- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Master Async Operations with Axios in Node.js
Discover practical techniques for making HTTP requests, error handling, and managing concurrent operations.
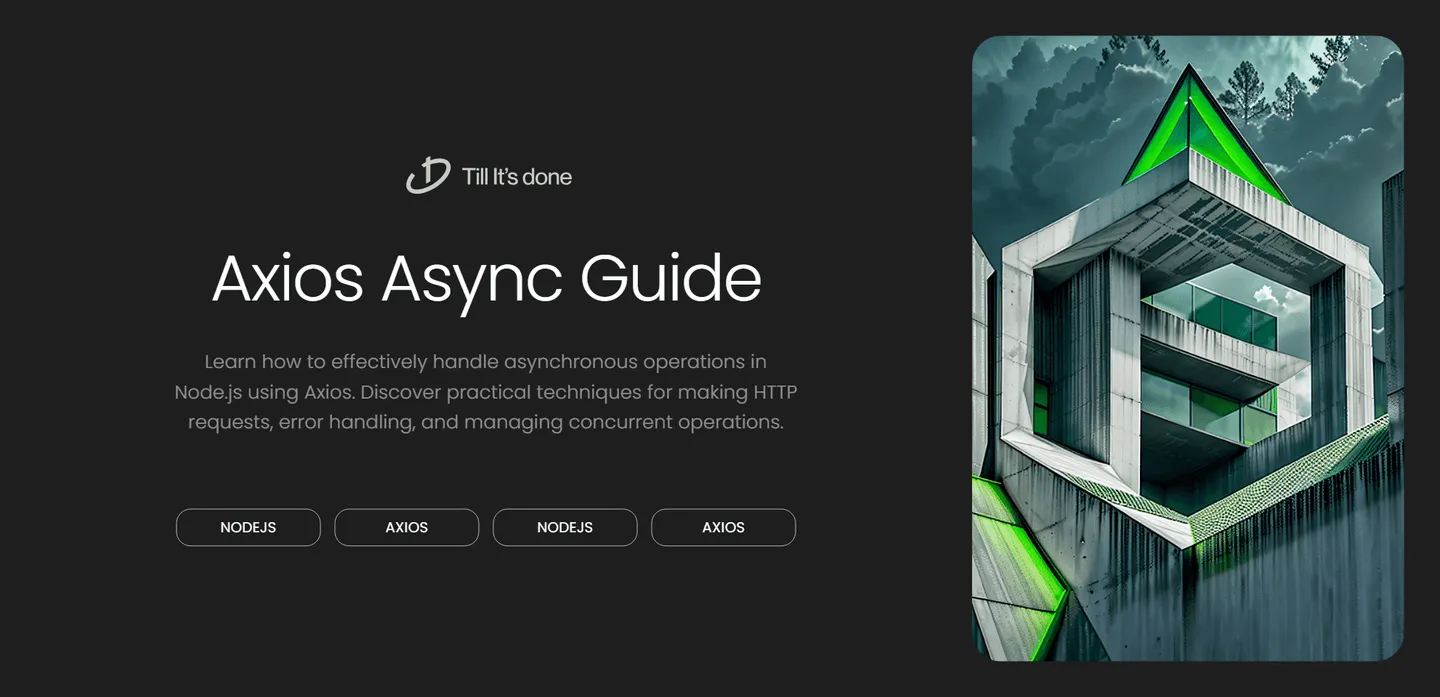
Handling Asynchronous Operations with Axios in Node.js
In today’s fast-paced web development landscape, handling HTTP requests efficiently is crucial. As a Node.js developer, I’ve found Axios to be an invaluable tool for managing asynchronous operations. Let’s dive into how we can master asynchronous requests using Axios in Node.js applications.
Understanding Axios Basics
When I first started working with Axios, I was amazed by its elegant promise-based structure. At its core, Axios simplifies HTTP requests while providing powerful features out of the box. Here’s how we can make basic GET and POST requests:
const axios = require('axios');
// Making a GET requestasync function fetchUserData() { try { const response = await axios.get('https://api.example.com/users'); return response.data; } catch (error) { console.error('Error fetching users:', error.message); }}
Advanced Error Handling
One thing I’ve learned through experience is that robust error handling can make or break your application. Axios provides multiple ways to handle errors gracefully:
const makeApiRequest = async () => { try { const response = await axios.post('https://api.example.com/data', { name: 'John', age: 30 }); console.log('Success:', response.data); } catch (error) { if (error.response) { // Server responded with an error status console.error('Server Error:', error.response.status); } else if (error.request) { // Request was made but no response received console.error('Network Error'); } else { // Something else went wrong console.error('Error:', error.message); } }};
Concurrent Requests with Promise.all
Sometimes we need to handle multiple requests simultaneously. Axios works beautifully with Promise.all, allowing us to execute parallel requests efficiently:
async function fetchMultipleEndpoints() { try { const endpoints = [ 'https://api.example.com/users', 'https://api.example.com/posts', 'https://api.example.com/comments' ];
const requests = endpoints.map(endpoint => axios.get(endpoint)); const responses = await Promise.all(requests);
return responses.map(response => response.data); } catch (error) { console.error('One or more requests failed:', error); }}
Interceptors and Global Configuration
Working with Axios becomes even more powerful when you utilize interceptors and global configurations. These features have saved me countless hours of repetitive coding:
// Global configurationaxios.defaults.baseURL = 'https://api.example.com';axios.defaults.timeout = 5000;
// Request interceptoraxios.interceptors.request.use(config => { config.headers['Authorization'] = `Bearer ${getToken()}`; return config;}, error => { return Promise.reject(error);});
// Response interceptoraxios.interceptors.response.use(response => { return response;}, error => { if (error.response.status === 401) { // Handle unauthorized access refreshToken(); } return Promise.reject(error);});
Remember, when working with asynchronous operations, it’s crucial to maintain a balance between performance and code readability. Axios helps achieve both, making it an excellent choice for modern Node.js applications.
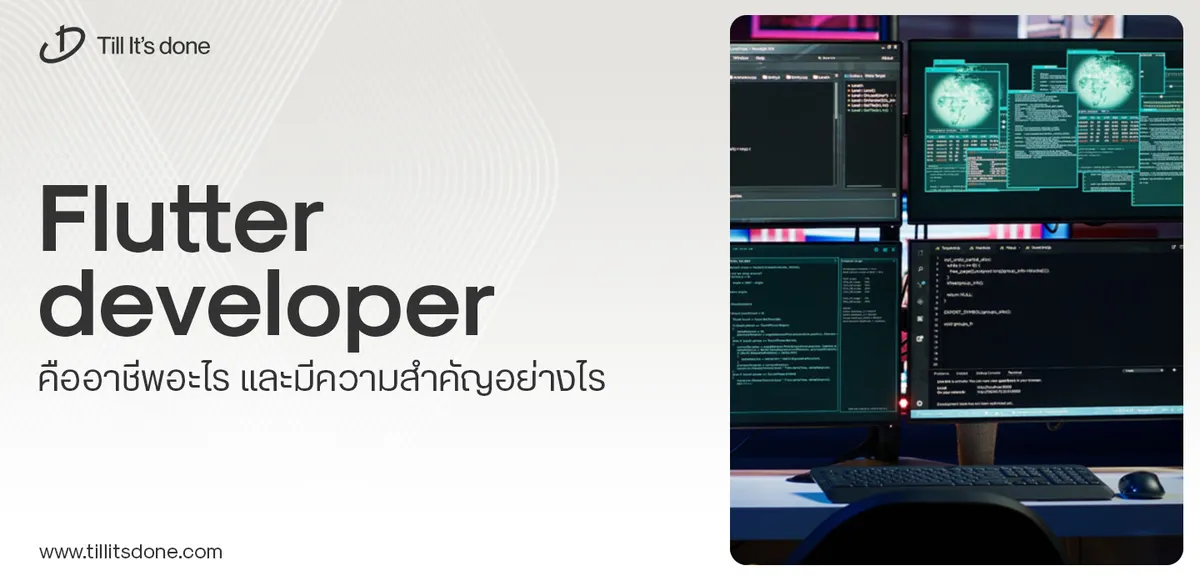
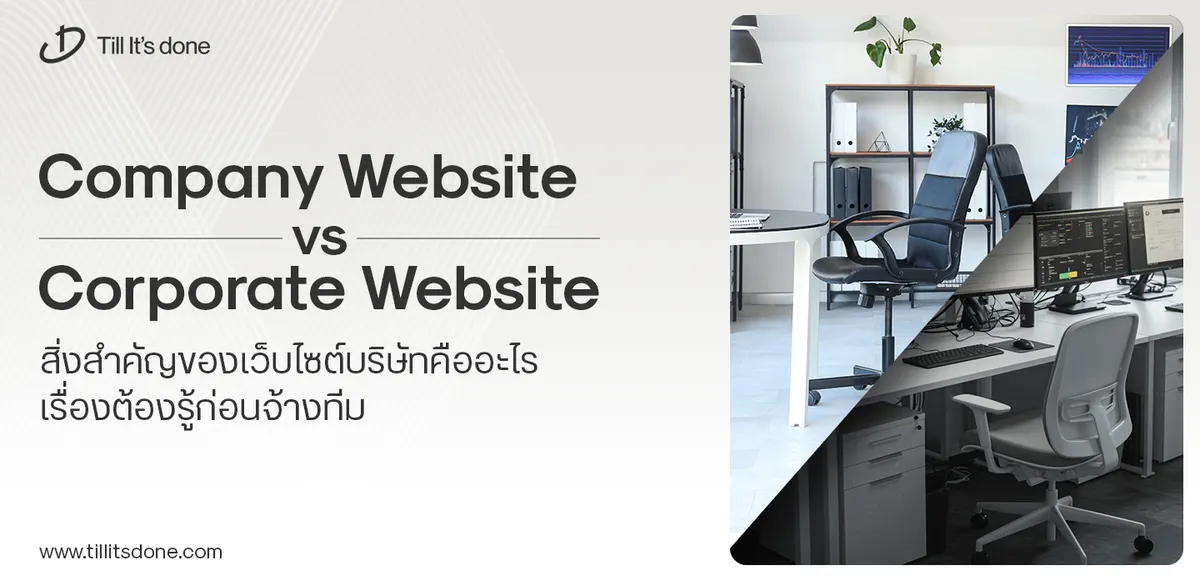
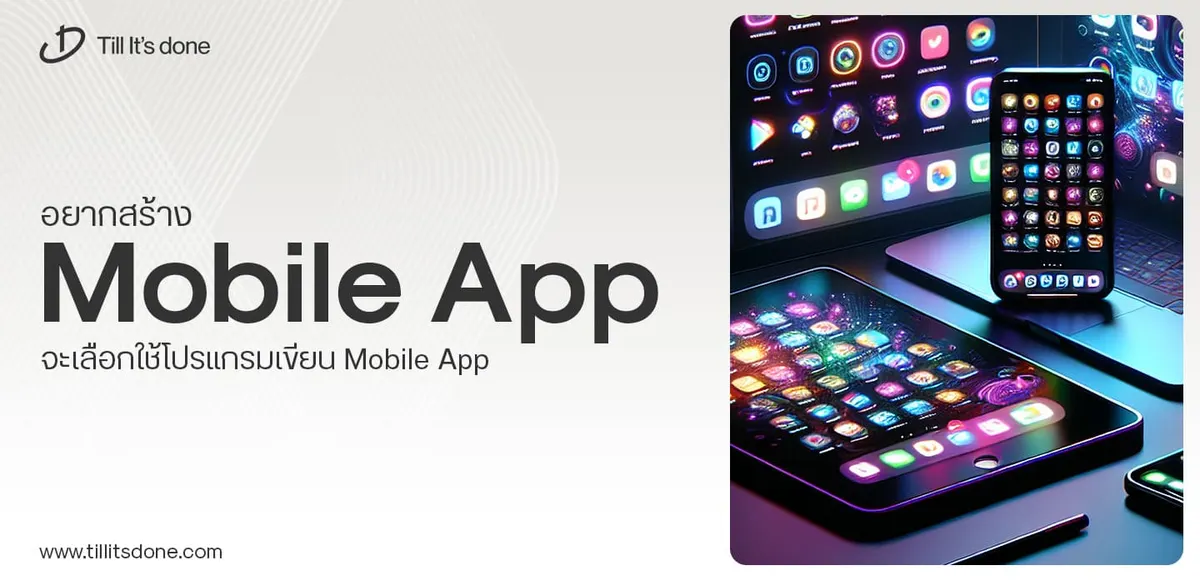
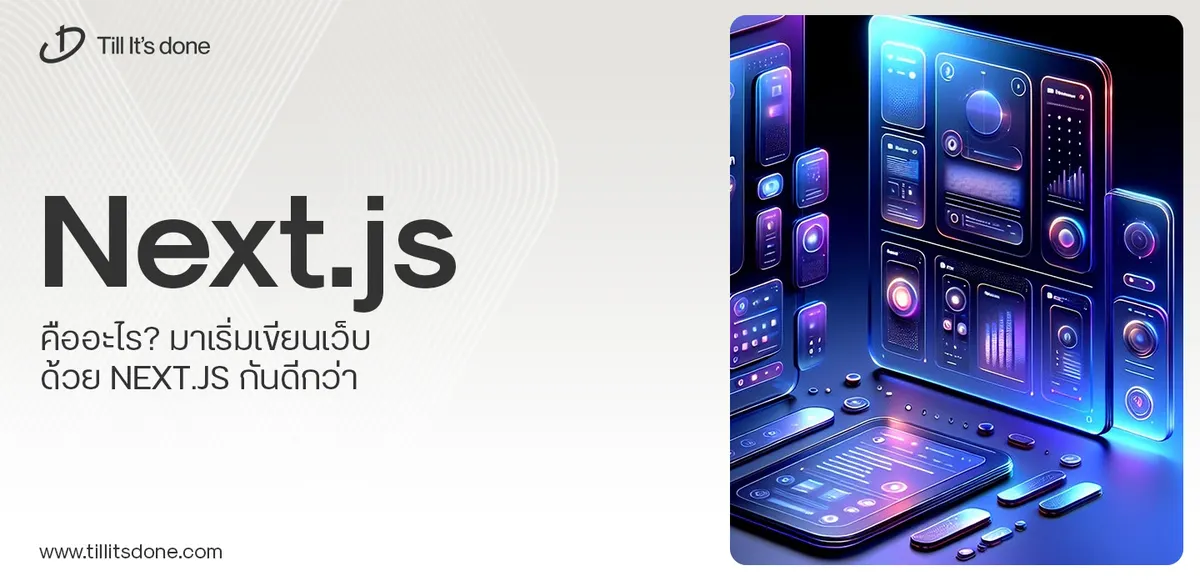
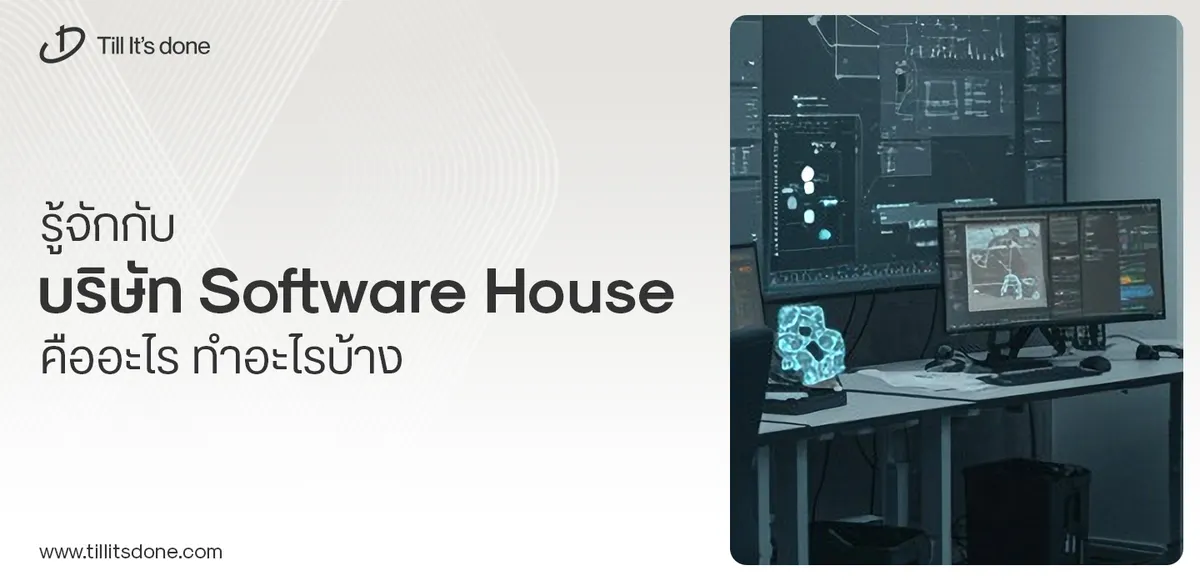
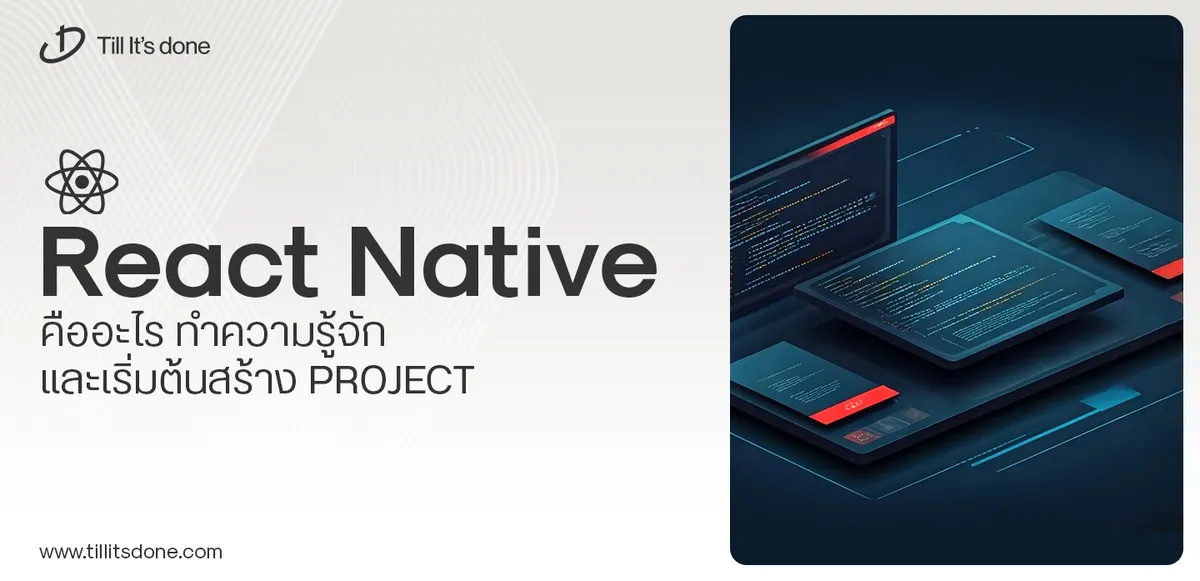
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.