- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Handle Complex UI States with BLoC in Flutter
Discover best practices, implementation strategies, and advanced patterns for building scalable Flutter applications.
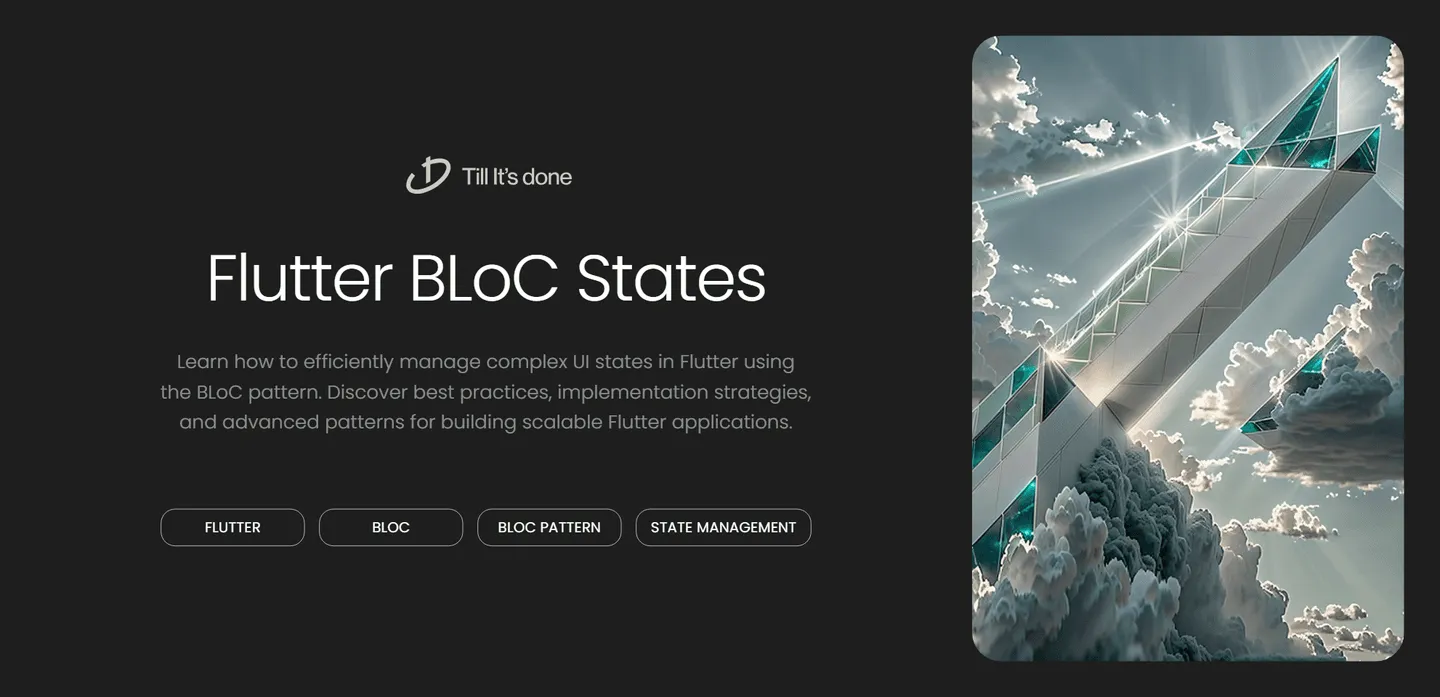
Handling Complex UI States with BLoC in Flutter
Managing state in complex Flutter applications can be challenging, especially when dealing with multiple data streams, user interactions, and dynamic UI updates. That’s where BLoC (Business Logic Component) pattern comes in – a powerful state management solution that helps us create maintainable and scalable applications.
Understanding the BLoC Pattern
Think of BLoC as a conductor in an orchestra, coordinating different instruments (states) to create a harmonious symphony (your app). It separates business logic from UI components, making your code cleaner and easier to test.
Implementing Complex UI States
Let’s dive into a real-world scenario. Imagine you’re building a social media feed that needs to handle multiple states: loading, error, empty content, and loaded content with pagination. Here’s how you can structure your states:
sealed class FeedState { const FeedState();}
class FeedInitial extends FeedState {}class FeedLoading extends FeedState {}class FeedLoaded extends FeedState { final List<Post> posts; final bool hasReachedMax;
const FeedLoaded({ required this.posts, this.hasReachedMax = false, });}class FeedError extends FeedState { final String message;
const FeedError(this.message);}
The beauty of BLoC lies in its ability to handle these states seamlessly. Your UI remains responsive while the BLoC manages all the complex state transitions behind the scenes.
Best Practices for Complex State Management
- Keep your BLoCs focused and single-responsibility driven
- Use sealed classes for state management to ensure type safety
- Implement proper error handling and loading states
- Consider using freezed for immutable state objects
- Leverage BLoC testing for robust state management
Remember to dispose of your BLoCs properly to prevent memory leaks:
@overrideFuture<void> close() { _streamSubscription?.cancel(); return super.close();}
By following these patterns, you can handle even the most complex UI states with grace and maintainability.
Advanced BLoC Patterns
For more complex scenarios, consider implementing state transformers and debounce operators. These can help you handle rapid UI updates and optimize performance:
Stream<FeedState> _transformStates( Stream<FeedEvent> events, Stream<FeedState> Function(FeedEvent) mapper,) { return events .debounceTime(const Duration(milliseconds: 300)) .switchMap(mapper);}
The BLoC pattern might seem complex at first, but it’s this complexity that gives us the power to handle sophisticated UI states with elegance. By separating concerns and maintaining a clear flow of data, we create applications that are not only powerful but also maintainable and scalable.
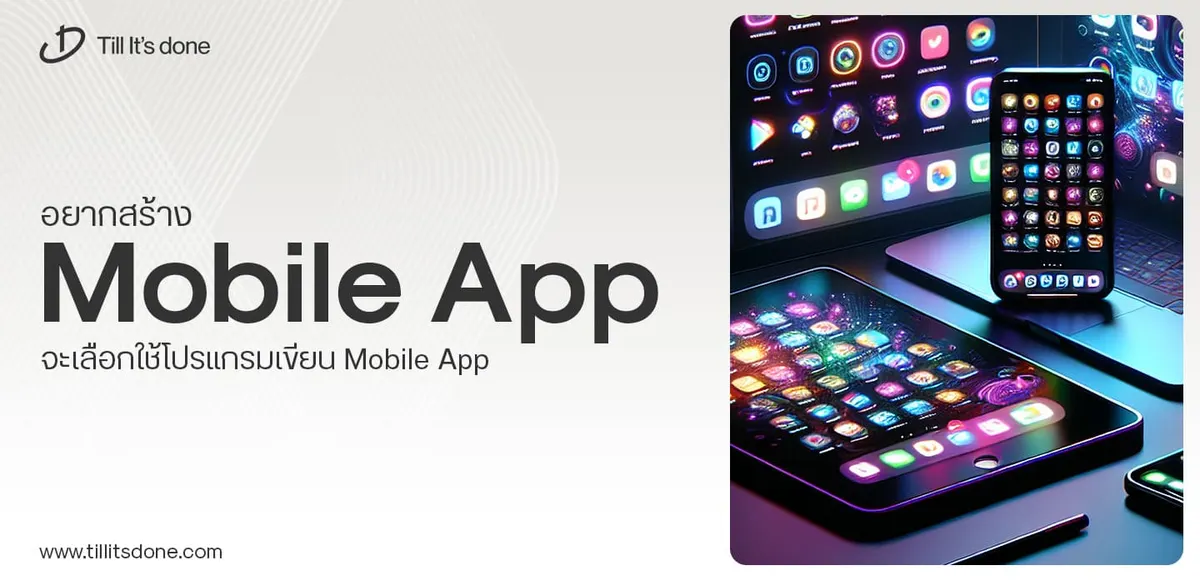
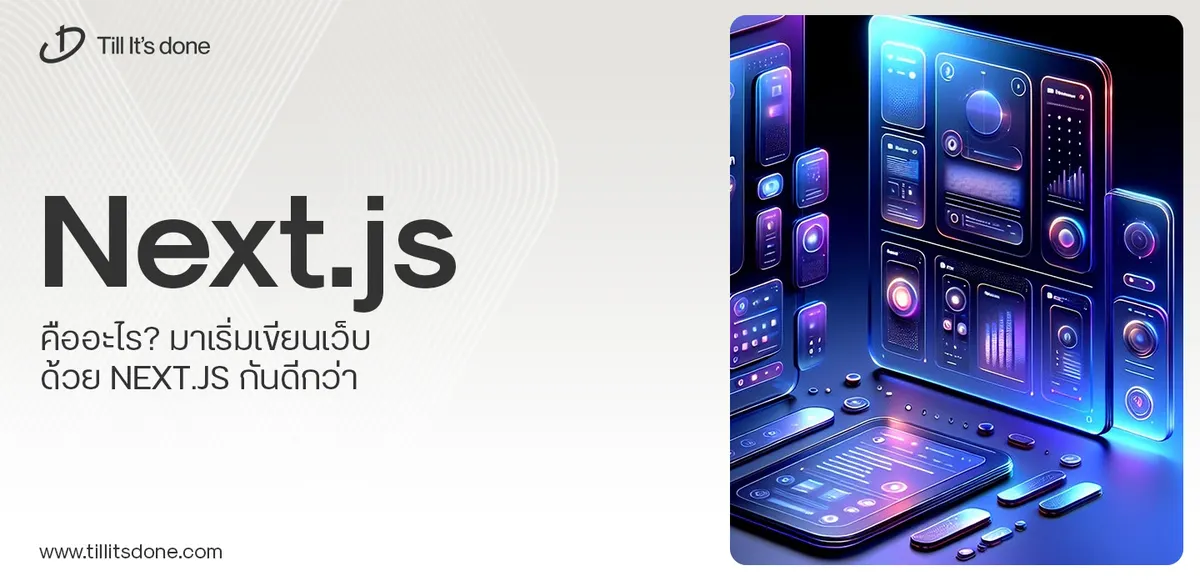
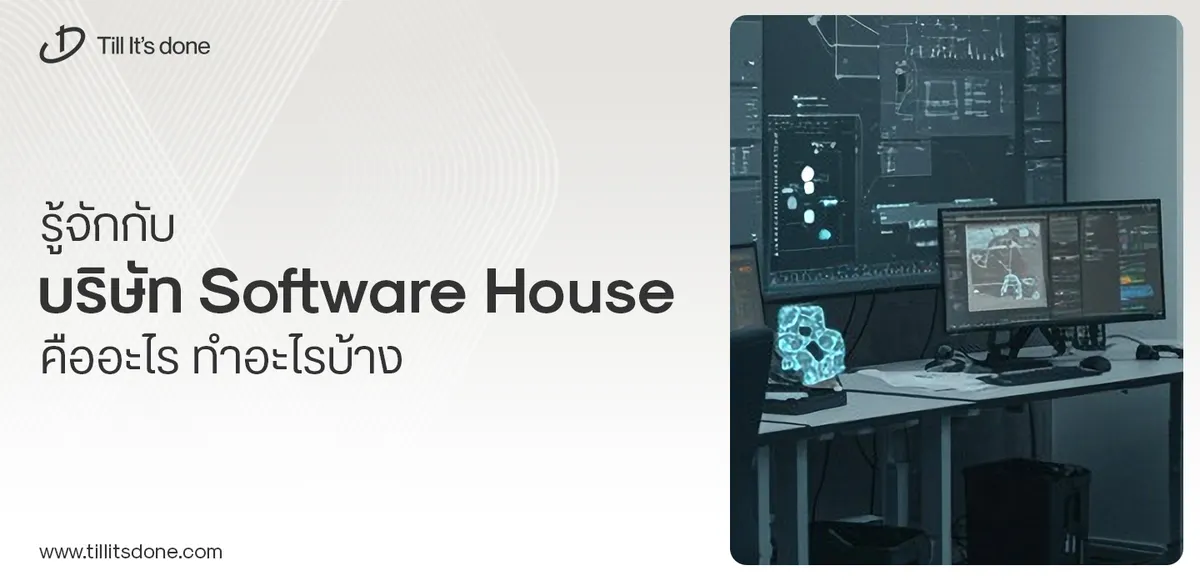
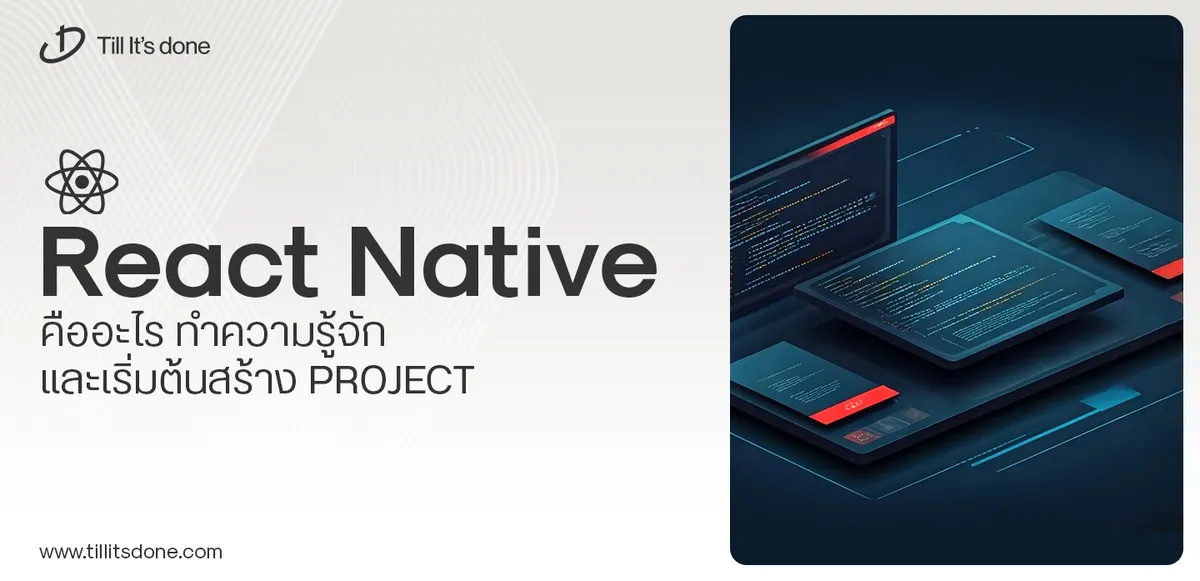
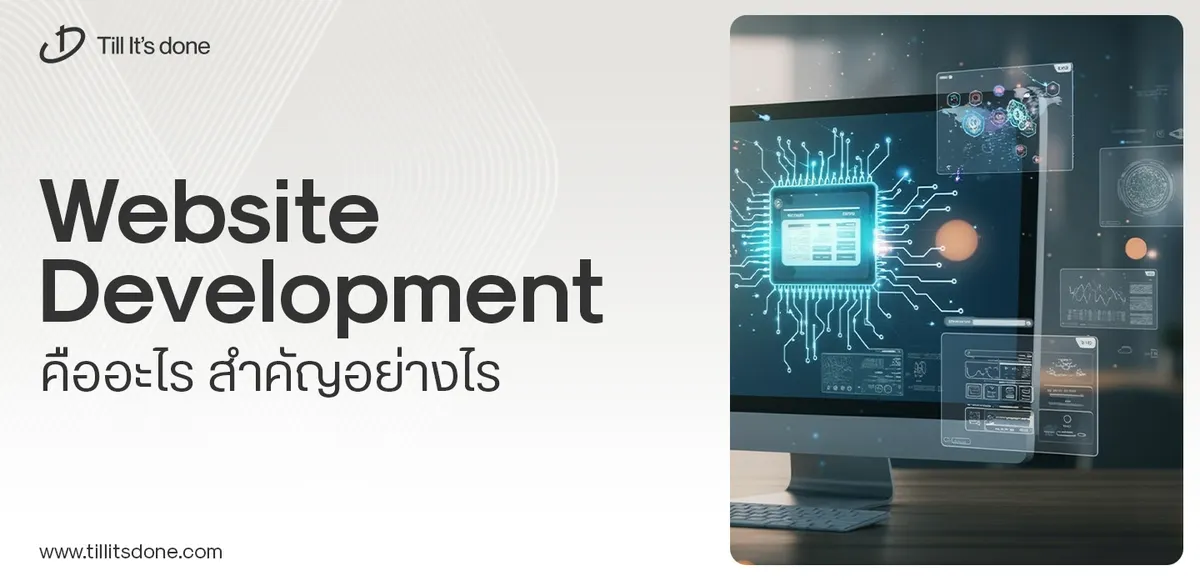
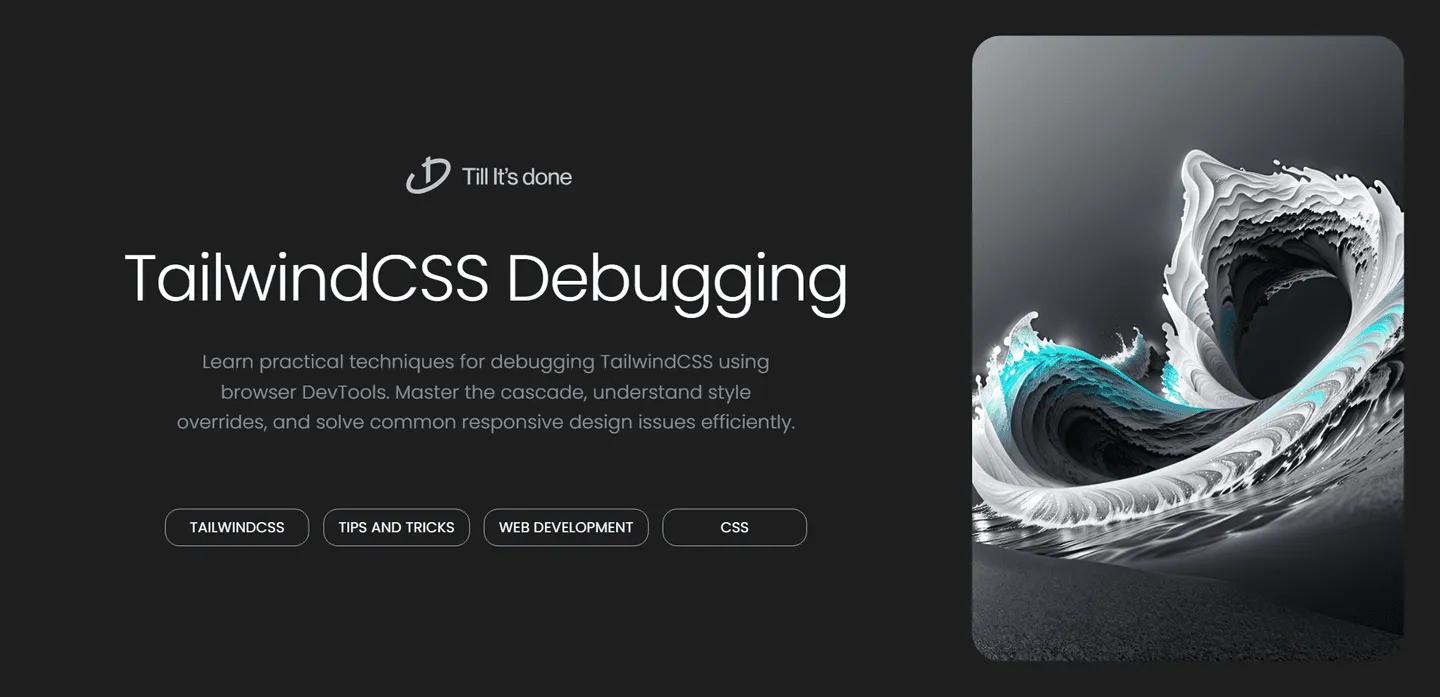
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.