- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Best Practices for Working with Axios in Node.js
Improve your HTTP client implementation today.

As a Node.js developer, making HTTP requests is a fundamental part of building modern applications. Axios has emerged as the go-to HTTP client for its elegance and powerful features. Let’s dive into the best practices that will help you write more maintainable and robust code with Axios.
Setting Up Axios Instances
Instead of using Axios directly, create reusable instances with predefined configurations. This approach keeps your code DRY and makes it easier to maintain consistent headers, base URLs, and timeouts across your application.
const axios = require('axios');
const api = axios.create({ baseURL: process.env.API_BASE_URL, timeout: 5000, headers: { 'Content-Type': 'application/json' }});
Error Handling and Interceptors
One of the most crucial aspects of working with HTTP requests is proper error handling. Axios interceptors provide a clean way to handle errors globally and transform responses consistently.
api.interceptors.response.use( response => response, error => { if (error.response?.status === 401) { // Handle authentication errors return refreshTokenAndRetry(error); } return Promise.reject(error); });
Request Cancellation
When building responsive applications, it’s essential to handle scenarios where requests need to be cancelled. Axios provides a built-in mechanism using cancel tokens.
const CancelToken = axios.CancelToken;const source = CancelToken.source();
api.get('/long-running-query', { cancelToken: source.token}).catch(error => { if (axios.isCancel(error)) { console.log('Request cancelled:', error.message); }});
// Cancel the requestsource.cancel('Operation cancelled by user');
Rate Limiting and Retries
Implement rate limiting and retry mechanisms to handle API restrictions and temporary failures gracefully. You can use libraries like axios-retry
or implement your own solution.
Request and Response Transformation
Use transforms to standardize your request data and response handling. This ensures consistent data formatting across your application.
const api = axios.create({ transformRequest: [(data) => { // Transform request data return JSON.stringify(data); }], transformResponse: [(data) => { // Transform response data return JSON.parse(data); }]});
Testing and Mocking
When writing tests, use axios-mock-adapter to simulate API responses. This allows you to test your code without making actual HTTP requests.
const MockAdapter = require('axios-mock-adapter');const mock = new MockAdapter(api);
mock.onGet('/users').reply(200, { users: [{ id: 1, name: 'John' }]});
Remember to always handle promises appropriately using async/await or proper promise chains, and implement proper logging for debugging and monitoring your applications in production.
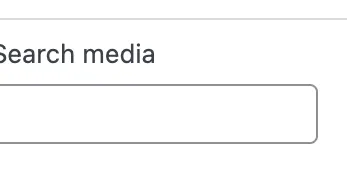





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.