- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Build Real-Time Apps with Next.js WebSockets
This guide covers setup, implementation, and advanced patterns for building modern interactive applications.
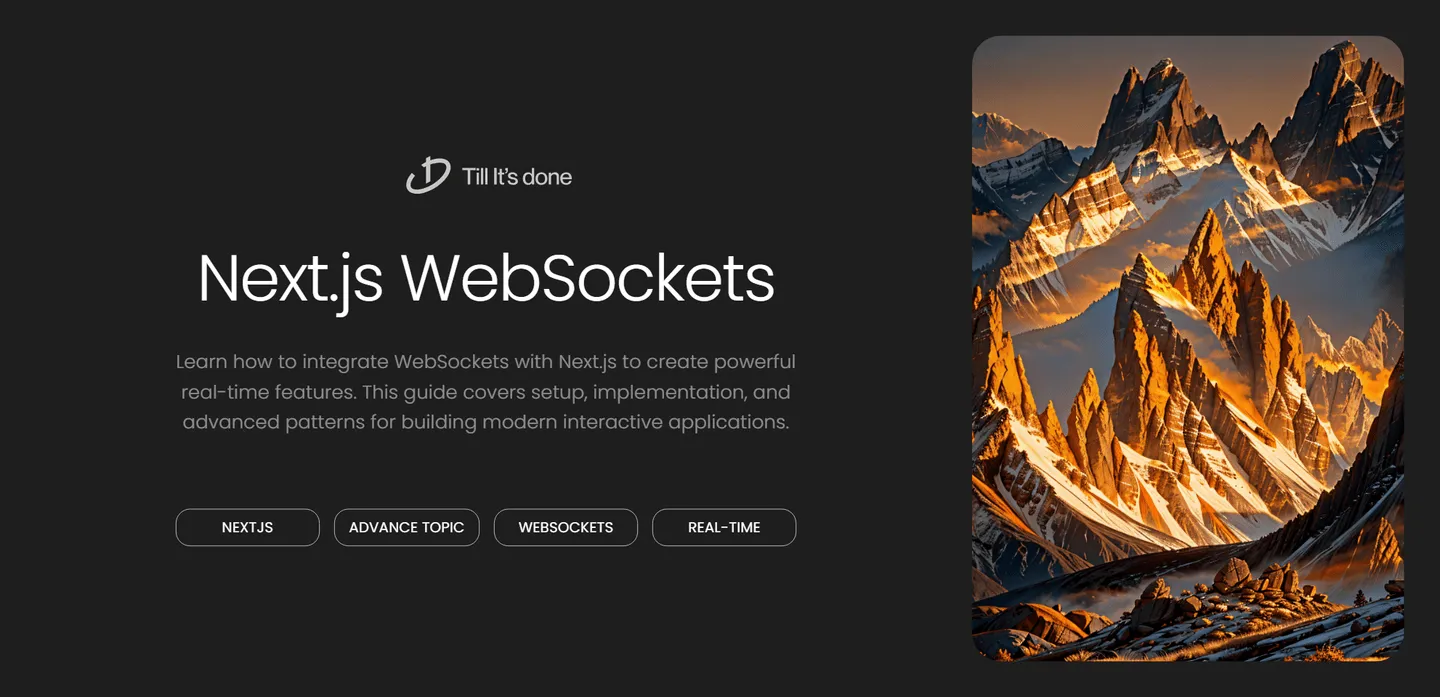
Integrating WebSockets with Next.js for Real-Time Features
In today’s fast-paced digital world, real-time features have become essential for modern web applications. Whether you’re building a chat system, live notifications, or collaborative tools, WebSocket integration in Next.js can elevate your application to the next level. Let’s dive deep into implementing WebSockets in your Next.js project.
Understanding WebSockets in Next.js
WebSockets create a persistent connection between the client and server, enabling bi-directional communication. Unlike traditional HTTP requests, WebSockets maintain an open connection, making them perfect for real-time updates and live features.
Setting Up WebSocket Server
First, let’s set up a WebSocket server using the ws
package. Create a new API route in your Next.js project under pages/api/websocket.js
:
import { Server } from 'ws'
const socketHandler = (req, res) => { if (!res.socket.server.ws) { const wss = new Server({ noServer: true })
wss.on('connection', socket => { socket.on('message', message => { wss.clients.forEach(client => { client.send(message.toString()) }) }) })
res.socket.server.ws = wss }
res.end()}
export default socketHandler
Implementing Client-Side Connection
To connect from the client side, we’ll create a custom hook for managing WebSocket connections:
const useWebSocket = () => { const [socket, setSocket] = useState(null) const [messages, setMessages] = useState([])
useEffect(() => { const ws = new WebSocket(`${process.env.NEXT_PUBLIC_WS_URL}`)
ws.onmessage = (event) => { setMessages(prev => [...prev, event.data]) }
setSocket(ws)
return () => ws.close() }, [])
return { socket, messages }}
Building Real-Time Features
Let’s implement a real-time chat feature using our WebSocket setup:
export default function Chat() { const { socket, messages } = useWebSocket() const [message, setMessage] = useState('')
const sendMessage = () => { if (socket && message) { socket.send(message) setMessage('') } }
return ( <div> <div className="messages"> {messages.map((msg, index) => ( <div key={index}>{msg}</div> ))} </div> <input value={message} onChange={(e) => setMessage(e.target.value)} /> <button onClick={sendMessage}>Send</button> </div> )}
Advanced WebSocket Patterns
Handling Reconnection
Implement a reconnection strategy to maintain connection stability:
const reconnect = (ws, url, attempts = 0) => { setTimeout(() => { if (attempts < 5) { const newWs = new WebSocket(url) newWs.onerror = () => reconnect(newWs, url, attempts + 1) return newWs } }, Math.min(1000 * Math.pow(2, attempts), 10000))}
Room-Based Communications
Implement rooms for grouped communications:
const joinRoom = (roomId) => { if (socket) { socket.send(JSON.stringify({ type: 'JOIN_ROOM', roomId })) }}
Remember to handle WebSocket cleanup properly in your components and implement error handling for production environments. WebSockets can significantly enhance your application’s real-time capabilities, but proper implementation and maintenance are crucial for optimal performance.
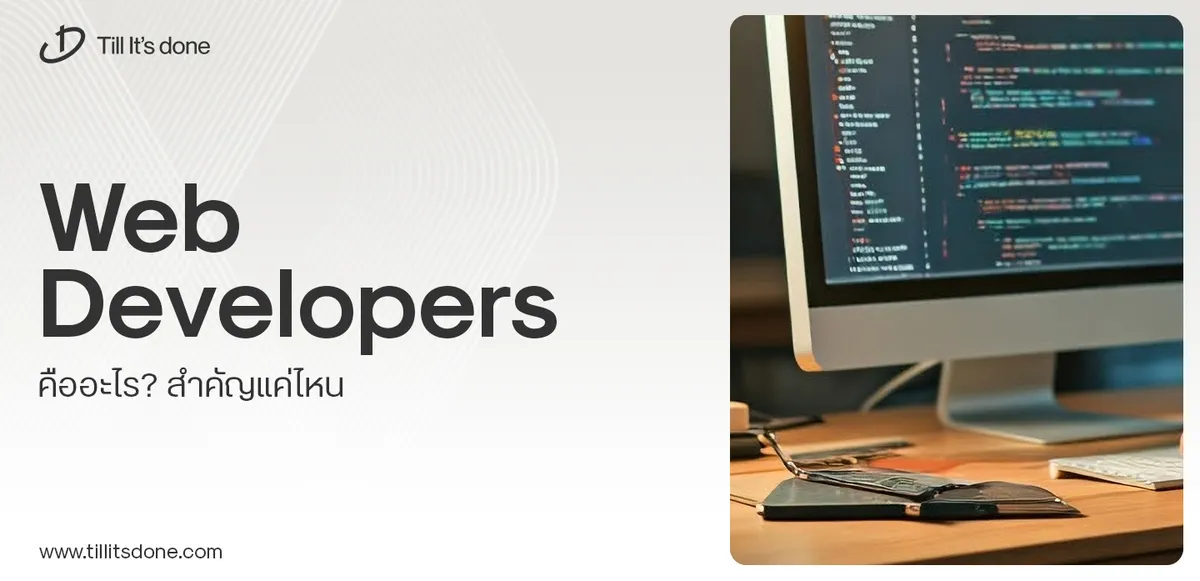
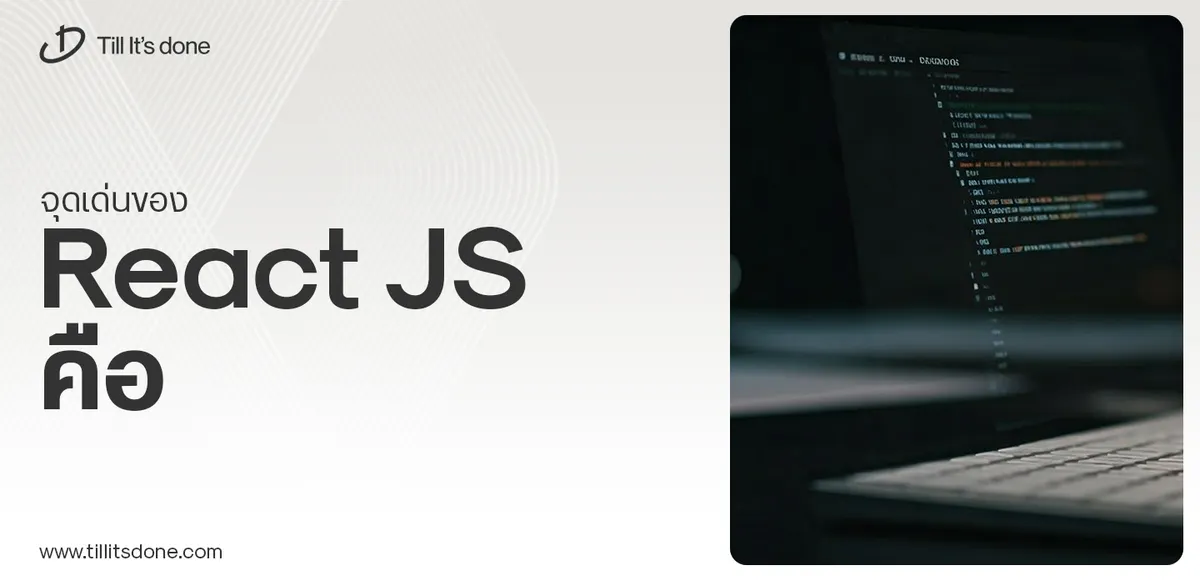
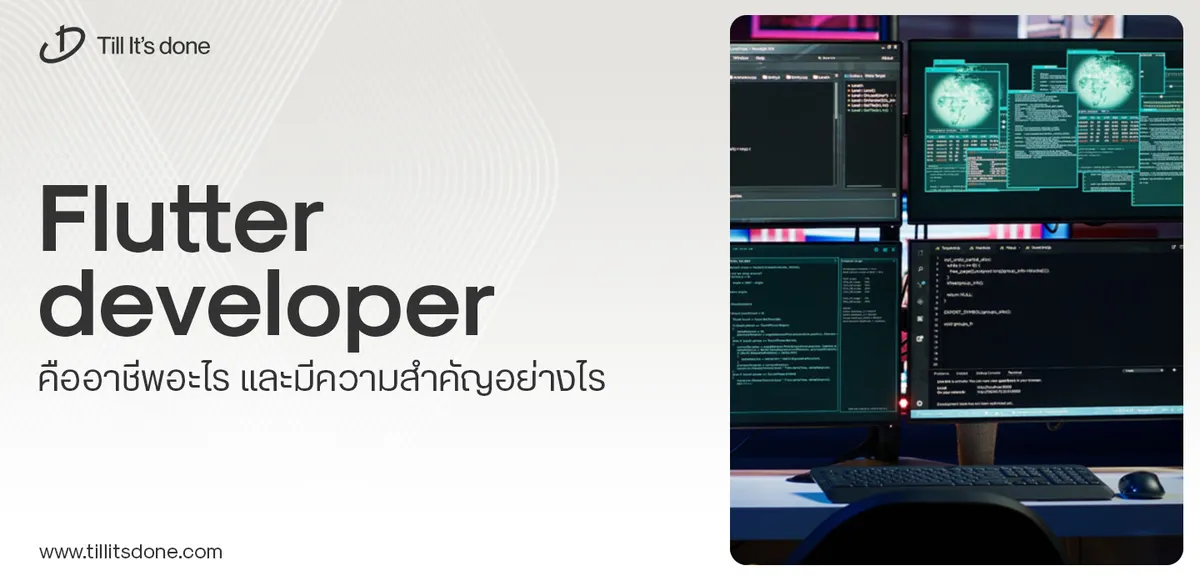
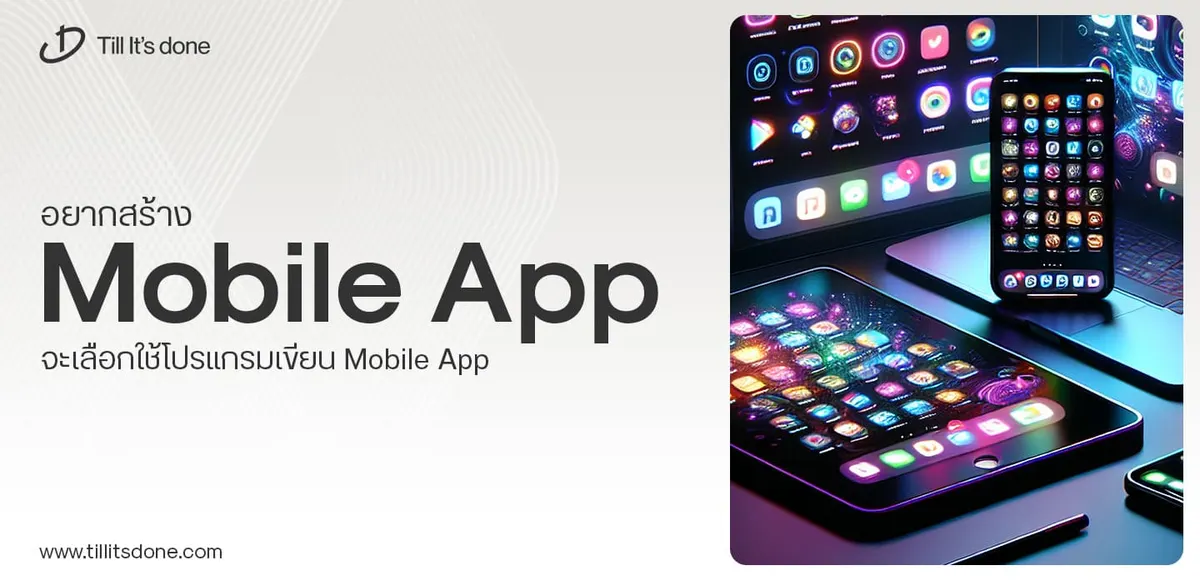
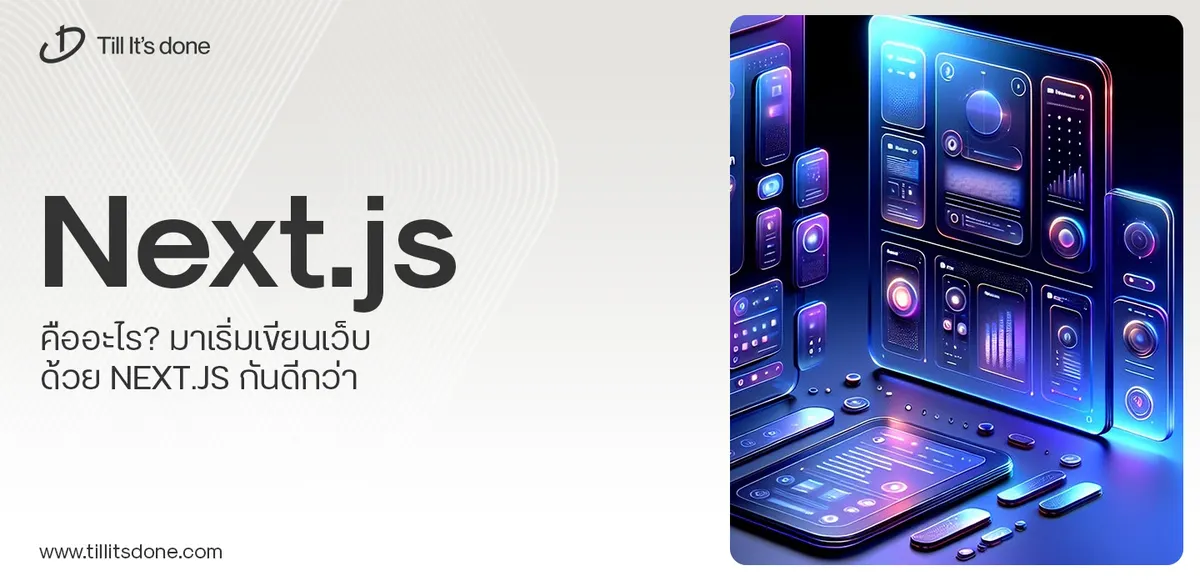
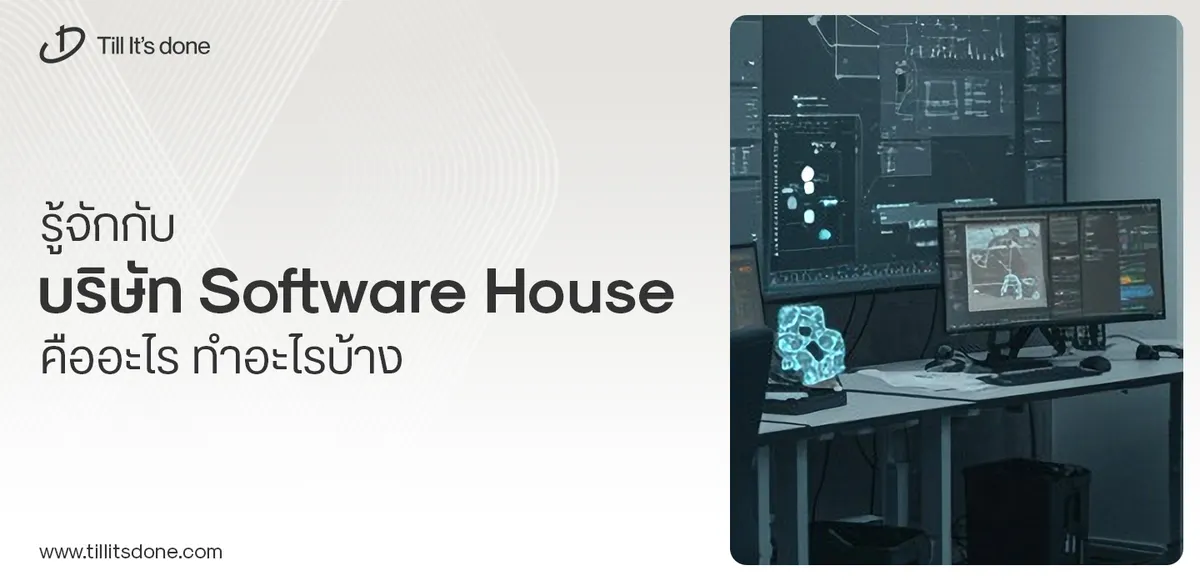
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.