- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using Zod with TypeScript in Node.js for Validation
Discover best practices for schema validation and type safety.
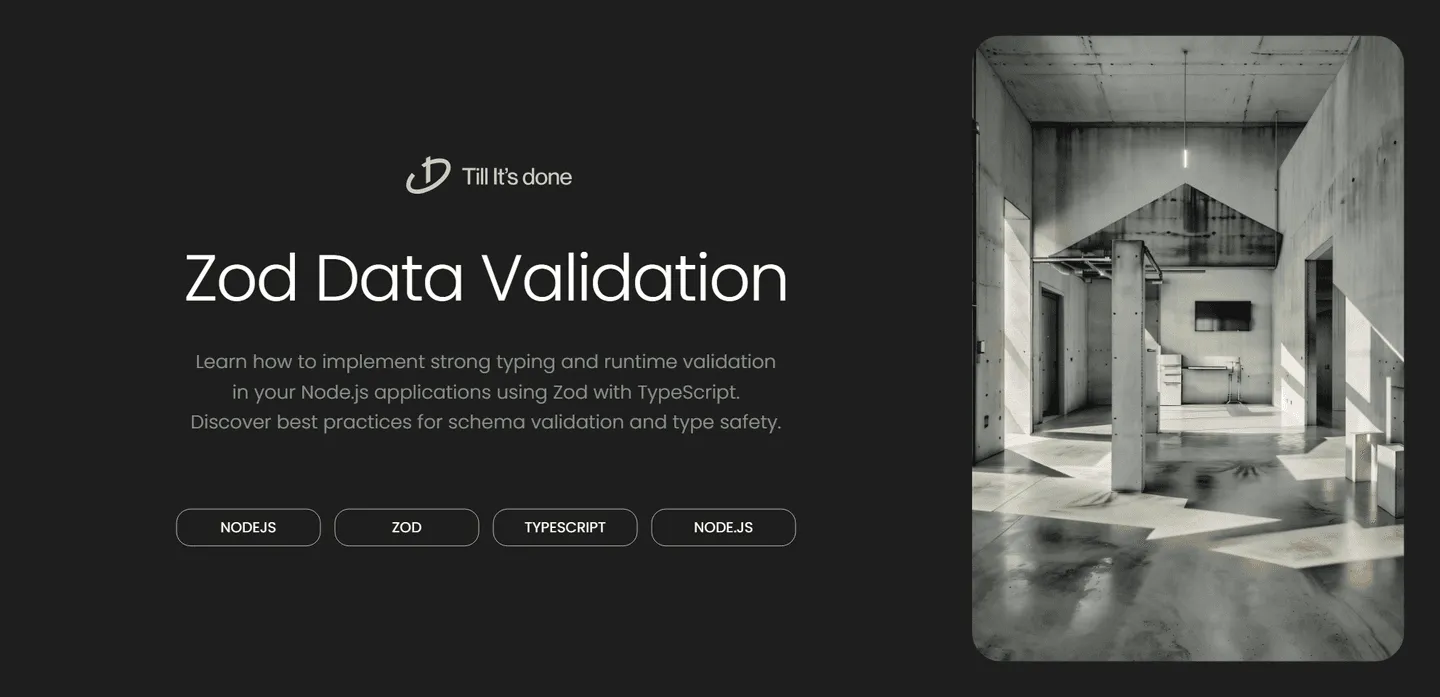
Using Zod with TypeScript in Node.js for Strong Typing and Validation
In the world of Node.js development, ensuring type safety and data validation is crucial for building robust applications. While TypeScript provides excellent static type checking, we often need runtime validation to ensure our data matches our expected schemas. This is where Zod comes in – a powerful schema declaration and validation library that works beautifully with TypeScript.
What Makes Zod Special?
Think of Zod as your data’s bouncer – it checks every piece of information entering your application and ensures it meets your exact specifications. Unlike traditional validation libraries, Zod was built with TypeScript in mind from day one, offering a seamless developer experience with incredible type inference.
Getting Started with Zod
Let’s dive into some practical examples of how to use Zod in your Node.js applications. First, you’ll need to set up your project with TypeScript and install Zod:
npm install zod
Basic Schema Definition
Zod makes it incredibly intuitive to define your data schemas. Here’s how you might define a user schema:
import { z } from 'zod';
const UserSchema = z.object({ name: z.string().min(2), email: z.string().email(), age: z.number().min(18), isActive: z.boolean().default(true)});
type User = z.infer<typeof UserSchema>;
Practical Use Cases
API Request Validation
One of the most common use cases for Zod is validating API requests. Here’s how you might validate an incoming request body:
import express from 'express';import { z } from 'zod';
const app = express();
const CreatePostSchema = z.object({ title: z.string().min(5).max(100), content: z.string().min(10), tags: z.array(z.string()).default([])});
app.post('/posts', (req, res) => { const result = CreatePostSchema.safeParse(req.body);
if (!result.success) { return res.status(400).json({ errors: result.error.format() }); }
// Your validated data is now available in result.data const post = result.data; // ... handle the post creation});
Advanced Features
Zod really shines when you need to handle complex data structures. You can create nested schemas, optional fields, and even conditional validation:
const AddressSchema = z.object({ street: z.string(), city: z.string(), country: z.string(), zipCode: z.string()});
const ExtendedUserSchema = UserSchema.extend({ addresses: z.array(AddressSchema), preferences: z.object({ newsletter: z.boolean(), theme: z.enum(['light', 'dark']).default('light') }).optional()});
Best Practices and Tips
- Always use
safeParse
instead ofparse
when handling user input to avoid throwing exceptions - Leverage Zod’s built-in error formatting for consistent error messages
- Create reusable schemas for common data structures
- Use TypeScript’s type inference with Zod schemas to maintain type safety throughout your application
Conclusion
Zod brings peace of mind to Node.js developers by combining the power of runtime validation with TypeScript’s static typing. It’s like having a strict but fair security system for your data – keeping the bad stuff out while letting the good stuff flow smoothly through your application.

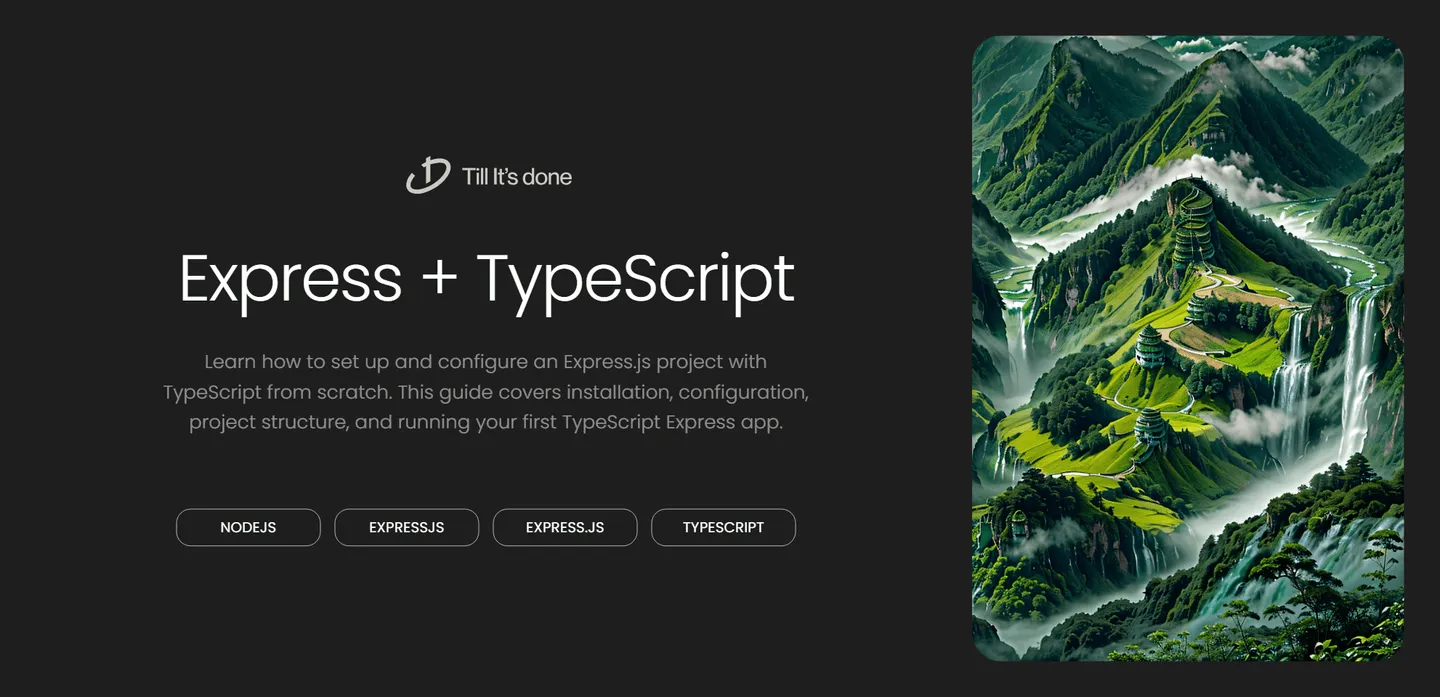
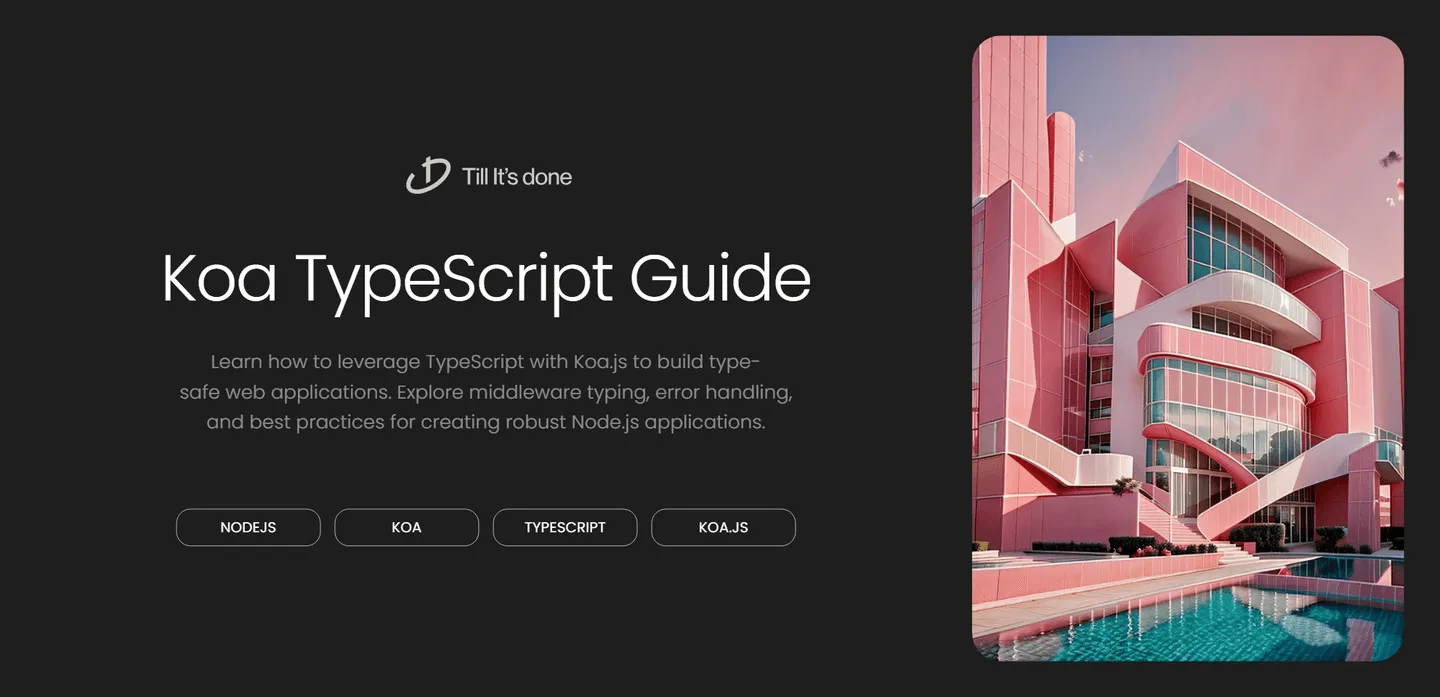

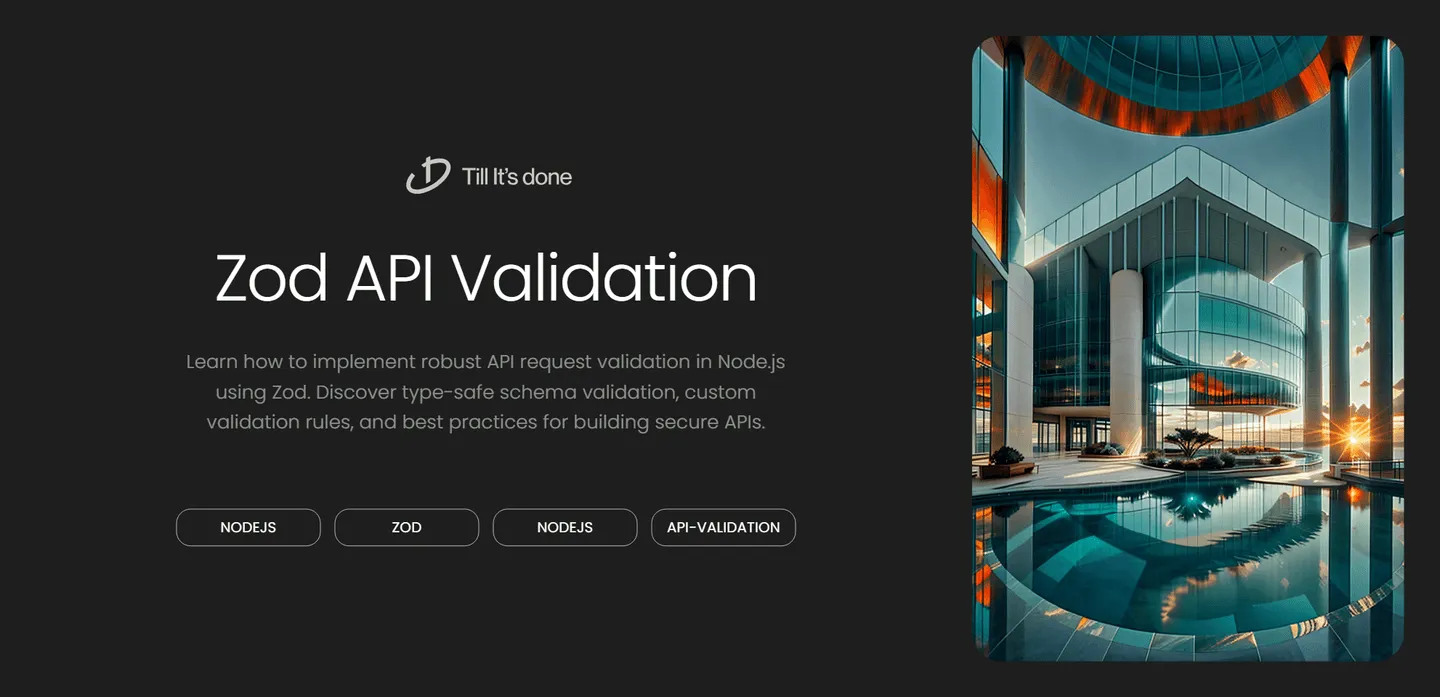

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.