- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Master Data Manipulation with Lodash in Node.js
Discover practical examples of array operations, object handling, and functional programming techniques.
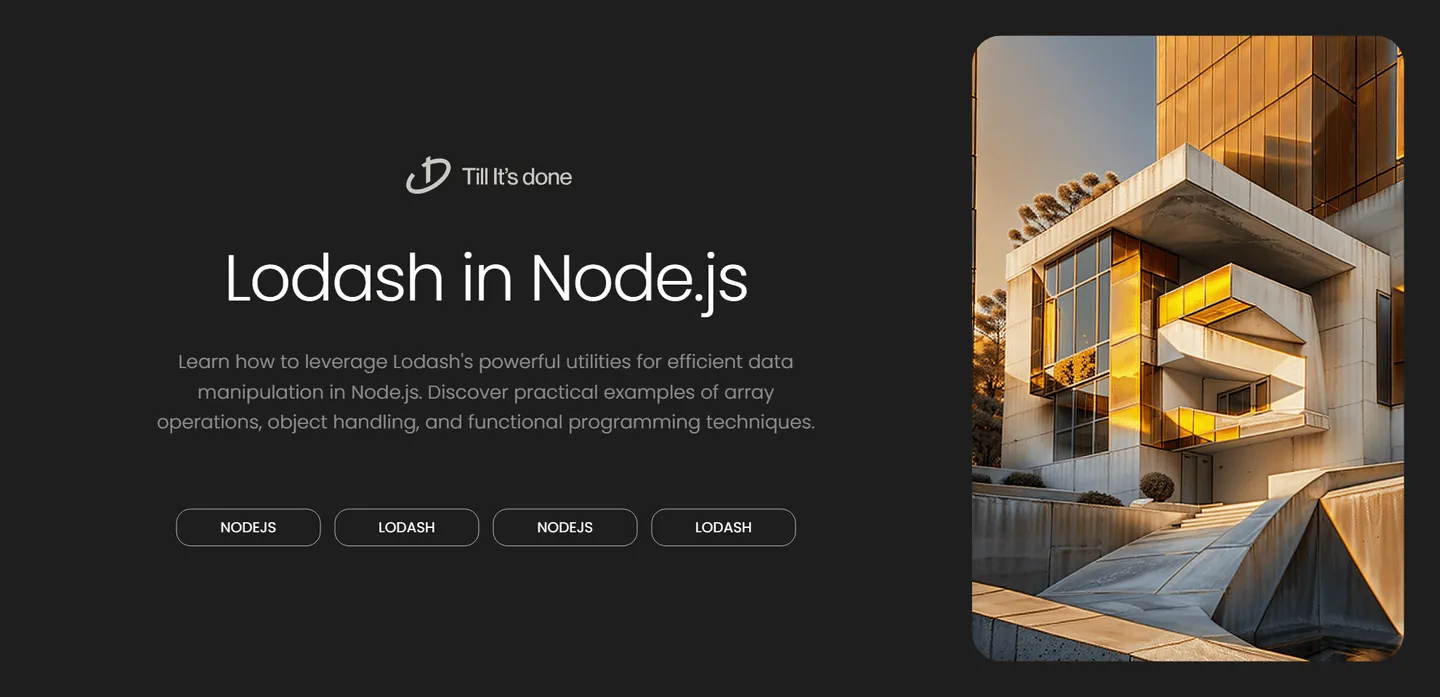
How to Use Lodash for Data Manipulation in Node.js
Have you ever found yourself writing complex data manipulation logic in Node.js and thinking, “There must be a better way”? Well, there is! Enter Lodash – your Swiss Army knife for handling arrays, objects, and more. Let’s dive into how this powerful utility library can make your life easier.
Why Lodash?
JavaScript already gives us plenty of array methods like map, filter, and reduce. So why do we need Lodash? The answer lies in its consistency, performance, and enhanced functionality. Lodash provides a unified API that works seamlessly across different data types and edge cases, making your code more reliable and easier to maintain.
Getting Started with Lodash
First, let’s install Lodash in your Node.js project:
npm install lodash
Then import it in your code:
const _ = require('lodash');
Working with Arrays
Let’s explore some powerful array manipulation techniques:
const users = [ { id: 1, name: 'John', age: 25 }, { id: 2, name: 'Jane', age: 30 }, { id: 3, name: 'Bob', age: 25 }];
// Group users by ageconst groupedByAge = _.groupBy(users, 'age');
// Find unique valuesconst ages = _.uniqBy(users, 'age');
// Chunk array into smaller piecesconst chunks = _.chunk(users, 2);
Object Manipulation Made Easy
Lodash really shines when working with objects:
const user = { name: 'John', address: { street: '123 Main St', city: 'Boston' }};
// Safely get nested propertiesconst city = _.get(user, 'address.city', 'Unknown');
// Create a new object with selected propertiesconst userInfo = _.pick(user, ['name', 'address.city']);
// Deep clone objectsconst userCopy = _.cloneDeep(user);
Chaining Operations
One of Lodash’s most powerful features is method chaining:
const result = _([1, 2, 3, 4, 5]) .map(n => n * 2) .filter(n => n > 5) .sum() .value();
Best Practices
- Import only what you need using individual functions:
const { get, map, filter } = require('lodash');
- Use Lodash alternatives when native methods are sufficient
- Leverage method chaining for complex operations
- Consider using Lodash’s FP (Functional Programming) module for pure functions
Conclusion
Lodash is an invaluable tool in any Node.js developer’s arsenal. It simplifies complex data manipulation tasks and helps you write cleaner, more maintainable code. Start incorporating these utilities into your projects, and you’ll wonder how you ever lived without them!
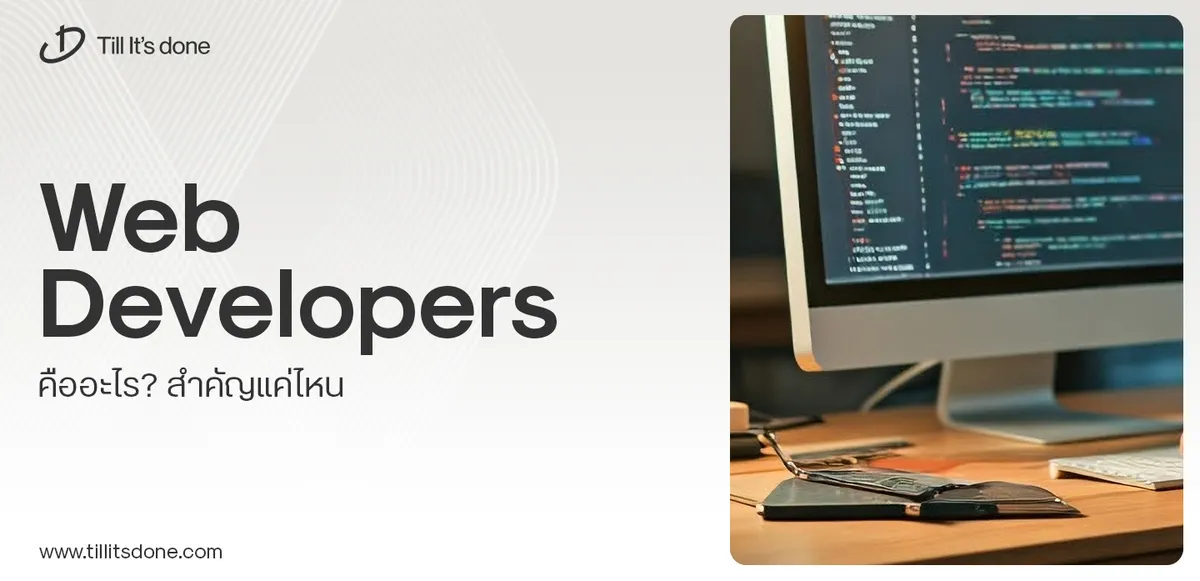
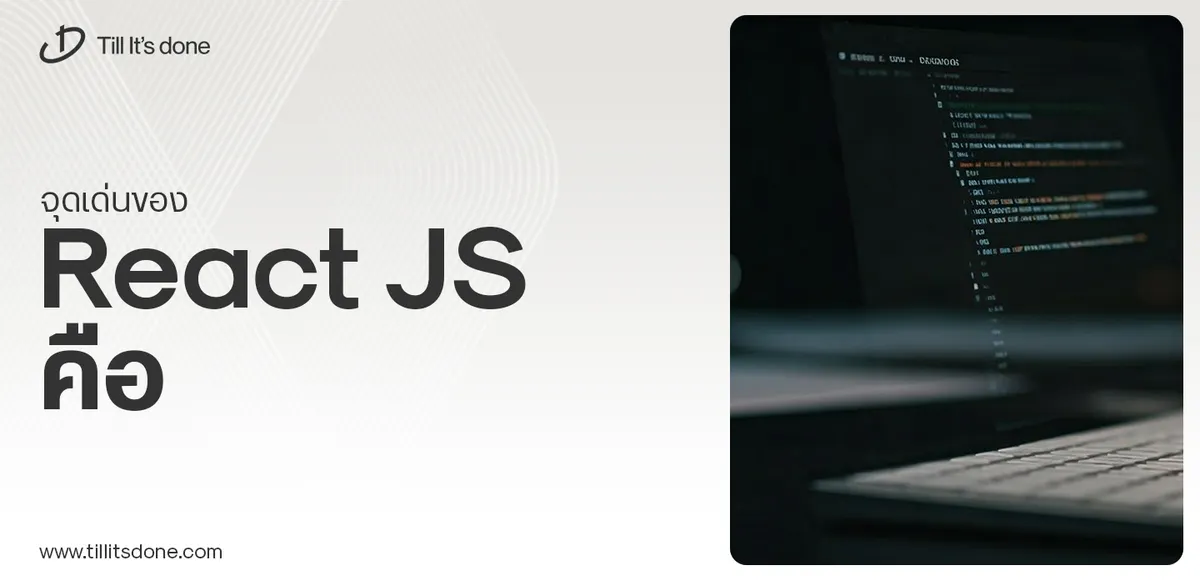
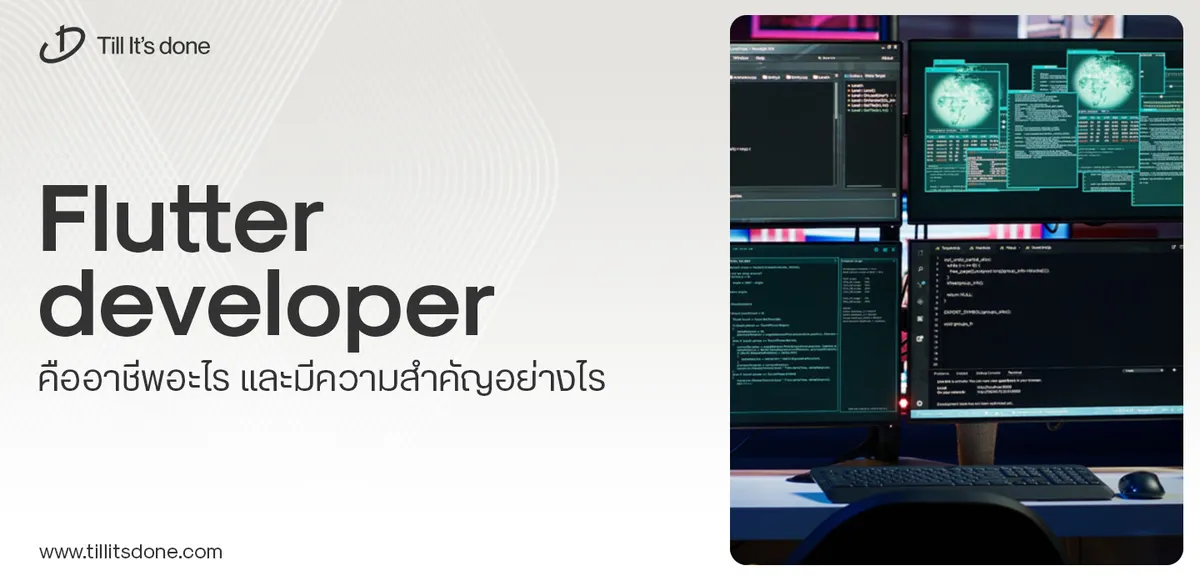
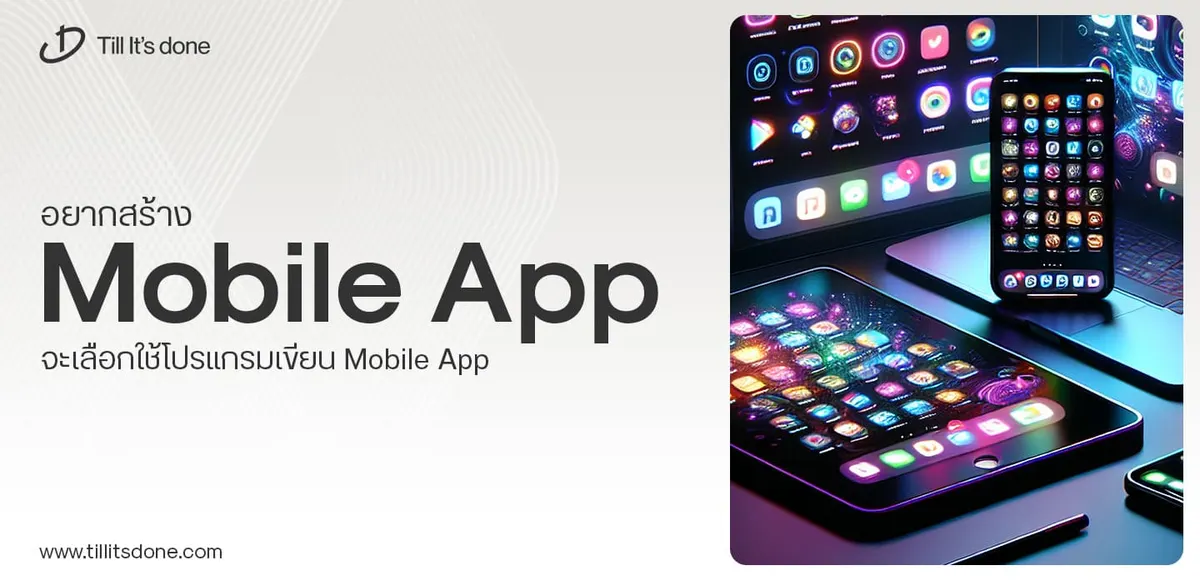
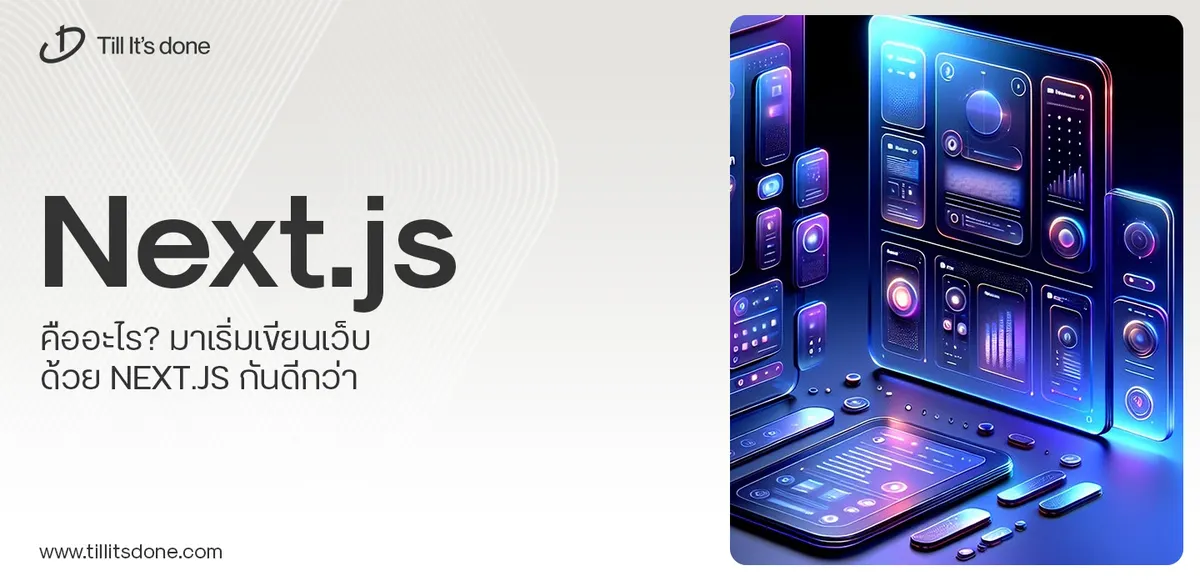
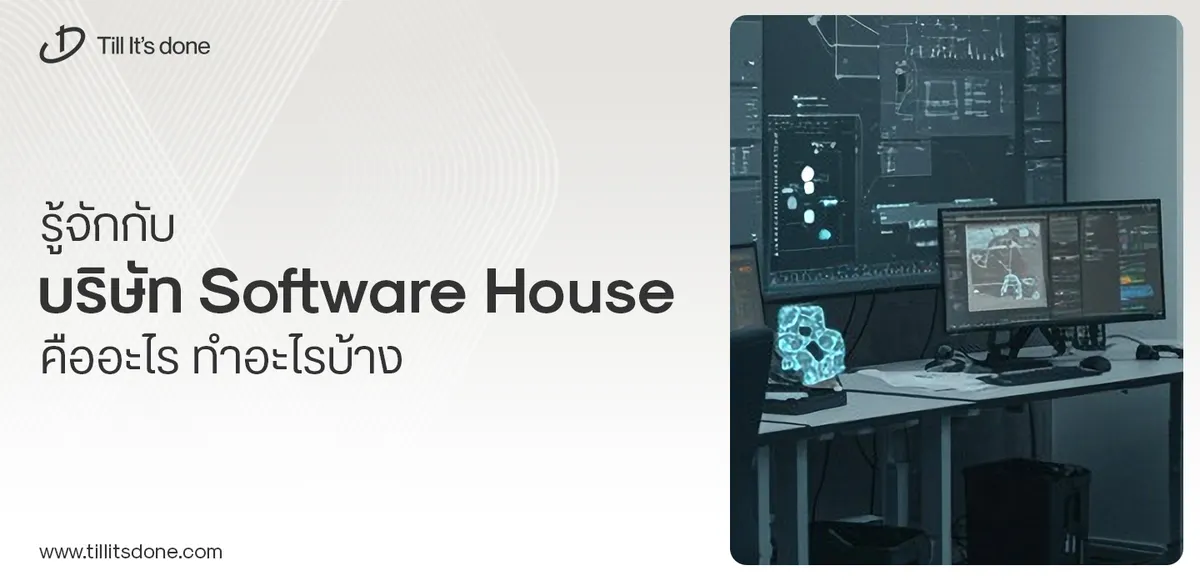
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.