- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Real-Time Data Handling with React Query Guide
Learn about automatic background updates, smart caching strategies, and optimistic updates for building responsive apps.

Real-Time Data Handling with React Query: A Deep Dive into Modern Data Management
In today’s fast-paced web applications, real-time data handling isn’t just a luxury—it’s a necessity. Whether you’re building a social media feed, a trading platform, or a collaborative tool, your users expect data to be fresh, synchronized, and instantly available. Enter React Query: a game-changing library that’s revolutionizing how we handle server state in React applications.
Why React Query?
Remember the days of managing loading states, error handling, and caching logic manually? Those days are behind us. React Query provides an elegant solution to these common challenges, offering a powerful toolkit that feels like it should have been part of React all along.
Key Features That Make React Query Shine
1. Automatic Background Updates
Think of React Query as your personal data butler. It quietly works in the background, ensuring your data stays fresh without you having to lift a finger. Here’s a practical example:
const { data, isLoading } = useQuery({ queryKey: ['todos'], queryFn: fetchTodos, refetchInterval: 1000, // Refetch every second});
2. Smart Caching Strategies
Gone are the days of reinventing the wheel with caching logic. React Query implements intelligent caching strategies that just work:
const useUserData = (userId) => { return useQuery({ queryKey: ['user', userId], queryFn: () => fetchUserById(userId), staleTime: 5 * 60 * 1000, // Data considered fresh for 5 minutes cacheTime: 30 * 60 * 1000, // Cache persists for 30 minutes });};
3. Optimistic Updates
Want to make your UI feel lightning-fast? Optimistic updates allow you to update the UI immediately while the server catches up:
const mutation = useMutation({ mutationFn: updateTodo, onMutate: async (newTodo) => { await queryClient.cancelQueries(['todos']); const previousTodos = queryClient.getQueryData(['todos']); queryClient.setQueryData(['todos'], (old) => [...old, newTodo]); return { previousTodos }; },});
Best Practices for Real-Time Data Handling
- Implement Proper Error Boundaries: Always prepare for the unexpected. React Query makes this easier with built-in error handling:
const { data, error, isError } = useQuery({ queryKey: ['data'], queryFn: fetchData, retry: 3, onError: (error) => { logger.error('Data fetch failed:', error); },});
- Leverage Window Focus Refetching: React Query automatically refetches data when users return to your app, ensuring they never see stale data:
const { data } = useQuery({ queryKey: ['prices'], queryFn: fetchPrices, refetchOnWindowFocus: true,});
- Implement Infinite Scroll: Handle large datasets efficiently with built-in pagination support:
const { data, fetchNextPage, hasNextPage,} = useInfiniteQuery({ queryKey: ['posts'], queryFn: fetchPostPage, getNextPageParam: (lastPage) => lastPage.nextCursor,});
Conclusion
React Query has fundamentally changed how we think about data management in React applications. By providing powerful primitives for real-time data handling, it allows developers to focus on building features rather than wrestling with data synchronization logic.
Remember, the key to successful real-time data handling isn’t just about implementing the right tools—it’s about understanding your application’s needs and choosing the right patterns to match them. React Query gives you the flexibility to do just that, with an API that’s both powerful and intuitive.
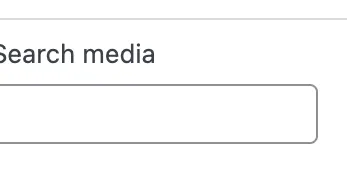





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.