- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Advanced Mocking with Jest: Test Like a Pro
Learn how to effectively use spies, stubs, and mocks to create robust tests for your JavaScript applications.
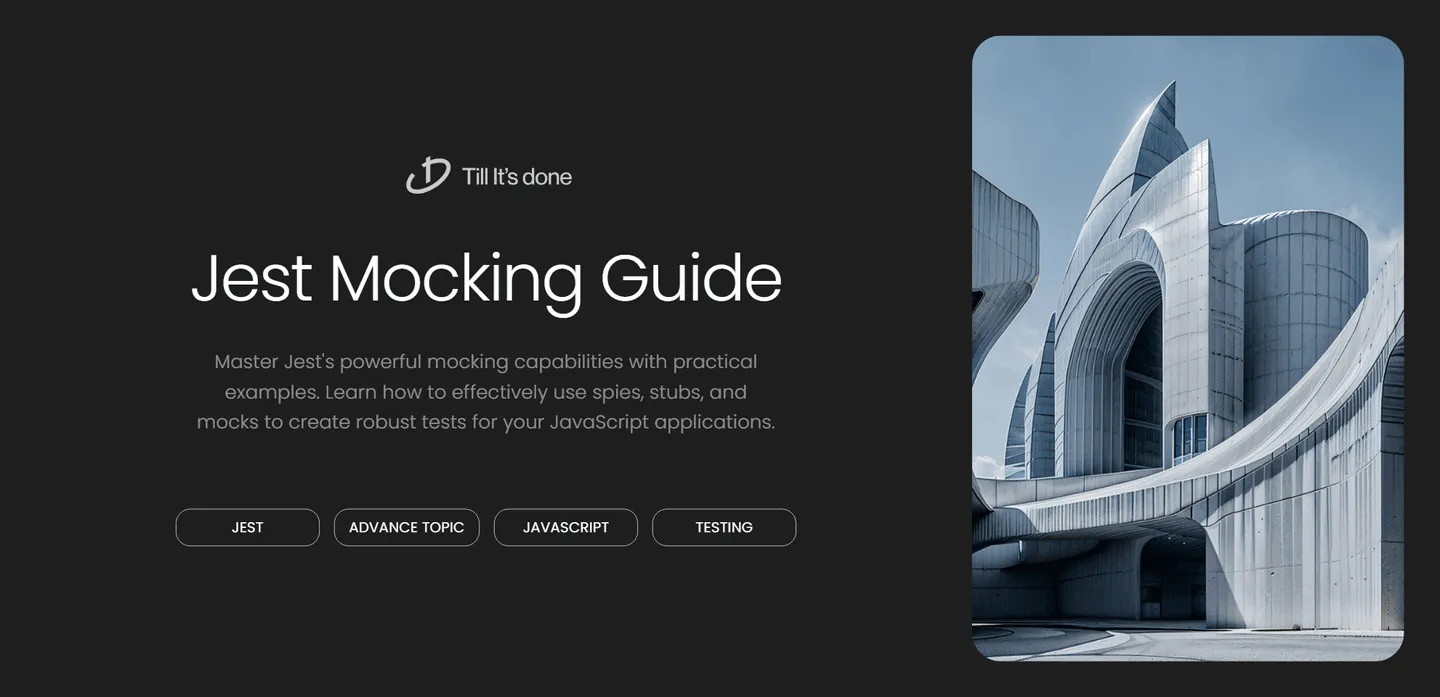
Advanced Mocking Techniques with Jest: Spies, Stubs, and Mocks
Testing modern applications can be tricky, especially when dealing with complex dependencies and external services. That’s where Jest’s advanced mocking capabilities come in handy. Let’s dive deep into the world of spies, stubs, and mocks to level up your testing game.
Understanding the Basics
Before we jump into the advanced stuff, let’s quickly refresh our understanding of these testing tools:
- Spies: Think of them as watchful observers that track how functions are used
- Stubs: Like stand-ins for real functions, providing predetermined responses
- Mocks: The complete replacements that can verify behavior
Spying on Functions
Spies are incredibly useful when you want to ensure that functions are called correctly without changing their actual behavior. Let’s look at a real-world example:
const userService = { notifyUser: (message) => { // Complex notification logic }};
describe('Notification System', () => { it('should notify user when important action occurs', () => { const notifySpy = jest.spyOn(userService, 'notifyUser');
// Trigger some action performImportantAction();
expect(notifySpy).toHaveBeenCalledWith('Action completed successfully'); });});
Mastering Stubs
Sometimes you want to control the behavior of your dependencies completely. That’s where stubs shine:
const fetchUserData = jest.fn().mockImplementation(() => { return Promise.resolve({ id: 1, name: 'Test User', role: 'admin' });});
describe('User Management', () => { it('should handle user data correctly', async () => { const user = await fetchUserData(); expect(user.role).toBe('admin'); });});
Creating Sophisticated Mocks
For complex scenarios, you might need to create comprehensive mocks that simulate entire modules or classes:
jest.mock('./databaseService', () => { return { connect: jest.fn(), query: jest.fn().mockImplementation((queryString) => { if (queryString.includes('SELECT')) { return Promise.resolve([{ id: 1, data: 'test' }]); } return Promise.resolve({ affected: 1 }); }), disconnect: jest.fn() };});
Best Practices and Common Pitfalls
- Always remember to clear mocks between tests using
jest.clearAllMocks()
- Use
jest.spyOn()
when you want to restore original behavior later - Be careful with mock implementations that might cause memory leaks
- Consider using
jest.isolateModules()
for tests that need completely isolated module states
Advanced Techniques
Here’s a powerful technique for testing error conditions:
const mockFn = jest.fn() .mockImplementationOnce(() => Promise.resolve('success')) .mockImplementationOnce(() => Promise.reject(new Error('fail')));
describe('Error Handling', () => { it('should handle success and failure scenarios', async () => { expect(await mockFn()).toBe('success'); // First call await expect(mockFn()).rejects.toThrow('fail'); // Second call });});
Conclusion
Mastering these advanced mocking techniques will make your tests more robust and maintainable. Remember, the goal is to create tests that are both thorough and readable.
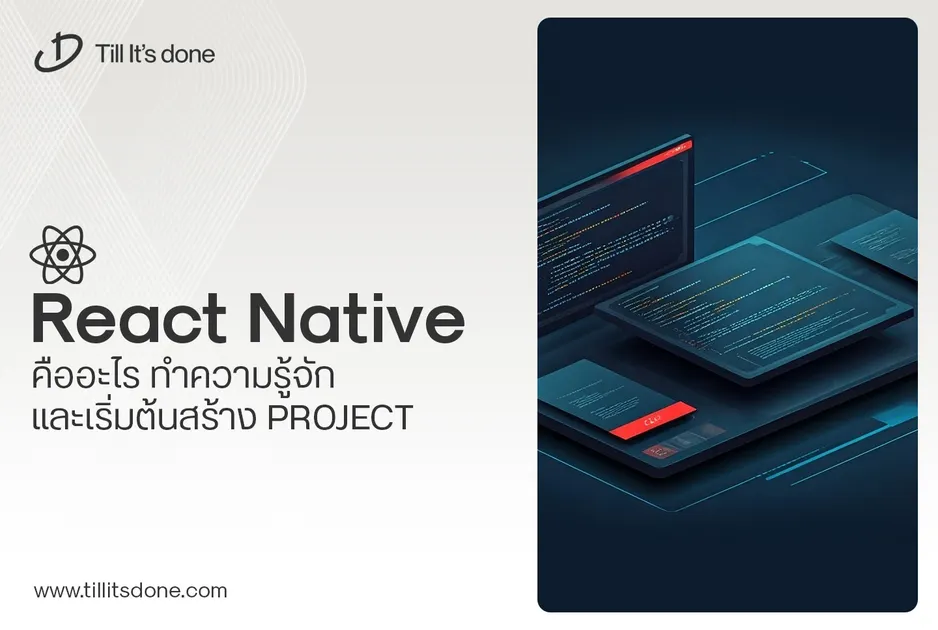
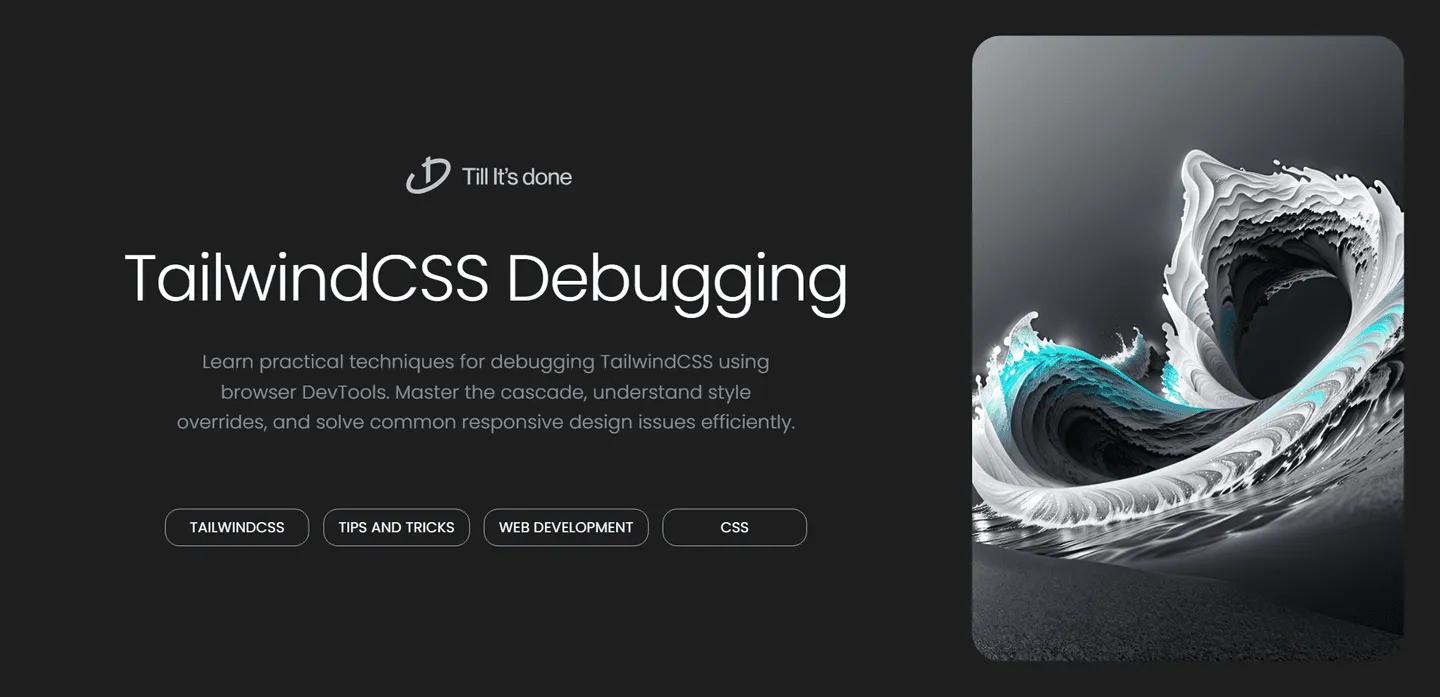
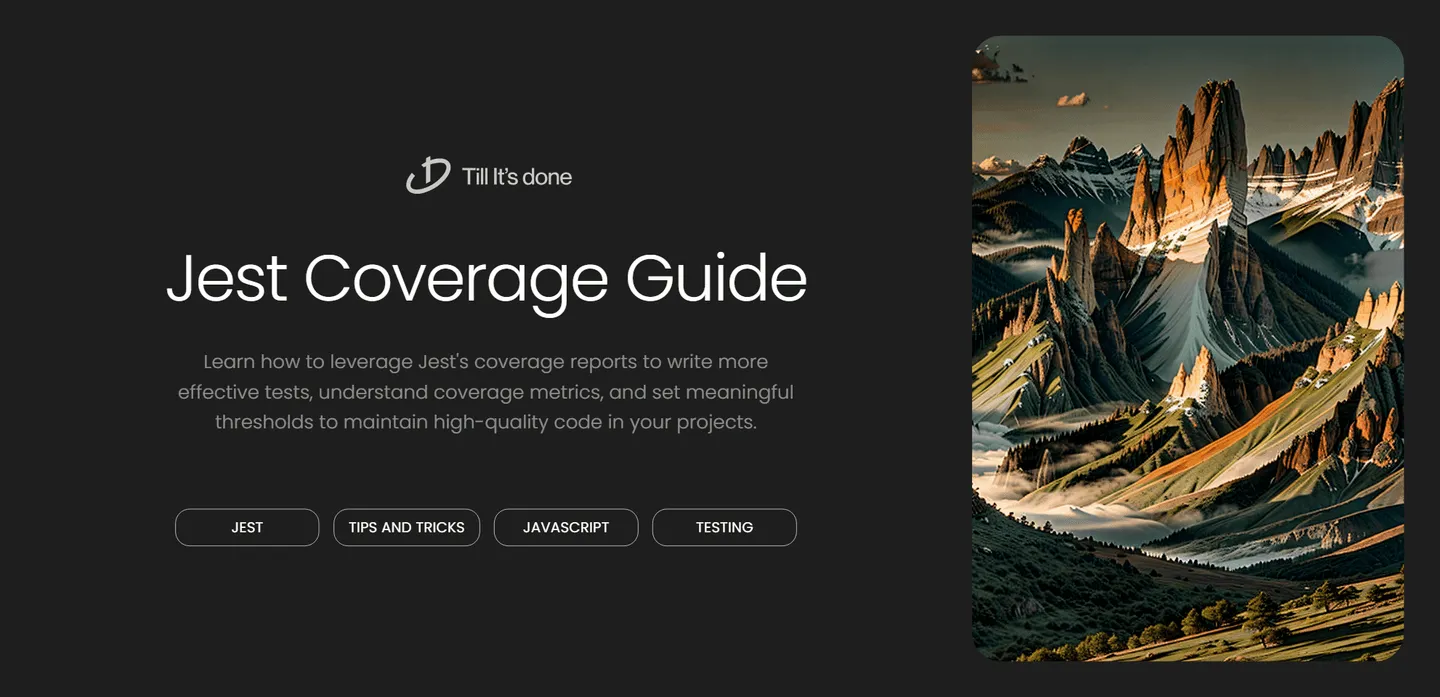
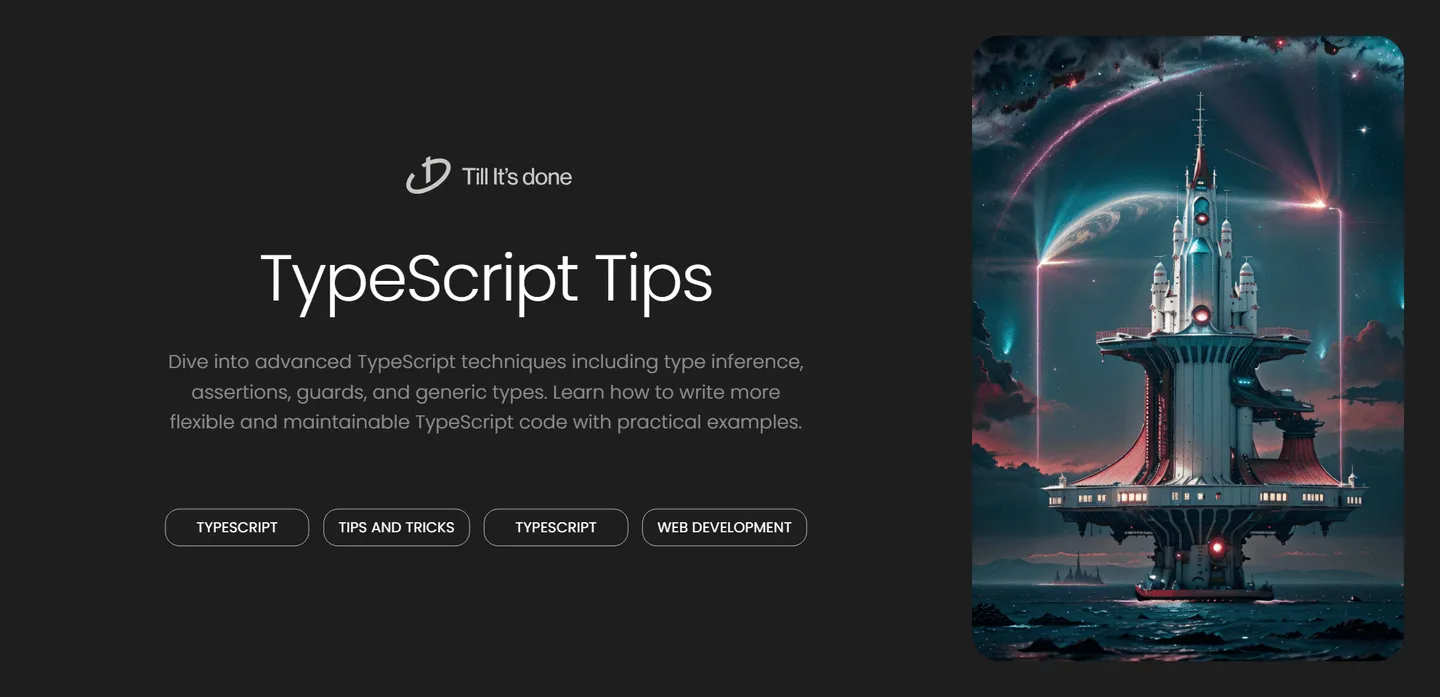
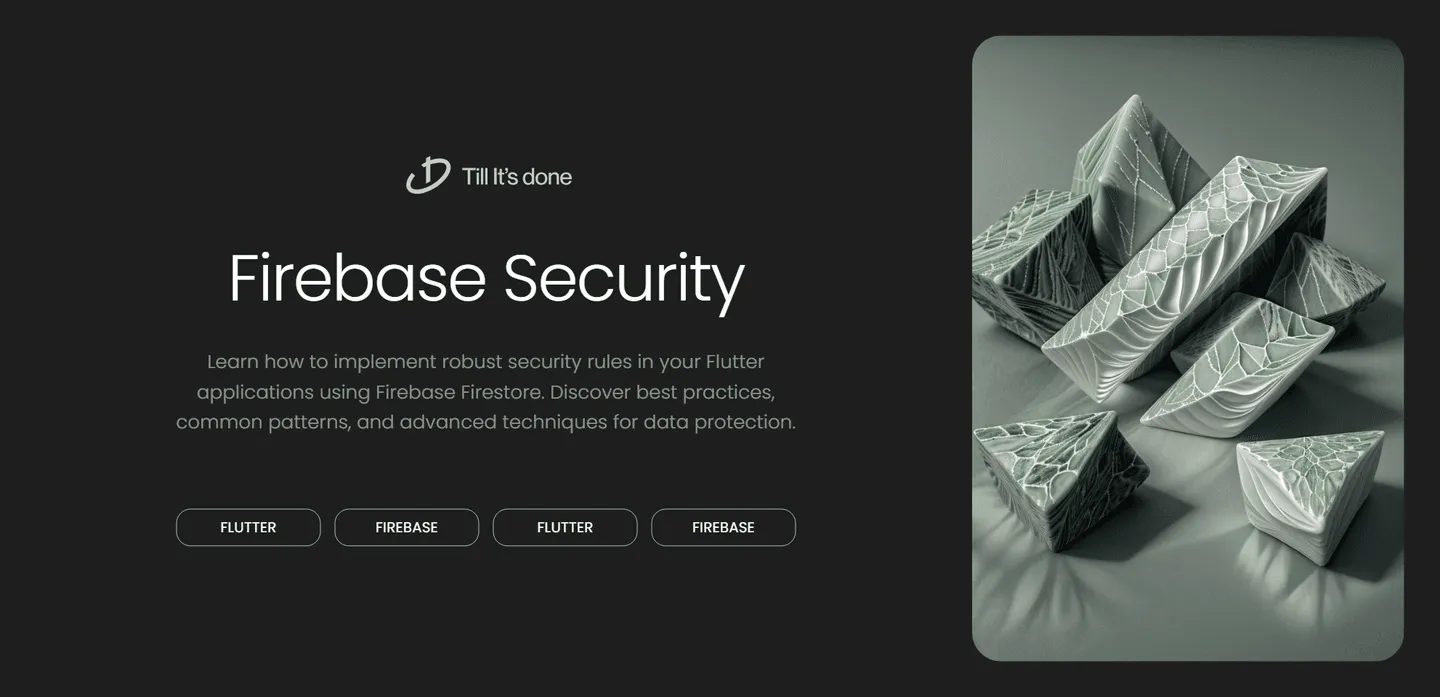
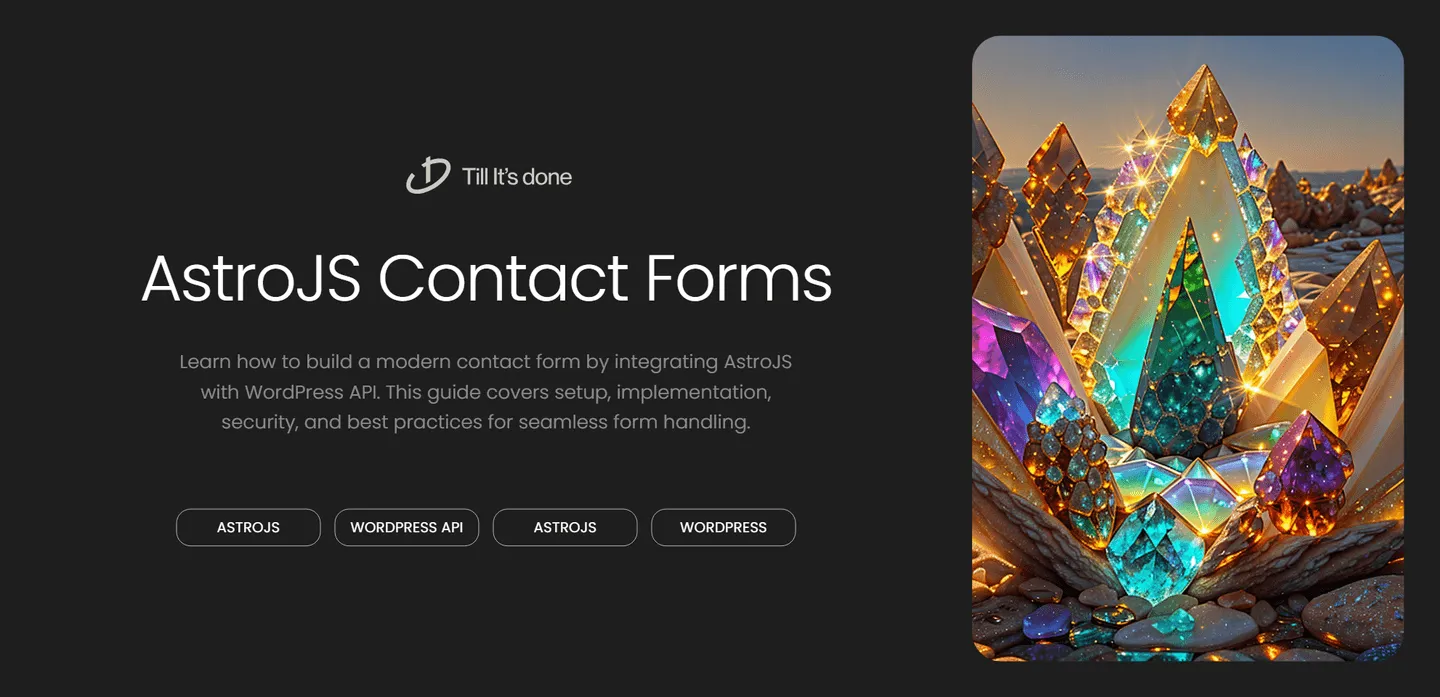
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.