- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Handling Asynchronous Data with Lodash in Node.js
Master parallel processing, throttling, and batch operations.

Handling Asynchronous Data with Lodash in Node.js
Working with asynchronous data in Node.js can sometimes feel like juggling multiple balls in the air. While JavaScript provides powerful built-in methods for handling promises and callbacks, combining these with data manipulation can quickly become complex. That’s where Lodash steps in – it’s like having a Swiss Army knife for data operations that plays nicely with Node.js’s asynchronous nature.
The Power of Lodash in Async Operations
One of the biggest challenges developers face is managing multiple asynchronous operations while maintaining clean, readable code. Lodash provides elegant solutions for these scenarios through its collection of utility functions that work seamlessly with promises and async operations.
Practical Examples in Action
Let’s explore some common scenarios where Lodash shines in handling async data:
Throttling API Requests
When dealing with multiple API requests, you’ll want to avoid overwhelming your server. Lodash’s _.throttle()
function is perfect for this:
const throttledRequest = _.throttle(async (data) => { const response = await makeAPIRequest(data); return response;}, 2000);
Batch Processing with Promise Chains
Sometimes you need to process large amounts of data in chunks. Lodash makes this elegant:
const processInChunks = async (items) => { const chunks = _.chunk(items, 5); const results = await Promise.all( chunks.map(chunk => Promise.all(chunk.map(item => processItem(item))) ) ); return _.flatten(results);};
Advanced Techniques
Lodash really shows its strength when combined with async/await patterns. Here’s a powerful pattern for handling parallel operations with rate limiting:
const asyncMap = async (array, iteratee, concurrency = 3) => { const chunks = _.chunk(array, concurrency); const results = [];
for (const chunk of chunks) { const chunkResults = await Promise.all( chunk.map(item => iteratee(item)) ); results.push(...chunkResults); }
return results;};
Best Practices and Tips
- Always consider memory usage when working with large datasets
- Use Lodash’s chain() for complex data transformations
- Combine Lodash methods with native Promise methods for optimal performance
- Take advantage of Lodash’s memoization for expensive async operations
Remember that while Lodash is powerful, it’s essential to use it judiciously. Not every operation needs to be wrapped in a Lodash method – sometimes vanilla JavaScript is the better choice.
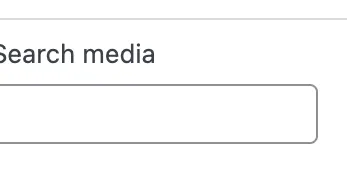





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.