- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Creating Reusable Components in Next.js
Discover best practices, implementation patterns, and testing strategies for component-based development.
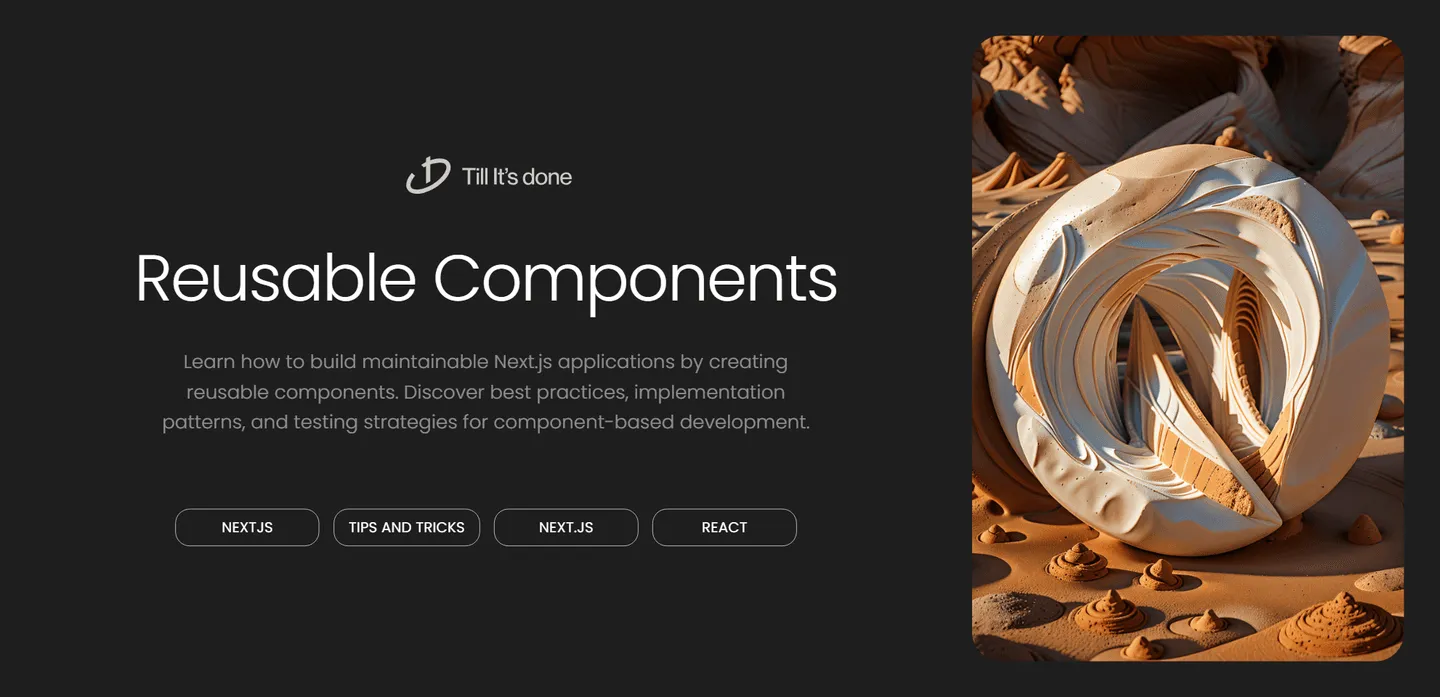
Creating Reusable Components in Next.js: A Practical Guide
Ever found yourself copying and pasting the same code across different pages in your Next.js project? Let’s dive into how you can write cleaner, more maintainable code by creating reusable components that will save you time and reduce redundancy in your projects.
Why Reusable Components Matter
Think of reusable components as your favorite LEGO blocks - they’re standardized pieces that you can mix and match to build something amazing. By creating components that can be used across your application, you’re not just saving time; you’re also ensuring consistency and making your codebase easier to maintain.
Best Practices for Component Creation
1. Keep Props Simple and Specific
Instead of creating components that accept every possible prop under the sun, focus on making them specific and purposeful. Here’s a practical example:
// Good: Focused and specificconst Button = ({ label, onClick, variant = 'primary' }) => { return ( <button onClick={onClick} className={`btn btn-${variant}`} > {label} </button> );};
// Too complex: Trying to handle too many casesconst Button = ({ label, onClick, variant, size, icon, disabled, loading, custom, ...props }) => { // This becomes harder to maintain};
2. Implement Component Composition
Break down complex components into smaller, more manageable pieces. This makes your code more modular and easier to test:
const Card = ({ children, title }) => { return ( <div className="card"> <CardHeader title={title} /> <CardContent>{children}</CardContent> </div> );};
const CardHeader = ({ title }) => <h2 className="card-title">{title}</h2>;const CardContent = ({ children }) => <div className="card-content">{children}</div>;
3. Use TypeScript for Better Component Interfaces
TypeScript adds an extra layer of safety to your components. Define clear interfaces for your props:
interface ButtonProps { label: string; onClick: () => void; variant?: 'primary' | 'secondary' | 'danger';}
const Button: React.FC<ButtonProps> = ({ label, onClick, variant = 'primary' }) => { // Component implementation};
Advanced Component Patterns
Higher-Order Components (HOCs)
Use HOCs to add functionality to existing components:
const withLoading = (WrappedComponent) => { return function WithLoadingComponent({ isLoading, ...props }) { if (isLoading) return <div>Loading...</div>; return <WrappedComponent {...props} />; };};
const MyComponent = ({ data }) => <div>{data}</div>;const MyComponentWithLoading = withLoading(MyComponent);
Custom Hooks for Reusable Logic
Extract common logic into custom hooks:
const useWindowSize = () => { const [windowSize, setWindowSize] = useState({ width: undefined, height: undefined, });
useEffect(() => { const handleResize = () => { setWindowSize({ width: window.innerWidth, height: window.innerHeight, }); };
handleResize(); window.addEventListener('resize', handleResize); return () => window.removeEventListener('resize', handleResize); }, []);
return windowSize;};
Testing Your Components
Remember to write tests for your reusable components. Use React Testing Library to write tests that mirror how your components are used:
import { render, fireEvent } from '@testing-library/react';
test('Button triggers onClick when clicked', () => { const handleClick = jest.fn(); const { getByText } = render( <Button label="Click me" onClick={handleClick} /> );
fireEvent.click(getByText('Click me')); expect(handleClick).toHaveBeenCalled();});
Conclusion
Creating reusable components is an art that combines good architecture, careful planning, and attention to user needs. By following these practices, you’ll create a component library that’s not only reusable but also maintainable and scalable.
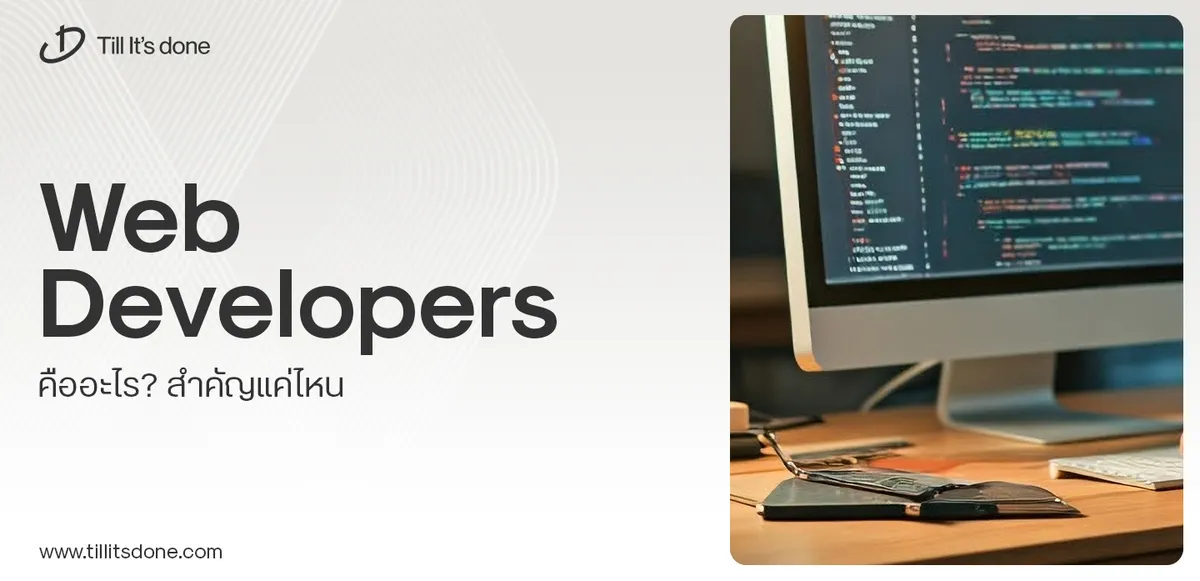
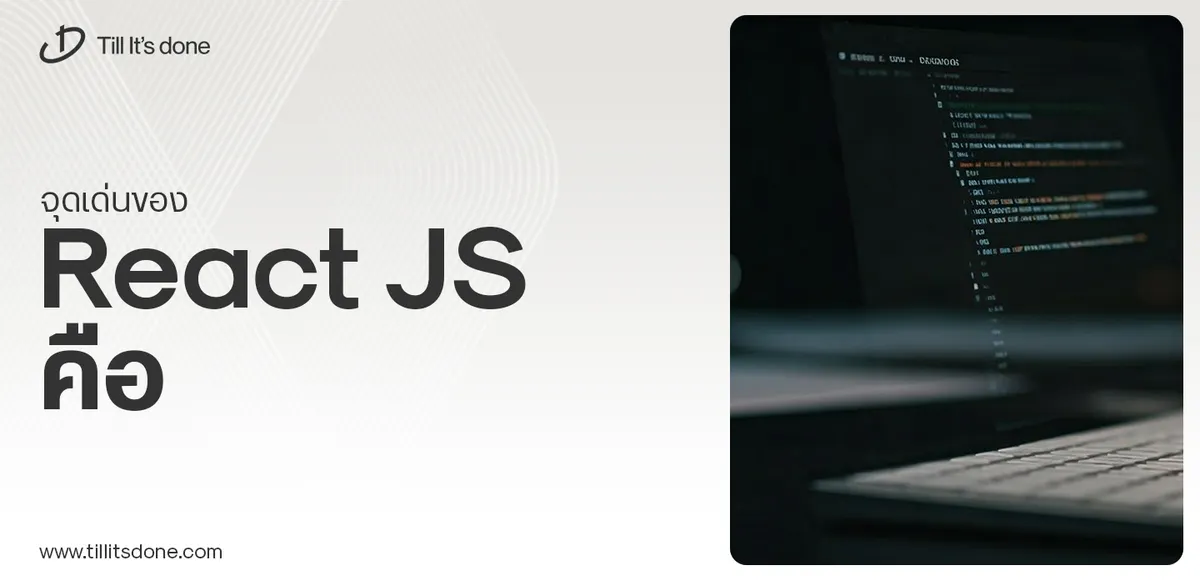
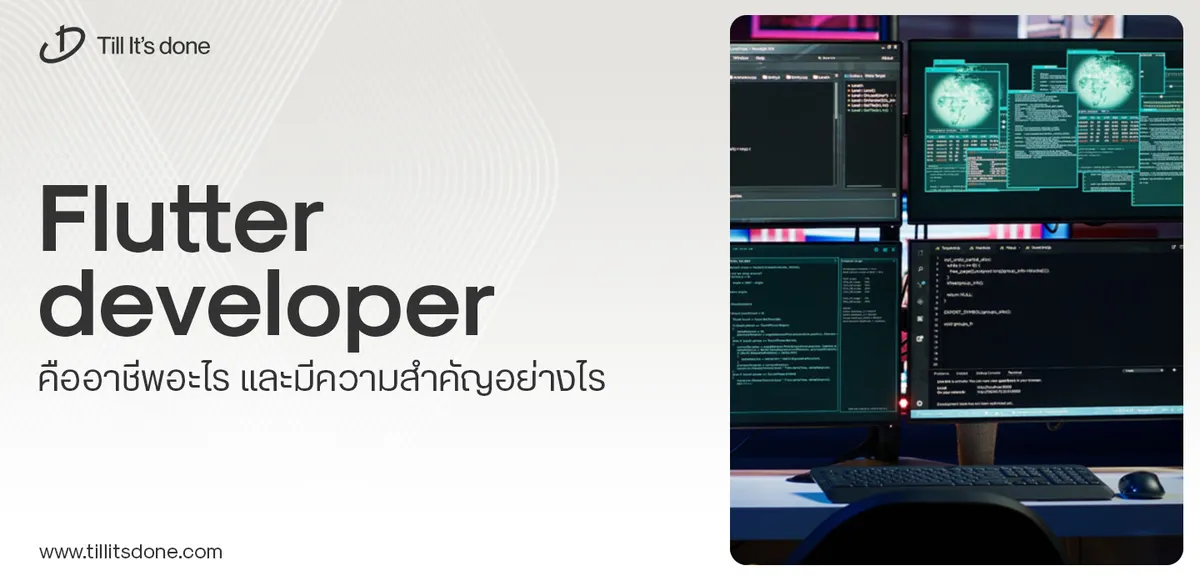
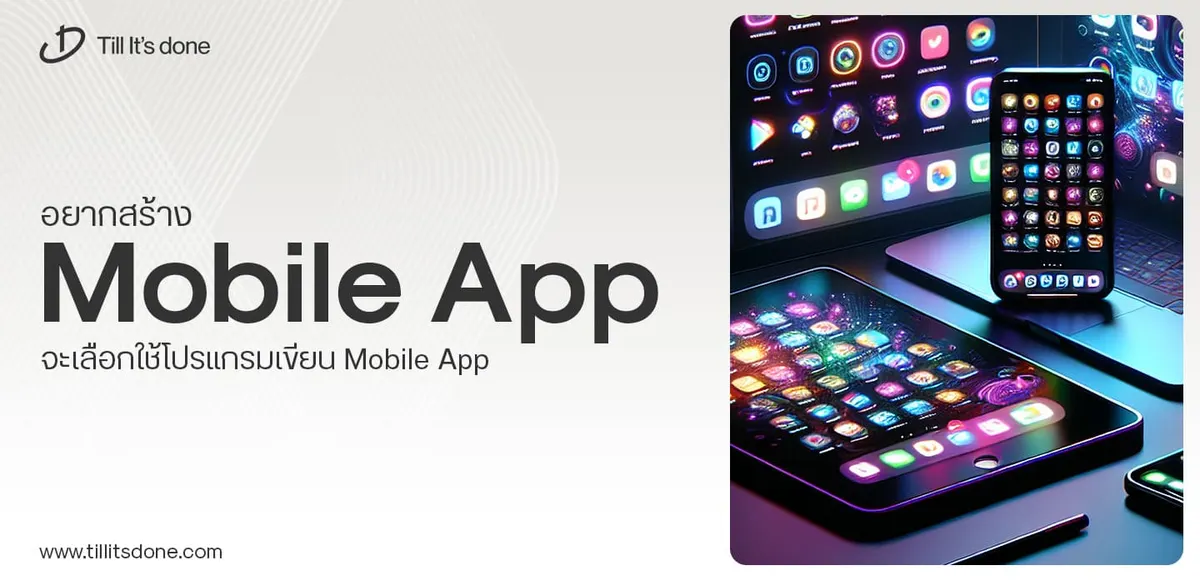
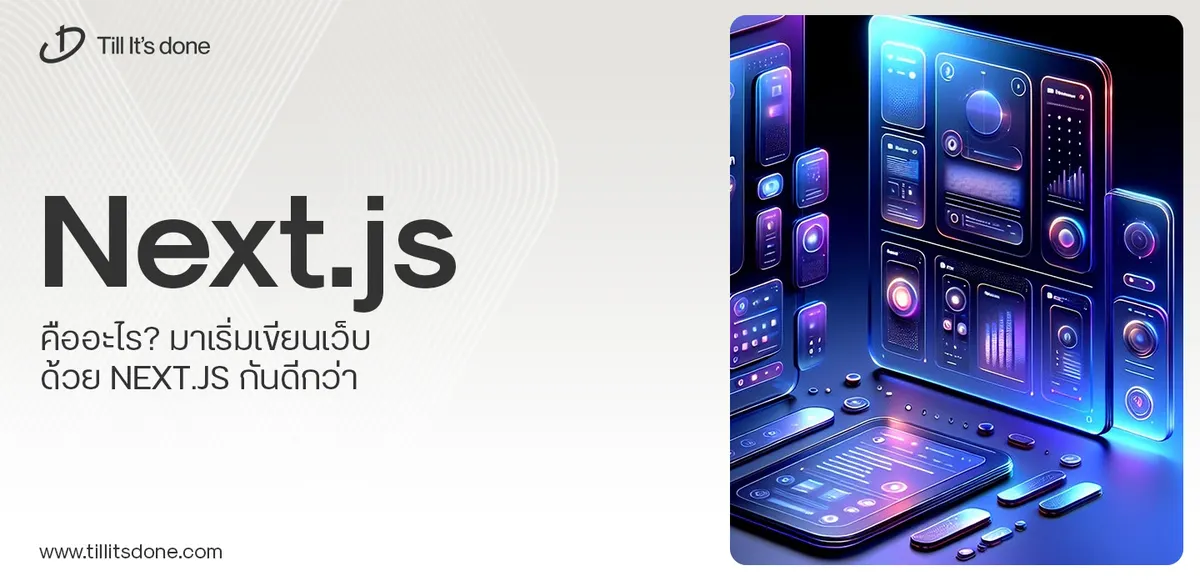
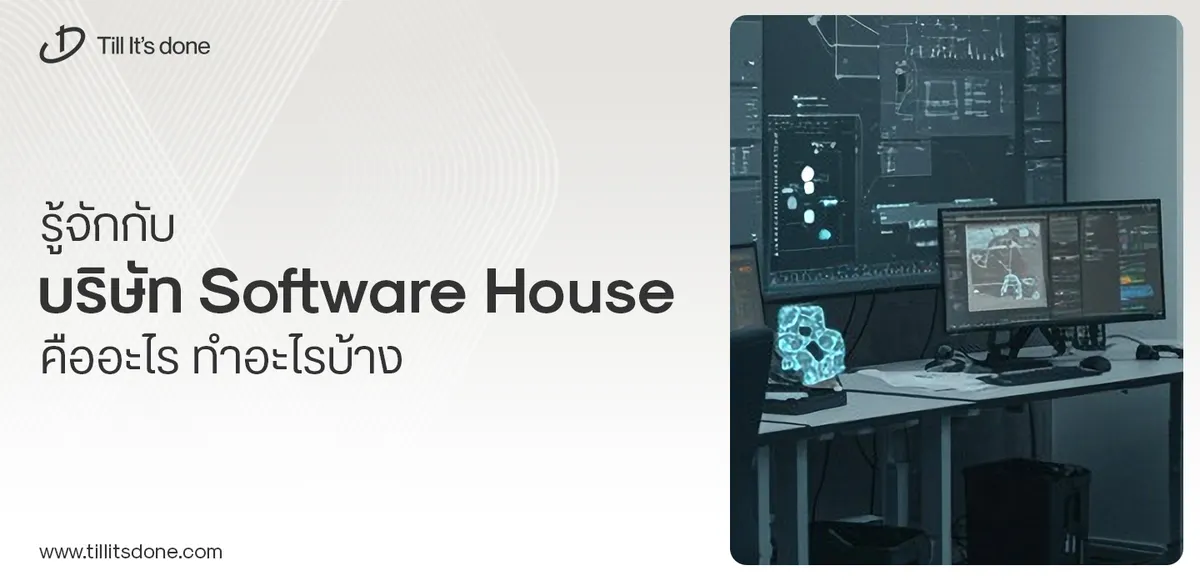
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.