- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Node.js Security Best Practices Guide 2024

How to Improve Node.js Security with Best Practices
Security should be a top priority when developing Node.js applications. Let’s explore essential practices to fortify your Node.js applications against common vulnerabilities and threats.
Keep Dependencies Updated and Secure
Regular maintenance of your project dependencies is crucial. Outdated packages often contain security vulnerabilities that malicious actors can exploit. Implement these practices:
- Use
npm audit
regularly to scan for known vulnerabilities - Keep your Node.js version up-to-date
- Implement automated security updates with tools like Dependabot
- Remove unused dependencies to minimize attack surface
Implement Proper Authentication and Authorization
Security begins with proper access control:
// Example using JSON Web Tokens (JWT)const jwt = require('jsonwebtoken');const secret = process.env.JWT_SECRET;
const createToken = (user) => { return jwt.sign({ id: user.id, role: user.role }, secret, { expiresIn: '24h' });};
Secure Your Environment Variables
Never expose sensitive information in your code:
- Use
.env
files for environment variables - Add
.env
to.gitignore
- Implement environment-specific configurations
- Use a secrets management service in production
Enable Security Headers
Implement security headers to protect against common web vulnerabilities:
const helmet = require('helmet');app.use(helmet());
Input Validation and Sanitization
Always validate and sanitize user input:
- Use validation libraries like Joi or express-validator
- Implement rate limiting
- Sanitize database queries
- Escape HTML to prevent XSS attacks
Monitor and Log Security Events
Implement comprehensive logging and monitoring:
- Use logging libraries like Winston or Morgan
- Set up automated alerts for suspicious activities
- Maintain audit logs for security-relevant events
- Implement error handling without exposing sensitive details
Remember that security is an ongoing process, not a one-time implementation. Regular security audits and staying informed about new vulnerabilities are essential for maintaining a robust Node.js application.




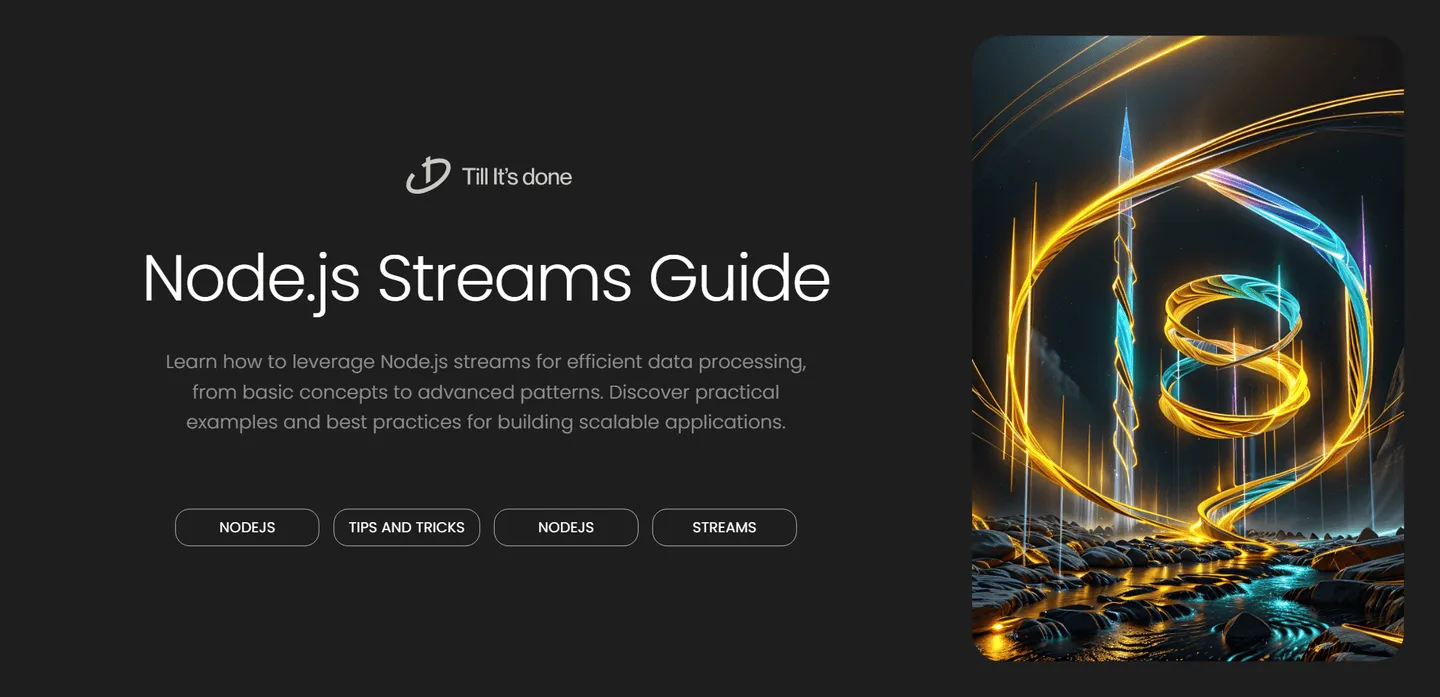

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.