- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Handle Async Validation with Zod in Node.js Apps
Discover best practices, type-safe patterns, and advanced techniques for handling complex validation scenarios.
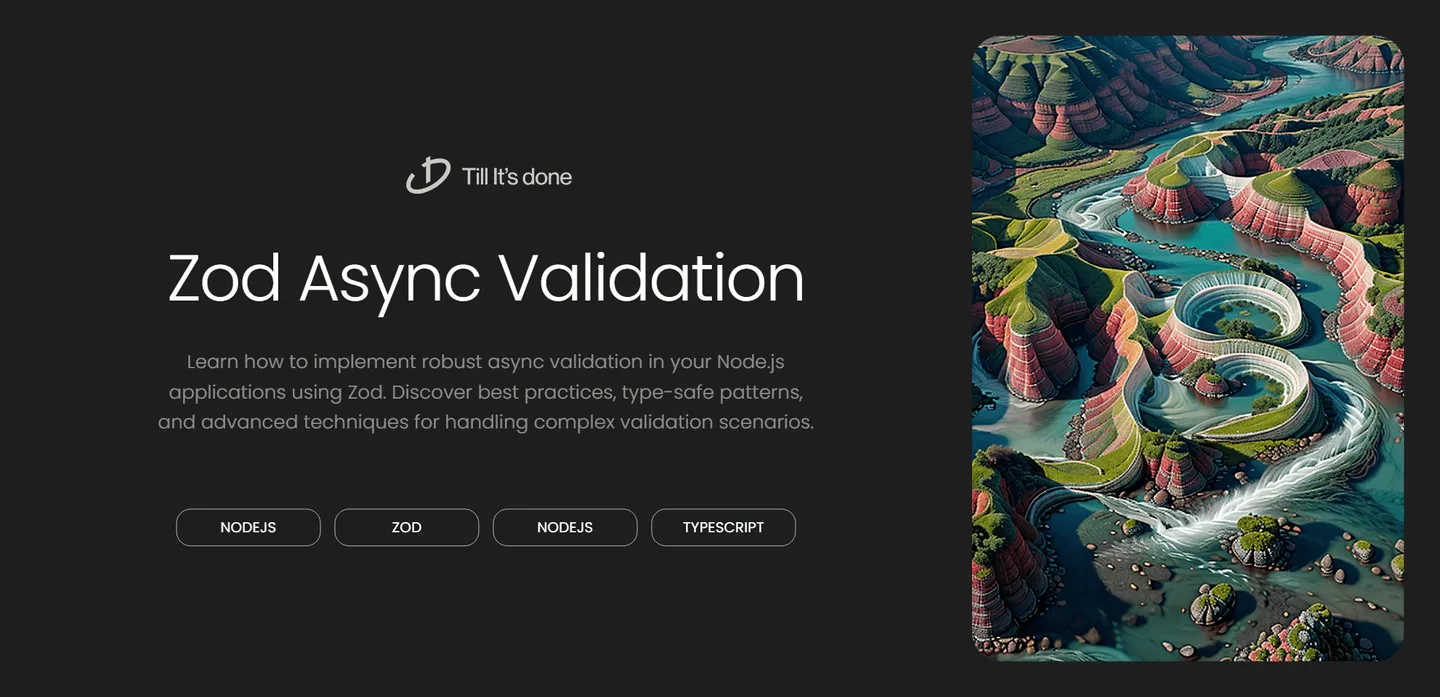
Handling Async Validation with Zod in Node.js
Ever found yourself wrestling with async validation in your Node.js applications? You’re not alone. Today, we’re diving into how Zod, the TypeScript-first schema validation library, can make async validation a breeze while keeping your code clean and type-safe.
The Challenge of Async Validation
When building real-world applications, we often need to validate data against external sources - whether it’s checking if a username is unique in our database or verifying an API key. These operations are inherently asynchronous, and handling them correctly can be tricky.
Enter Zod’s Async Refinements
Zod provides an elegant solution through its .refine()
method, which can handle both synchronous and asynchronous validation. Here’s how you can implement it in your code:
import { z } from "zod";import { db } from "./database";
const UserSchema = z.object({ username: z.string() .min(3) .refine( async (username) => { const user = await db.users.findUnique({ where: { username } }); return !user; // username is available if no user is found }, { message: "Username already taken" } ), email: z.string().email(),});
Best Practices for Async Validation
When working with async validation, keep these tips in mind:
- Always handle potential errors gracefully
- Consider caching validation results when appropriate
- Implement timeouts for external service calls
- Use proper error messages to guide users
Advanced Patterns
Let’s look at a more complex example that combines multiple async validations:
const RegistrationSchema = z.object({ username: z.string(), email: z.string().email(), apiKey: z.string(),}).refine( async (data) => { const [usernameAvailable, emailAvailable, apiKeyValid] = await Promise.all([ checkUsername(data.username), checkEmail(data.email), validateApiKey(data.apiKey), ]); return usernameAvailable && emailAvailable && apiKeyValid; }, { message: "Invalid registration data", path: ["registration"], // error path });
Error Handling and Type Safety
One of the best parts about using Zod for async validation is that it maintains type safety throughout the process. When validation fails, you get strongly typed error objects that you can handle appropriately:
try { const result = await UserSchema.parseAsync(formData); // result is fully typed} catch (error) { if (error instanceof z.ZodError) { console.log(error.errors); // typed validation errors }}
Conclusion
Async validation doesn’t have to be a headache. With Zod’s powerful features and TypeScript integration, you can handle complex validation scenarios while maintaining clean, type-safe code. Start implementing these patterns in your Node.js applications, and you’ll see the difference in both code quality and developer experience.
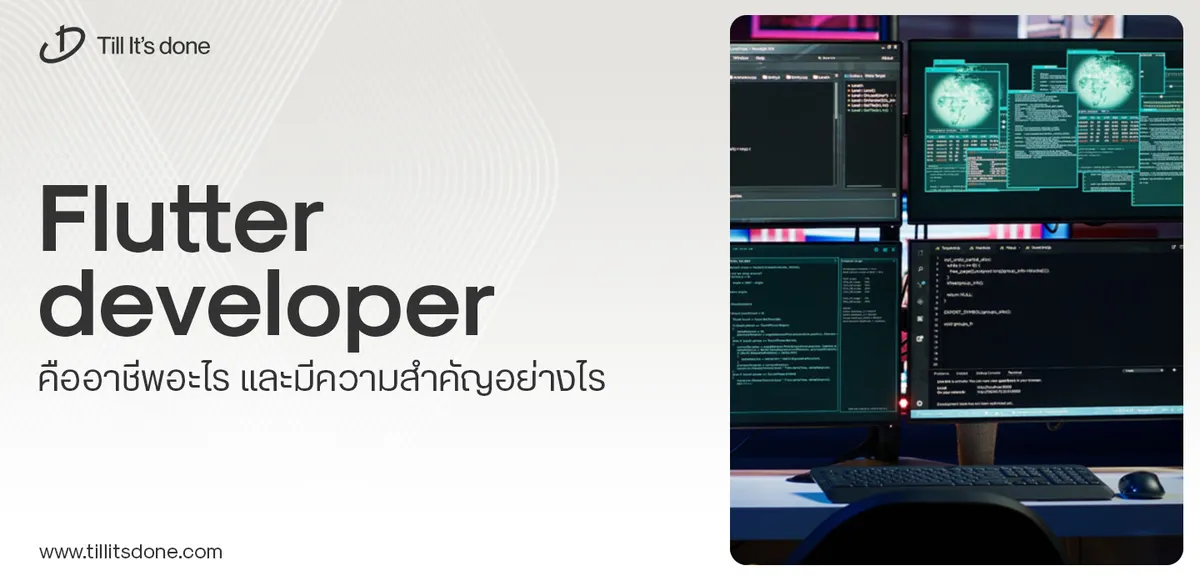
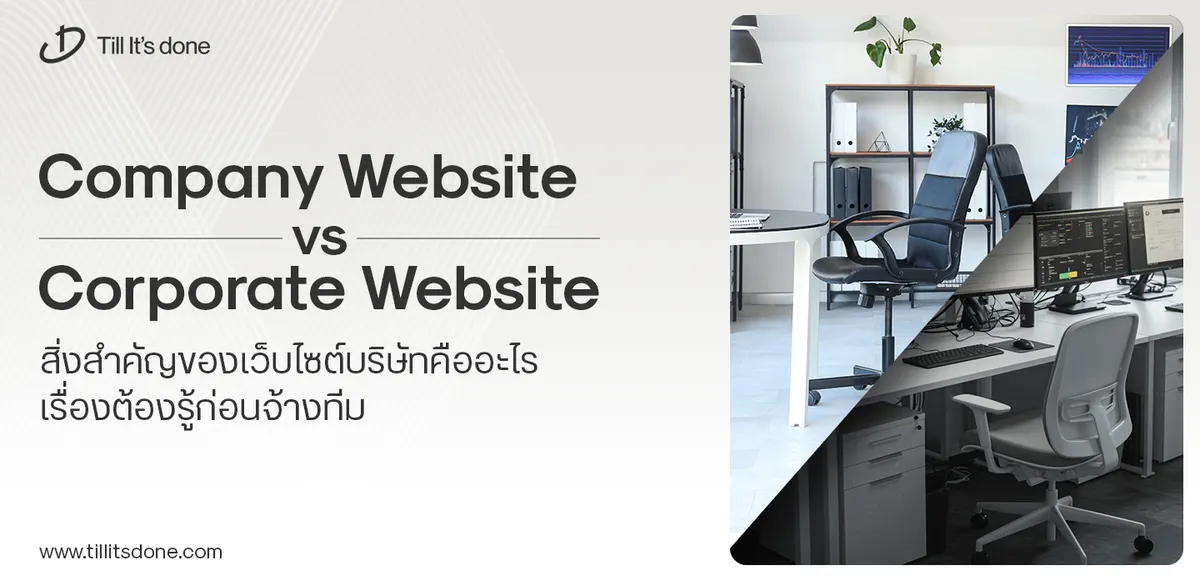
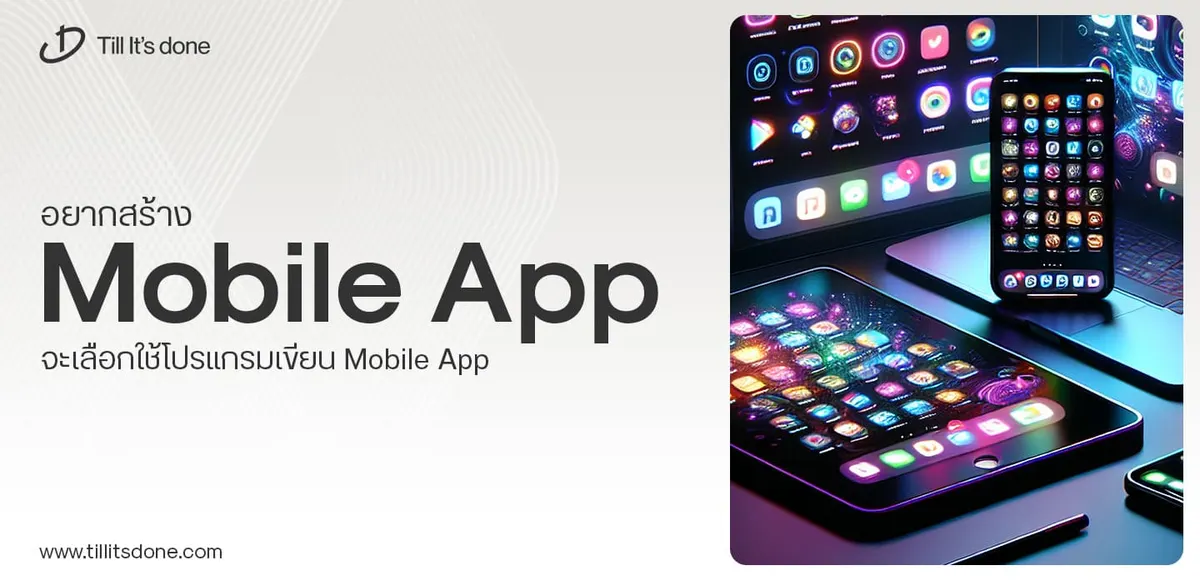
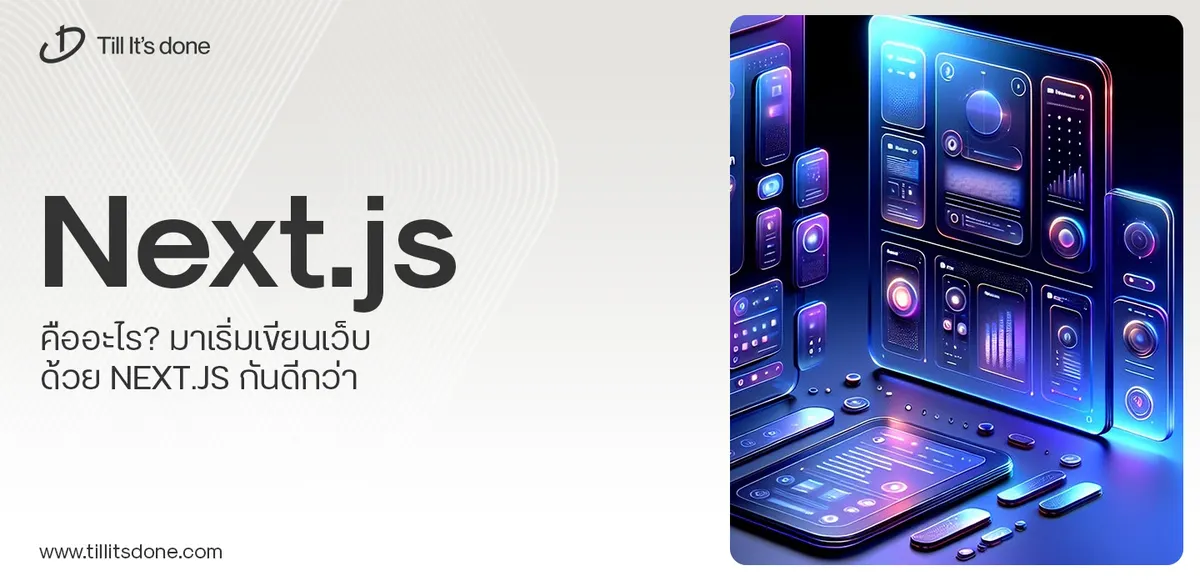
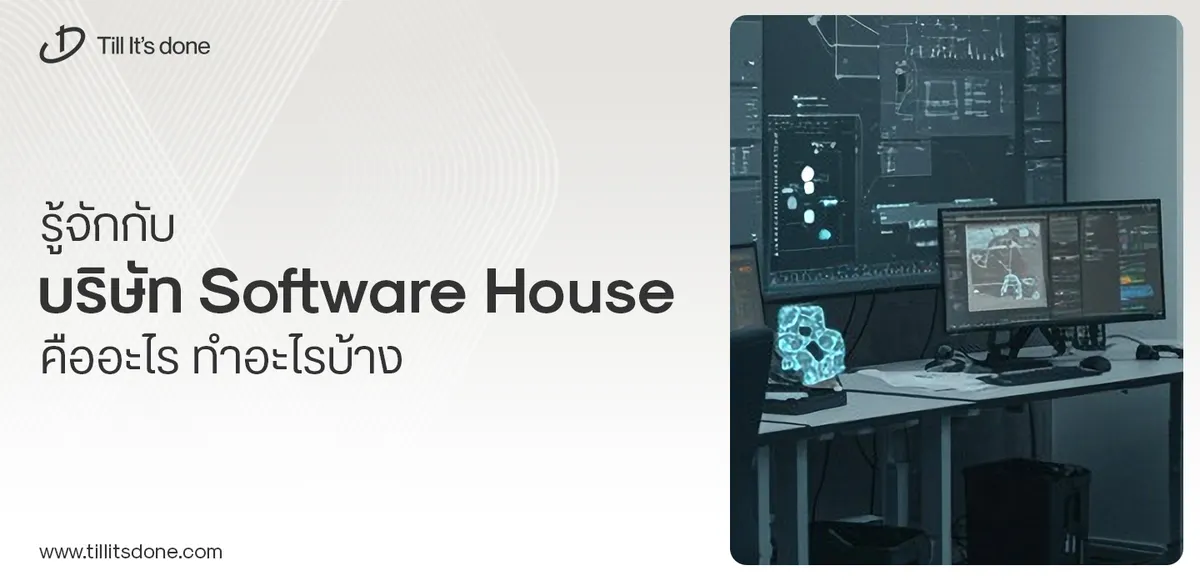
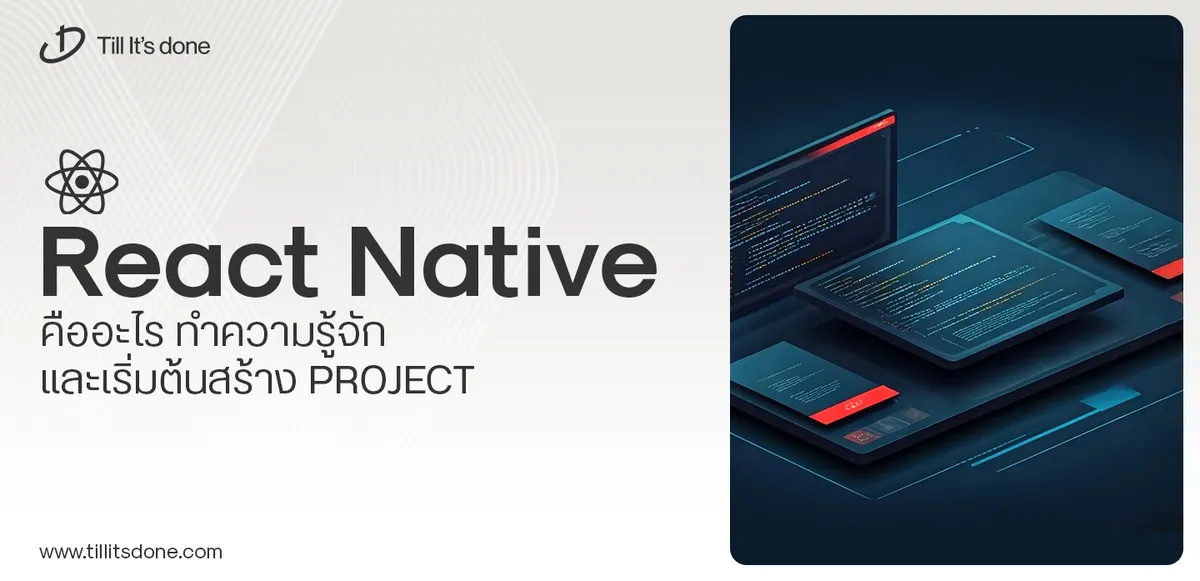
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.