- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Secure Axios API Calls in React: Tips & Tricks
Learn about interceptors, error handling, rate limiting, and data validation to build robust and secure React applications.
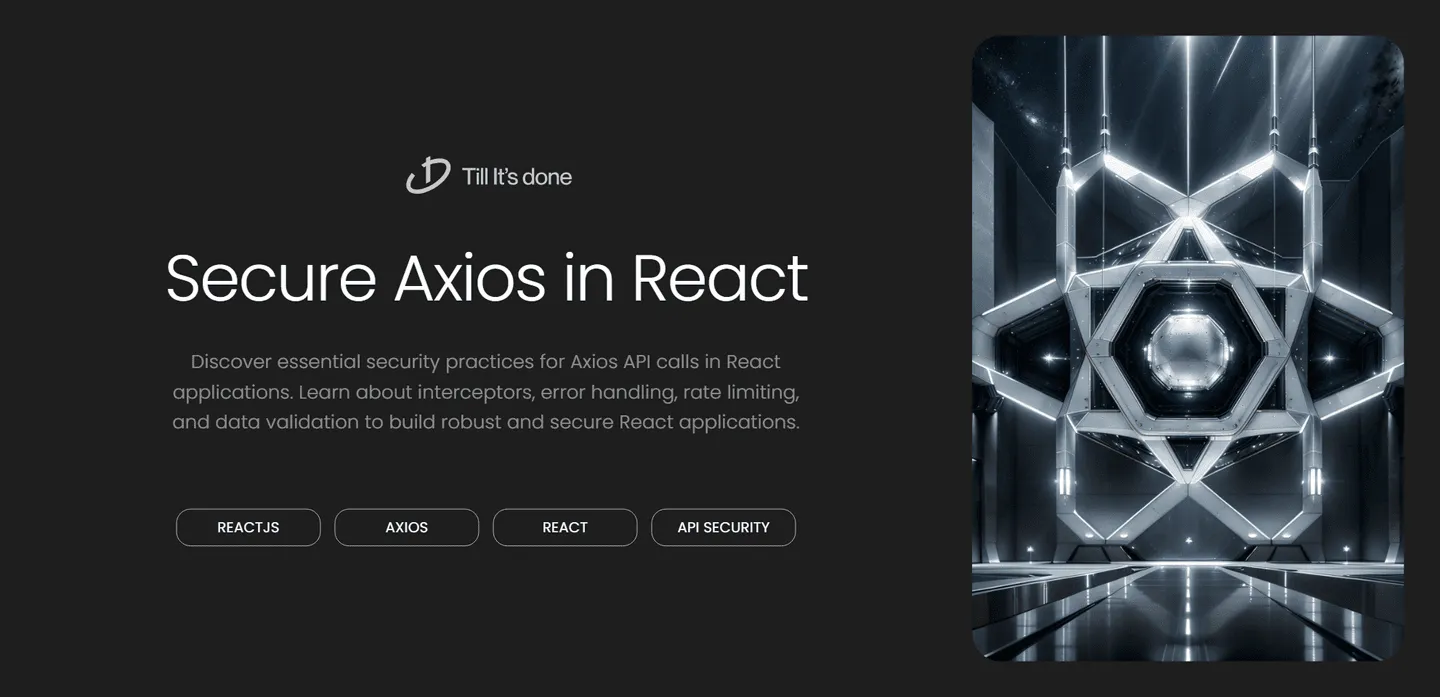
Secure Axios API Calls in React: Tips and Tricks
In today’s web development landscape, making secure API calls is crucial for protecting sensitive data and maintaining user trust. As a React developer who frequently works with APIs, I’ve learned that Axios isn’t just about sending requests – it’s about implementing robust security measures that safeguard your application. Let’s dive into some essential tips and tricks for securing your Axios API calls in React.
Setting Up a Secure Axios Instance
Creating a secure Axios instance is your first line of defense. Instead of making direct Axios calls throughout your application, configure a base instance with security headers and interceptors:
const axiosInstance = axios.create({ baseURL: process.env.REACT_APP_API_BASE_URL, timeout: 5000, headers: { 'Content-Type': 'application/json', 'X-Requested-With': 'XMLHttpRequest' }});
Implementing Request Interceptors
Request interceptors are powerful tools for adding authentication tokens and handling errors consistently:
axiosInstance.interceptors.request.use( config => { const token = localStorage.getItem('token'); if (token) { config.headers.Authorization = `Bearer ${token}`; } return config; }, error => Promise.reject(error));
Error Handling and Response Validation
Never trust raw API responses. Always validate and sanitize your data before using it in your application:
axiosInstance.interceptors.response.use( response => { if (response.data) { return sanitizeData(response.data); } return response; }, error => { if (error.response?.status === 401) { // Handle unauthorized access handleUnauthorized(); } return Promise.reject(error); });
Best Practices for Production
Remember to implement these essential security measures:
- Use environment variables for sensitive data
- Implement retry logic for failed requests
- Set appropriate timeout values
- Add request cancellation for unmounted components
- Implement rate limiting
- Use HTTPS only in production
- Regularly update Axios and its dependencies
Rate Limiting and Caching
Implement rate limiting to prevent abuse and use caching to improve performance:
const rateLimiter = new RateLimiter(100, 60000); // 100 requests per minuteconst cache = new Map();
async function secureApiCall(endpoint) { if (cache.has(endpoint)) { return cache.get(endpoint); }
await rateLimiter.removeTokens(1); const response = await axiosInstance.get(endpoint); cache.set(endpoint, response.data); return response.data;}
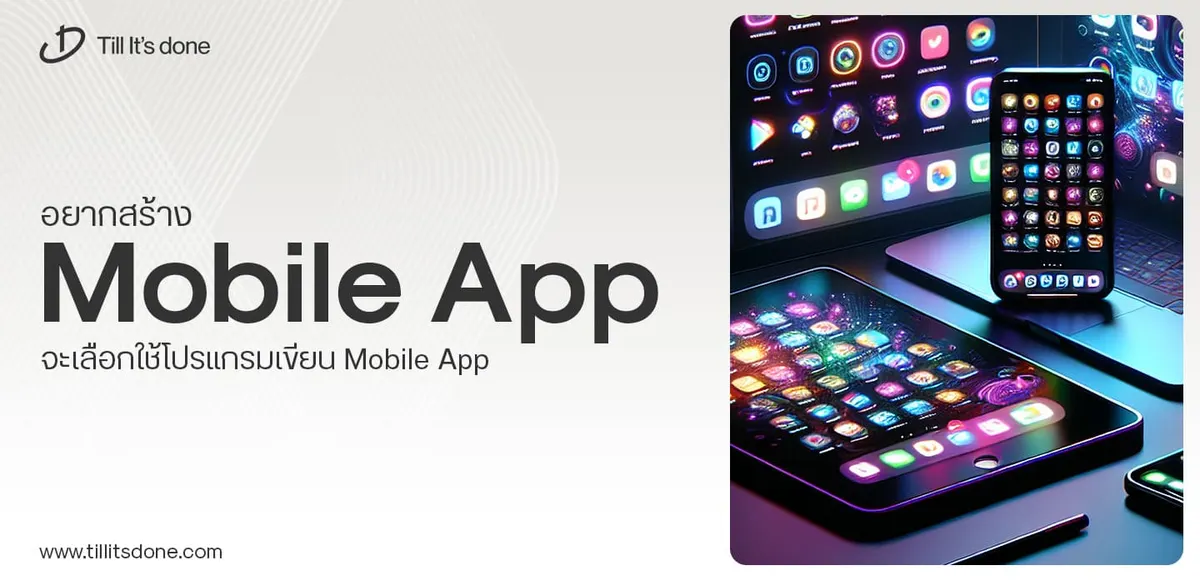
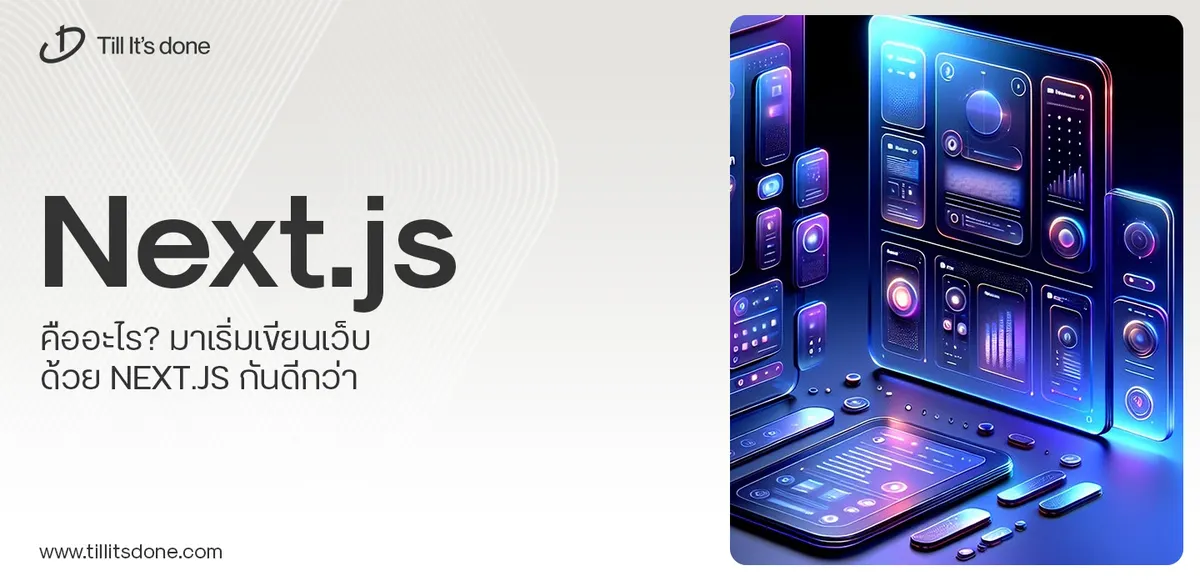
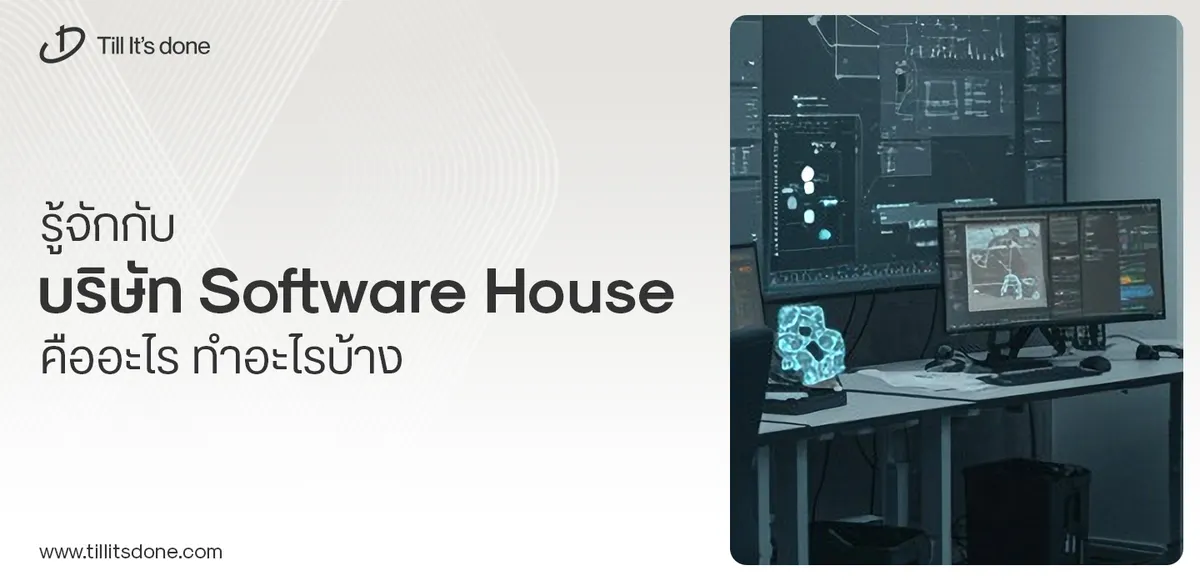
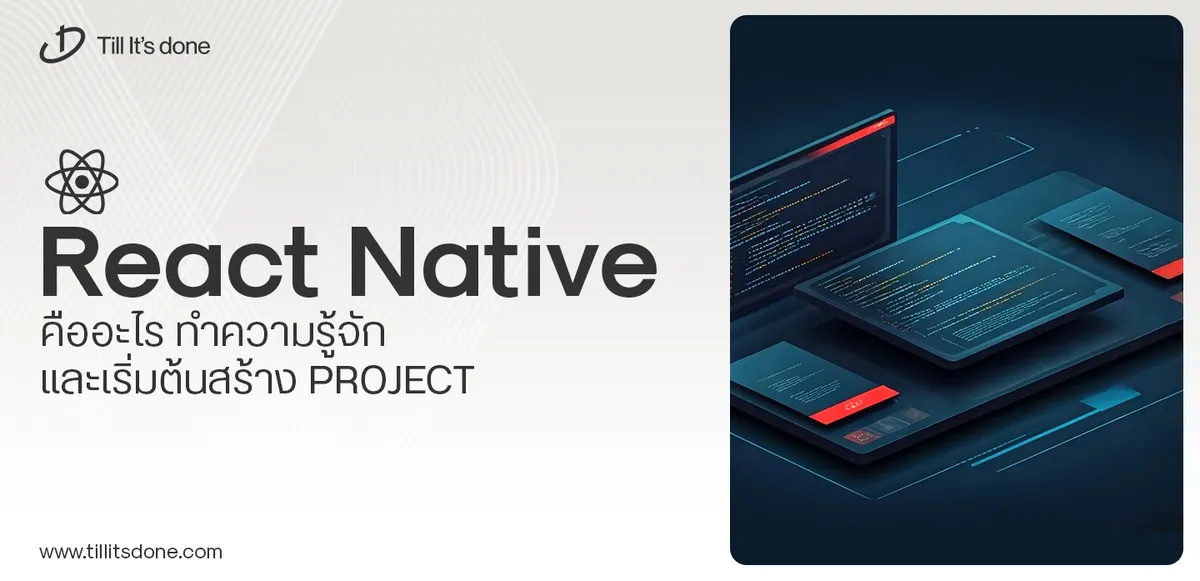
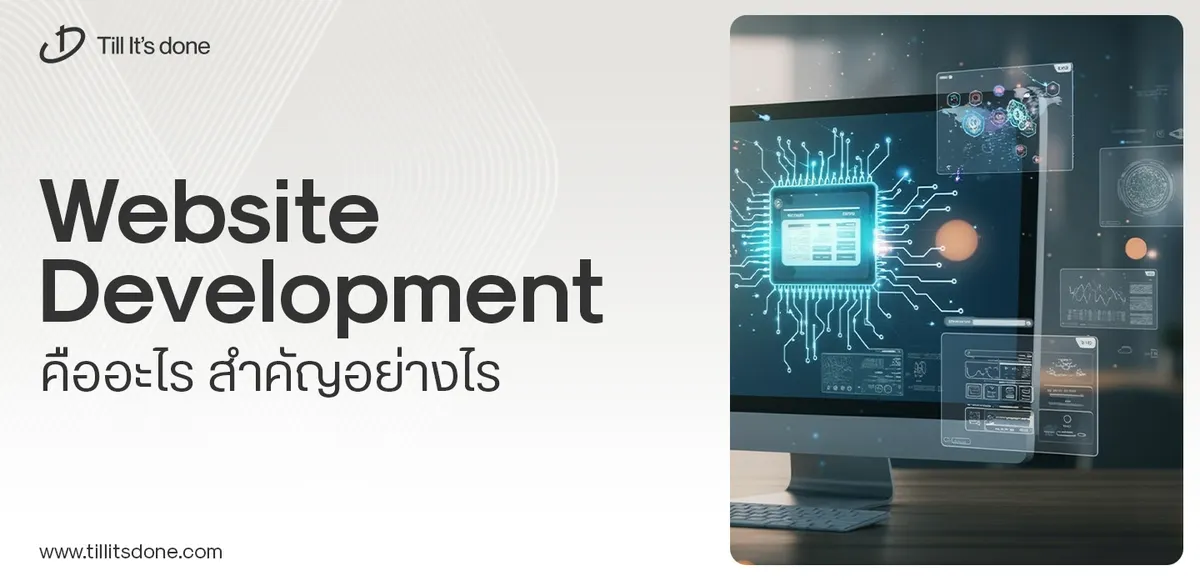
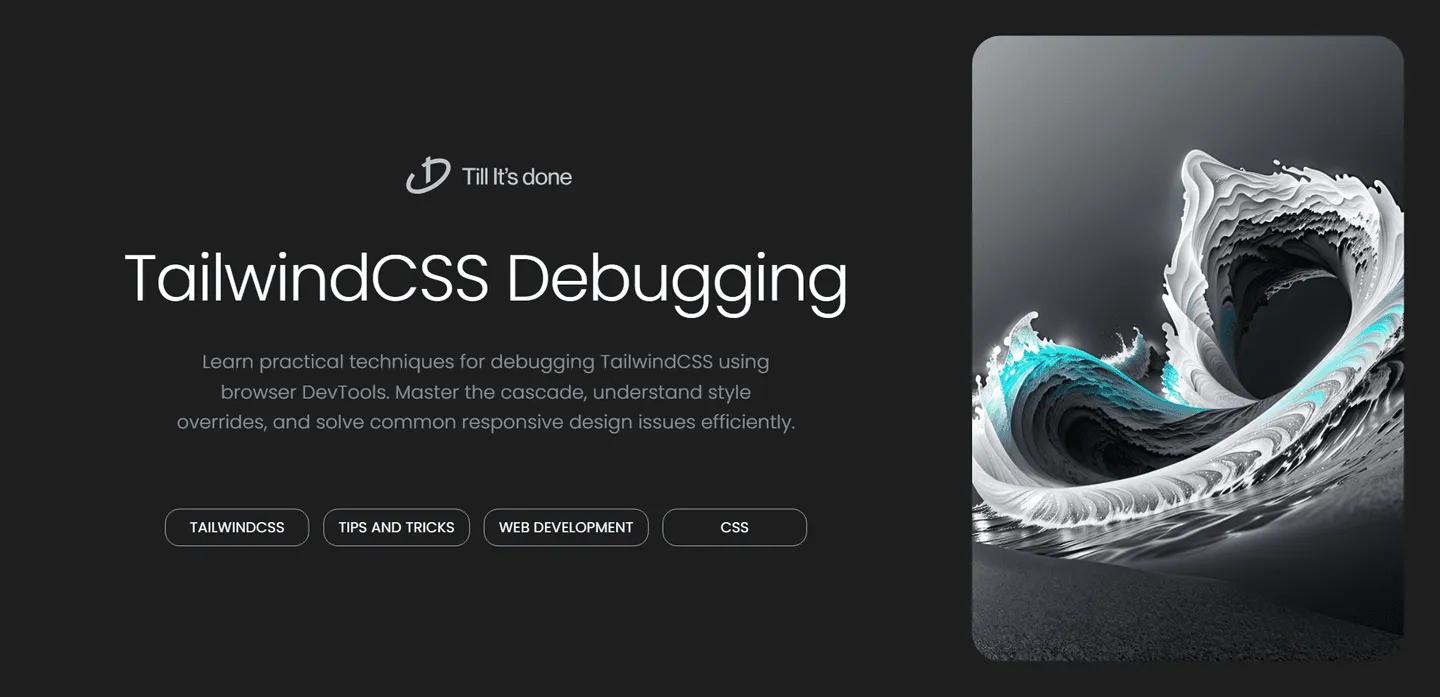
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.