- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
GraphQL Subscriptions in Node.js: Real-Time Data
Explore setup, best practices, error handling, and scaling considerations for building reactive applications.
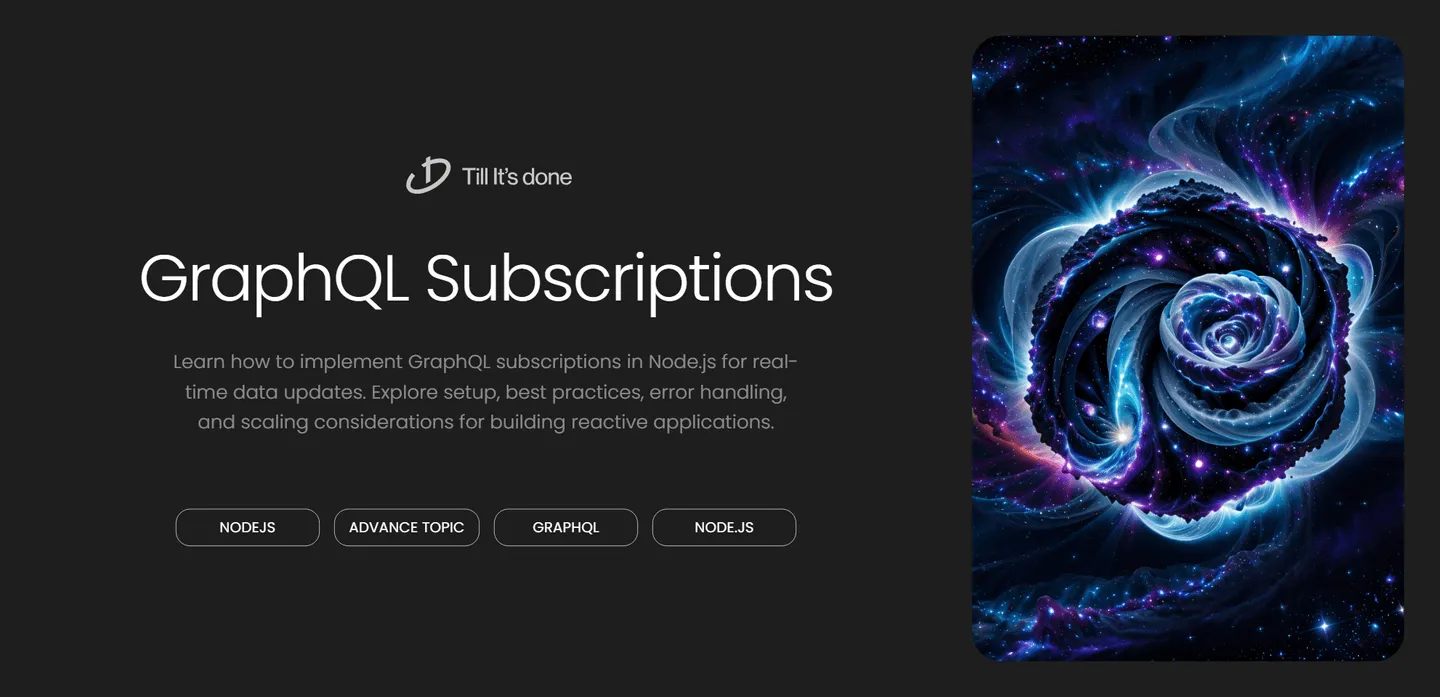
Implementing GraphQL Subscriptions in Node.js for Real-Time Data
In today’s fast-paced digital world, real-time data updates have become crucial for modern applications. GraphQL subscriptions provide an elegant solution for implementing real-time functionality in your Node.js applications. Let’s dive deep into how we can implement this powerful feature.
Understanding GraphQL Subscriptions
Think of GraphQL subscriptions as a persistent connection between the client and server – like having a dedicated phone line where the server can instantly notify you about specific events. Unlike queries and mutations, subscriptions maintain an open connection, enabling real-time data updates.
Setting Up the Environment
First, let’s set up our Node.js project with the necessary dependencies:
npm initnpm install graphql graphql-yoga graphql-subscriptions
Implementing the Subscription Server
Here’s how we can create a basic subscription server:
const { createServer } = require('graphql-yoga')const { PubSub } = require('graphql-subscriptions')
const pubsub = new PubSub()
const typeDefs = ` type Message { id: ID! content: String! timestamp: String! }
type Query { messages: [Message!] }
type Mutation { postMessage(content: String!): Message! }
type Subscription { newMessage: Message! }`
const messages = []
const resolvers = { Query: { messages: () => messages }, Mutation: { postMessage: (_, { content }) => { const message = { id: messages.length + 1, content, timestamp: new Date().toISOString() } messages.push(message) pubsub.publish('NEW_MESSAGE', { newMessage: message }) return message } }, Subscription: { newMessage: { subscribe: () => pubsub.asyncIterator(['NEW_MESSAGE']) } }}
const server = createServer({ schema: { typeDefs, resolvers }})
server.start()
Best Practices and Optimization
- Handle Connection Lifecycle: Always implement proper cleanup when clients disconnect.
- Implement Filtering: Allow clients to subscribe only to relevant data.
- Add Authentication: Secure your subscriptions with proper authentication.
- Manage Memory: Be mindful of memory usage with many concurrent connections.
Error Handling
Implement robust error handling to maintain connection stability:
const onConnect = (connectionParams) => { if (!isValid(connectionParams)) { throw new Error('Invalid connection parameters') } return true}
const onDisconnect = (webSocket) => { console.log('Client disconnected') // Cleanup logic here}
Scaling Considerations
For production environments, consider using Redis or other pub/sub systems to handle subscriptions across multiple server instances. This ensures reliable message delivery in distributed systems.
Remember, while GraphQL subscriptions are powerful, they should be used judiciously. Not every real-time update needs a subscription – sometimes polling or regular queries might be more appropriate for your use case.
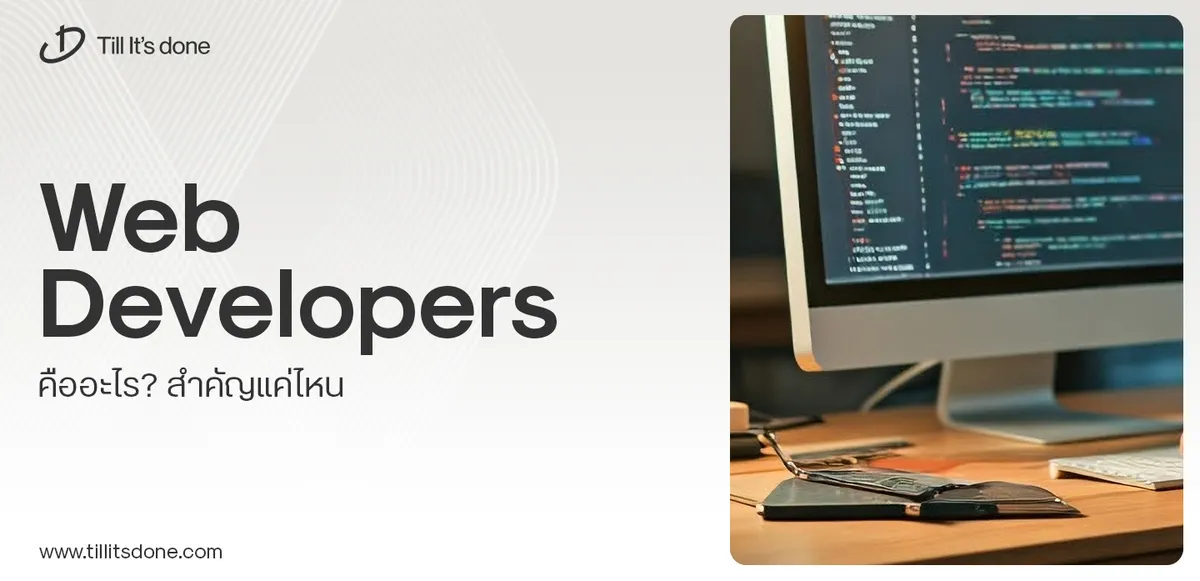
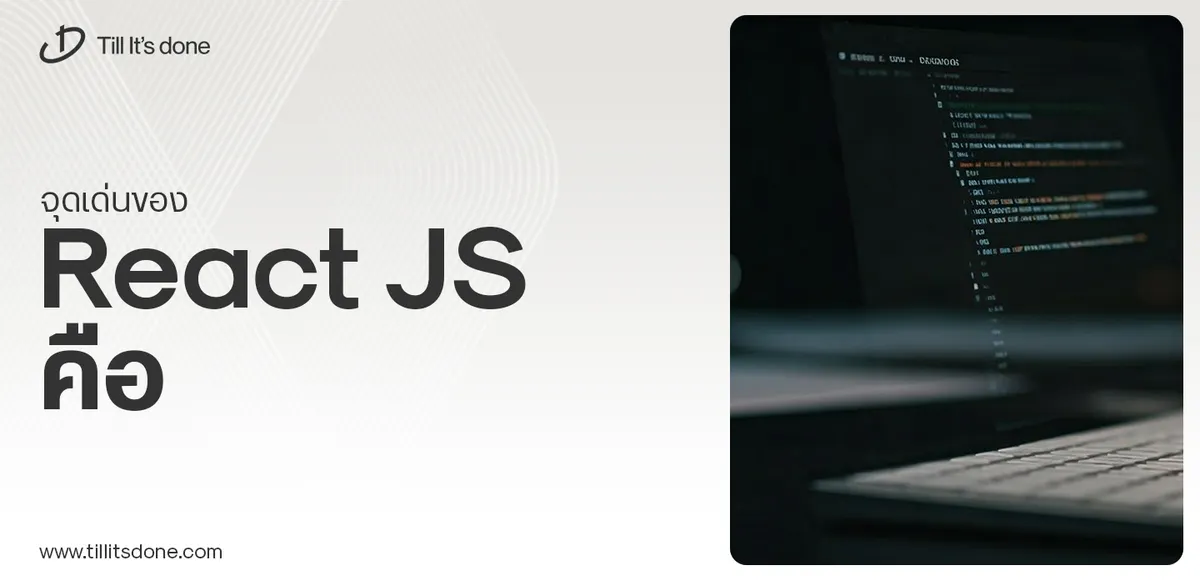
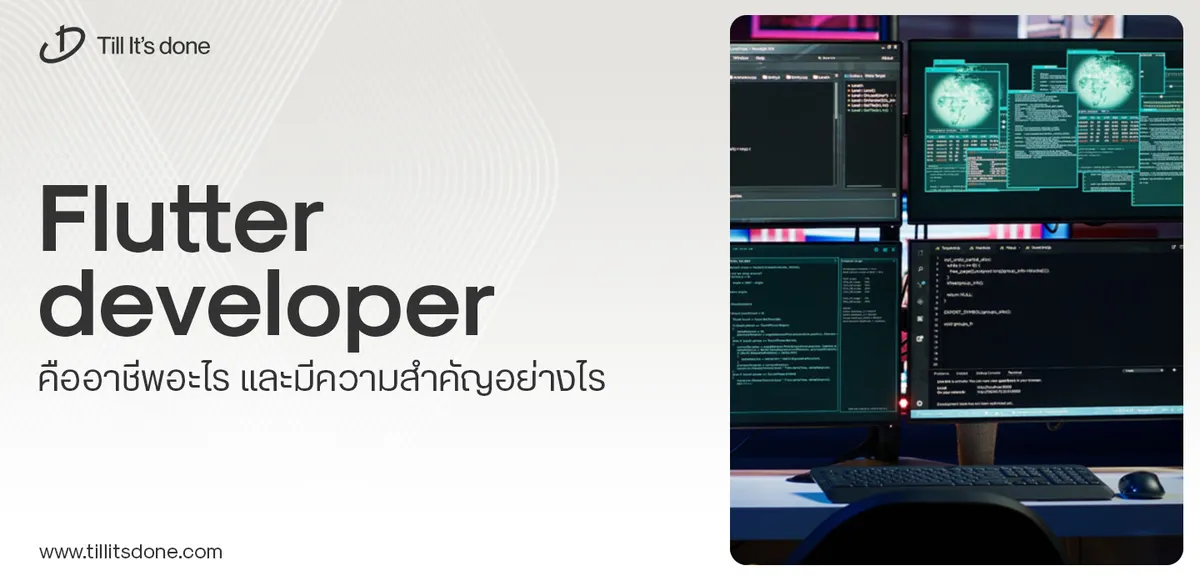
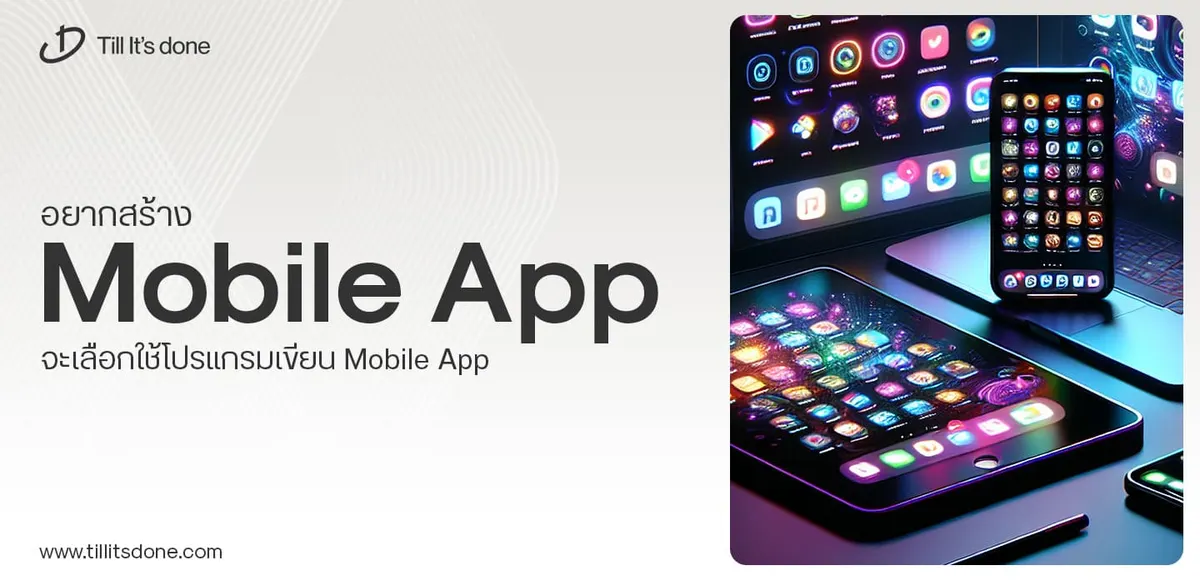
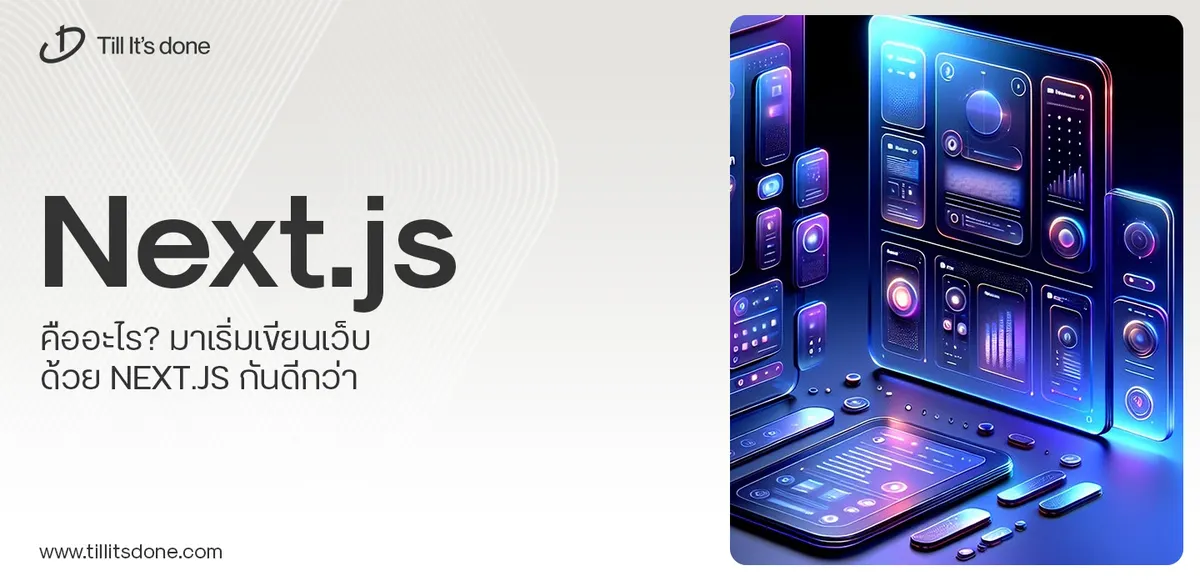
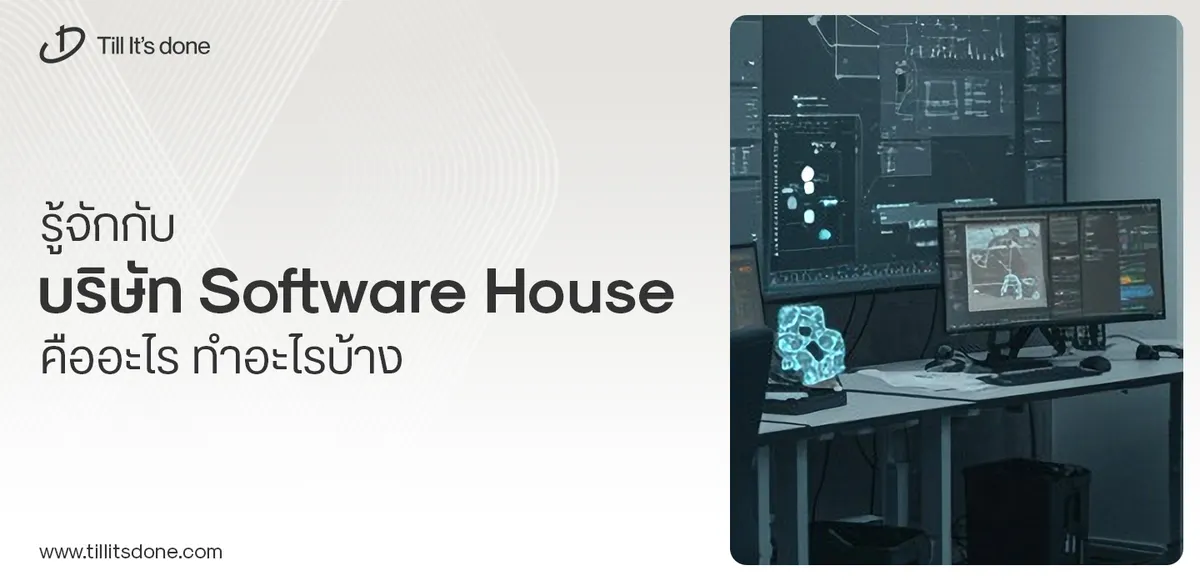
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.