- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
TypeScript and Dependency Injection Guide
Discover how to write more maintainable, testable, and scalable code using modern DI patterns.
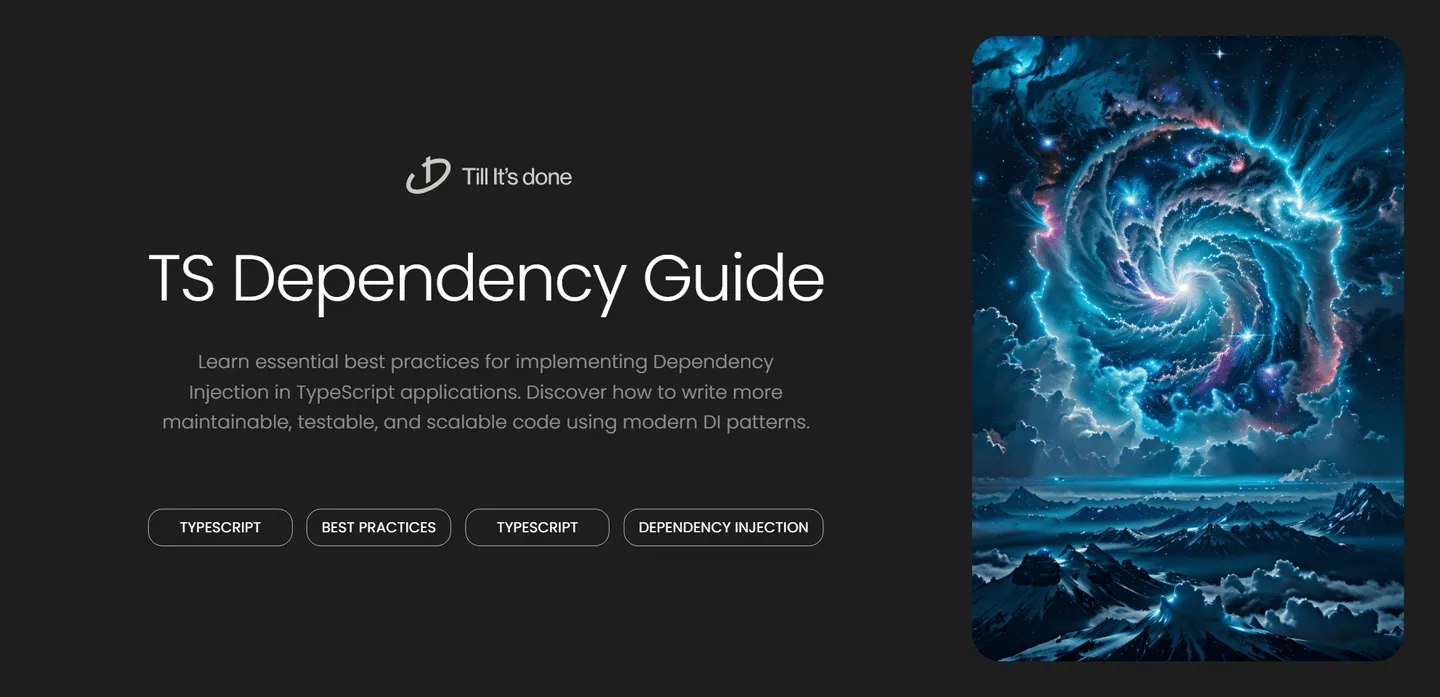
TypeScript and Dependency Injection: Best Practices
Dependency Injection (DI) is a powerful design pattern that can significantly improve your TypeScript applications’ maintainability, testability, and scalability. In this guide, we’ll explore how to implement DI effectively in TypeScript while following industry best practices.
Understanding the Fundamentals
At its core, Dependency Injection is about making your code more modular and loosely coupled. Instead of having classes create their dependencies internally, we inject them from the outside. This approach makes our code more flexible and easier to test.
Best Practices for TypeScript DI
1. Use Interfaces for Dependency Contracts
Always define clear interfaces for your dependencies. This practice makes your code more maintainable and enables easy mocking for tests:
interface IUserService { getUser(id: string): Promise<User>; updateUser(user: User): Promise<void>;}
class UserService implements IUserService { // Implementation}
2. Leverage Constructor Injection
Constructor injection is the most straightforward and recommended way to implement DI in TypeScript:
class UserController { constructor( private readonly userService: IUserService, private readonly logger: ILogger ) {}}
3. Implement IoC Containers
Using an Inversion of Control (IoC) container can greatly simplify dependency management. Popular options include InversifyJS or TypeDI:
@injectable()class UserRepository { // Implementation}
@injectable()class UserService { constructor( @inject(UserRepository) private repository: UserRepository ) {}}
4. Avoid Service Locator Pattern
While it might seem convenient, avoid using the Service Locator pattern as it makes dependencies implicit and harder to track:
// ❌ Don't do thisclass UserService { private repository = Container.get(UserRepository);}
// ✅ Do this insteadclass UserService { constructor(private repository: UserRepository) {}}
5. Implement Proper Scoping
Be mindful of dependency lifecycles and implement proper scoping:
@injectable()@singleton()class ConfigService { // Shared configuration service}
@injectable()@scoped(Lifecycle.Request)class UserSession { // Request-scoped service}
Best Practices for Testing
With proper DI implementation, testing becomes much more straightforward. You can easily mock dependencies:
describe('UserService', () => { it('should create user', async () => { const mockRepository = { save: jest.fn() }; const service = new UserService(mockRepository); // Test implementation });});
Conclusion
Implementing Dependency Injection in TypeScript not only makes your code more maintainable but also promotes better architecture decisions. By following these best practices, you’ll create more robust and testable applications that are easier to maintain and scale.
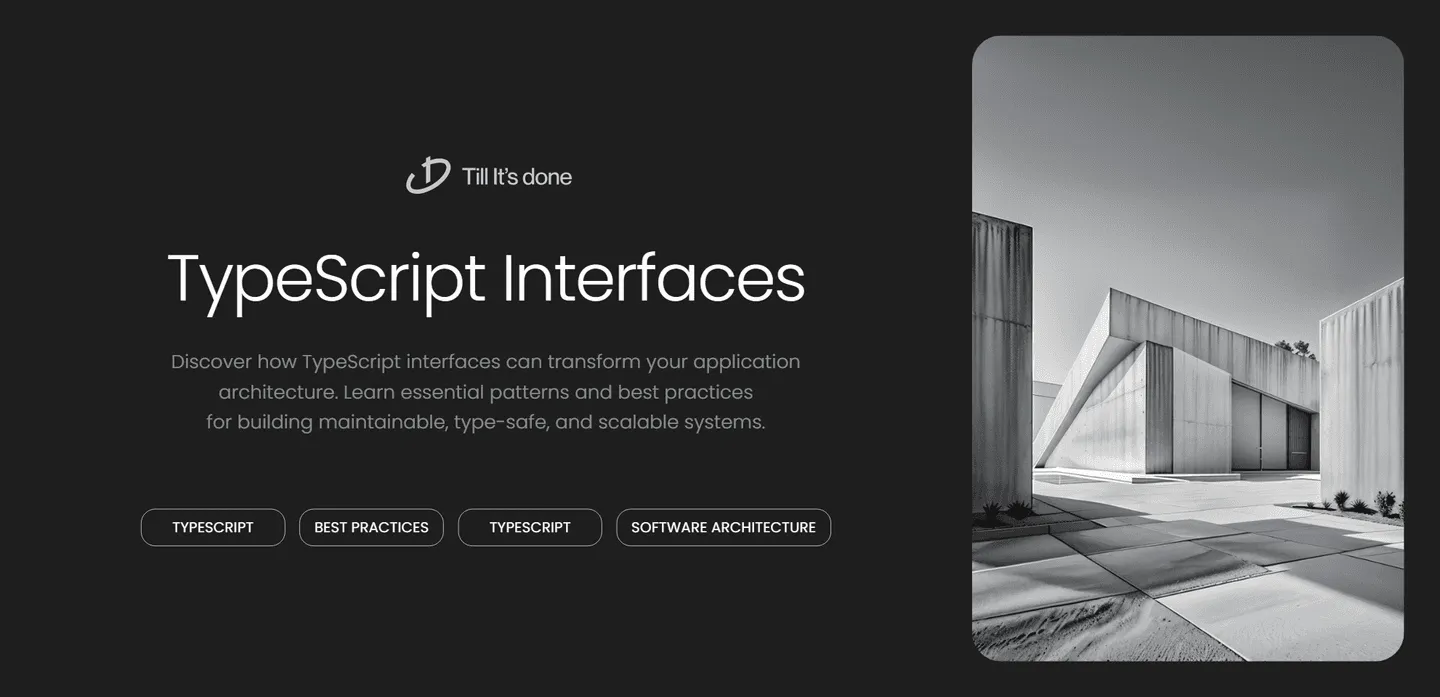
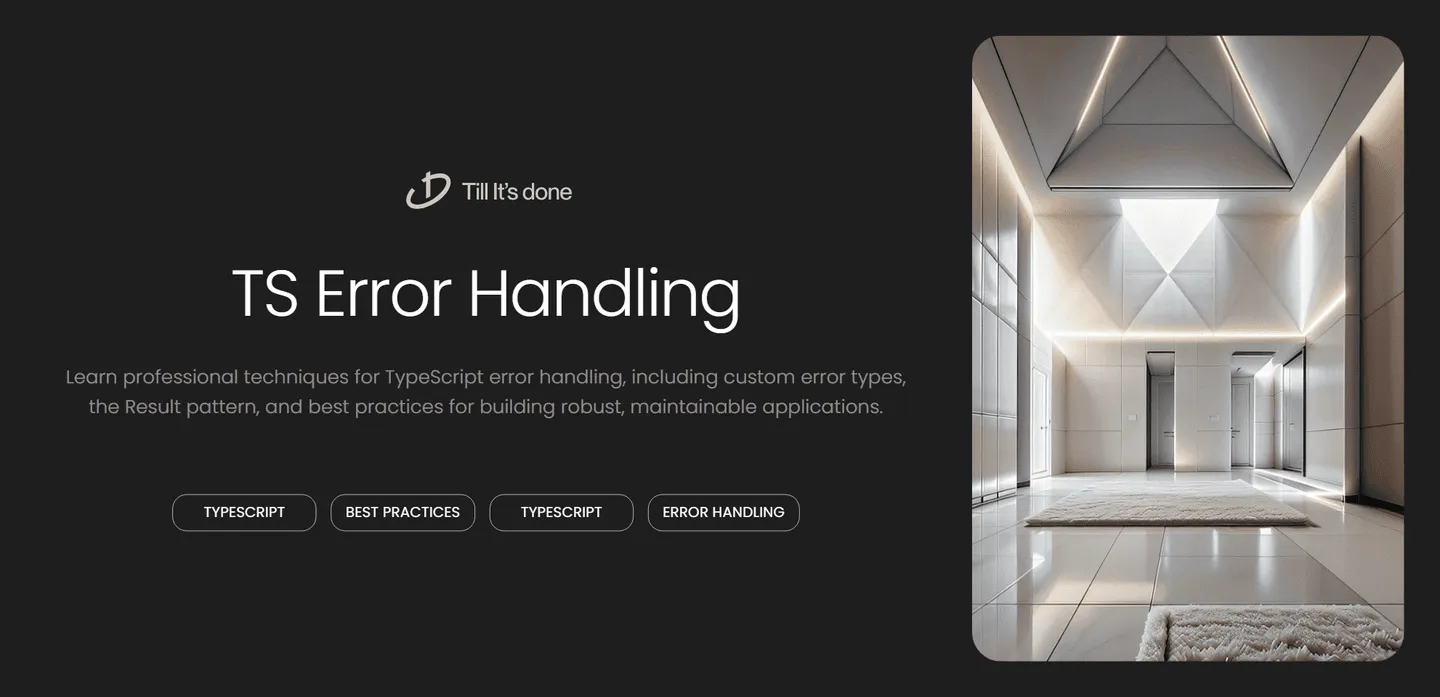
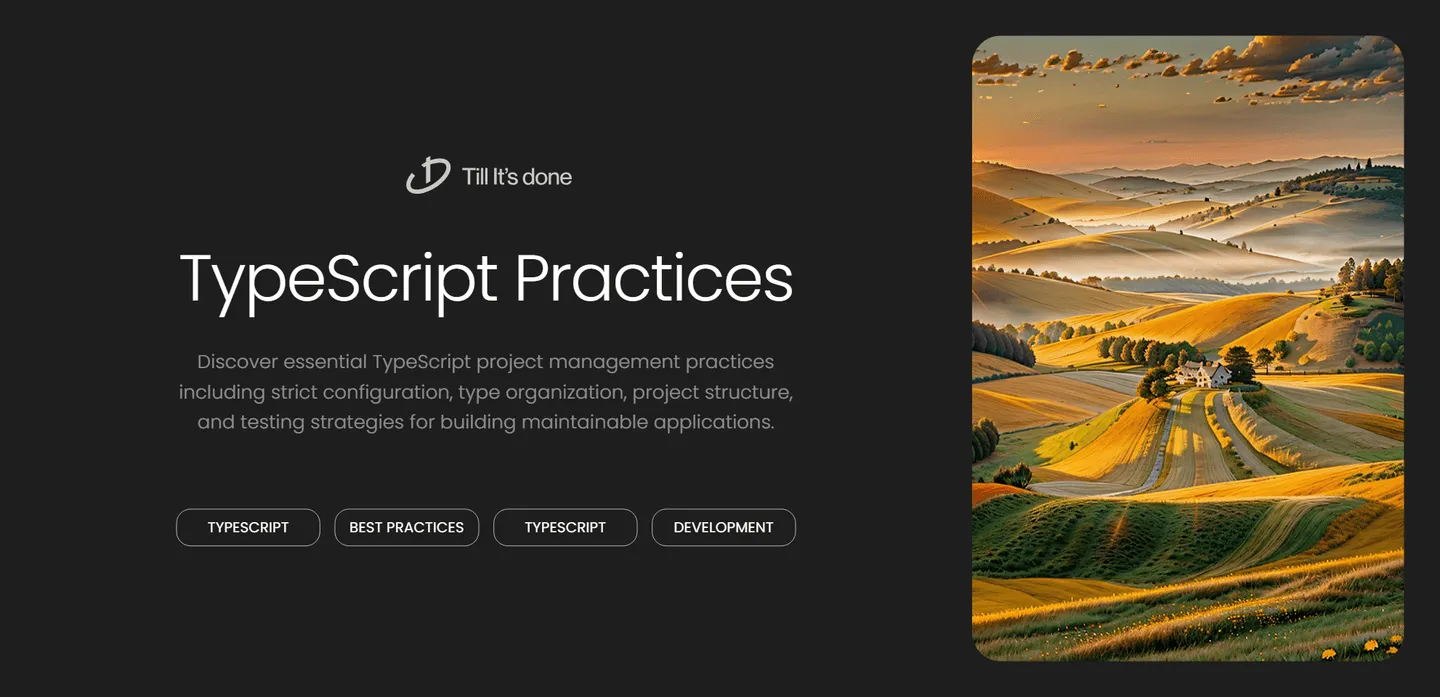
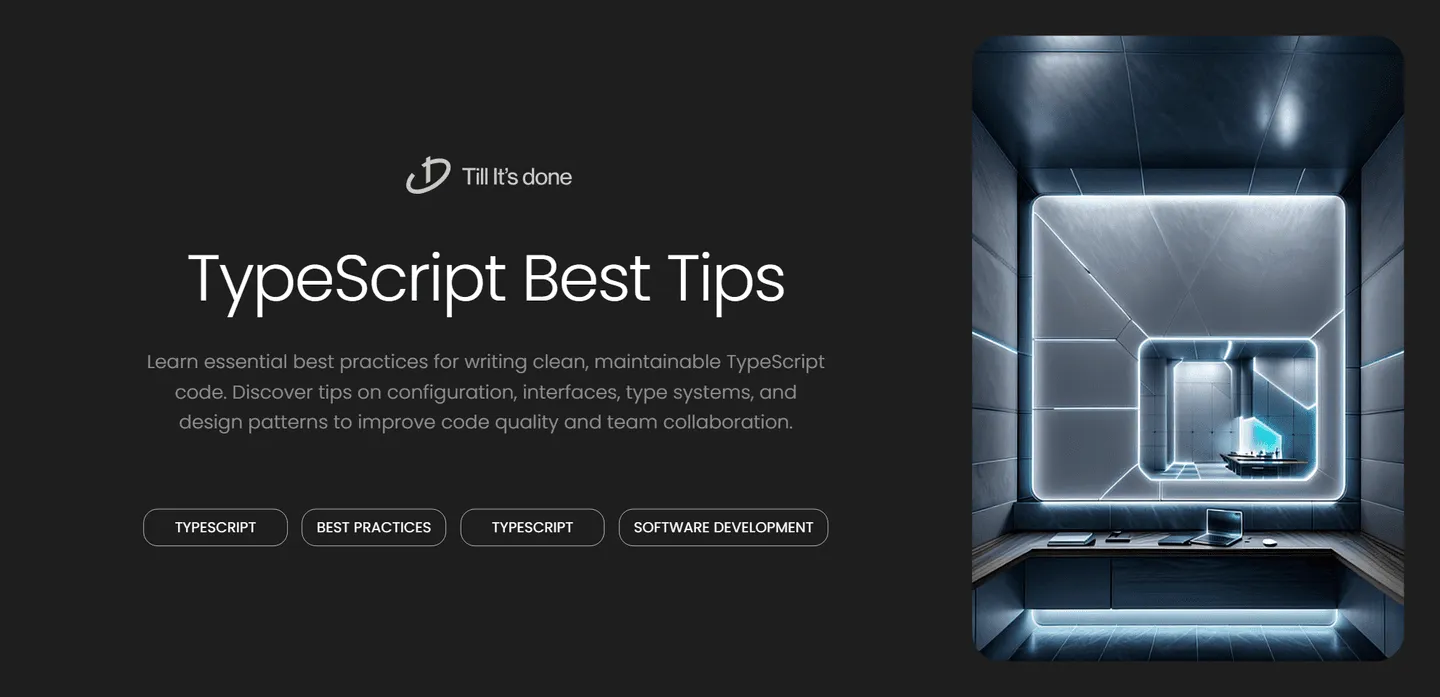
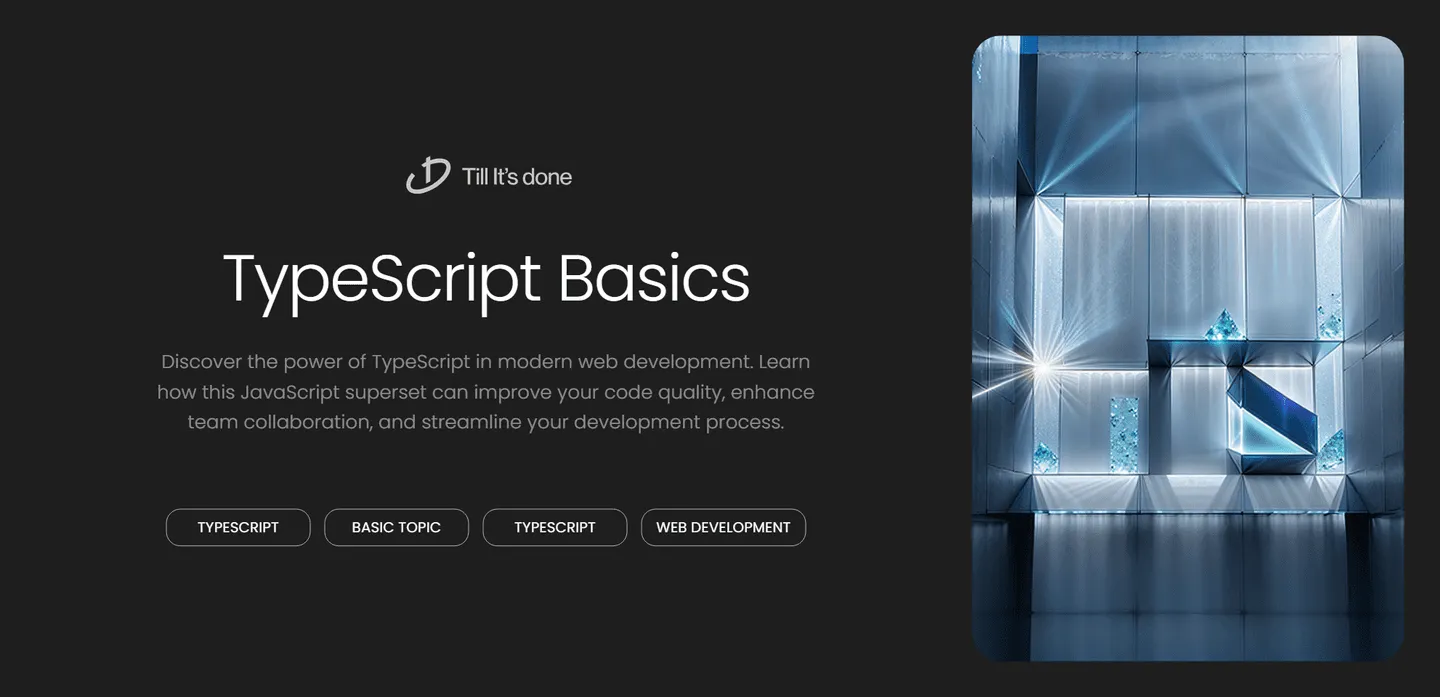
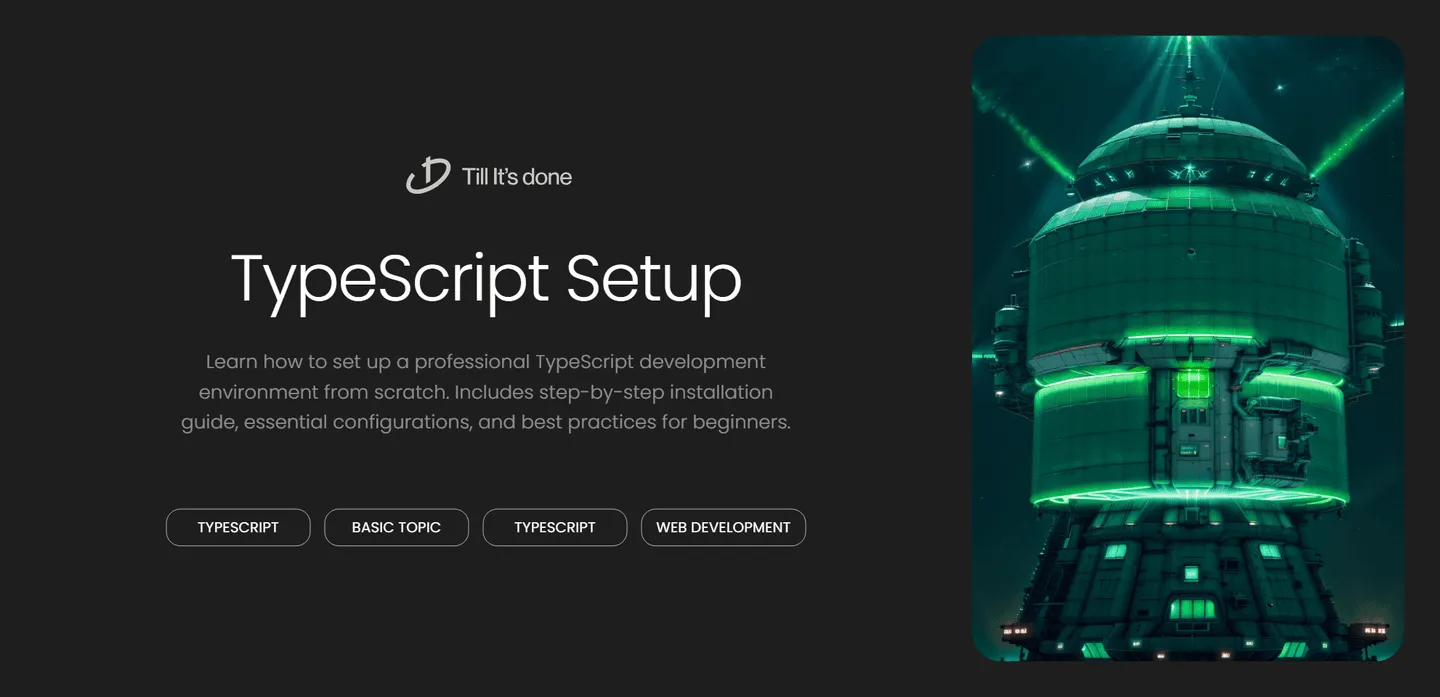
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.