- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Best Practices for Async Programming in Node.js
Learn about Promises, async/await patterns, error handling, parallel execution, and memory management for building efficient applications.
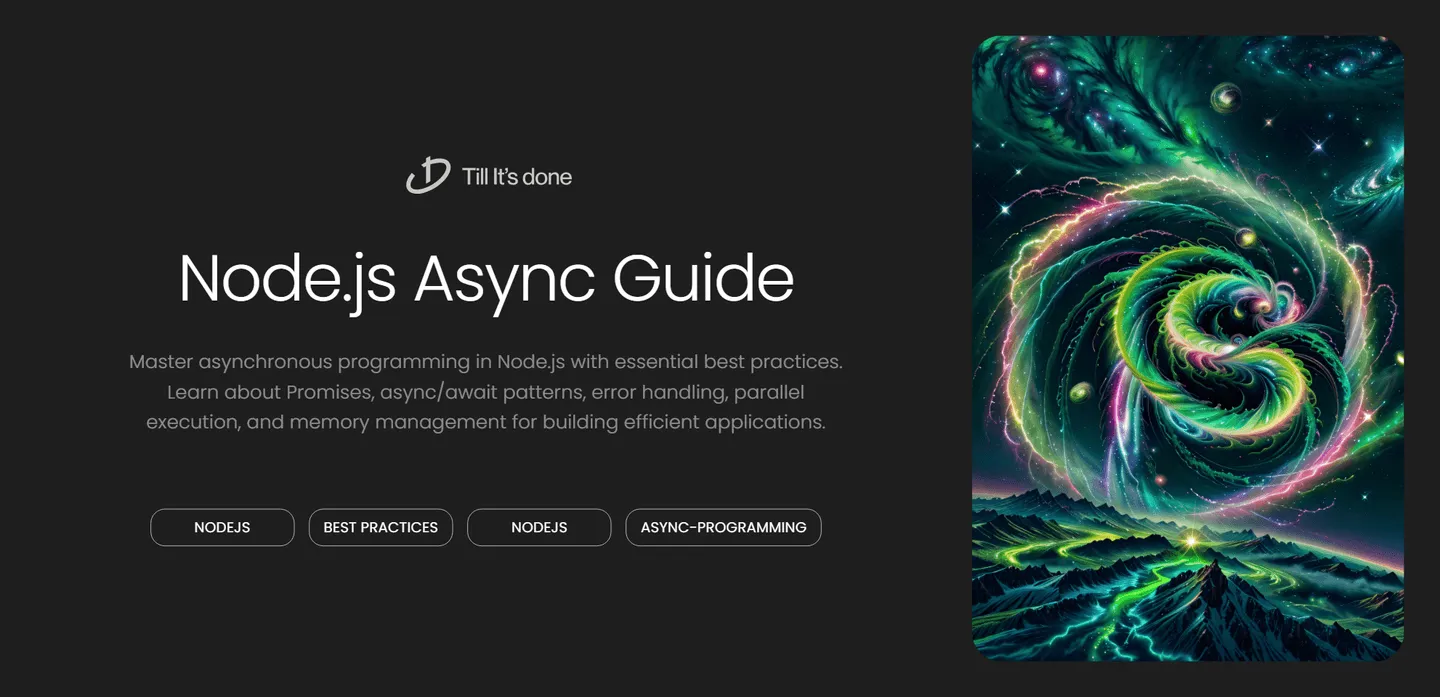
Best Practices for Asynchronous Programming in Node.js
Asynchronous programming is at the heart of Node.js, enabling non-blocking operations that keep your applications responsive and efficient. Let’s dive into the best practices that will help you write better async code.
Understanding Promises and Async/Await
The foundation of modern async programming in Node.js lies in Promises and the async/await syntax. Instead of callback hell, embrace these cleaner patterns:
// Instead of this:fetchUser(function(user) { getUserPosts(user, function(posts) { getPostComments(posts, function(comments) { // Nested callback hell }); });});
// Do this:async function getUserData() { const user = await fetchUser(); const posts = await getUserPosts(user); const comments = await getPostComments(posts); return { user, posts, comments };}
Error Handling Best Practices
Always implement proper error handling in your async operations. Use try-catch blocks with async/await and handle Promise rejections appropriately:
async function handleUserData() { try { const result = await processData(); return result; } catch (error) { logger.error('Data processing failed:', error); throw new CustomError('Failed to process user data'); }}
Parallel Execution
When operations don’t depend on each other, run them in parallel to improve performance:
// Run multiple independent operations simultaneouslyconst [users, products, categories] = await Promise.all([ fetchUsers(), fetchProducts(), fetchCategories()]);
Memory Management and Performance
- Use streaming for large data operations
- Implement proper cleanup in async operations
- Avoid memory leaks by properly closing connections
- Implement circuit breakers for external service calls
Rate Limiting and Queuing
Implement rate limiting for API calls and use queues for heavy operations:
const queue = new Queue({ concurrency: 2, timeout: 5000});
queue.on('error', handleError);queue.add(() => processTask());
Remember, good async programming isn’t just about making code work - it’s about making it work efficiently, reliably, and maintainably. By following these practices, you’ll create more robust Node.js applications that can handle real-world challenges effectively.
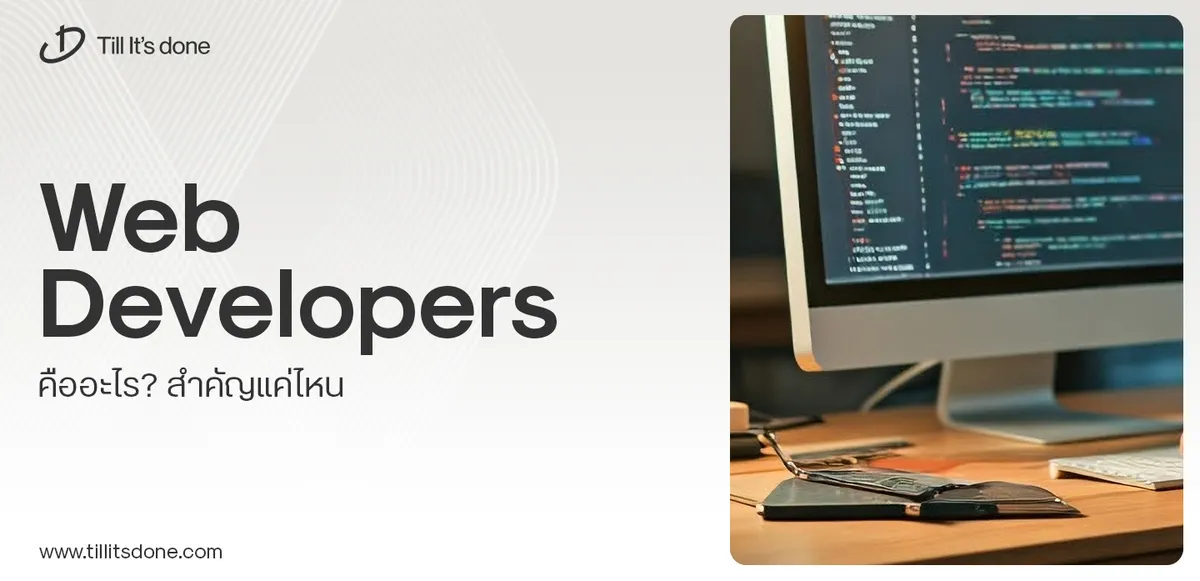
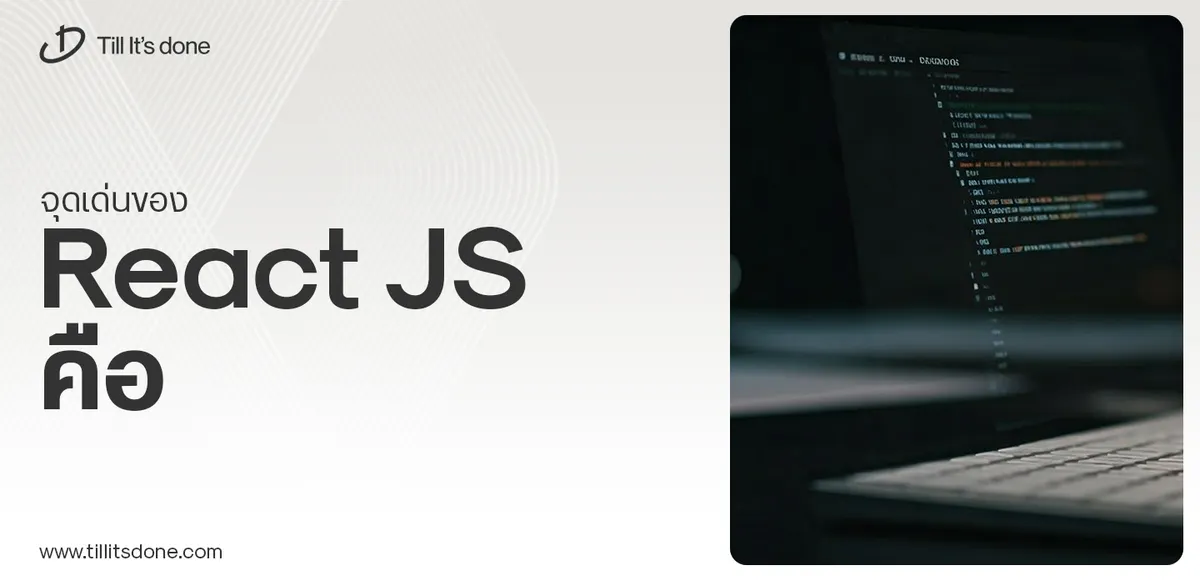
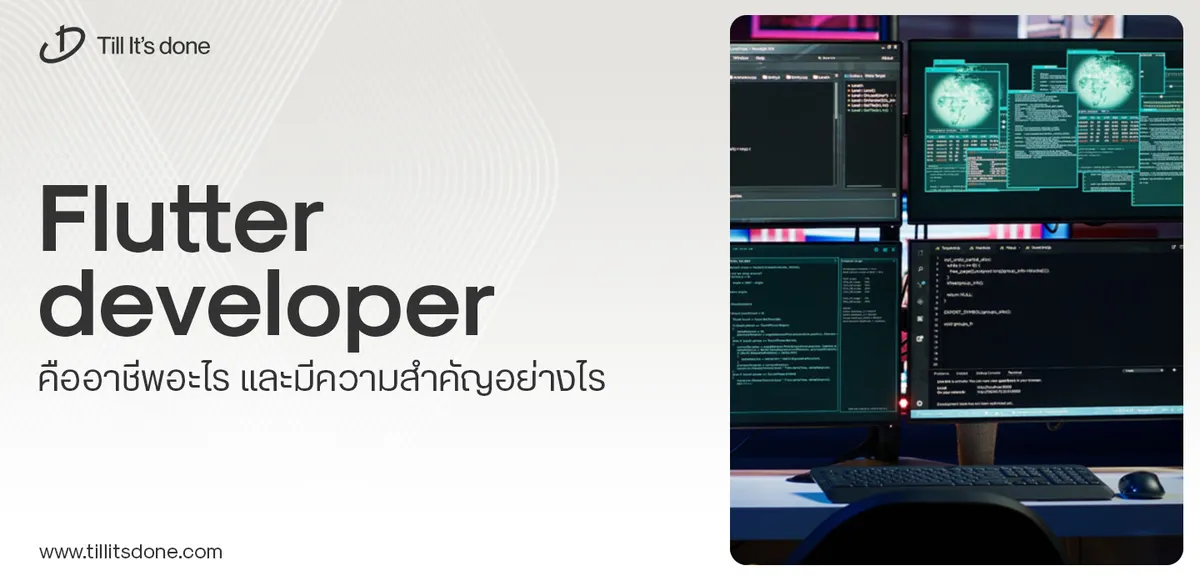
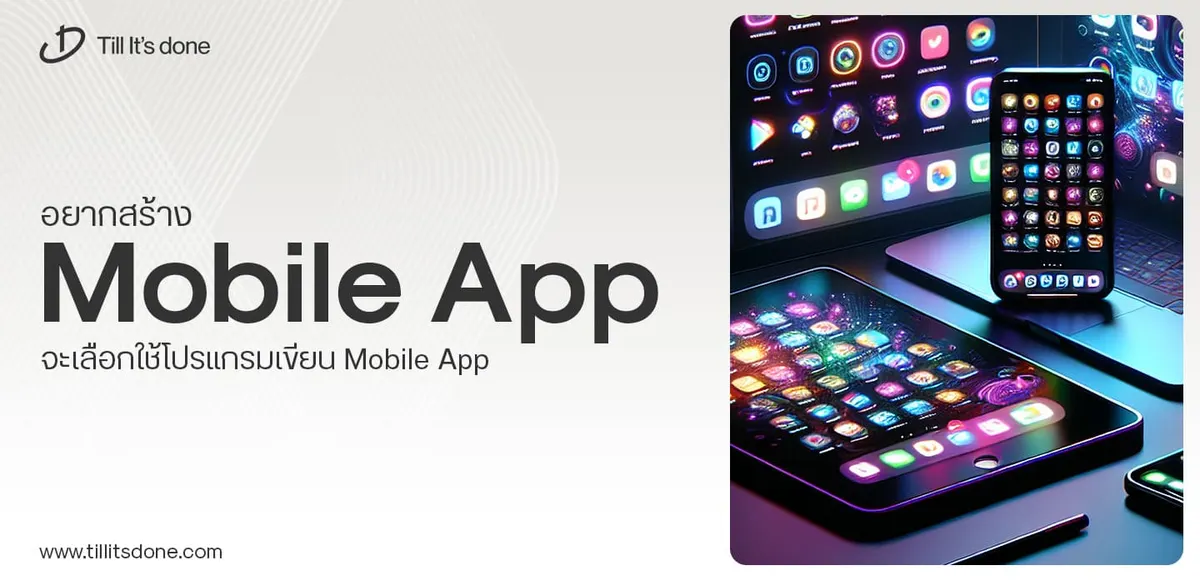
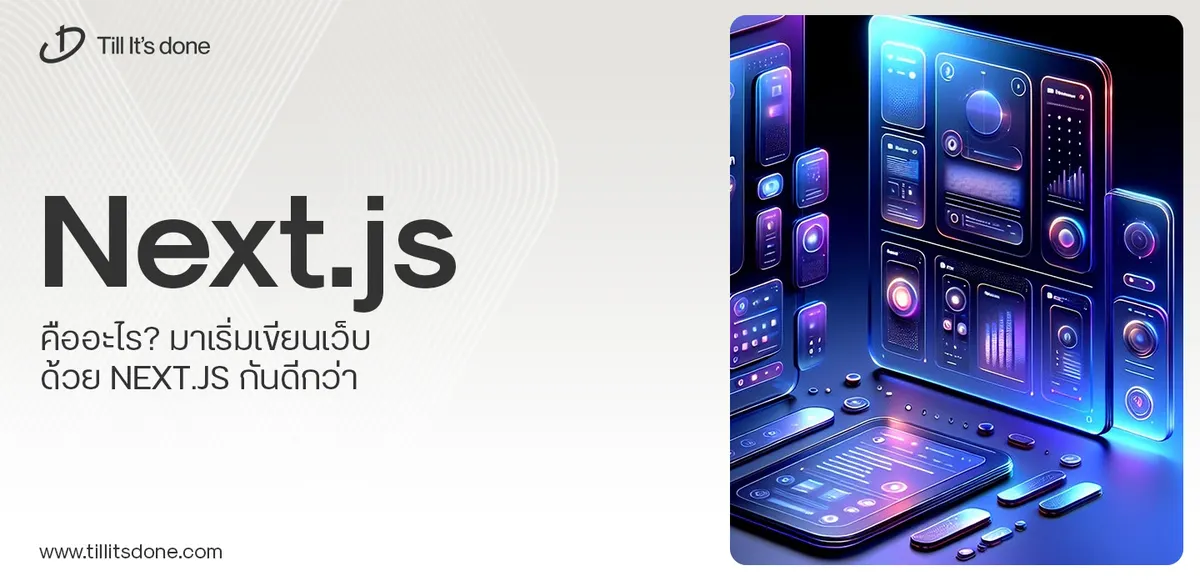
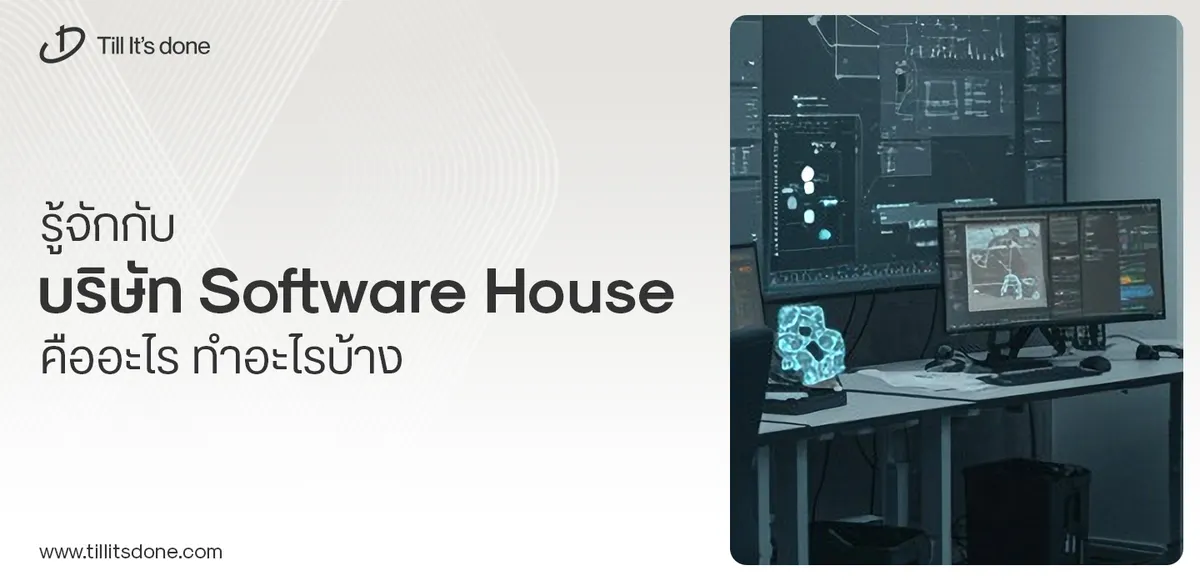
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.