- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Master React State with Context API Guide
Learn best practices, optimization techniques, and advanced patterns for building scalable applications.

Mastering React’s Context API: A Deep Dive into Modern State Management
The Context API has revolutionized how we handle state management in React applications, offering a clean and efficient alternative to prop drilling. Let’s explore how to leverage this powerful feature effectively.
Understanding the Basics
Remember the days of passing props through multiple component layers? We’ve all been there. The Context API swoops in like a superhero, providing a way to share values between components without explicitly passing props through every level of the component tree.
Think of Context as a broadcasting system in your React application. Instead of playing telephone with your data, you’re setting up a direct channel of communication between components.
Setting Up Context Like a Pro
Let’s break down the implementation process into digestible chunks. First, we create our context:
const ThemeContext = React.createContext();
Then, we set up our provider to wrap the components that need access to our shared state:
function ThemeProvider({ children }) { const [theme, setTheme] = useState('light');
return ( <ThemeContext.Provider value={{ theme, setTheme }}> {children} </ThemeContext.Provider> );}
Best Practices for Context Usage
Here’s something many developers overlook: Context isn’t just for global state. You can use multiple contexts for different concerns in your application. Think of it as having different radio frequencies for different types of messages.
Custom hooks make Context even more powerful. Create a dedicated hook for each context:
function useTheme() { const context = useContext(ThemeContext); if (context === undefined) { throw new Error('useTheme must be used within a ThemeProvider'); } return context;}
Performance Optimization Tips
One common pitfall is unnecessary re-renders. To avoid this, split your context into smaller, more focused pieces. Instead of one massive context storing everything, create separate contexts for different purposes.
Remember: Context is not a replacement for all state management. Use local state for component-specific data and Context for truly shared state.
Advanced Patterns
Consider implementing the Provider Pattern with composition:
function AppProviders({ children }) { return ( <ThemeProvider> <UserProvider> <SettingsProvider> {children} </SettingsProvider> </UserProvider> </ThemeProvider> );}
When to Use Context API
Context shines in scenarios like:
- Theme switching
- User authentication state
- Language preferences
- Feature flags
- Shopping cart state
However, for complex state management with frequent updates, consider combining Context with useReducer or exploring other solutions like Redux.
Remember, the Context API is a powerful tool in your React toolkit. Use it wisely, and it’ll help you build more maintainable and scalable applications. Happy coding!




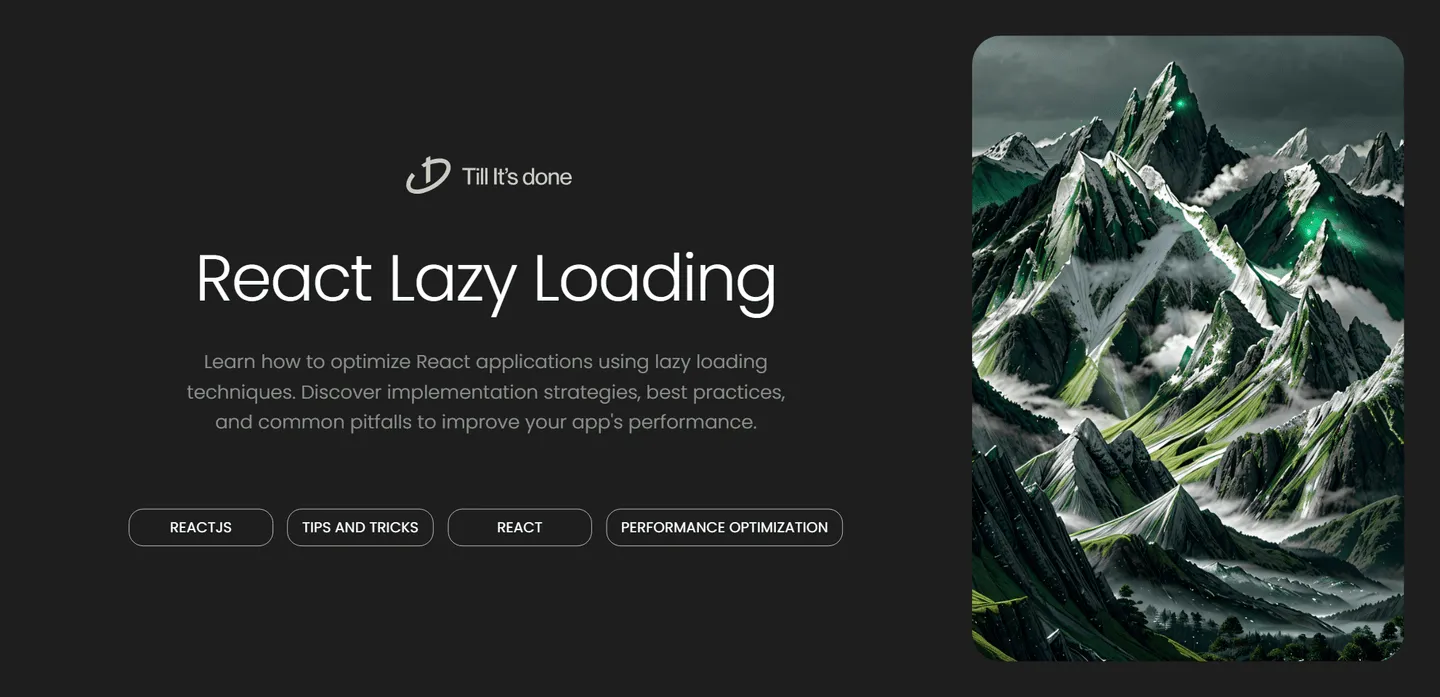

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.