- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Validating API Responses with Zod in Astro.js
Master type-safe data handling, prevent runtime errors, and build more reliable web applications.

In the world of modern web development, handling API responses safely isn’t just a best practice—it’s essential. Today, we’ll explore how to leverage Zod’s powerful validation capabilities in your Astro.js applications to ensure your data is exactly what you expect it to be.
Let’s dive into an elegant solution that will help you sleep better at night, knowing your application won’t break due to unexpected API responses.
Why Zod for API Validation?
When fetching data from external APIs, we often trust that the response will match our expectations. However, in the real world, APIs can change, return unexpected data, or even fail in ways we didn’t anticipate. This is where Zod shines, offering a robust solution for runtime type validation.
Setting Up Zod in Your Astro Project
First, let’s add Zod to your Astro.js project. The setup is straightforward:
npm install zod
Creating Your First Schema
Let’s say we’re building a blog platform and fetching post data from an API. Here’s how we can define a schema for our blog posts:
import { z } from 'zod';
const PostSchema = z.object({ id: z.number(), title: z.string(), content: z.string(), publishedAt: z.string().datetime(), author: z.object({ name: z.string(), email: z.string().email() })});
type Post = z.infer<typeof PostSchema>;
Implementing Validation in Astro Routes
Now, let’s put this into practice in an Astro route:
---import { z } from 'zod';
const PostSchema = z.object({ // ... schema definition ...});
async function fetchPost(id: number) { try { const response = await fetch(`https://api.example.com/posts/${id}`); const data = await response.json();
// Validate the response const validatedPost = PostSchema.parse(data); return validatedPost; } catch (error) { if (error instanceof z.ZodError) { console.error('Validation error:', error.errors); return null; } throw error; }}
const post = await fetchPost(1);---
{post ? ( <article> <h1>{post.title}</h1> <p>{post.content}</p> </article>) : ( <p>Post not found or invalid</p>)}
Advanced Validation Techniques
Zod offers more advanced features that can make your validation even more robust:
const ApiResponse = z.object({ data: z.array(PostSchema), metadata: z.object({ totalPages: z.number(), currentPage: z.number(), itemsPerPage: z.number() }).optional(), status: z.enum(['success', 'error'])});
Error Handling and User Feedback
When validation fails, provide meaningful feedback to your users while logging the details for debugging:
try { const validated = ApiResponse.parse(data);} catch (error) { if (error instanceof z.ZodError) { // Log the error details for debugging console.error('Validation failed:', error.errors);
// Show user-friendly message return { status: 400, body: { message: 'Unable to process the data. Please try again later.' } }; }}
Conclusion
By implementing Zod validation in your Astro.js applications, you’re adding a crucial layer of type safety that protects your application from unexpected data structures. This not only prevents runtime errors but also makes your codebase more maintainable and robust.
Remember, the small overhead of setting up proper validation pays off immensely in the long run by catching issues early and providing clear error messages when things go wrong.
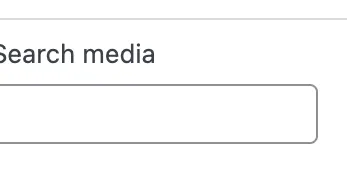





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.