- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Building Complex Conditional Types in TypeScript
Learn how to create sophisticated type-safe abstractions, from basic patterns to recursive types and practical applications.
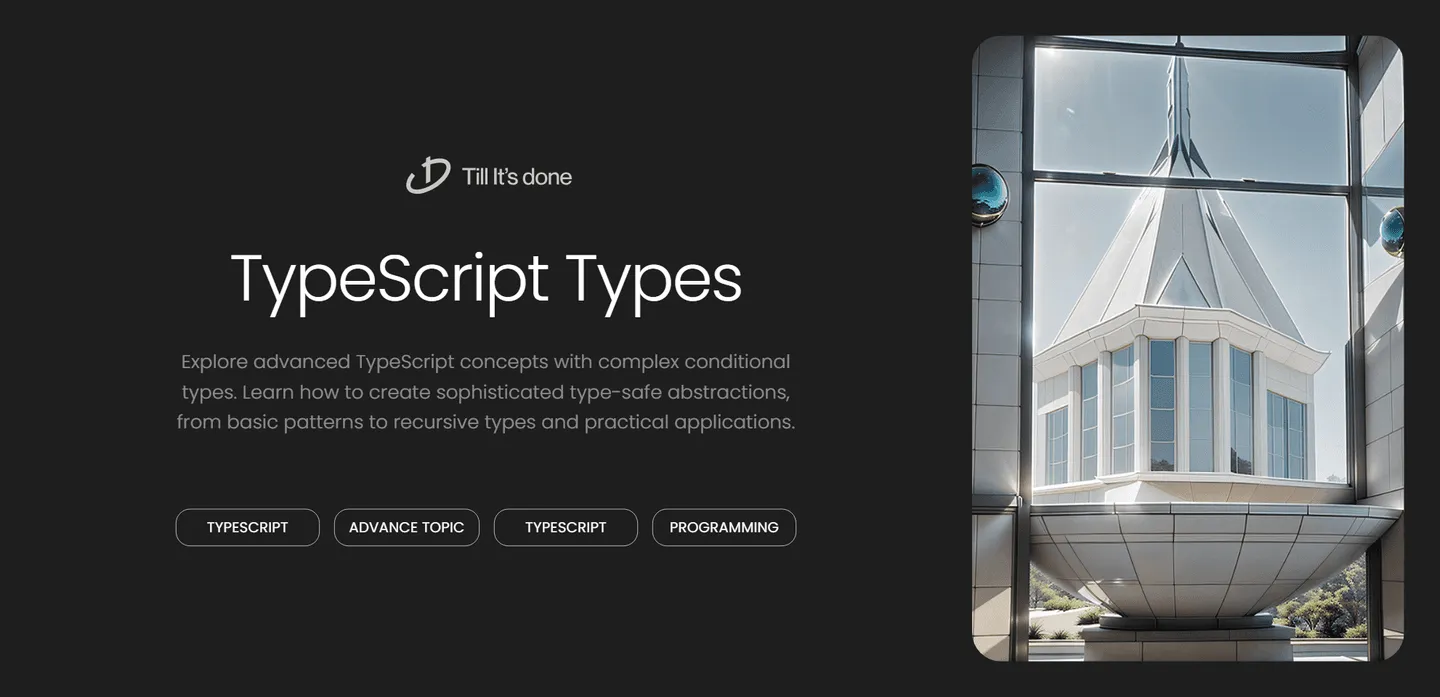
Building Complex Conditional Types in TypeScript
TypeScript’s type system is incredibly powerful, and one of its most fascinating features is conditional types. Today, let’s dive deep into building complex conditional types that can make your code more type-safe and expressive.
Understanding the Basics
Think of conditional types as “if statements” for your types. Just like how we write logic in our code, we can write logic in our type system. The basic syntax looks like this:
type CheckNumber\<T\> = T extends number ? "Is Number" : "Not Number";
Going Beyond Simple Conditionals
Let’s explore some real-world scenarios where complex conditional types shine.
Pattern Matching with Multiple Conditions
One powerful pattern is creating types that can match multiple conditions. Here’s how we can build a type that handles different data structures:
type DataStructureType\<T\> = T extends Array<any> ? "array" : T extends Map<any, any> ? "map" : T extends Set<any> ? "set" : "other";
Building Recursive Conditional Types
Sometimes we need to create types that can handle nested structures. Here’s an example of a deep unwrapping type:
type Unwrap\<T\> = T extends Promise<infer U> ? Unwrap<U> : T extends Array<infer V> ? Array<Unwrap<V>> : T;
Practical Applications
Let’s look at some practical examples where complex conditional types solve real problems:
Type-Safe Event Handler
type EventMap = { click: { x: number; y: number }; keypress: { key: string }; focus: undefined;};
type EventHandler<T extends keyof EventMap> = ( data: EventMap[T]) => void;
Utility Type for API Responses
type ApiResponse\<T\> = { success: true; data: T; error: null;} | { success: false; data: null; error: string;};
Best Practices and Tips
- Break down complex types into smaller, reusable pieces
- Use meaningful names for type parameters
- Add comments to explain complex type logic
- Consider performance implications with deeply nested types
- Test your types with edge cases
Remember, while conditional types are powerful, they should be used judiciously. The goal is to make your code more maintainable and type-safe, not to create unnecessary complexity.
Conclusion
Complex conditional types are a game-changer in TypeScript. They allow us to create sophisticated type-safe abstractions that can greatly improve our code quality. As you build more complex applications, mastering these concepts will become increasingly valuable.
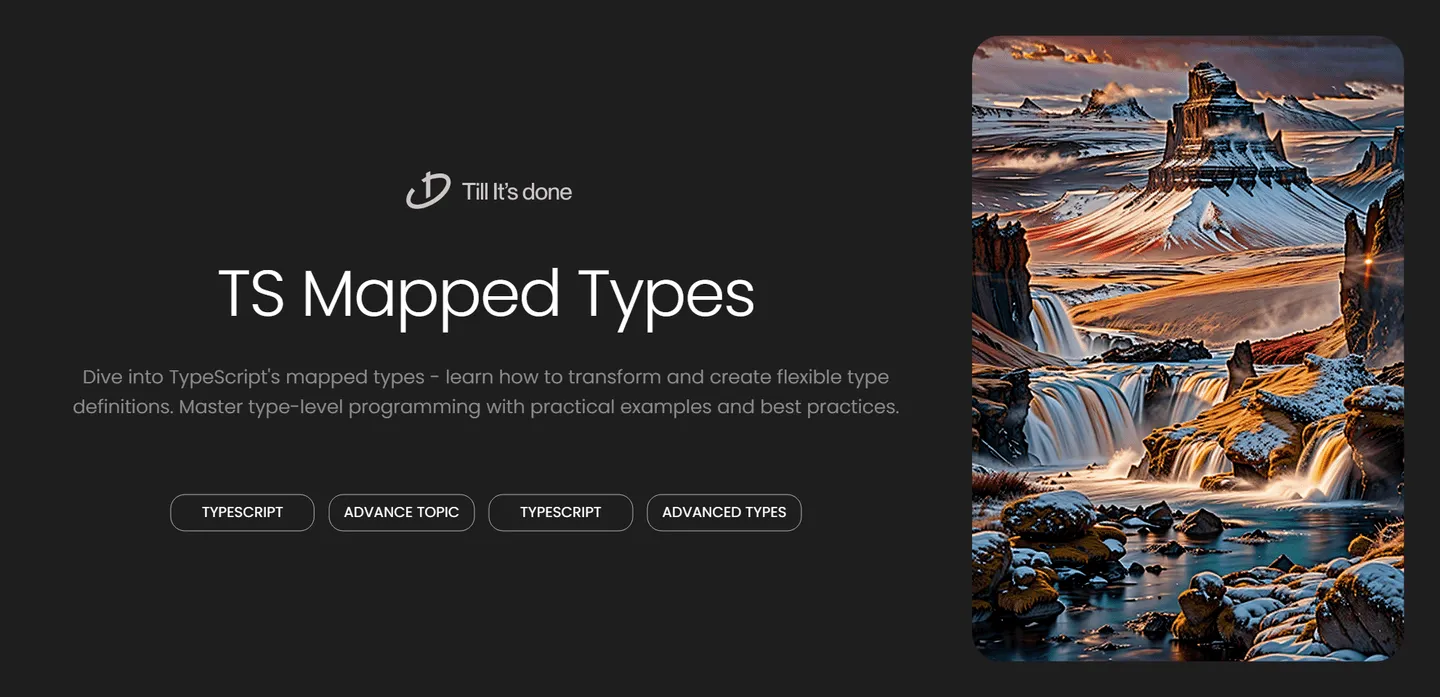
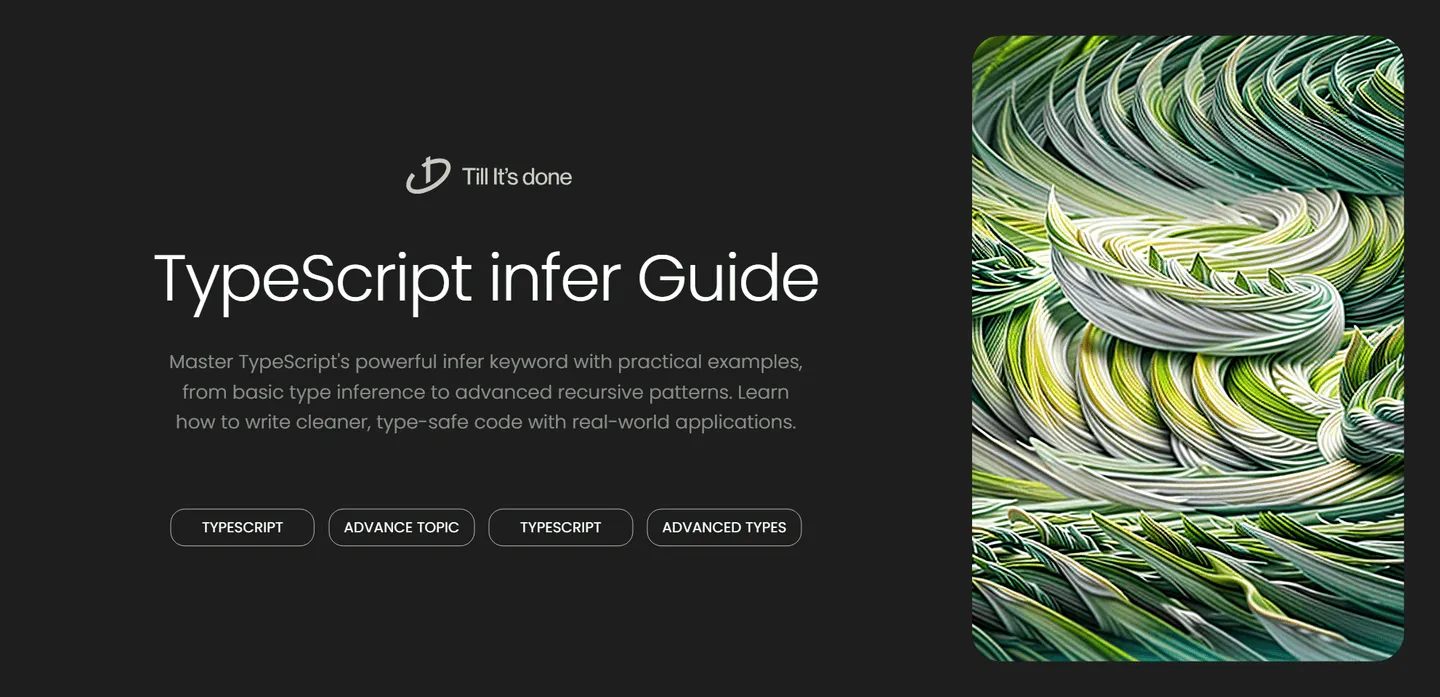
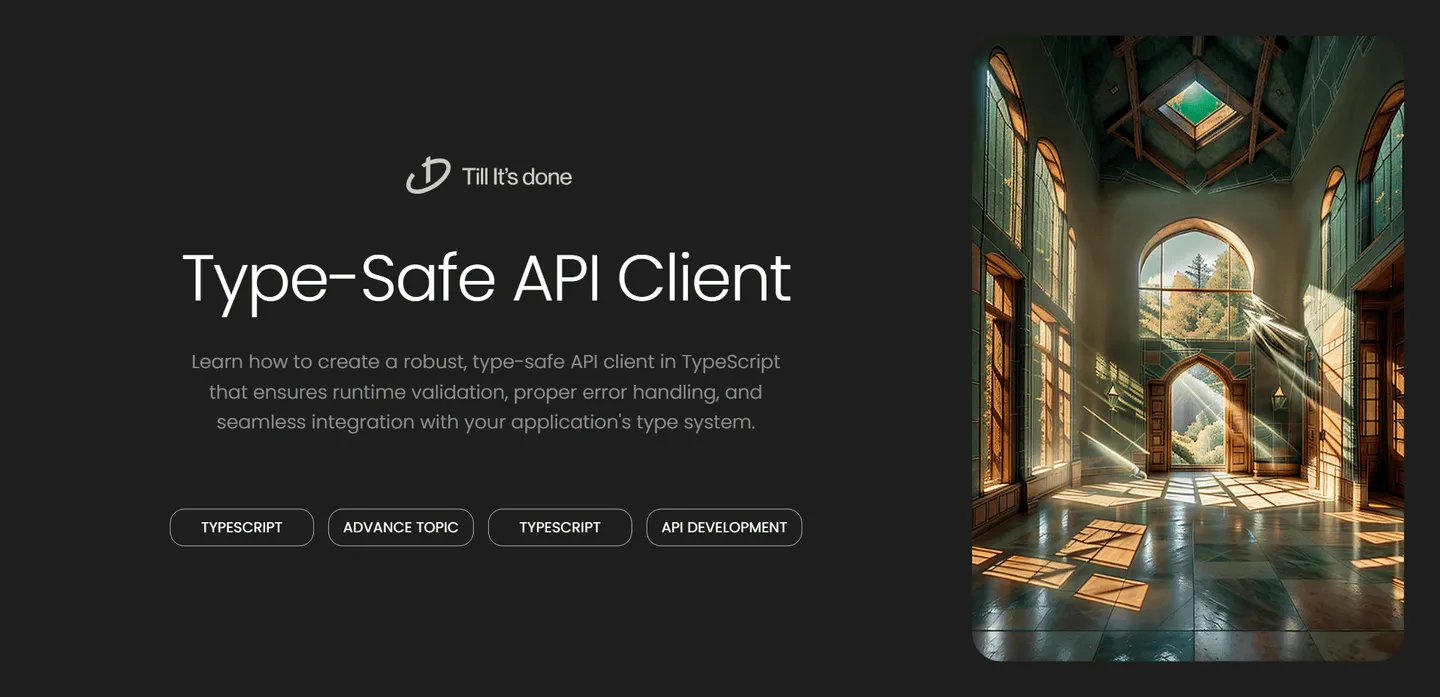
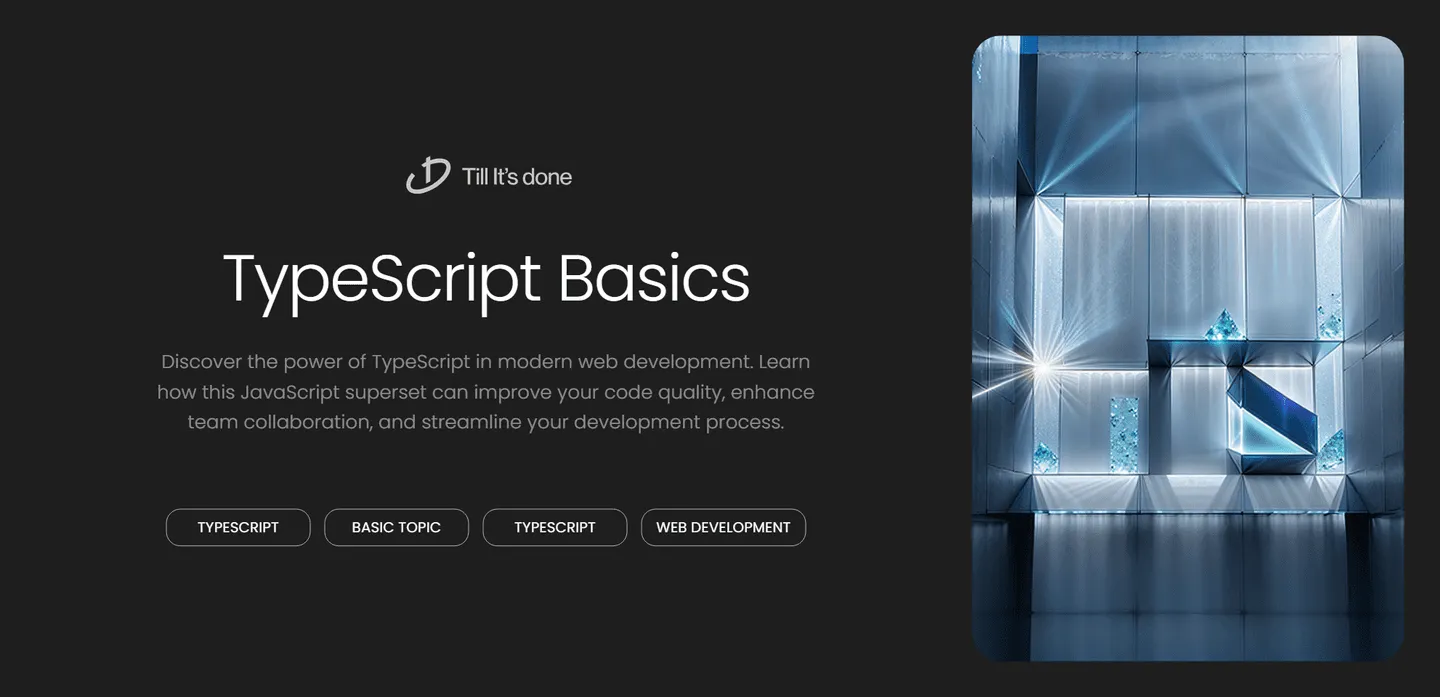
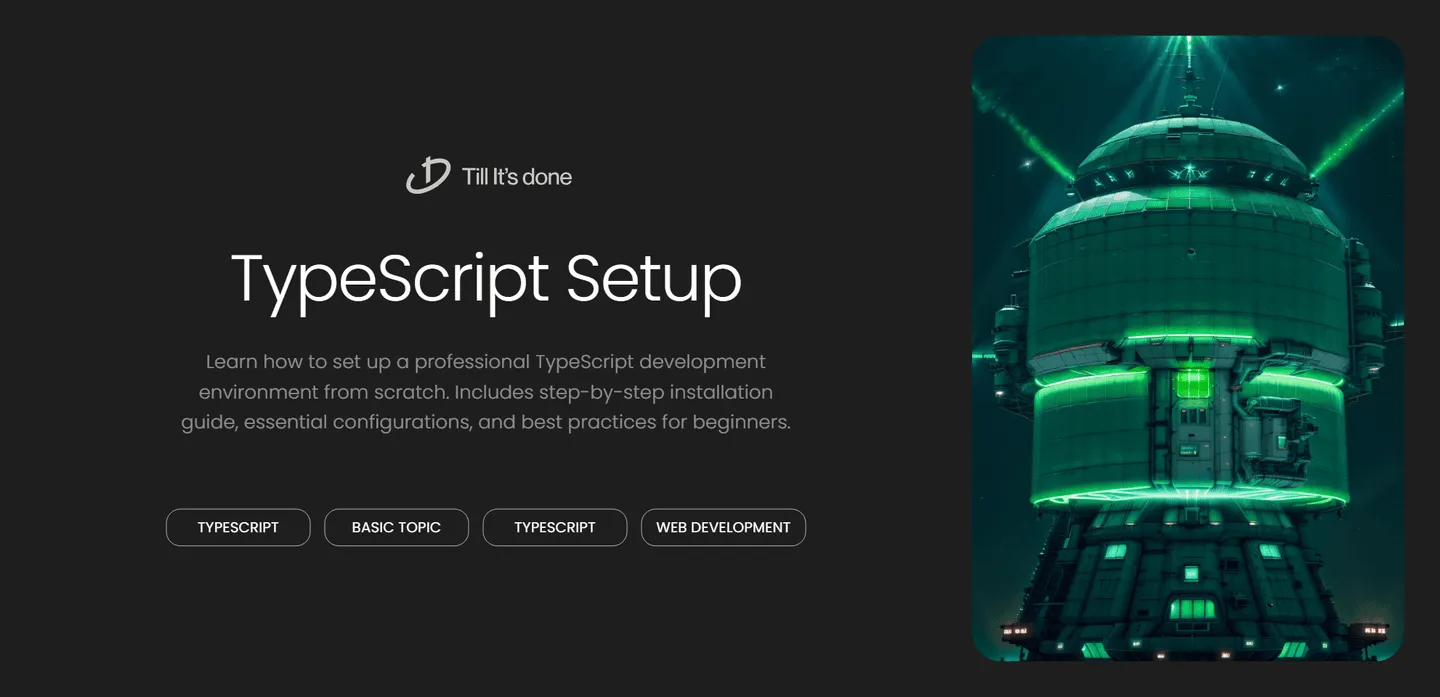
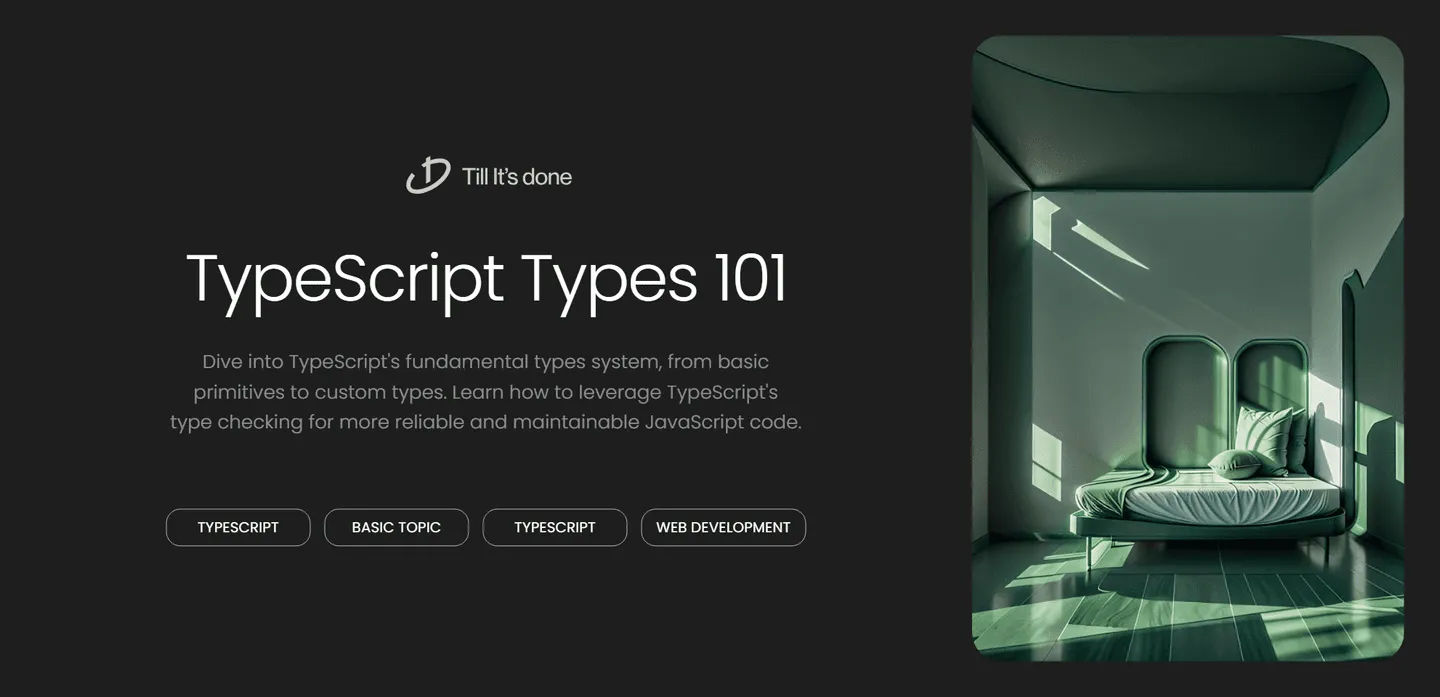
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.