- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Secure WordPress API Routes in Next.js Apps
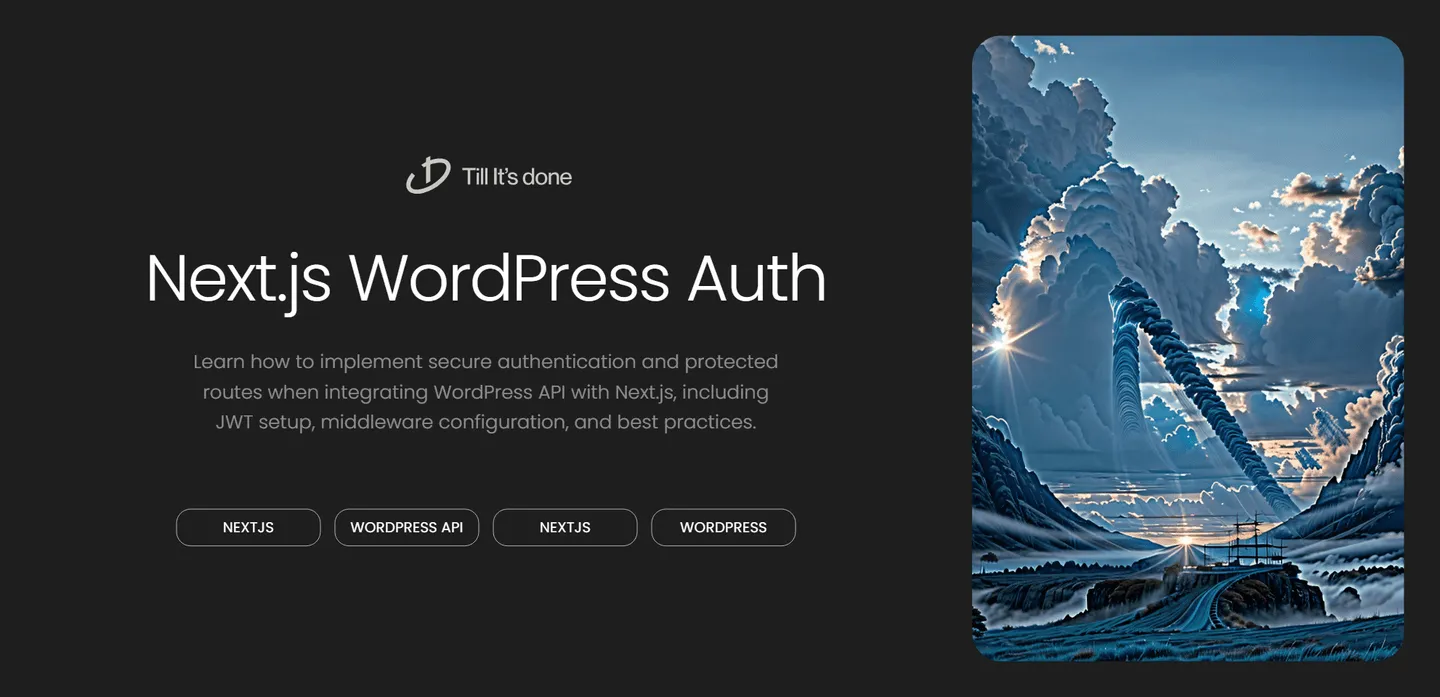
Authentication and Protected Routes with WordPress API in Next.js
Building secure web applications is crucial in today’s digital landscape. When combining the power of Next.js with WordPress as a headless CMS, implementing proper authentication and protected routes becomes essential. Let’s dive into how we can achieve this while maintaining a smooth user experience.
Understanding WordPress Authentication
WordPress REST API uses JWT (JSON Web Tokens) for authentication. This modern approach provides a secure way to handle user sessions without storing sensitive information on the client side. The token acts as a digital passport, allowing users to access protected resources.
Setting Up JWT Authentication
Before diving into the implementation, you’ll need to set up JWT authentication on your WordPress site. The “JWT Authentication for WP REST API” plugin is a popular choice for this purpose. Once installed, it provides the endpoints we need for authentication.
Here’s how we’ll structure our authentication flow:
- User submits login credentials
- WordPress validates and returns a JWT token
- Next.js stores the token securely
- Protected routes use this token for verification
Implementing Protected Routes
Let’s walk through the essential components of our authentication system. First, we’ll need a middleware to check for valid tokens:
export { default } from 'next-auth/middleware'
export const config = { matcher: ['/dashboard/:path*', '/profile/:path*']}
Next, create a custom hook to manage authentication state:
export const useAuth = () => { const [isAuthenticated, setIsAuthenticated] = useState(false) const router = useRouter()
const login = async (credentials) => { try { const response = await fetch('/api/auth/login', { method: 'POST', body: JSON.stringify(credentials) }) const data = await response.json()
if (data.token) { // Store token securely localStorage.setItem('authToken', data.token) setIsAuthenticated(true) router.push('/dashboard') } } catch (error) { console.error('Login failed:', error) } }
// ... logout and other auth methods}
Creating Protected API Routes
Your Next.js API routes need protection too. Here’s how to verify tokens before allowing access:
import { verifyToken } from '@/lib/auth'
export default async function handler(req, res) { try { const token = req.headers.authorization?.split(' ')[1] if (!token) { return res.status(401).json({ message: 'No token provided' }) }
const verified = await verifyToken(token) if (!verified) { return res.status(403).json({ message: 'Invalid token' }) }
// Handle protected route logic here
} catch (error) { res.status(500).json({ message: 'Internal server error' }) }}
Best Practices and Security Considerations
- Always use HTTPS in production
- Implement token refresh mechanisms
- Clear tokens on logout
- Set appropriate token expiration times
- Handle token validation errors gracefully
- Use secure storage methods for tokens
Error Handling
Your authentication system should handle various scenarios gracefully:
- Invalid credentials
- Expired tokens
- Network errors
- Unauthorized access attempts
Provide clear feedback to users while maintaining security by not exposing sensitive information in error messages.
Putting It All Together
By implementing these authentication measures, you create a secure foundation for your Next.js application while leveraging WordPress’s powerful content management capabilities. Remember to regularly update dependencies and review security best practices as they evolve.
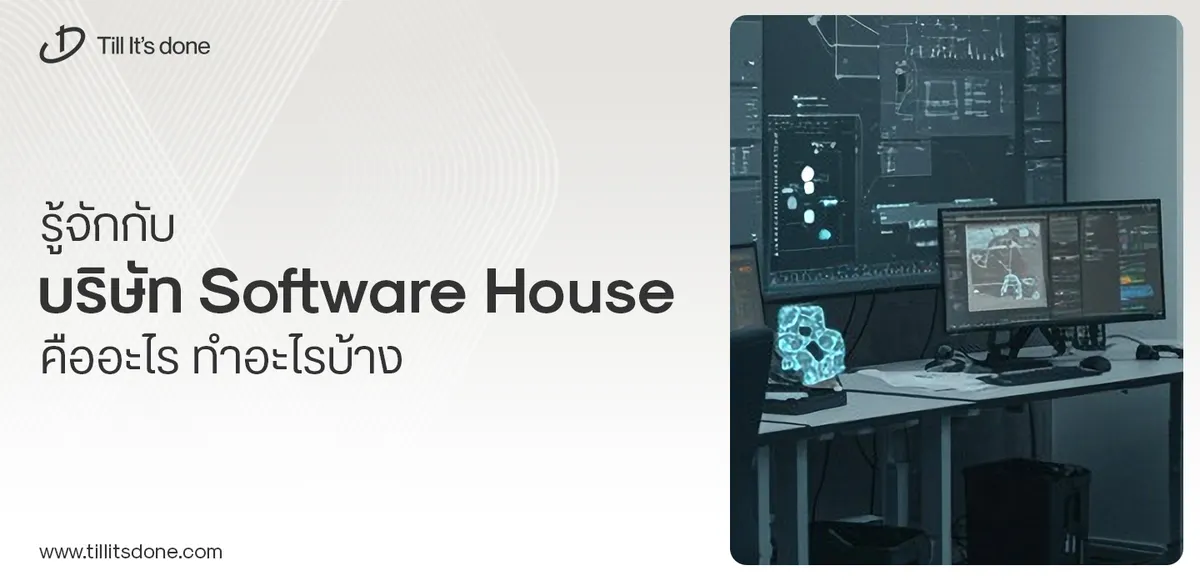
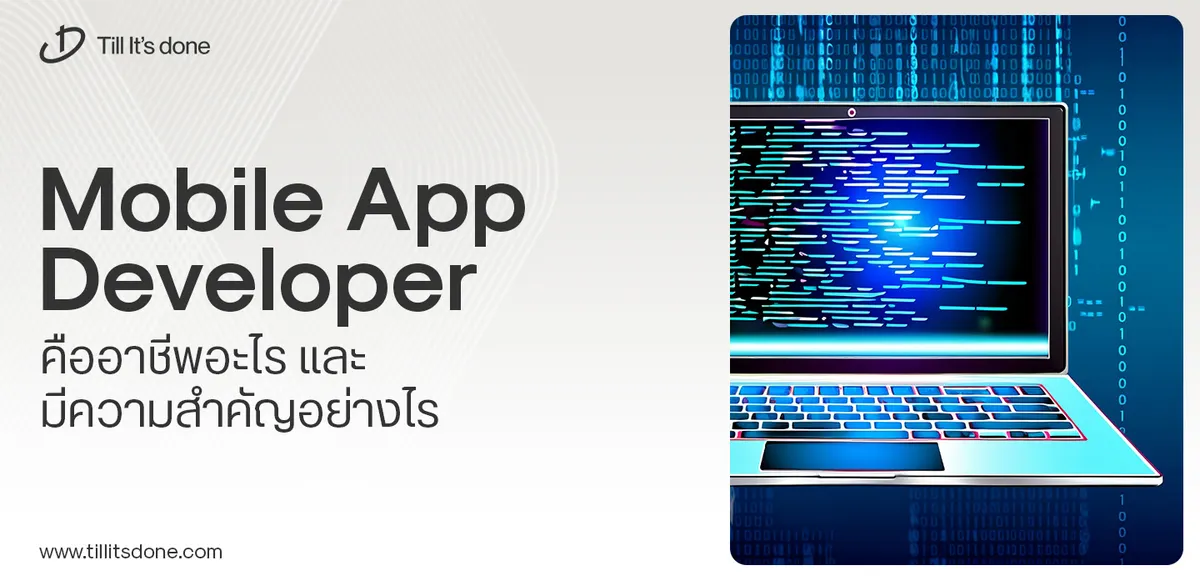
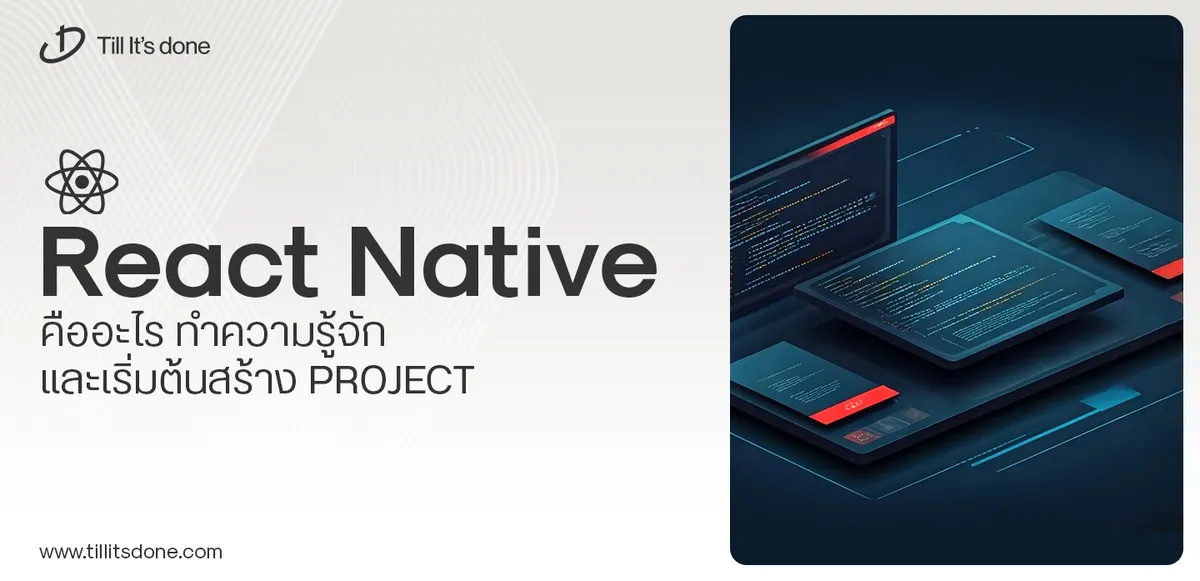
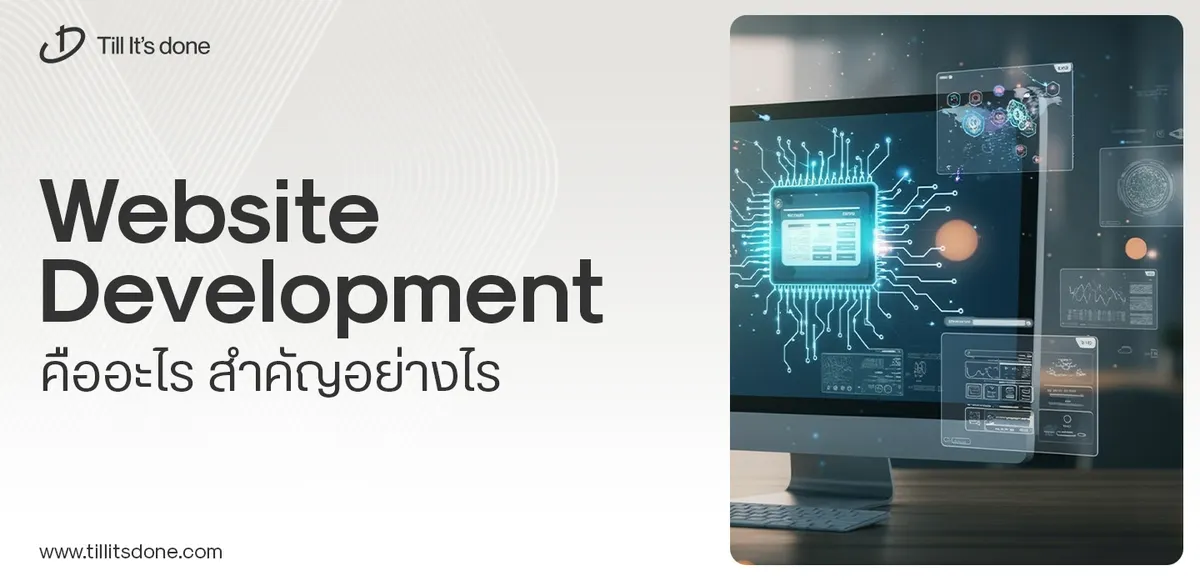
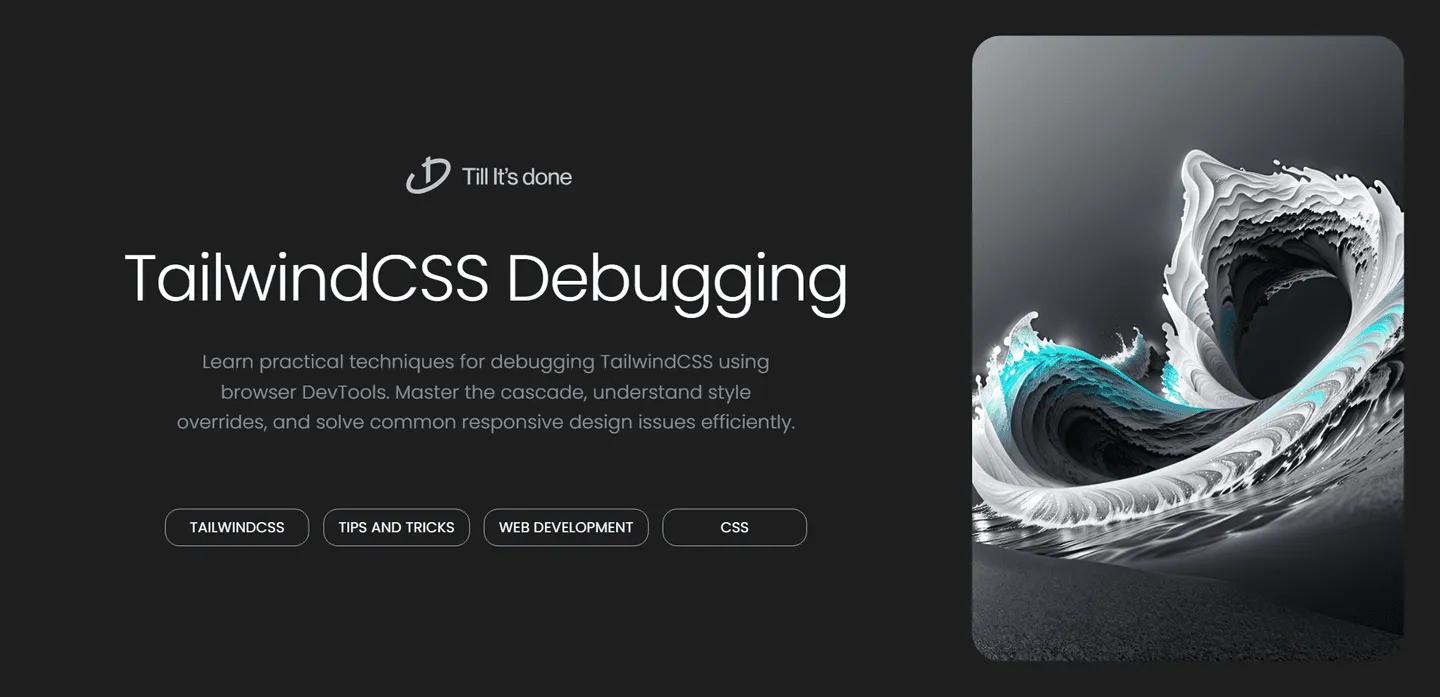
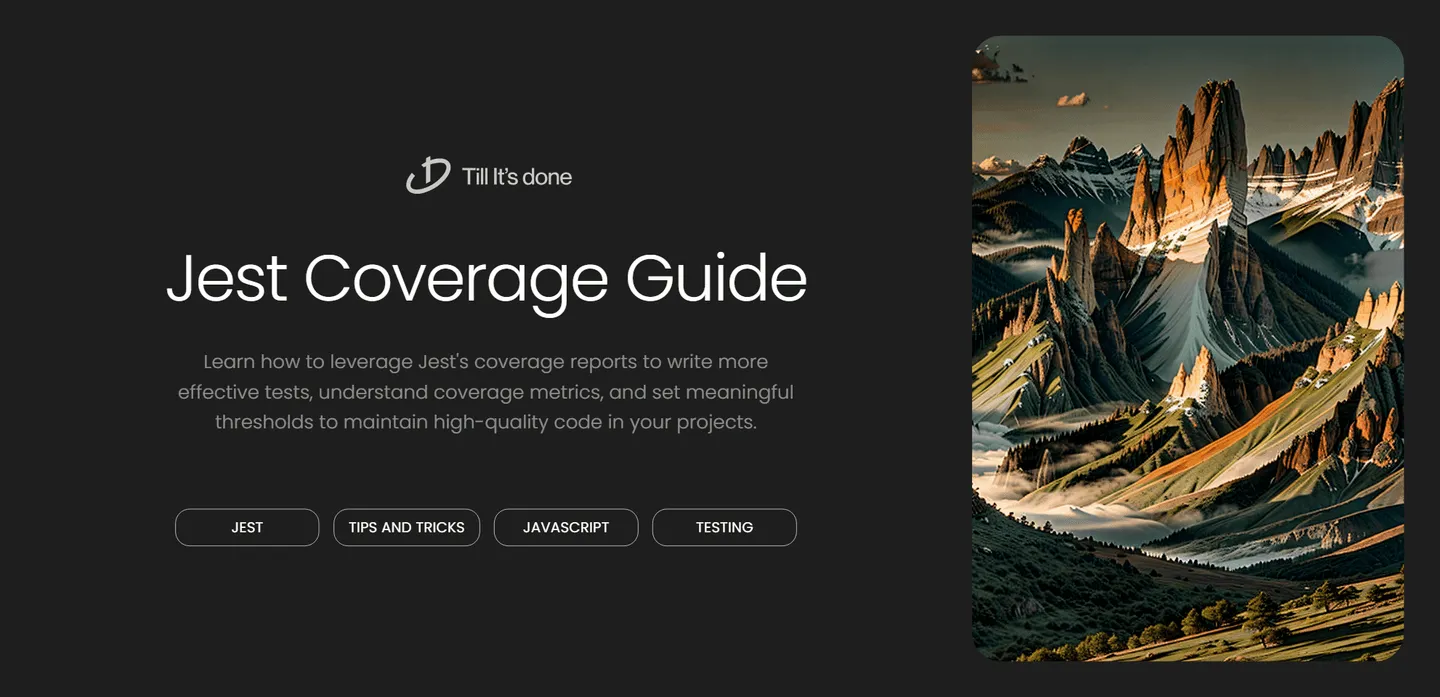
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.