- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Conditional Rendering in ReactJS: Dynamic UIs
Learn to create dynamic user interfaces using if statements, ternary operators, logical operators, and switch cases.

Conditional Rendering in ReactJS: Making Your UI Dynamic and Responsive
Have you ever wondered how Facebook shows different content to logged-in and logged-out users? Or how Gmail displays different interfaces based on whether you have new emails or not? That’s conditional rendering in action, and today we’re diving deep into this fundamental React concept.
What is Conditional Rendering?
Think of conditional rendering as a traffic controller for your UI elements. Just like a traffic light decides which cars can pass through, conditional rendering determines which components or elements should appear on your screen based on certain conditions.
The Basic Building Blocks
Let’s start with the simplest form of conditional rendering - the if statement. Imagine you’re building a notification system:
function NotificationBadge({ messages }) { if (messages.length === 0) { return null; } return <div className="badge">{messages.length}</div>;}
The Mighty Ternary Operator
While if statements are great, sometimes we want something more concise. Enter the ternary operator - the Swiss Army knife of conditional rendering:
function Welcome({ isLoggedIn }) { return ( <div> {isLoggedIn ? <h1>Welcome back!</h1> : <h1>Please log in</h1> } </div> );}
The Logical && Operator: Your Short-Circuit Friend
When you just need to show or hide something, the && operator becomes your best friend:
function TodoList({ todos, isLoading }) { return ( <div> {isLoading && <Spinner />} {todos.map(todo => <TodoItem key={todo.id} {...todo} />)} </div> );}
Switch Cases: When You Need Multiple Conditions
Sometimes life isn’t just true or false. When you need to handle multiple conditions, switch cases come in handy:
function PaymentStatus({ status }) { switch(status) { case 'pending': return <PendingPayment />; case 'completed': return <Success />; case 'failed': return <Error />; default: return <ProcessingPayment />; }}
Best Practices and Tips
- Keep it simple - if your conditional logic gets too complex, consider breaking it into smaller components
- Use early returns for cleaner code
- Consider extracting complex conditions into readable variables
- Remember that all your conditions should handle loading and error states
Remember, conditional rendering is not just about showing and hiding elements - it’s about creating intuitive, responsive interfaces that enhance user experience. Master these patterns, and you’ll be well on your way to building more dynamic and engaging React applications.
Whether you’re building a simple toggle button or a complex user dashboard, these conditional rendering techniques will help you create more elegant and maintainable React applications. Happy coding!
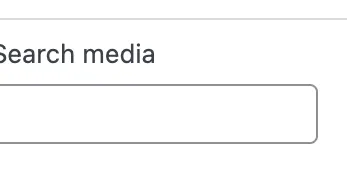





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.