- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using Axios Interceptors in Node.js Apps
Discover practical examples of authentication, error handling, and data transformation.
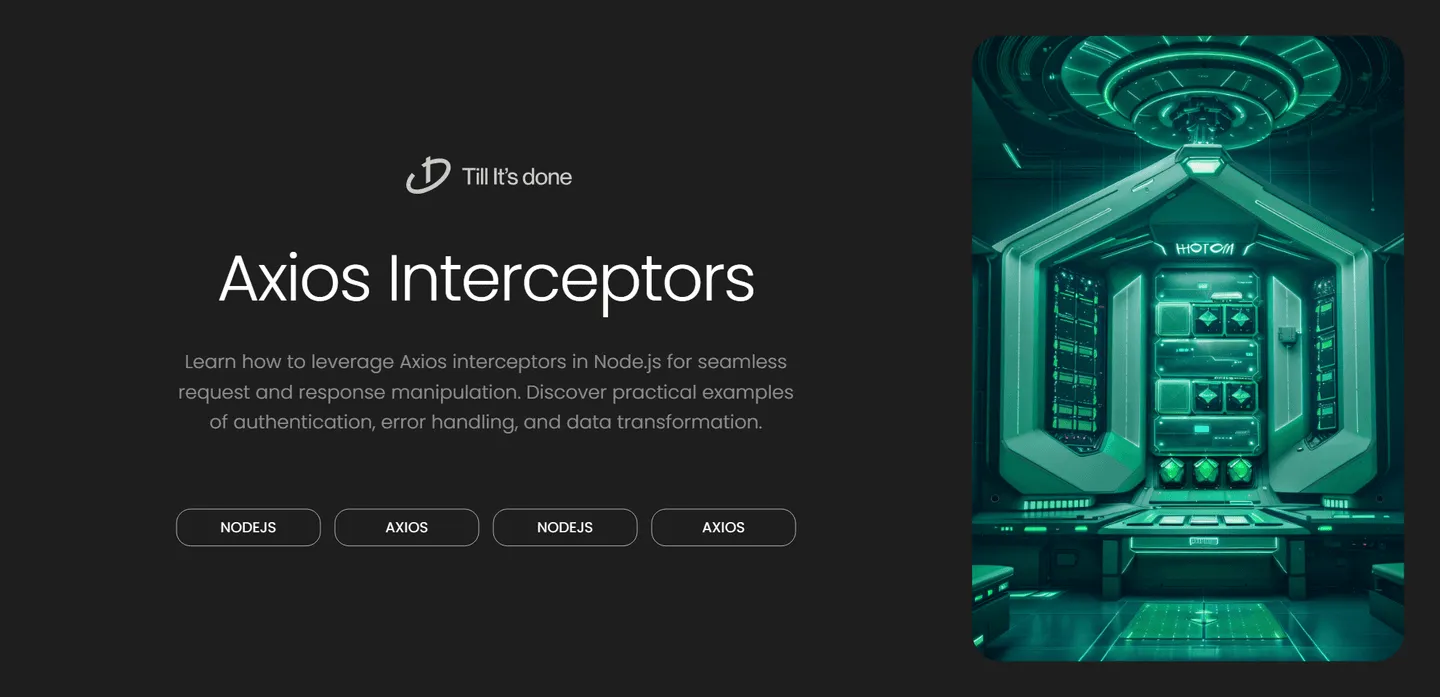
Using Axios Interceptors for Request and Response Manipulation in Node.js
Ever wondered how to globally handle API requests and responses in your Node.js application? Let me introduce you to one of the most powerful features of Axios - Interceptors. They’re like magical gatekeepers that can inspect, modify, or even reject requests and responses before they reach their destination.
What Are Axios Interceptors?
Think of interceptors as middleware for your HTTP requests. They sit between your application and the server, allowing you to perform actions before a request is sent or after a response is received. It’s like having a security checkpoint that can check, stamp, or modify your “HTTP passport” before letting it through.
Setting Up Request Interceptors
Let’s dive into how we can set up request interceptors. Here’s a practical example:
// Create an Axios instanceconst axios = require('axios');const api = axios.create({ baseURL: 'https://api.example.com'});
// Add a request interceptorapi.interceptors.request.use( (config) => { // Add authentication header config.headers.Authorization = `Bearer ${getToken()}`;
// Add timestamp to requests config.headers['Request-Time'] = new Date().toISOString();
return config; }, (error) => { return Promise.reject(error); });
This interceptor automatically adds an authentication token and timestamp to every request. Pretty neat, right?
Response Interceptors in Action
Response interceptors are equally powerful. They can transform data, handle errors globally, or even retry failed requests:
api.interceptors.response.use( (response) => { // Transform response data if (response.data.items) { response.data.items = response.data.items.map(item => ({ ...item, processed: true })); }
return response; }, async (error) => { if (error.response.status === 401) { // Refresh token and retry request await refreshToken(); return api(error.config); } return Promise.reject(error); });
Real-World Use Cases
Interceptors shine in many scenarios:
- Automatically handling authentication
- Logging requests and responses
- Adding correlation IDs for request tracking
- Transforming request/response data formats
- Implementing retry mechanisms
- Showing/hiding global loading indicators
Best Practices
Remember these golden rules when working with interceptors:
- Keep them focused and lightweight
- Handle errors properly
- Maintain clear separation of concerns
- Document any transformations
- Be mindful of the order of multiple interceptors
Error Handling Tips
Always implement proper error handling in your interceptors. Here’s a robust approach:
api.interceptors.response.use( response => response, error => { if (!error.response) { // Network or connection error console.error('Network Error:', error.message); return Promise.reject(new Error('Network error occurred')); }
switch (error.response.status) { case 401: // Handle unauthorized return handleUnauthorized(error); case 404: // Handle not found return Promise.reject(new Error('Resource not found')); default: // Handle other errors return Promise.reject(error); } });
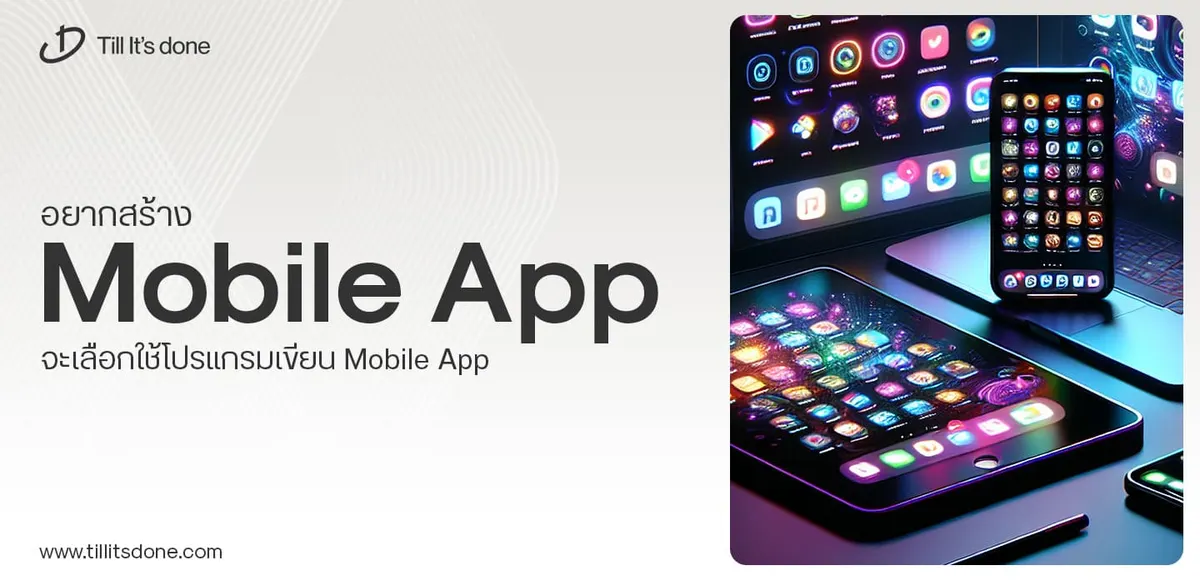
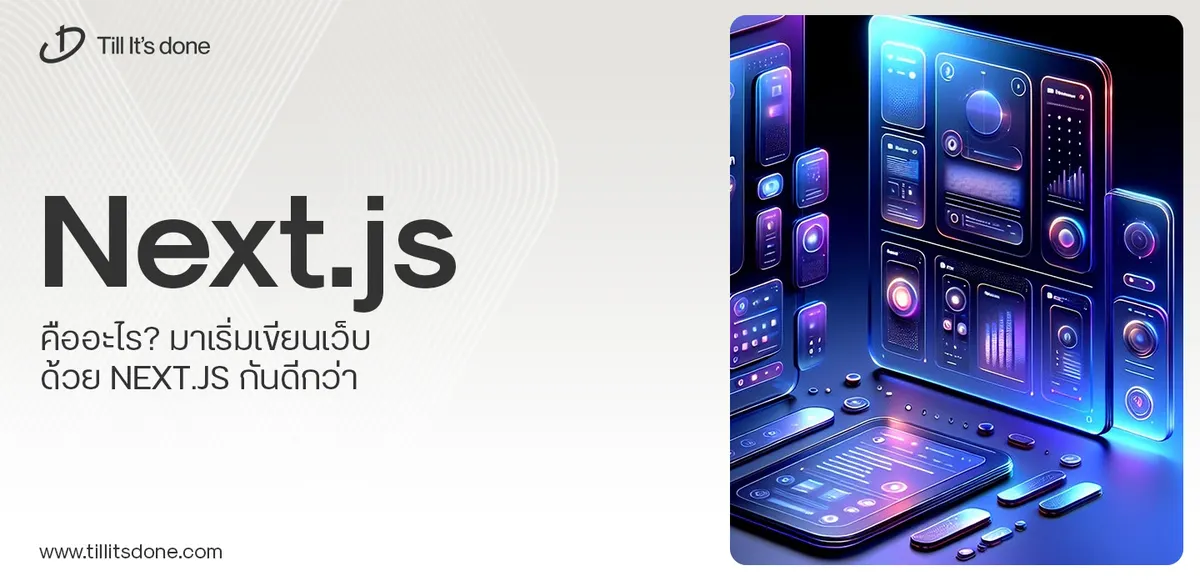
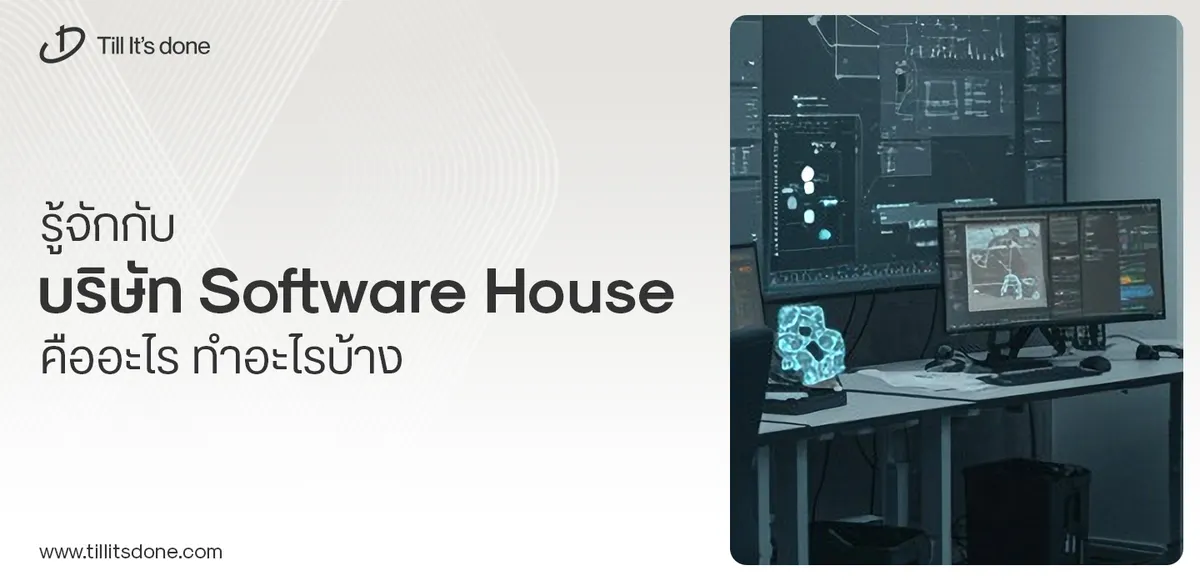
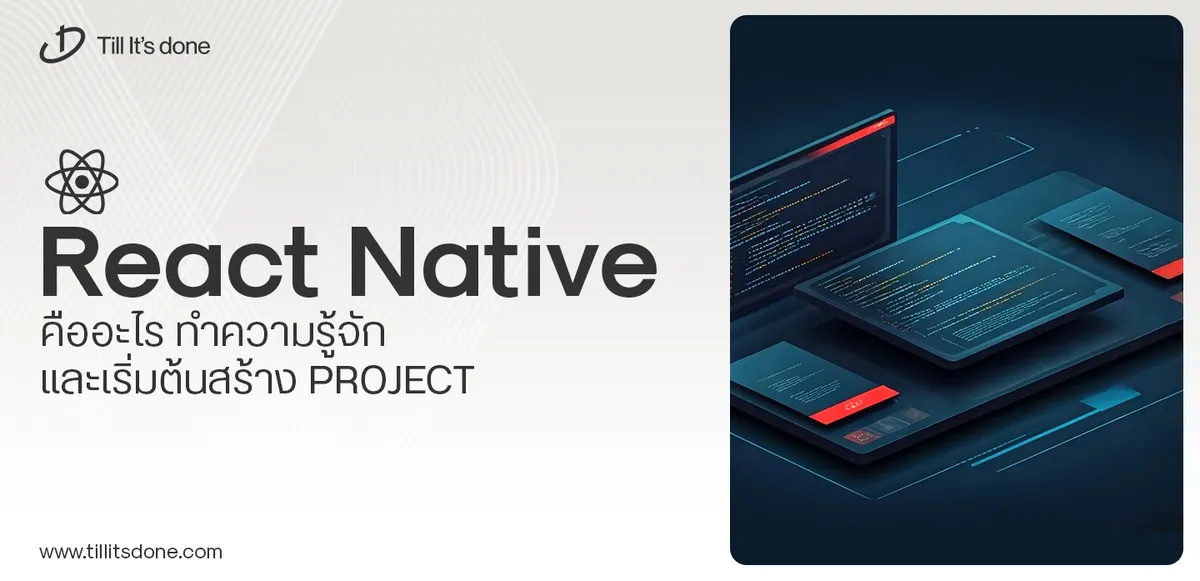
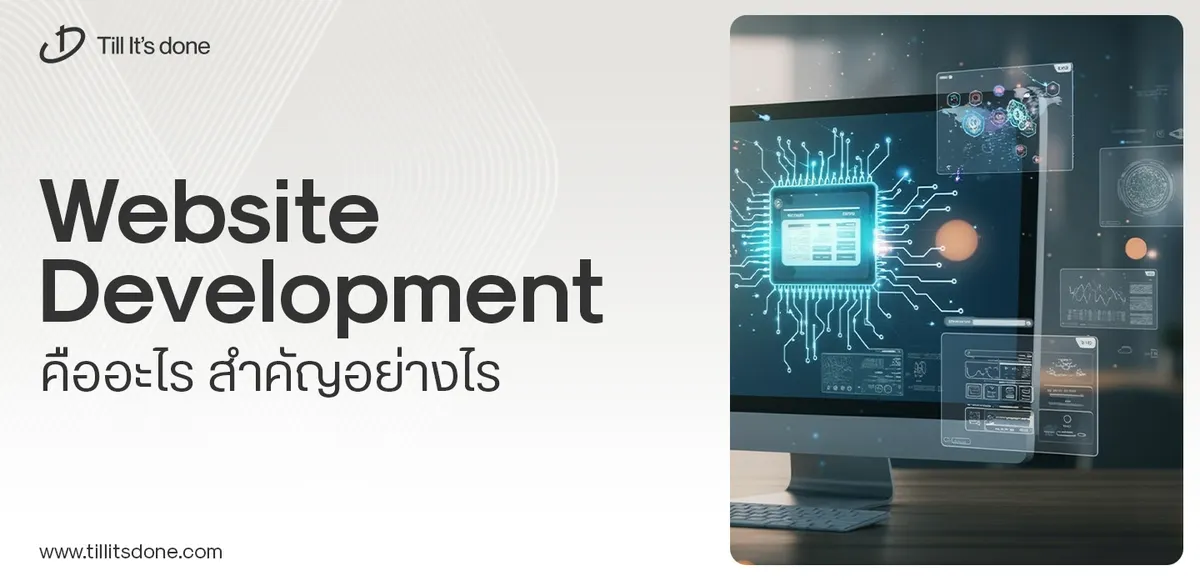
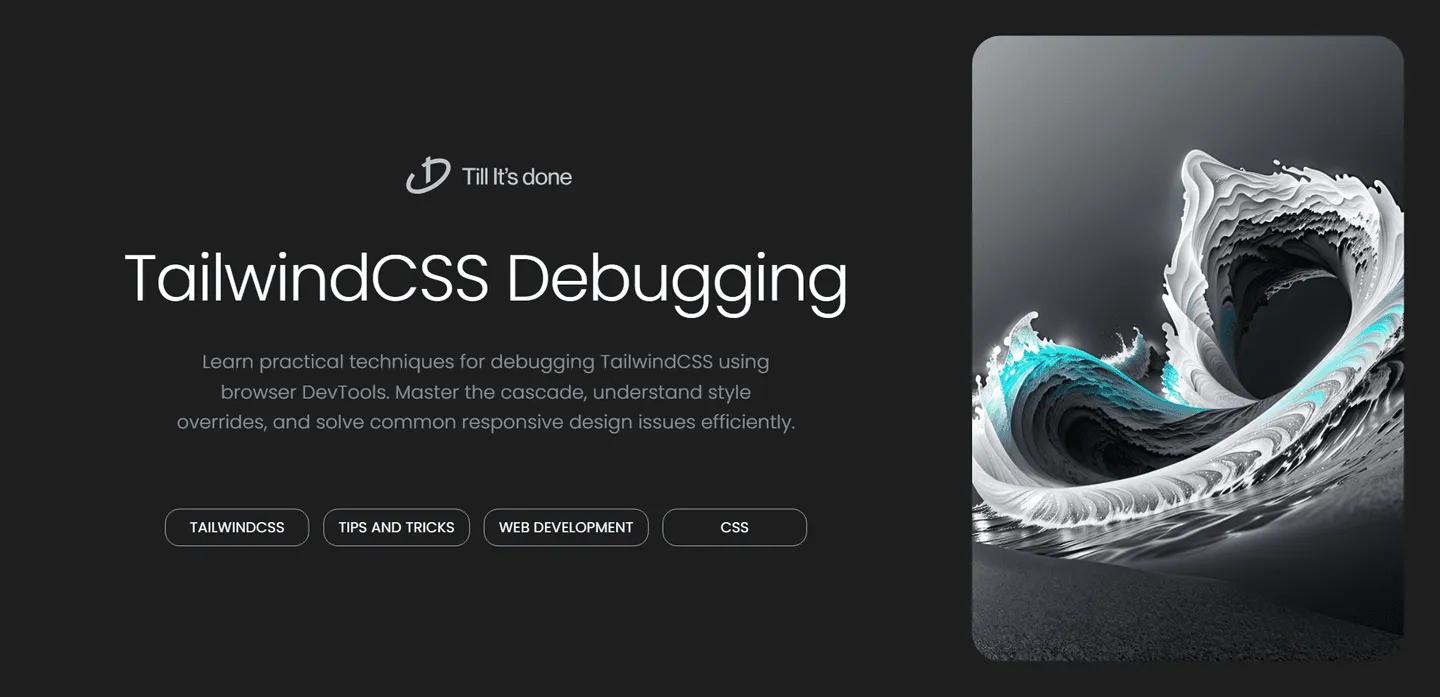
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.