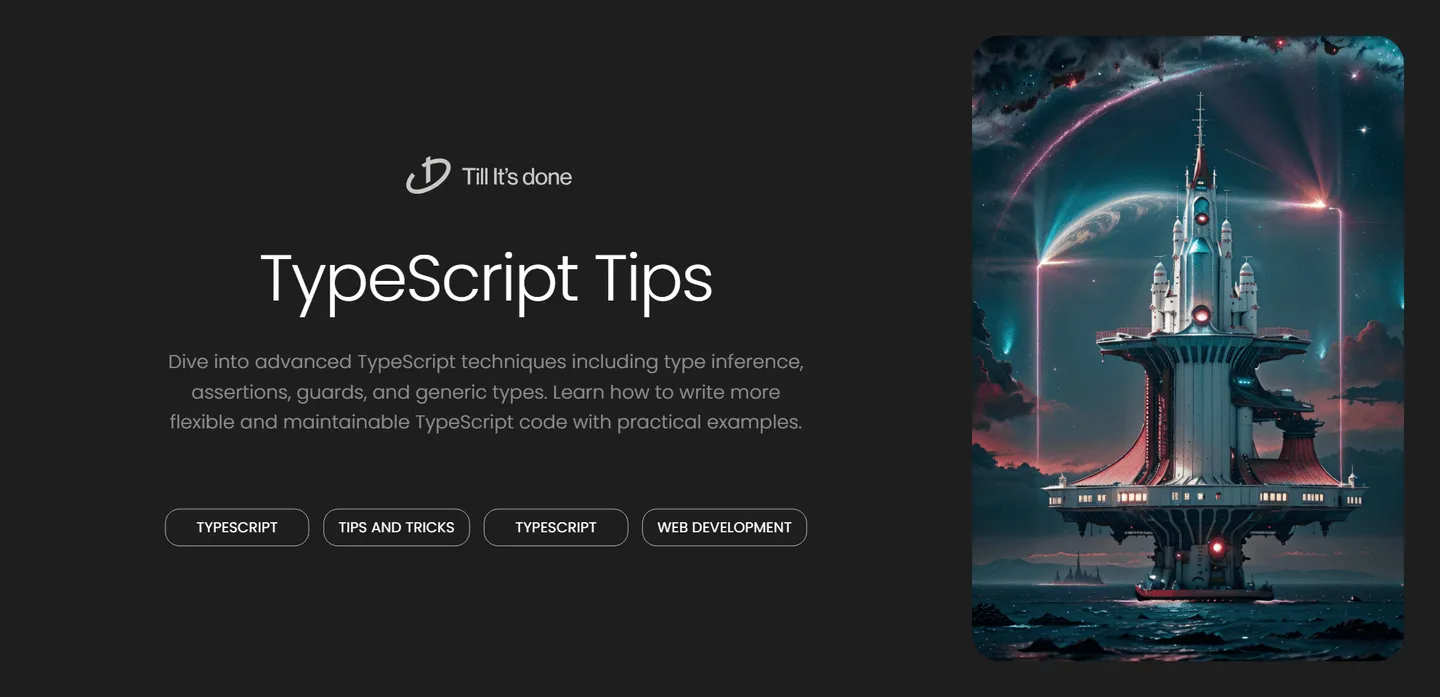
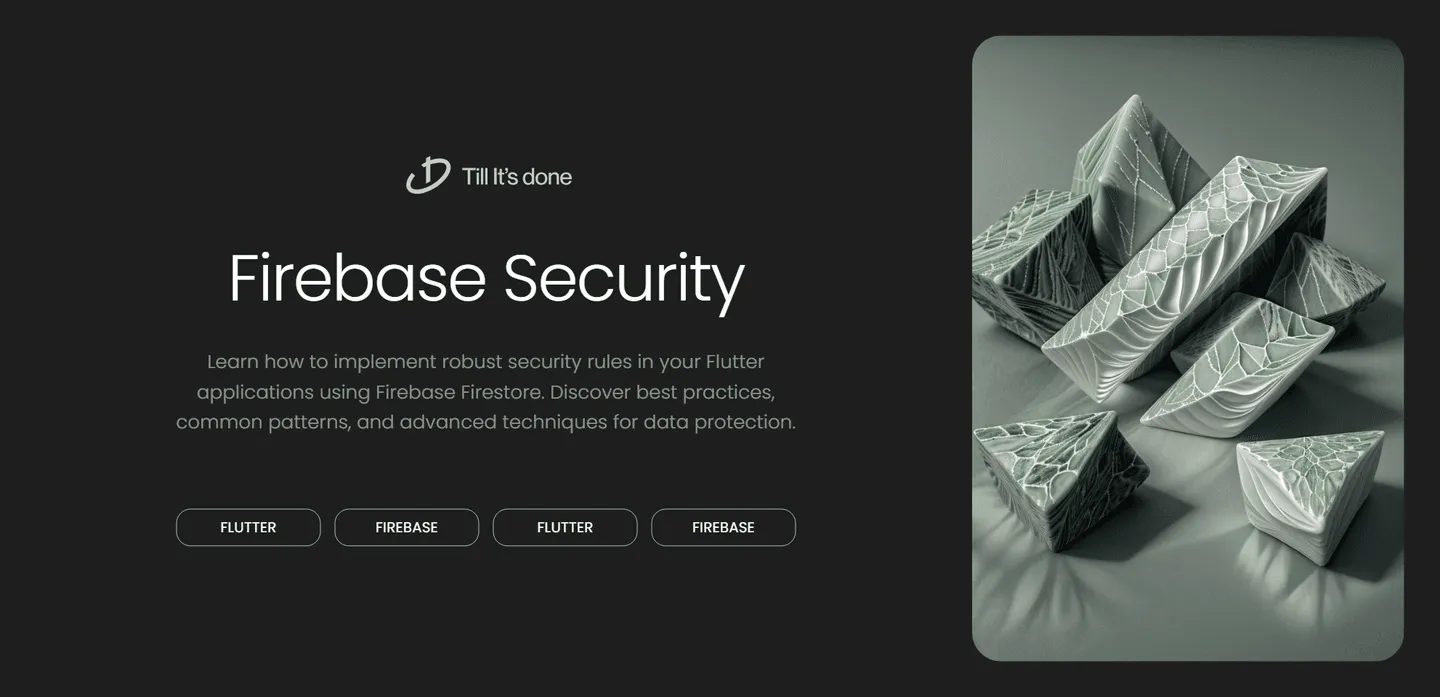
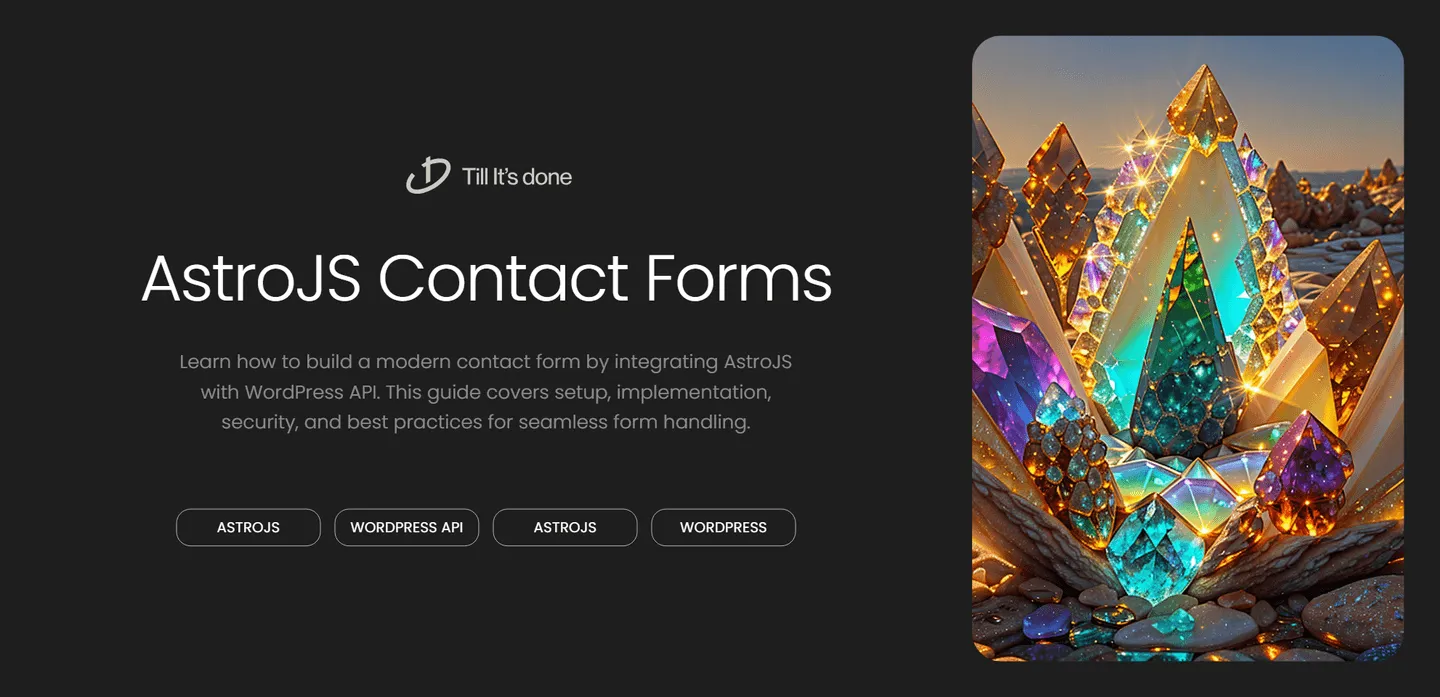
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.