- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Getting Started with Apollo Client in ReactJS
Explore setup, queries, mutations, and best practices for building scalable React applications.

Getting Started with Apollo Client in ReactJS
Are you tired of managing complex API calls and state management in your React applications? Apollo Client might just be the solution you’re looking for. In this guide, we’ll explore how to integrate Apollo Client with ReactJS to create efficient and scalable applications that handle GraphQL data like a breeze.
What is Apollo Client?
Apollo Client is a comprehensive state management library that simplifies the process of working with GraphQL APIs in your frontend applications. Think of it as your personal data courier, seamlessly fetching, caching, and managing data between your React application and your GraphQL server.
Setting Up Apollo Client
Let’s start by adding Apollo Client to your React project. First, install the necessary dependencies:
npm install @apollo/client graphql
Next, create your Apollo Client instance. In your project’s root file (usually index.js
or App.js
), add the following configuration:
import { ApolloClient, InMemoryCache, ApolloProvider } from '@apollo/client';
const client = new ApolloClient({ uri: 'YOUR_GRAPHQL_API_ENDPOINT', cache: new InMemoryCache()});
Wrap your React application with the ApolloProvider to make the client available throughout your component tree:
function App() { return ( <ApolloProvider client={client}> <YourAppComponents /> </ApolloProvider> );}
Making Your First Query
Now comes the exciting part - fetching data! Apollo Client provides the useQuery
hook for making GraphQL queries in your components:
import { useQuery, gql } from '@apollo/client';
const GET_BOOKS = gql` query GetBooks { books { title author } }`;
function BookList() { const { loading, error, data } = useQuery(GET_BOOKS);
if (loading) return <p>Loading...</p>; if (error) return <p>Error :(</p>;
return ( <ul> {data.books.map(book => ( <li key={book.title}> {book.title} by {book.author} </li> ))} </ul> );}
Mutations and Cache Updates
Apollo Client really shines when it comes to mutations. Here’s how you can update data on your server and automatically update your UI:
const ADD_BOOK = gql` mutation AddBook($title: String!, $author: String!) { addBook(title: $title, author: $author) { title author } }`;
function AddBook() { const [addBook] = useMutation(ADD_BOOK, { update(cache, { data: { addBook } }) { cache.modify({ fields: { books(existingBooks = []) { const newBookRef = cache.writeFragment({ data: addBook, fragment: gql` fragment NewBook on Book { title author } ` }); return [...existingBooks, newBookRef]; } } }); } });}
Best Practices and Tips
- Always use fragments to share common fields between queries
- Implement proper error handling and loading states
- Take advantage of Apollo Client’s powerful caching capabilities
- Use the Apollo Developer Tools browser extension for debugging
- Consider implementing optimistic UI updates for better user experience
With Apollo Client, you’ve got a powerful toolkit at your disposal for building robust React applications with GraphQL. The combination of declarative data fetching, intelligent caching, and seamless integration with React makes it an invaluable addition to your development stack.
Start small, experiment with the features, and gradually incorporate more advanced concepts as you become comfortable with the basics. Happy coding!

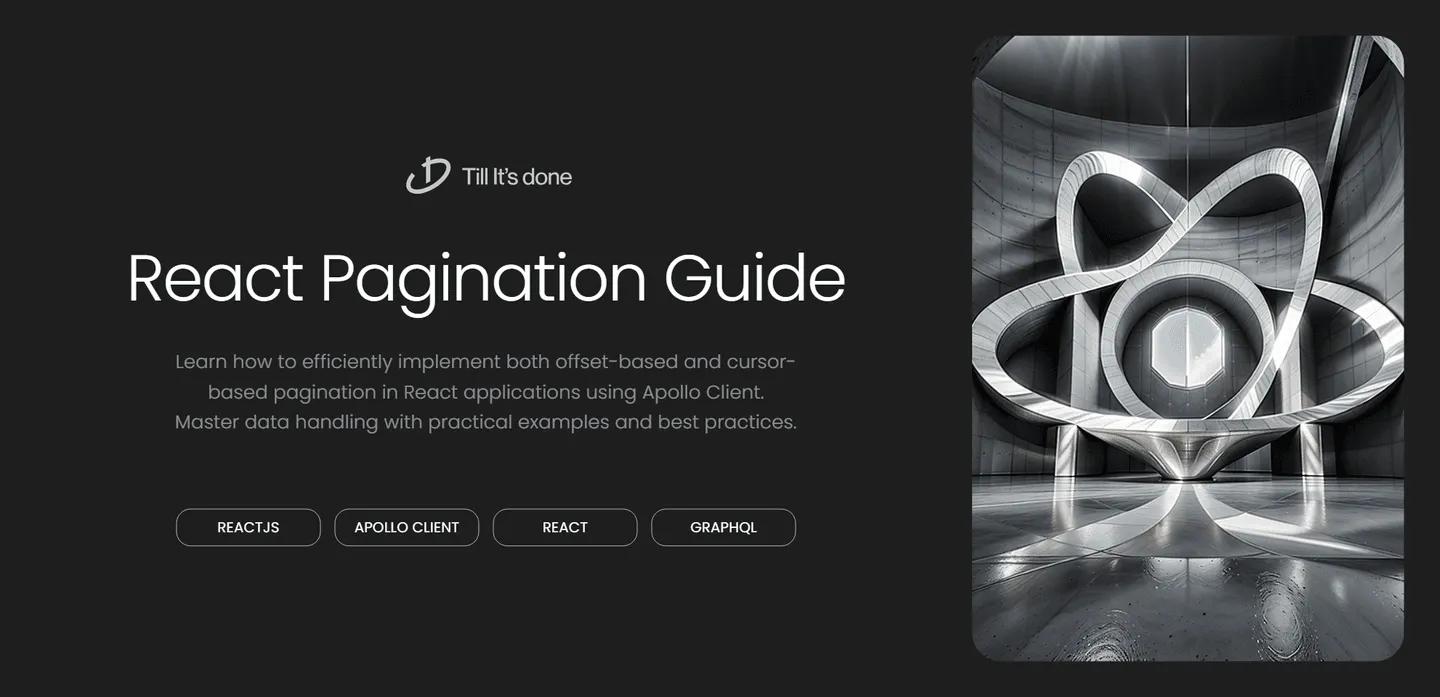




Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.