- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Testing Your Gin Application: Complete Guide
From basic HTTP testing to advanced middleware validation, master the art of testing your Gin web services.
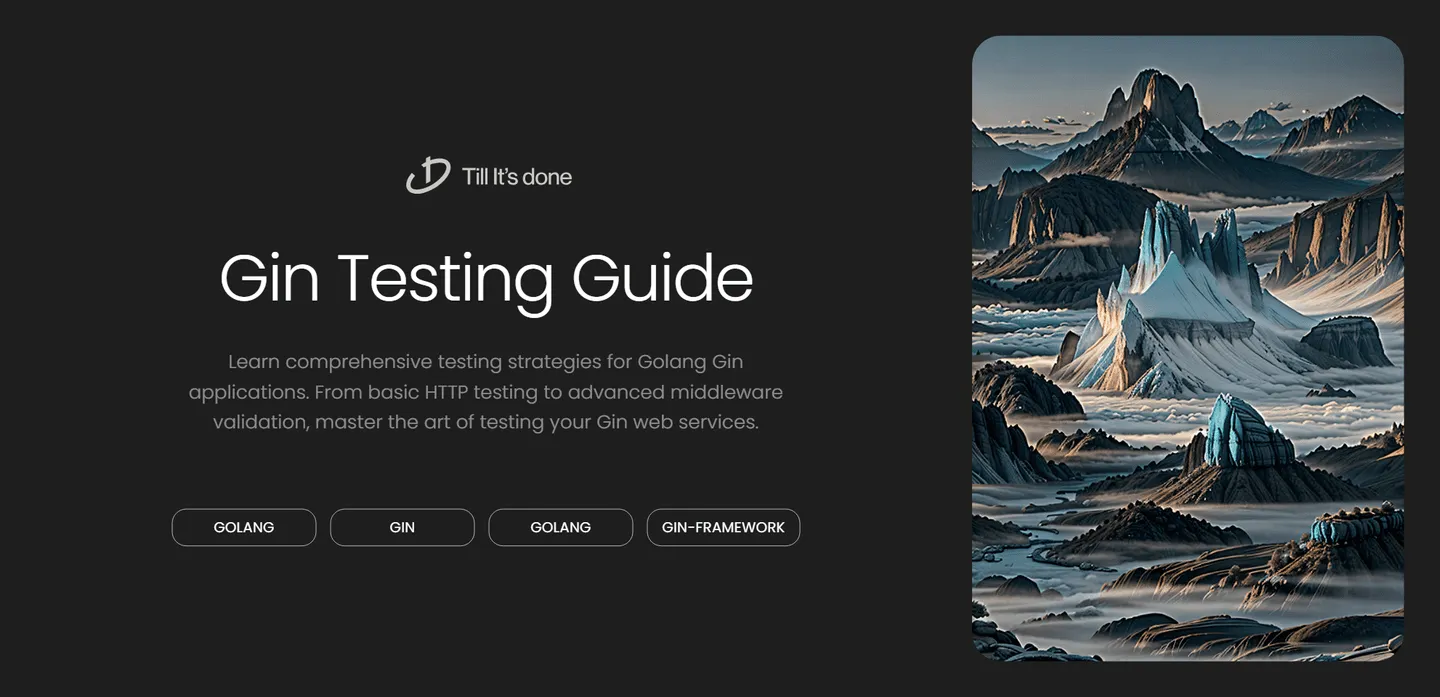
Testing Your Gin Application: A Complete Guide
Testing is a crucial aspect of building reliable Go applications with the Gin framework. Whether you’re developing a small API or a complex web service, proper testing ensures your application behaves correctly and remains maintainable. In this comprehensive guide, we’ll explore various testing approaches and best practices for Gin applications.
Understanding Gin’s Testing Utilities
Gin provides built-in utilities that make testing your HTTP handlers straightforward and efficient. The framework includes a test recorder and methods to simulate HTTP requests, allowing you to verify your endpoints’ behavior without starting a real server.
Setting Up Your Testing Environment
Before diving into testing, ensure your project has a proper testing structure. Create test files with the _test.go
suffix alongside your handler files. This convention makes it easy to locate and maintain your tests.
Writing Your First Test
Let’s start with a basic example of testing a GET endpoint. We’ll walk through creating a test case, setting up the router, and validating the response.
func TestGetUserHandler(t *testing.T) { // Set up the router router := gin.Default() router.GET("/user/:id", GetUser)
// Create a test request w := httptest.NewRecorder() req, _ := http.NewRequest("GET", "/user/1", nil) router.ServeHTTP(w, req)
// Assert the response assert.Equal(t, 200, w.Code)}
Testing Different HTTP Methods
Your application likely handles various HTTP methods. Let’s explore how to test POST, PUT, and DELETE endpoints effectively.
Testing POST Requests with JSON Payload
When testing endpoints that expect JSON data, you’ll need to properly format your test requests:
func TestCreateUserHandler(t *testing.T) { router := gin.Default() router.POST("/users", CreateUser)
payload := `{"name": "John Doe", "email": "john@example.com"}` req, _ := http.NewRequest("POST", "/users", strings.NewReader(payload)) req.Header.Set("Content-Type", "application/json")}
Advanced Testing Techniques
Middleware Testing
Testing middleware is crucial as it often handles important cross-cutting concerns like authentication and logging:
func TestAuthMiddleware(t *testing.T) { router := gin.Default() router.Use(AuthMiddleware()) router.GET("/protected", ProtectedHandler)
// Test without auth token w := httptest.NewRecorder() req, _ := http.NewRequest("GET", "/protected", nil) router.ServeHTTP(w, req) assert.Equal(t, 401, w.Code)}
Testing Response Content
Don’t just test status codes – verify the response body, headers, and any other relevant data:
func TestUserResponseContent(t *testing.T) { // ... setup code ...
var response map[string]interface{} json.Unmarshal(w.Body.Bytes(), &response)
assert.Equal(t, "John Doe", response["name"]) assert.Equal(t, "john@example.com", response["email"])}
Best Practices and Common Pitfalls
- Always clean up test data after your tests run
- Use table-driven tests for testing multiple scenarios
- Mock external dependencies like databases and APIs
- Keep your tests focused and follow the Single Responsibility Principle
- Use meaningful test names that describe the scenario being tested
Conclusion
Testing is an investment in your application’s quality and maintainability. By following these practices and examples, you can build a comprehensive test suite that gives you confidence in your Gin application’s reliability.
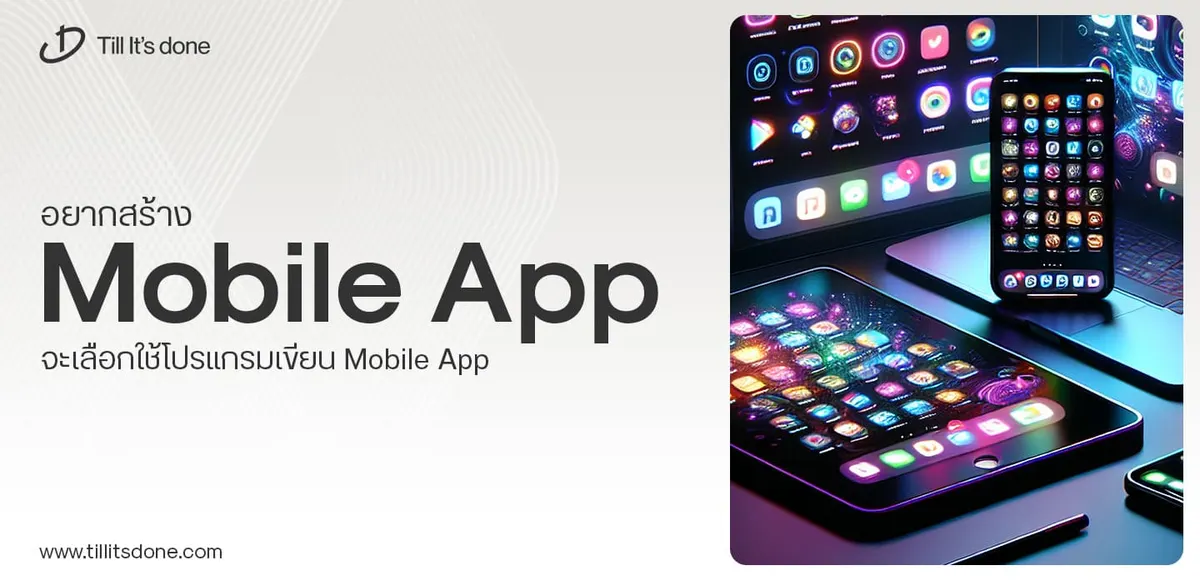
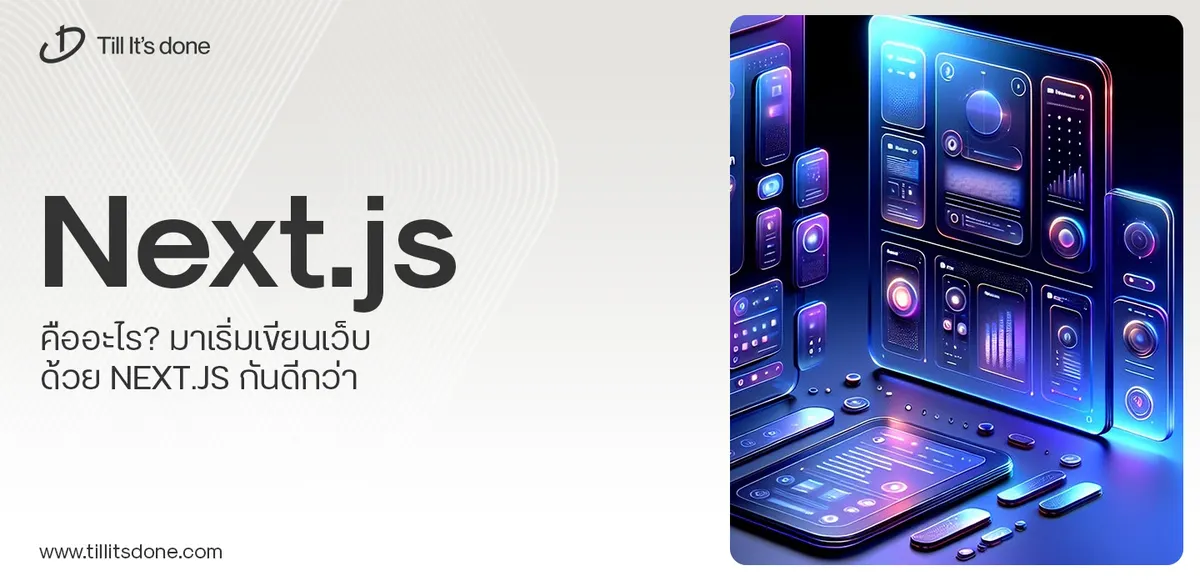
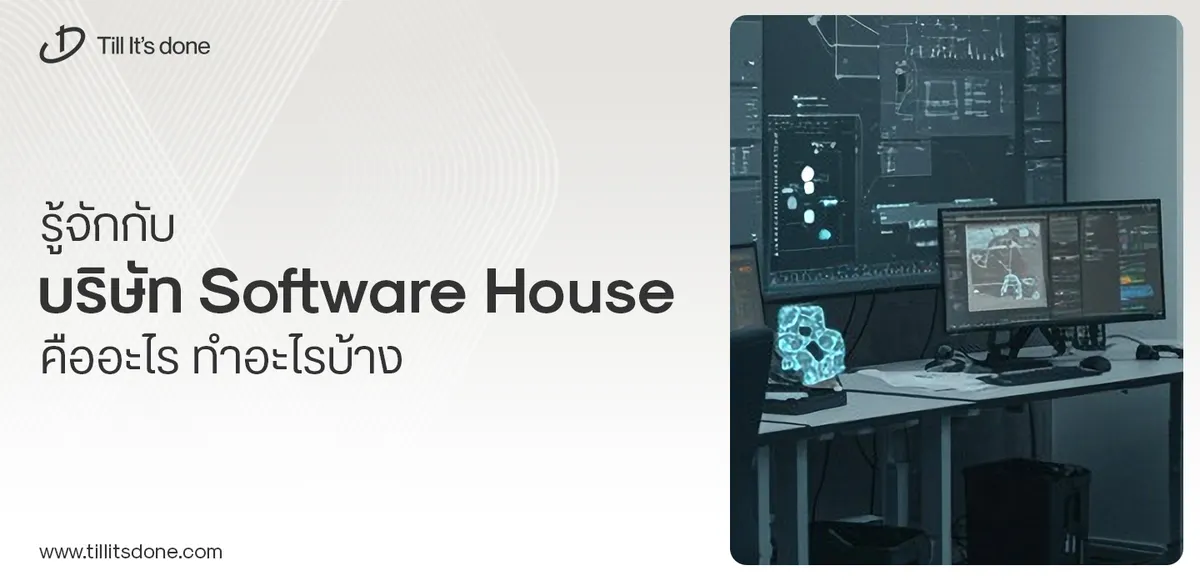
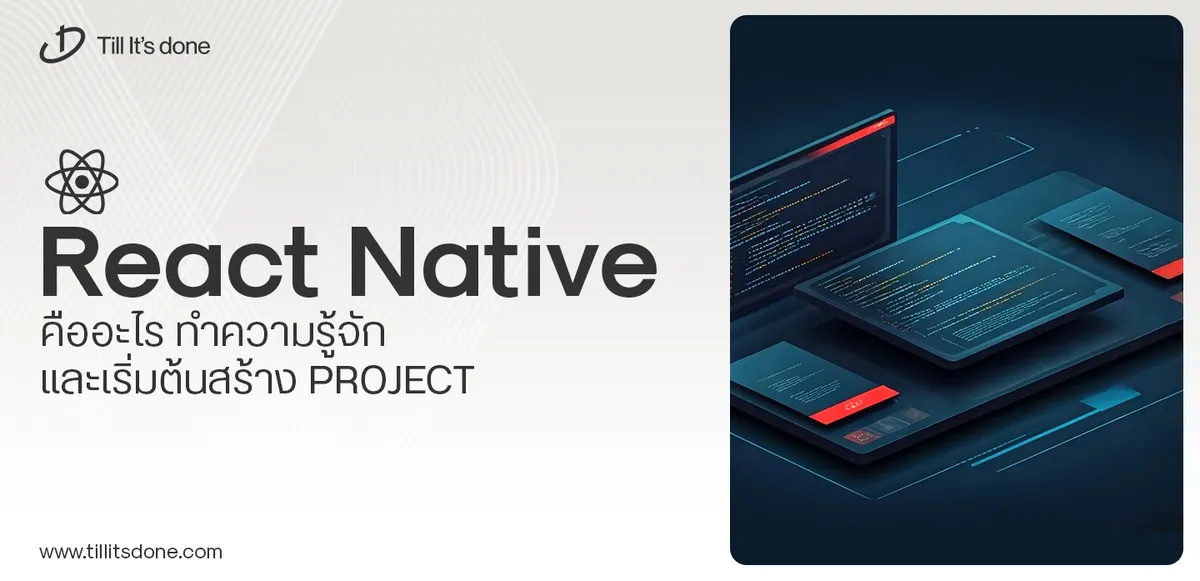
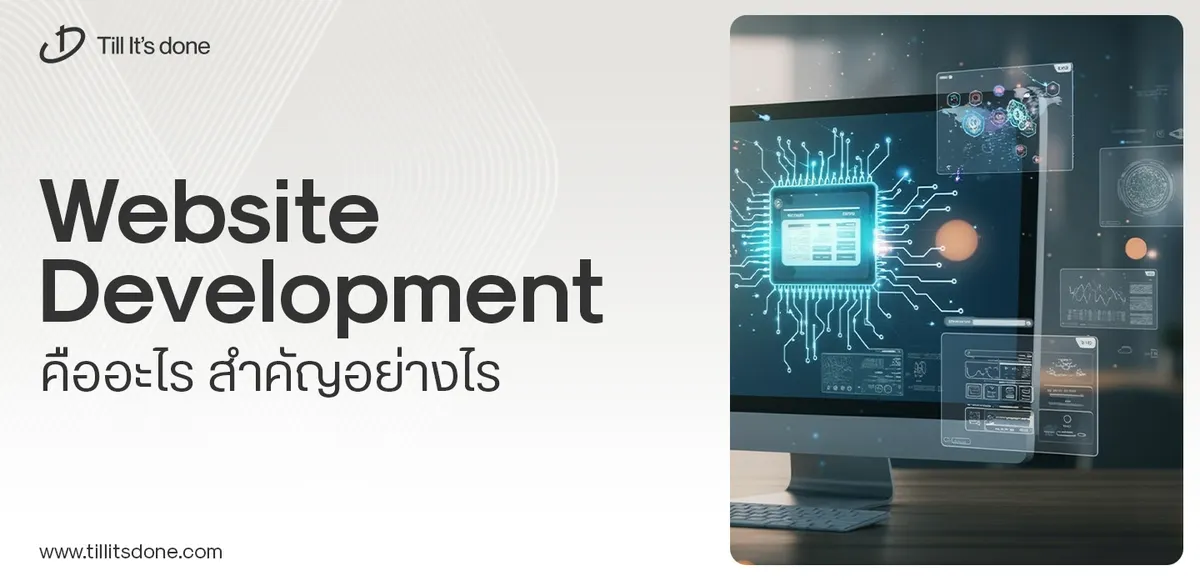
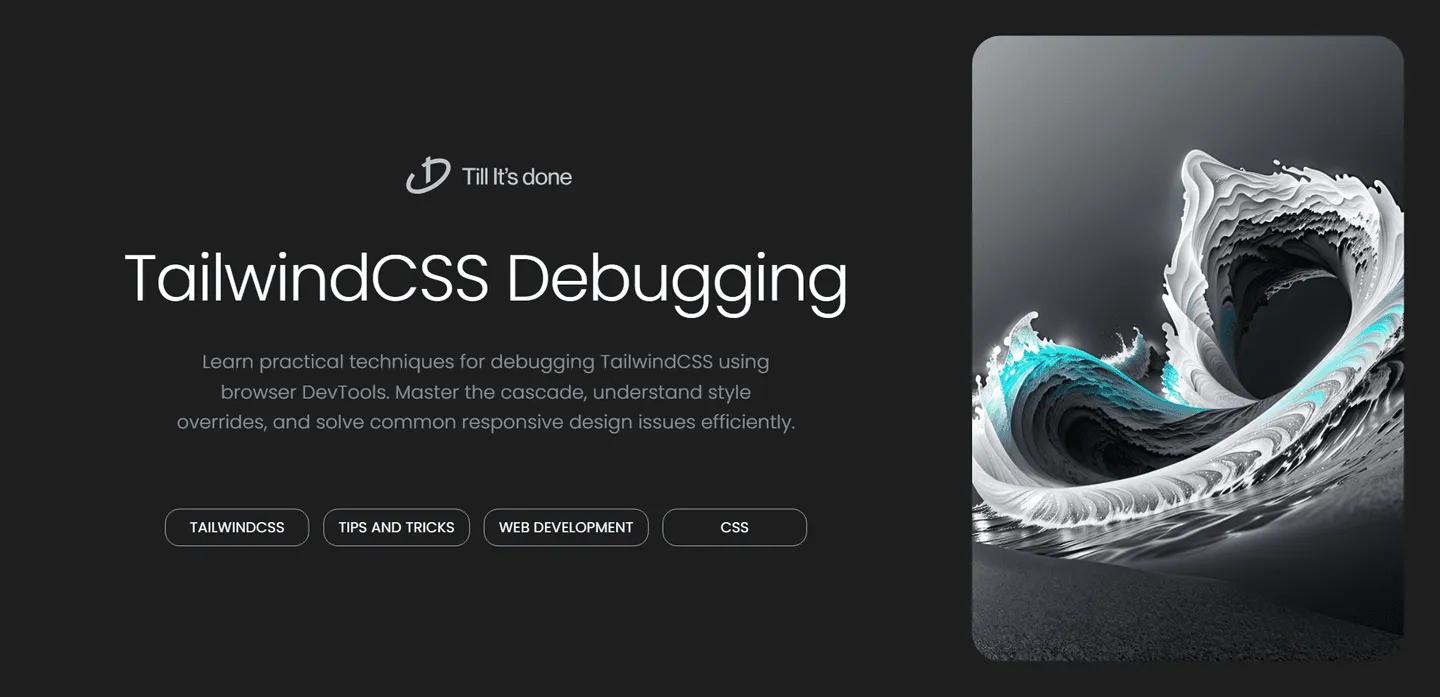
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.