- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
React Hooks: useState and useEffect Guide
Discover how to manage state and side effects in functional components with practical examples and best practices.
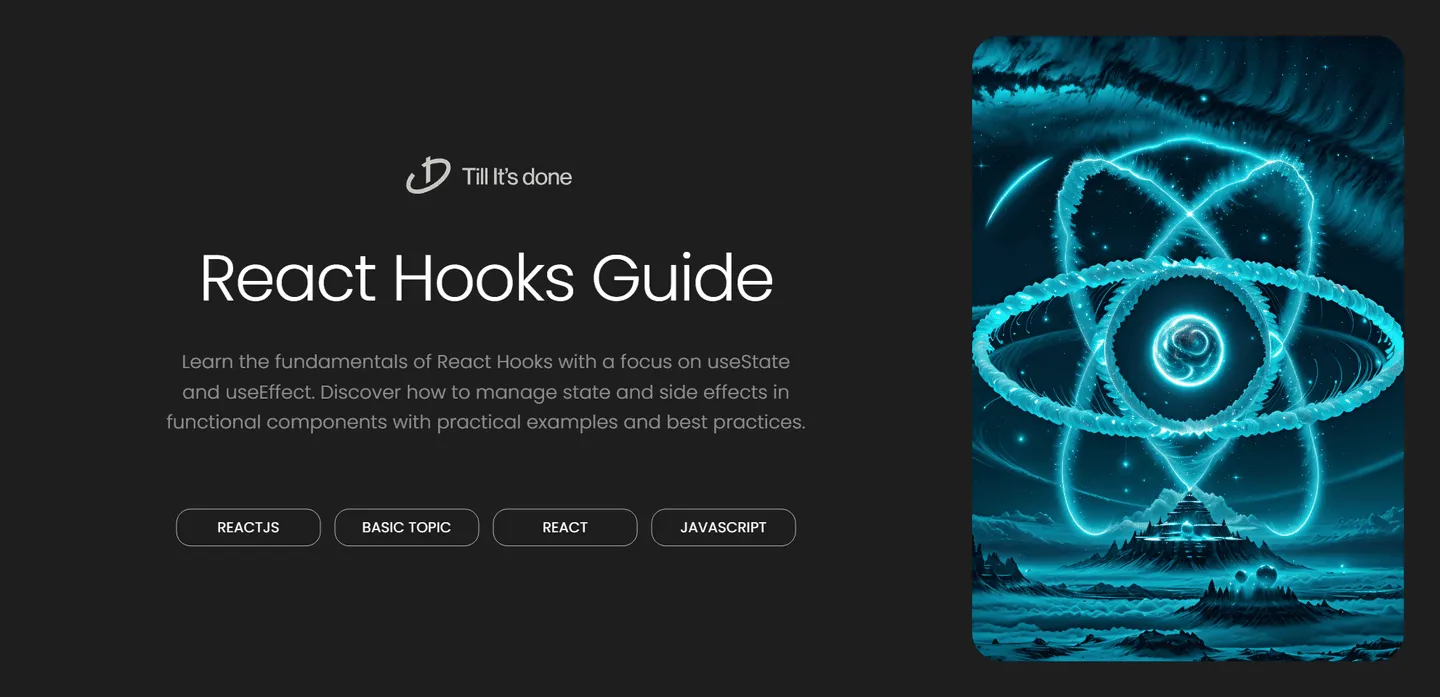
Introduction to React Hooks: useState and useEffect
Hey there! Today, I’m super excited to dive into one of the game-changing features of React - Hooks! If you’ve been working with React components, you’re about to discover how Hooks can make your code cleaner and more powerful. Let’s focus on the two most commonly used hooks: useState and useEffect.
What are React Hooks?
Think of Hooks as special functions that let you “hook into” React’s features. Before Hooks came along, you needed to write class components to use state and lifecycle methods. Now, we can use these features in functional components - pretty cool, right?
Understanding useState
The useState hook is like your component’s memory. It helps you keep track of data that can change over time. Let’s look at a simple example:
import { useState } from 'react';
function Counter() { const [count, setCount] = useState(0);
return ( <div> <p>You clicked {count} times</p> <button onClick={() => setCount(count + 1)}> Click me </button> </div> );}
Here’s what’s happening:
- useState returns an array with two items: the current state value and a function to update it
- The initial state (0 in our example) is what we pass to useState()
- When we call setCount, React knows it needs to re-render our component
Exploring useEffect
useEffect is your way to handle side effects in your components. Think of it as your component’s connection to the outside world. It’s perfect for:
- Fetching data
- Setting up subscriptions
- Manually changing the DOM
Here’s a practical example:
import { useState, useEffect } from 'react';
function WindowWidth() { const [width, setWidth] = useState(window.innerWidth);
useEffect(() => { const handleResize = () => setWidth(window.innerWidth); window.addEventListener('resize', handleResize);
// Cleanup function return () => { window.removeEventListener('resize', handleResize); }; }, []); // Empty dependency array
return <p>Window width: {width}</p>;}
The key things to remember about useEffect:
- It runs after every render by default
- The dependency array (second argument) controls when it runs
- The cleanup function (returned function) runs before the component unmounts
Best Practices and Tips
- Always name your custom hooks starting with “use”
- Keep your hooks at the top level of your component
- Don’t call hooks inside loops or conditions
- Use multiple useEffects for different concerns
- Don’t forget to clean up side effects when necessary
These hooks are just the beginning of what’s possible with React. Once you get comfortable with useState and useEffect, you’ll find yourself writing more maintainable and efficient React applications. Happy coding! 🚀
Remember: Practice makes perfect, so don’t worry if it takes some time to get used to thinking in hooks. Keep experimenting and building things!
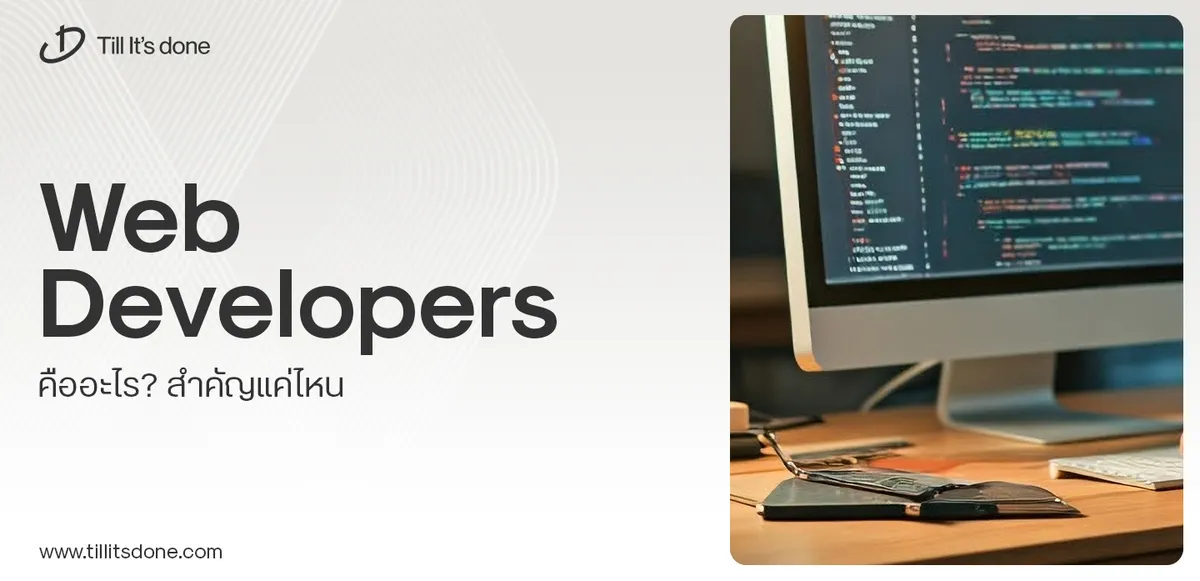
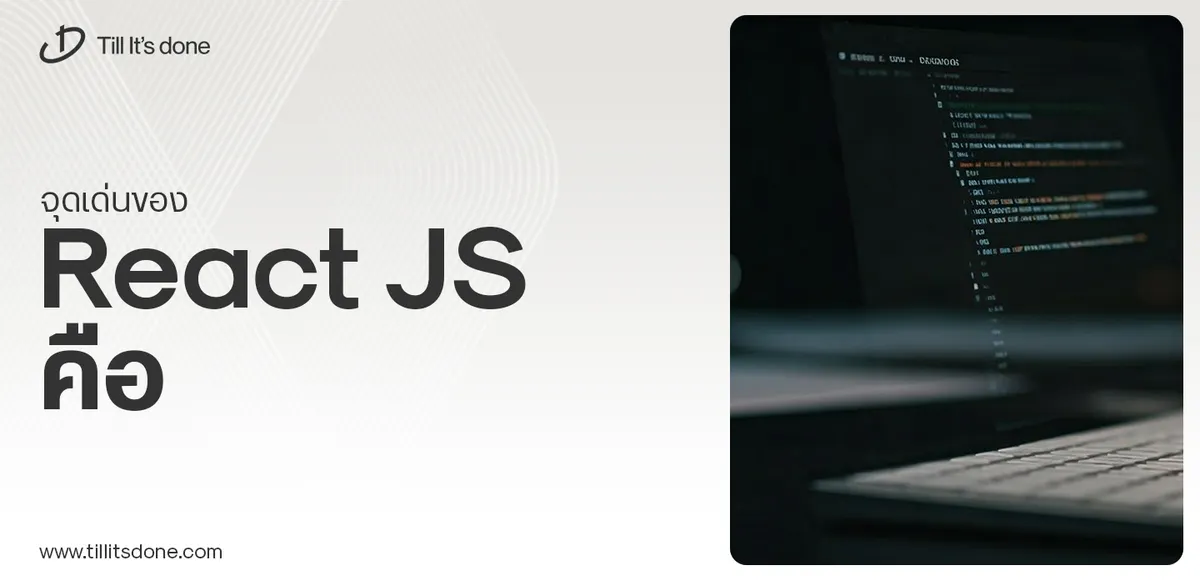
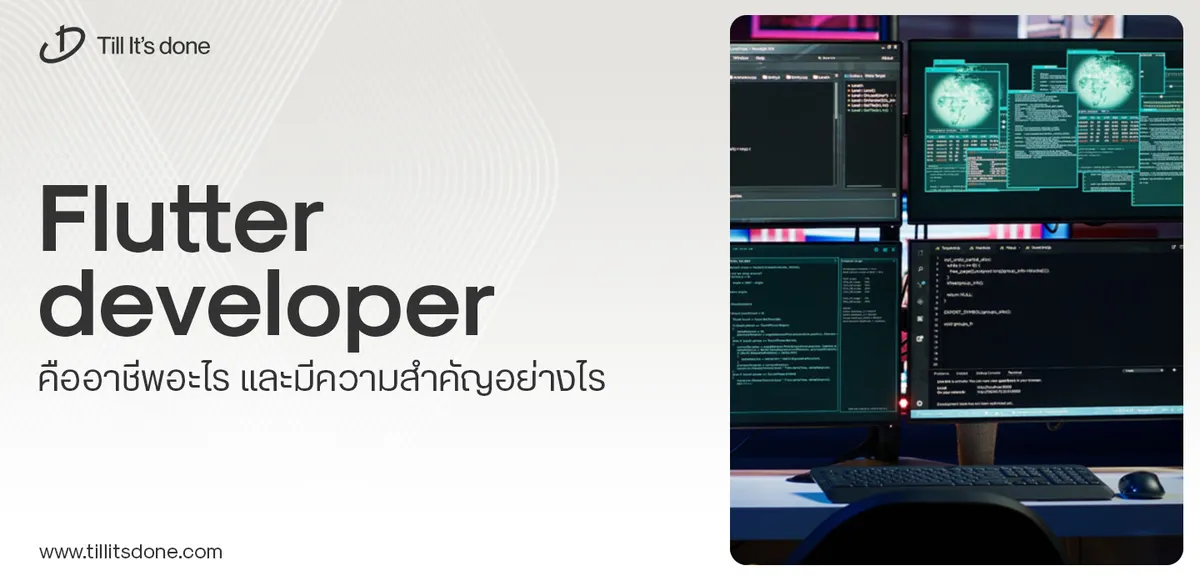
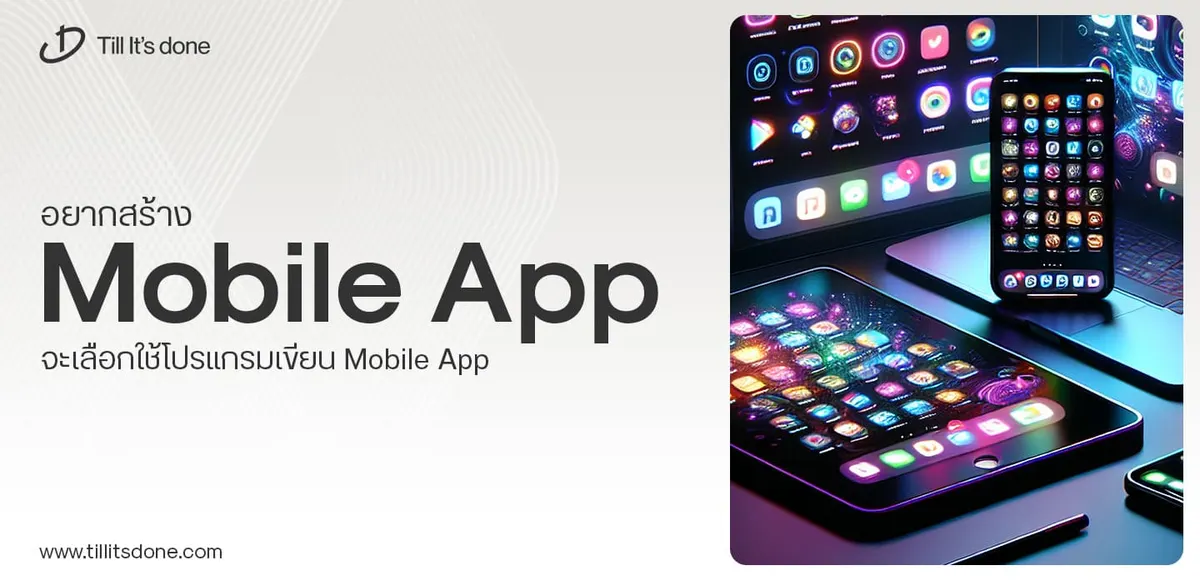
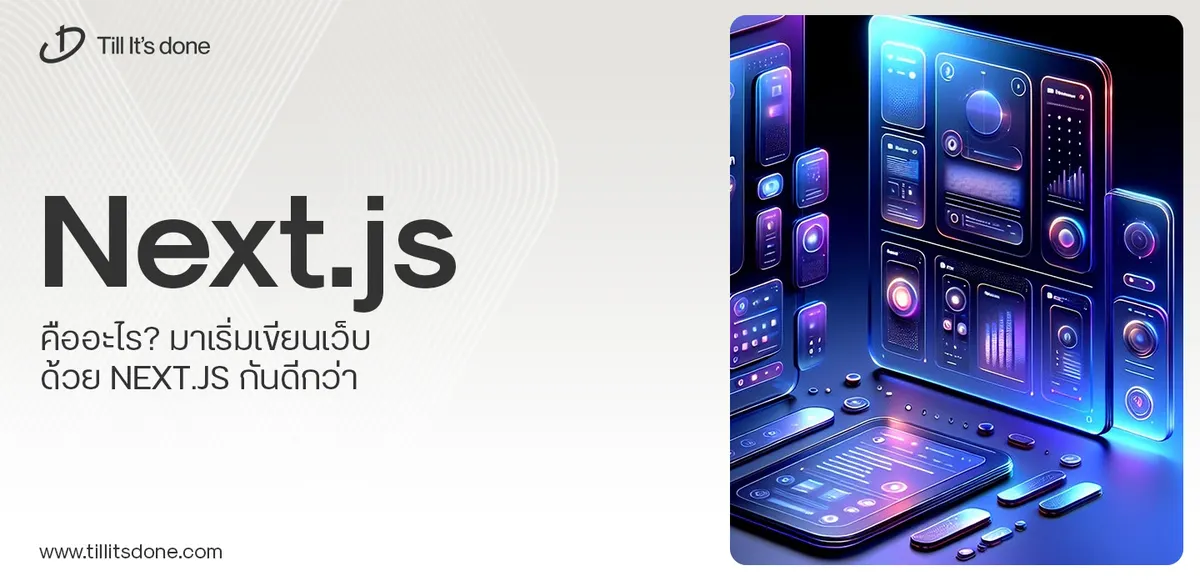
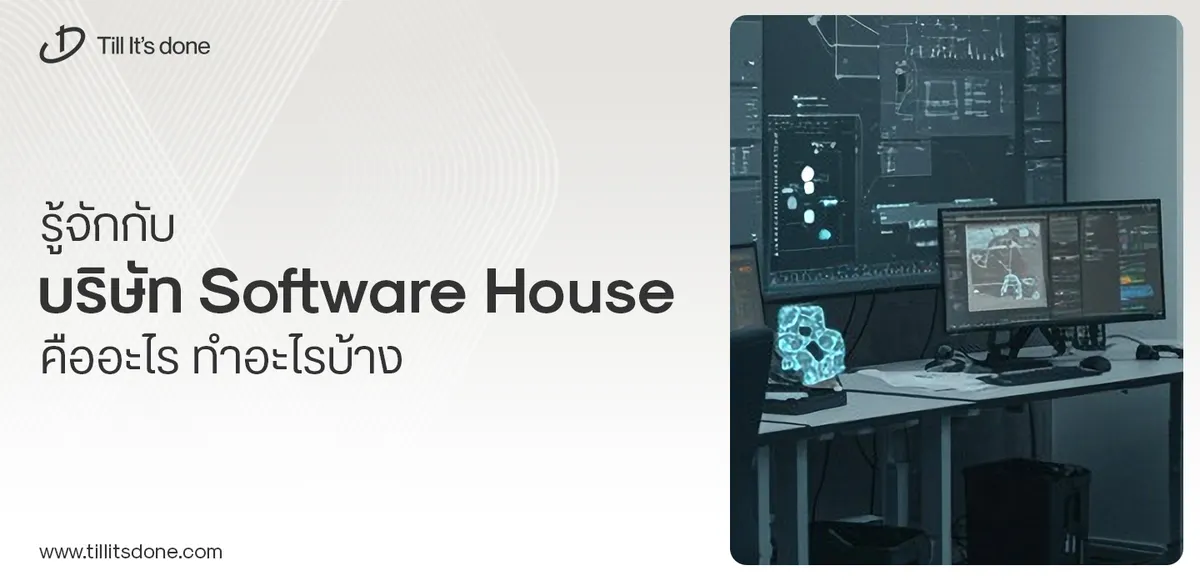
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.