- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Managing Data Fetching in AstroJS with API Routes
Discover efficient patterns for caching, error handling, and rate limiting to build robust web applications.
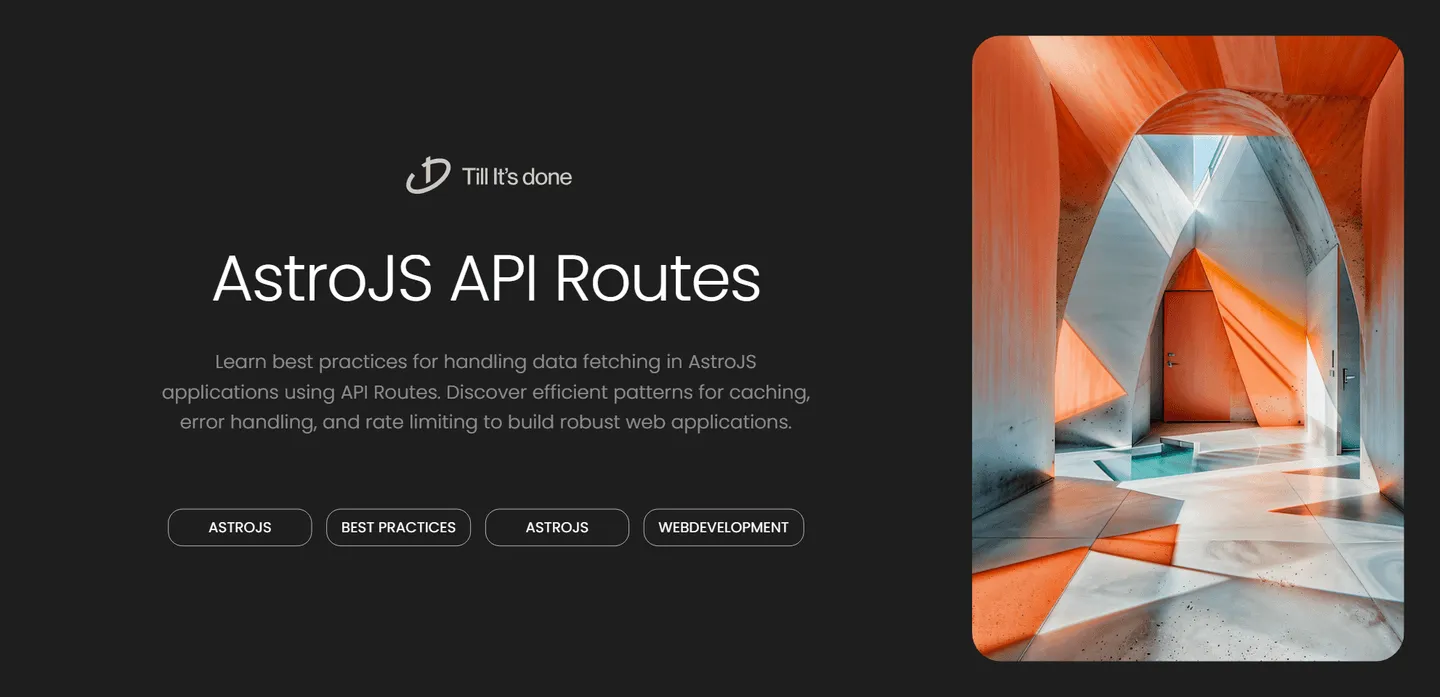
Ever wondered how to efficiently handle data fetching in your AstroJS applications? Today, we’re diving deep into one of the most powerful features of AstroJS - API Routes. I’ll share my experiences and best practices that I’ve learned while building production applications.
Why API Routes?
Think of API routes as your application’s data highway. Instead of directly connecting your frontend to external services, API routes act as a secure middleware layer. This approach has saved me countless hours of debugging and made my applications more maintainable.
Setting Up API Routes in AstroJS
Creating an API route in AstroJS is surprisingly straightforward. In the src/pages/api
directory, we can define our endpoints. Here’s a real-world example I’ve used:
export async function GET() { try { const users = await db.query('SELECT * FROM users'); return new Response(JSON.stringify(users), { status: 200, headers: { 'Content-Type': 'application/json' } }); } catch (error) { return new Response(JSON.stringify({ error: error.message }), { status: 500 }); }}
Best Practices for Data Fetching
- Implement Caching Strategies
Remember to leverage AstroJS’s built-in caching capabilities. I’ve found that proper caching can dramatically improve application performance, especially for frequently accessed data.
- Error Handling
Always implement robust error handling. Here’s a pattern I swear by:
export async function safeFetch(url: string) { try { const response = await fetch(url); if (!response.ok) throw new Error(`HTTP error! status: ${response.status}`); return await response.json(); } catch (error) { console.error('Fetch error:', error); throw error; }}
- Rate Limiting
Don’t forget to implement rate limiting for your API routes. This has saved my applications from potential abuse numerous times.
Advanced Patterns
One pattern that’s worked wonders for me is implementing a request queue for heavy operations:
export class RequestQueue { private queue: Array<() => Promise<any>> = []; private processing = false;
async add(request: () => Promise<any>) { this.queue.push(request); if (!this.processing) { await this.process(); } }
private async process() { this.processing = true; while (this.queue.length > 0) { const request = this.queue.shift(); await request(); } this.processing = false; }}
Conclusion
Remember, the key to successful data fetching in AstroJS isn’t just about writing code - it’s about creating maintainable, scalable patterns that grow with your application. Start with these patterns, adapt them to your needs, and you’ll be well on your way to building robust applications.
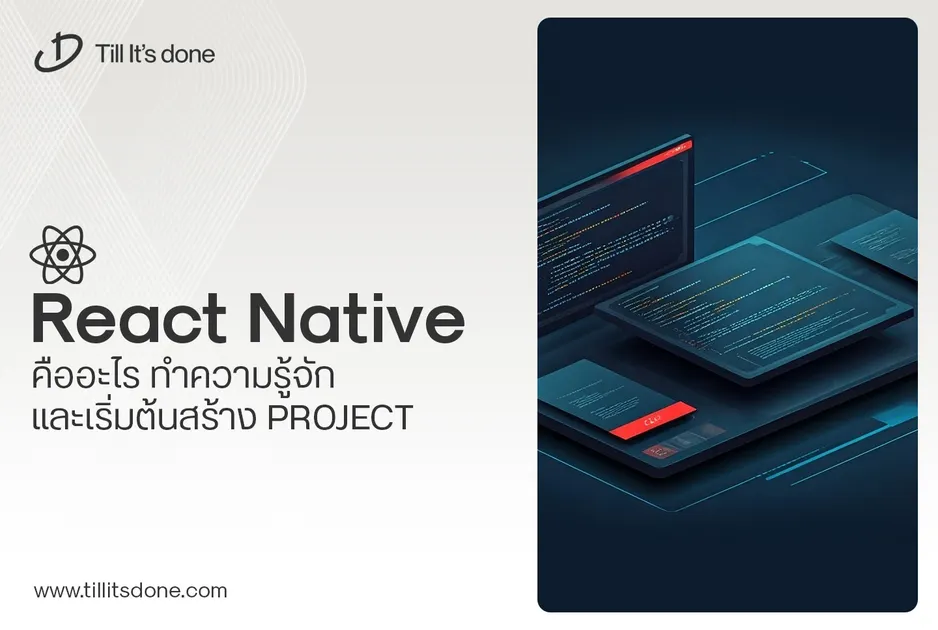
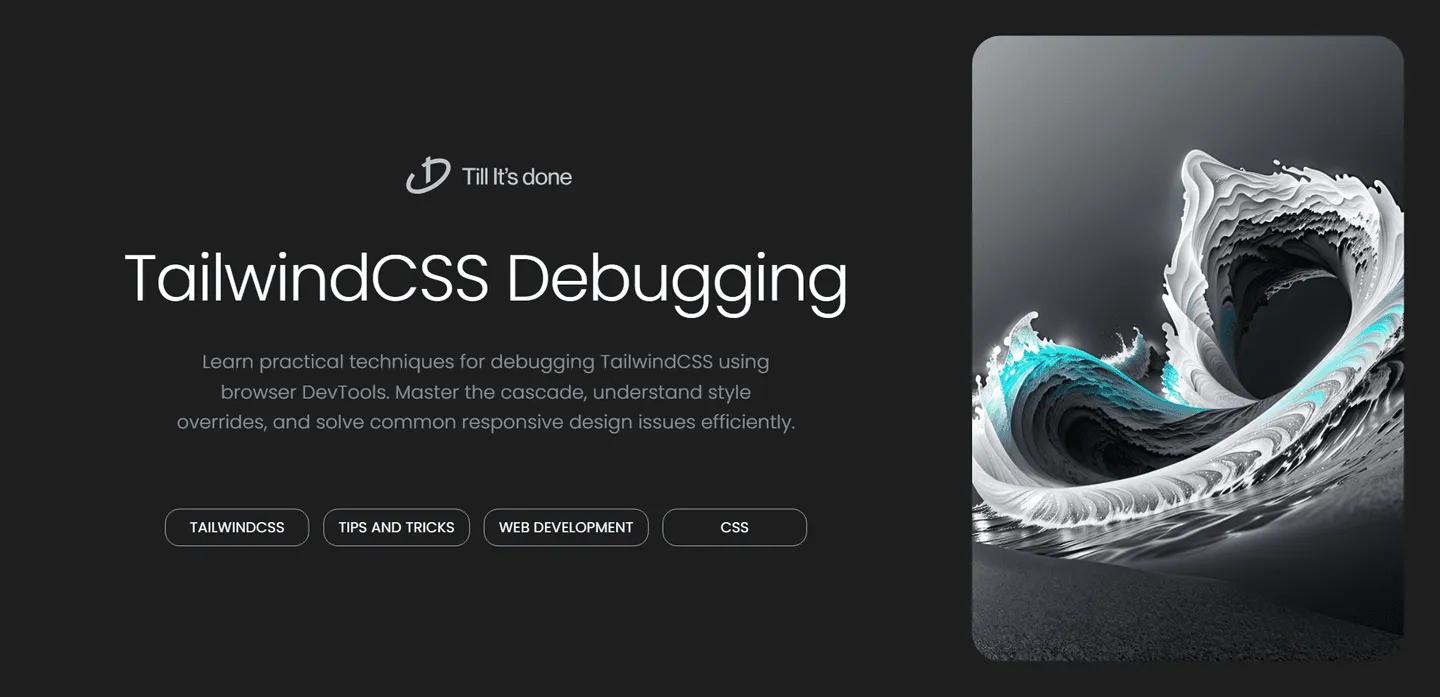
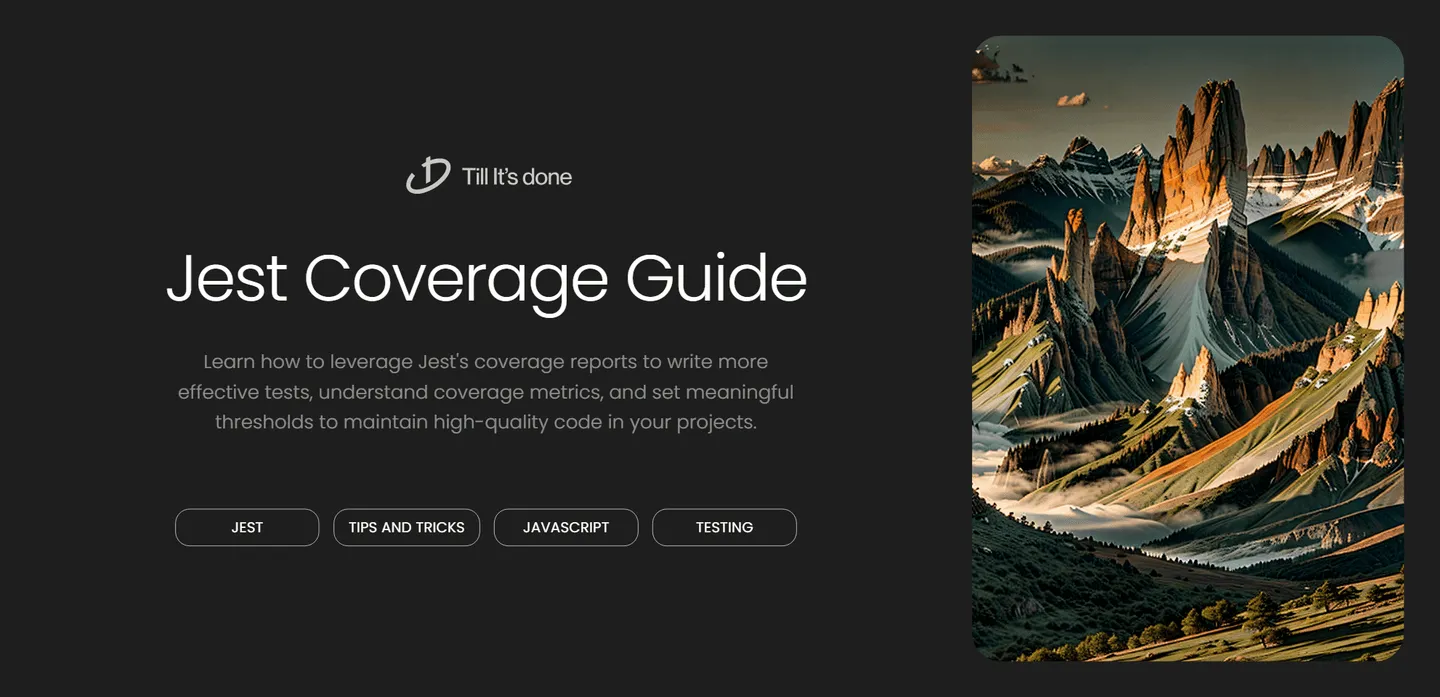
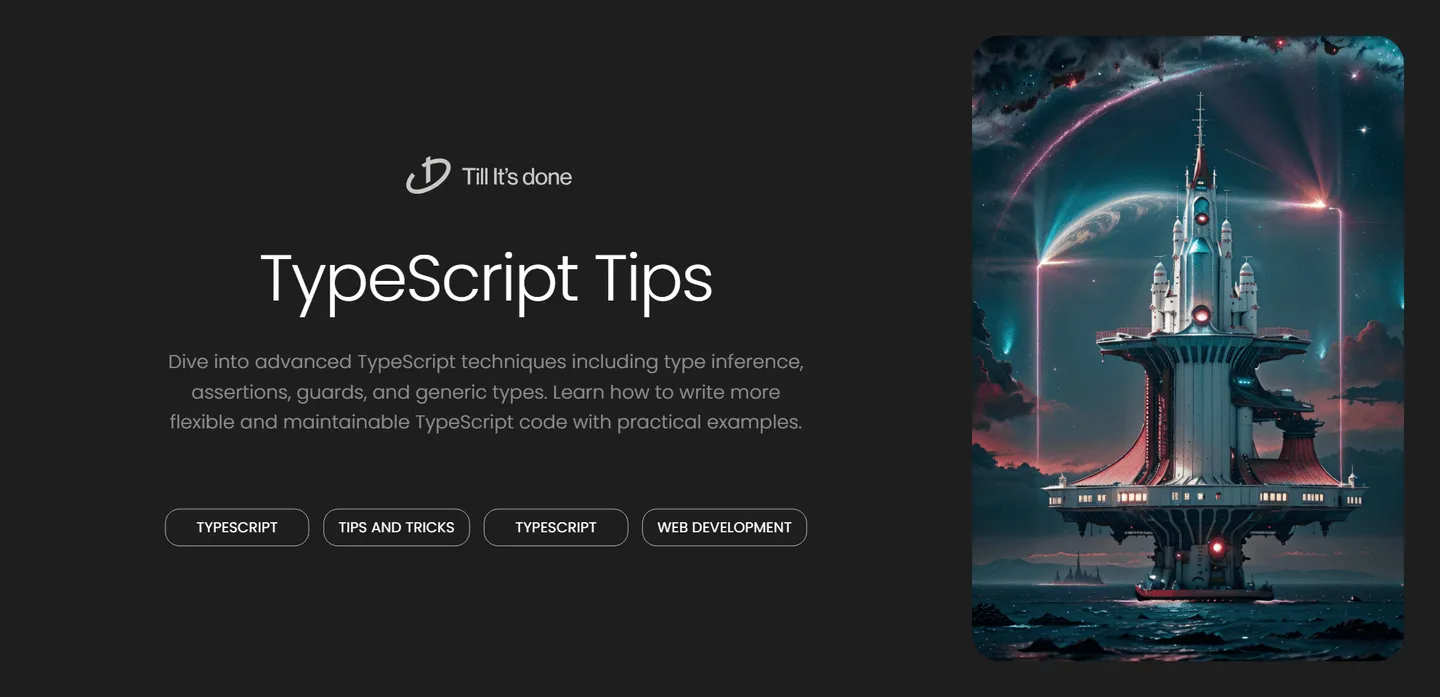
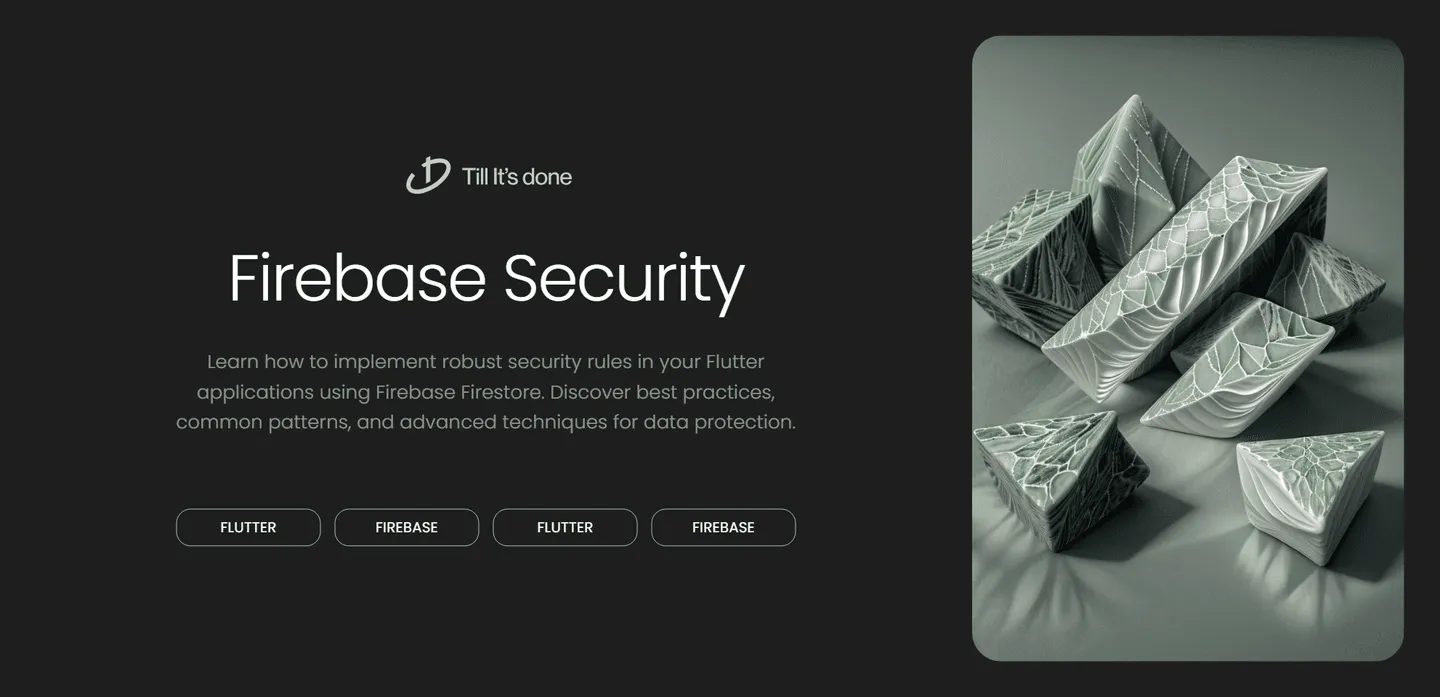
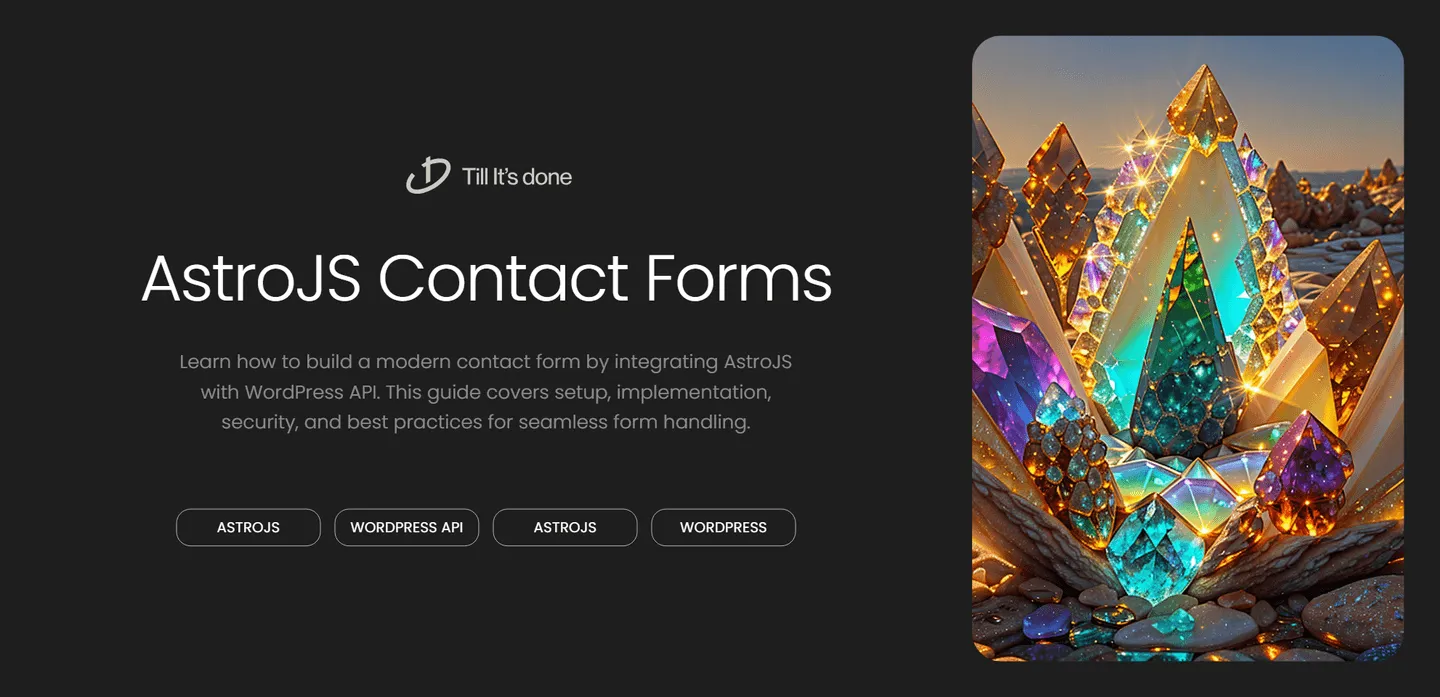
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.