- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Testing React Query Components: A Guide
Master the best practices for reliable testing.

How to Test Components That Use React Query
Testing React components that use React Query can be challenging at first, but with the right approach and tools, we can make it straightforward and reliable. Let’s dive into how we can effectively test these components while maintaining good testing practices.
Setting Up the Testing Environment
Before we start writing tests, we need to set up our testing environment properly. React Query provides a QueryClientProvider
that needs to wrap our components during testing. We’ll create a custom wrapper to make this process easier.
import { QueryClient, QueryClientProvider } from '@tanstack/react-query'import { render } from '@testing-library/react'
export function renderWithClient(ui: React.ReactElement) { const queryClient = new QueryClient({ defaultOptions: { queries: { retry: false, }, }, })
return render( <QueryClientProvider client={queryClient}> {ui} </QueryClientProvider> )}
Writing Your First Test
Let’s say we have a component that fetches and displays a list of todos. Here’s how we can test it:
describe('TodoList', () => { it('displays todos when data is fetched successfully', async () => { const { findByText } = renderWithClient(<TodoList />)
await waitFor(() => { expect(screen.getByText('Todo 1')).toBeInTheDocument() }) })})
Mocking API Responses
One crucial aspect of testing React Query components is properly mocking the API responses. We can use MSW (Mock Service Worker) or Jest’s mocking capabilities:
const mockTodos = [ { id: 1, title: 'Todo 1' }, { id: 2, title: 'Todo 2' },]
jest.mock('axios', () => ({ get: jest.fn(() => Promise.resolve({ data: mockTodos }))}))
Testing Different States
Remember to test your components in different states: loading, error, and success. React Query makes this easy with its built-in states:
it('shows loading state', () => { const { getByText } = renderWithClient(<TodoList />) expect(getByText('Loading...')).toBeInTheDocument()})
it('shows error state', async () => { // Mock failed API call jest.spyOn(axios, 'get').mockRejectedValueOnce(new Error('Failed to fetch'))
const { findByText } = renderWithClient(<TodoList />) await findByText('Error: Failed to fetch')})
Best Practices
- Always clean up after each test by clearing the query cache
- Use
waitFor
orfindBy
queries for asynchronous operations - Test loading, success, and error states
- Mock API responses consistently
- Use meaningful test descriptions
- Keep tests focused and isolated

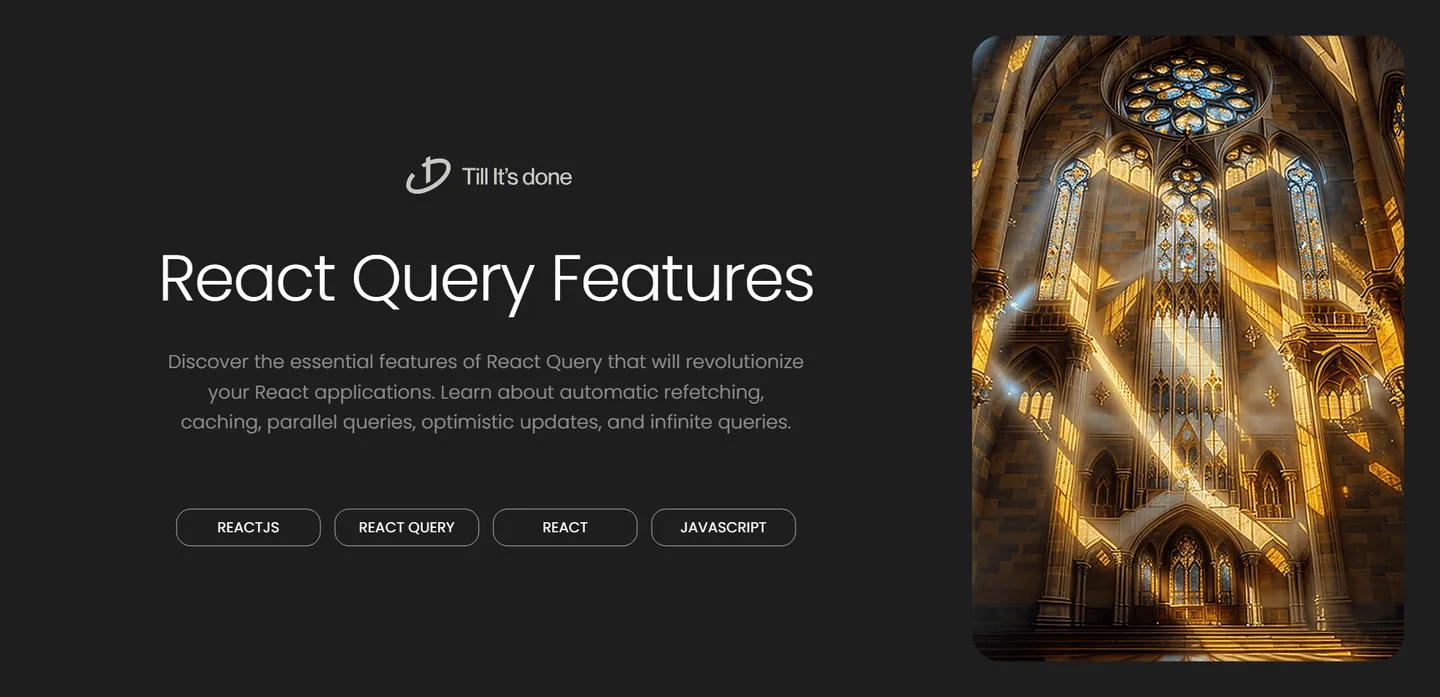


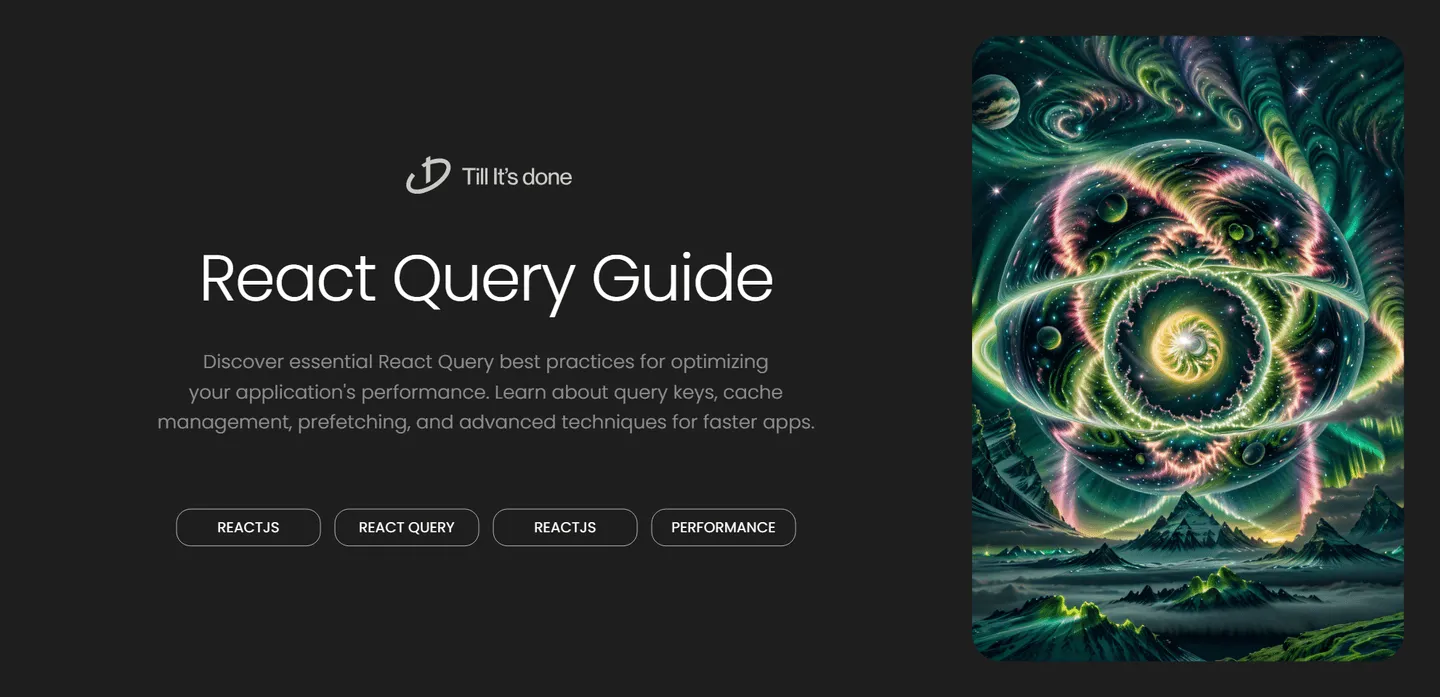

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.