- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Jest with React Testing Library Guide
This guide covers setup, best practices, common patterns, and debugging tips for reliable testing.
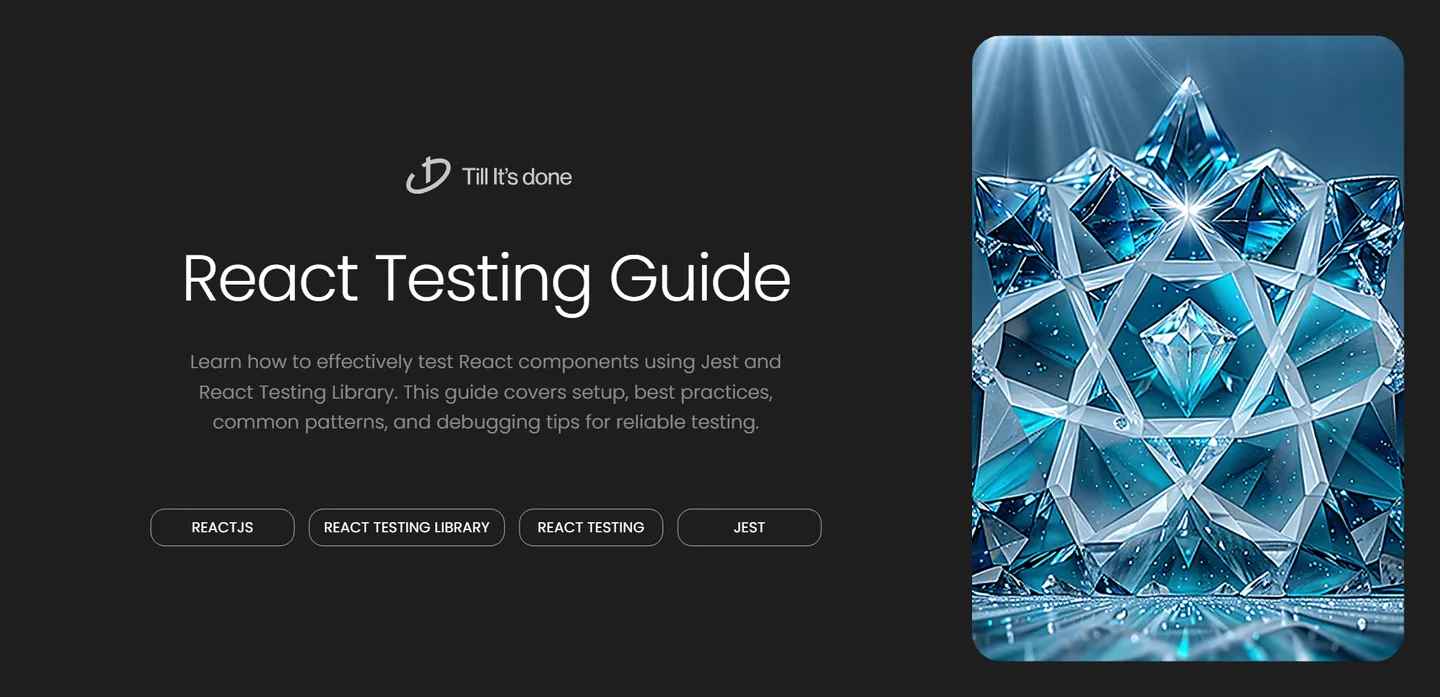
How to Use Jest with React Testing Library for Effective Testing
Testing is a crucial aspect of modern web development, and when it comes to React applications, the combination of Jest and React Testing Library provides a robust testing solution. In this guide, we’ll explore how to effectively use these tools together to create maintainable and reliable tests for your React components.
Getting Started with Jest and React Testing Library
If you’re using Create React App, you’re in luck – Jest and React Testing Library come pre-configured. For other setups, you’ll need to install the necessary dependencies:
npm install --save-dev @testing-library/react @testing-library/jest-dom jest
Understanding the Testing Philosophy
React Testing Library promotes testing your applications in a way that resembles how users interact with your app. Instead of testing implementation details, we focus on testing behavior. This approach leads to more reliable and maintainable tests.
Key Principles:
The more your tests resemble how your software is used, the more confidence they can provide. This means:
- Query elements by accessibility roles, labels, and text content
- Interact with elements as users would
- Focus on testing behavior, not implementation
Writing Your First Test
Let’s look at a practical example of testing a simple counter component:
import { render, screen, fireEvent } from '@testing-library/react';import Counter from './Counter';
test('counter increments when increment button is clicked', () => { render(<Counter />);
const incrementButton = screen.getByRole('button', { name: /increment/i }); const countDisplay = screen.getByText(/count:/i);
fireEvent.click(incrementButton);
expect(countDisplay).toHaveTextContent('Count: 1');});
Best Practices for Testing React Components
-
Use the Right Queries The React Testing Library provides several ways to query elements. Here’s the recommended priority order:
- getByRole
- getByLabelText
- getByPlaceholderText
- getByText
- getByDisplayValue
-
Test User Interactions
test('form submission works correctly', async () => {render(<LoginForm />);await userEvent.type(screen.getByLabelText(/username/i), 'testuser');await userEvent.type(screen.getByLabelText(/password/i), 'password123');await userEvent.click(screen.getByRole('button', { name: /submit/i }));expect(await screen.findByText(/welcome/i)).toBeInTheDocument();}); -
Testing Async Operations When testing asynchronous operations, use the
findBy
queries instead ofgetBy
:test('loads data successfully', async () => {render(<DataComponent />);expect(await screen.findByText(/loading/i)).toBeInTheDocument();expect(await screen.findByText(/data loaded/i)).toBeInTheDocument();});
Common Testing Patterns
Testing User Events
import userEvent from '@testing-library/user-event';
test('input update works correctly', async () => { const user = userEvent.setup(); render(<TextInput />);
await user.type(screen.getByRole('textbox'), 'Hello'); expect(screen.getByRole('textbox')).toHaveValue('Hello');});
Testing Error States
test('shows error message on invalid input', async () => { render(<Form />);
await userEvent.click(screen.getByRole('button', { name: /submit/i }));
expect(screen.getByRole('alert')).toHaveTextContent(/required field/i);});
Debugging Tips
When tests fail, React Testing Library provides helpful debugging tools:
screen.debug(); // Prints the current DOM state
Use the testing-playground
Chrome extension to help you find the right queries for your elements.
Conclusion
Jest and React Testing Library form a powerful combination for testing React applications. By following these patterns and best practices, you can create a robust test suite that gives you confidence in your application’s behavior. Remember to focus on testing from the user’s perspective and avoid testing implementation details.
Start small, test the critical paths first, and gradually build up your test coverage. With consistent practice, writing effective tests will become second nature, leading to more reliable and maintainable React applications.
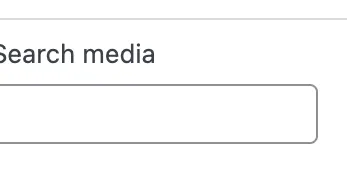





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.