- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
How to Mock Dependencies in Bloc Tests
This guide covers setup, basic mocking, best practices, and advanced scenarios to help you write better tests for your Flutter applications.

How to Mock Dependencies in Bloc Tests
Testing is a crucial part of developing robust Flutter applications, especially when working with the BLoC pattern. Today, let’s dive into how to effectively mock dependencies in Bloc tests to ensure your code is working as expected.
Understanding the Importance of Mocking
When testing Blocs, we often need to simulate various dependencies like repositories, API clients, or other services. Mocking these dependencies allows us to test our Bloc’s logic in isolation and create predictable test scenarios.
Setting Up Your Test Environment
First, let’s look at what we need to get started. Make sure you have the following dependencies in your pubspec.yaml
:
dev_dependencies: bloc_test: ^9.0.0 mockito: ^5.0.0 build_runner: ^2.0.0
Creating a Mock Repository
Let’s say we have a UserBloc that depends on a UserRepository. Here’s how we can create a mock:
class MockUserRepository extends Mock implements UserRepository {}
Writing Your First Mock Test
Here’s a practical example of how to test a UserBloc with a mocked repository:
void main() { late UserBloc userBloc; late MockUserRepository mockUserRepository;
setUp(() { mockUserRepository = MockUserRepository(); userBloc = UserBloc(repository: mockUserRepository); });
blocTest<UserBloc, UserState>( 'emits [UserLoading, UserLoaded] when FetchUser is added', build: () { when(mockUserRepository.getUser()) .thenAnswer((_) async => User(id: '1', name: 'John')); return userBloc; }, act: (bloc) => bloc.add(FetchUser()), expect: () => [ isA<UserLoading>(), isA<UserLoaded>(), ], );}
Best Practices for Mocking
- Keep your mocks simple and focused
- Test both success and failure scenarios
- Verify mock interactions when necessary
- Use meaningful test descriptions
- Group related tests together
Remember that good tests should be readable, maintainable, and provide value by catching potential issues before they reach production.
Advanced Mocking Techniques
Sometimes you’ll need to mock more complex scenarios. Here’s how to handle them:
test('handles complex mock interactions', () { when(mockRepository.getData()) .thenAnswer((_) async => 'first call') .thenAnswer((_) async => 'second call');
verify(mockRepository.getData()).called(2);});
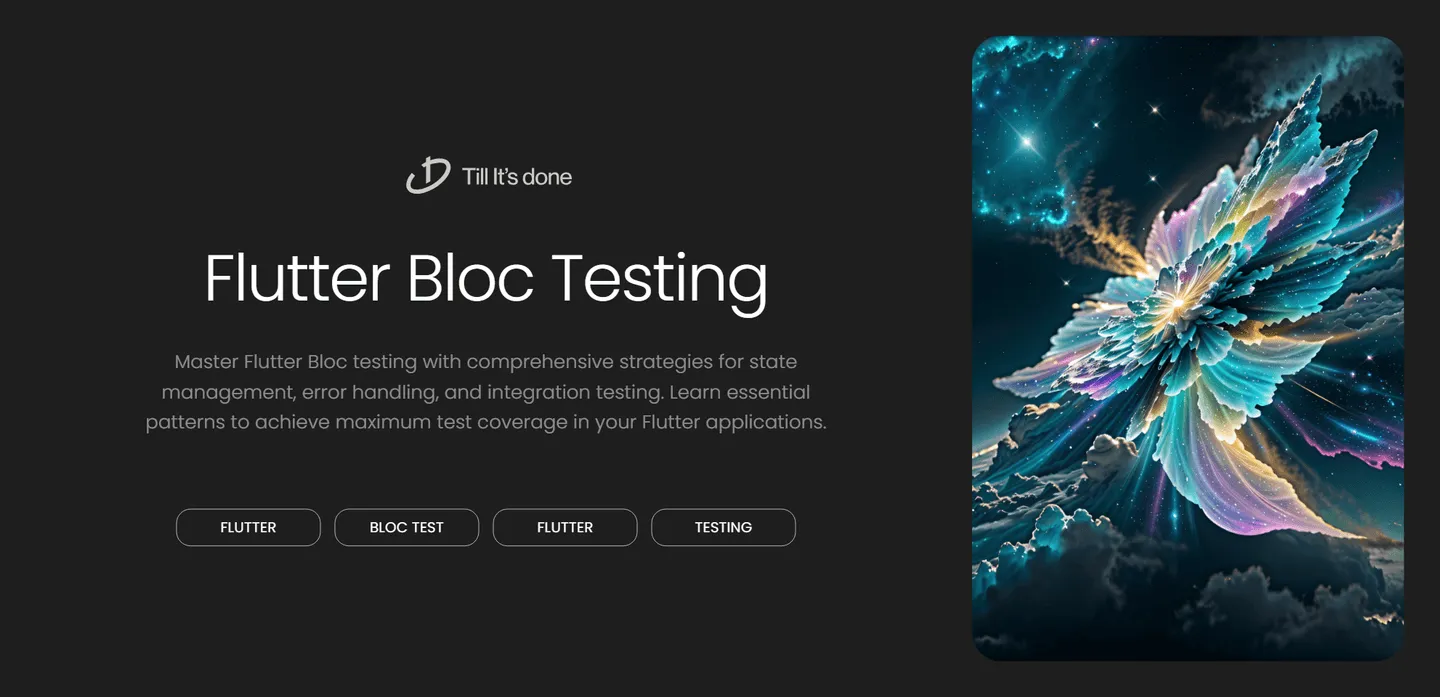





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.