- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Firebase Storage Image Upload Guide for Flutter
This guide covers setup, implementation, best practices, and security considerations.
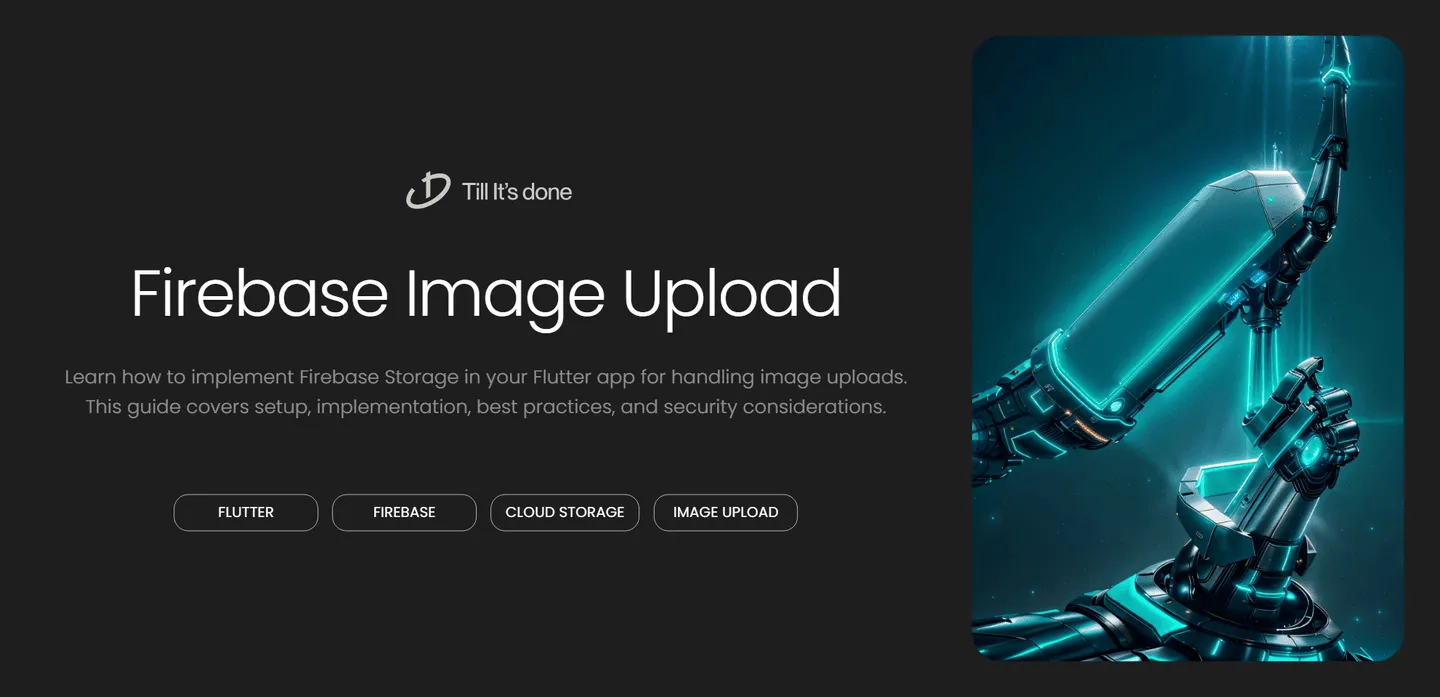
How to Use Firebase Storage for Image Uploads in Flutter
Managing image uploads is a crucial feature in many modern apps. Whether you’re building a social media platform, an e-commerce app, or a simple photo-sharing service, Firebase Storage provides a robust solution for handling file uploads in Flutter applications. Let’s dive into how you can implement this functionality in your Flutter app.
Getting Started
Before we jump into the code, make sure you have Firebase properly set up in your Flutter project. This includes adding the necessary dependencies and initializing Firebase in your app.
First, you’ll need to add these dependencies to your pubspec.yaml
:
dependencies: firebase_core: ^latest_version firebase_storage: ^latest_version image_picker: ^latest_version
Implementing Image Upload
The process of uploading images to Firebase Storage can be broken down into three main steps:
- Selecting an image from the device
- Uploading it to Firebase Storage
- Getting the download URL
Let’s start by picking an image:
Future<void> pickImage() async { final ImagePicker _picker = ImagePicker(); final XFile? image = await _picker.pickImage(source: ImageSource.gallery);
if (image != null) { // Process the image await uploadImage(File(image.path)); }}
Now, let’s implement the upload functionality:
Future<String> uploadImage(File imageFile) async { // Create a unique file name String fileName = DateTime.now().millisecondsSinceEpoch.toString();
// Create a reference to the file location Reference ref = FirebaseStorage.instance.ref().child('images/$fileName');
// Start the upload task UploadTask uploadTask = ref.putFile(imageFile);
// Wait for the upload to complete and get the download URL TaskSnapshot taskSnapshot = await uploadTask; String downloadURL = await taskSnapshot.ref.getDownloadURL();
return downloadURL;}
Best Practices and Tips
- Always handle errors properly using try-catch blocks
- Implement upload progress indicators for better user experience
- Consider implementing image compression before upload
- Set up proper Firebase Storage security rules
- Cache downloaded images for better performance
Here’s a more complete example with error handling and progress tracking:
Future<void> uploadWithProgress(File imageFile) async { try { String fileName = DateTime.now().millisecondsSinceEpoch.toString(); Reference ref = FirebaseStorage.instance.ref().child('images/$fileName'); UploadTask uploadTask = ref.putFile(imageFile);
uploadTask.snapshotEvents.listen((TaskSnapshot snapshot) { double progress = (snapshot.bytesTransferred / snapshot.totalBytes) * 100; print('Upload progress: $progress%'); });
await uploadTask; String downloadURL = await ref.getDownloadURL(); print('Upload complete! URL: $downloadURL'); } catch (e) { print('Error uploading image: $e'); }}
Security Considerations
Remember to set up proper security rules in your Firebase Console. Here’s a basic example:
rules_version = '2';service firebase.storage { match /b/{bucket}/o { match /{allPaths=**} { allow read: if request.auth != null; allow write: if request.auth != null && request.resource.size < 5 * 1024 * 1024; // 5MB limit } }}
By following these steps and implementing proper error handling and security measures, you’ll have a robust image upload system in your Flutter application using Firebase Storage.
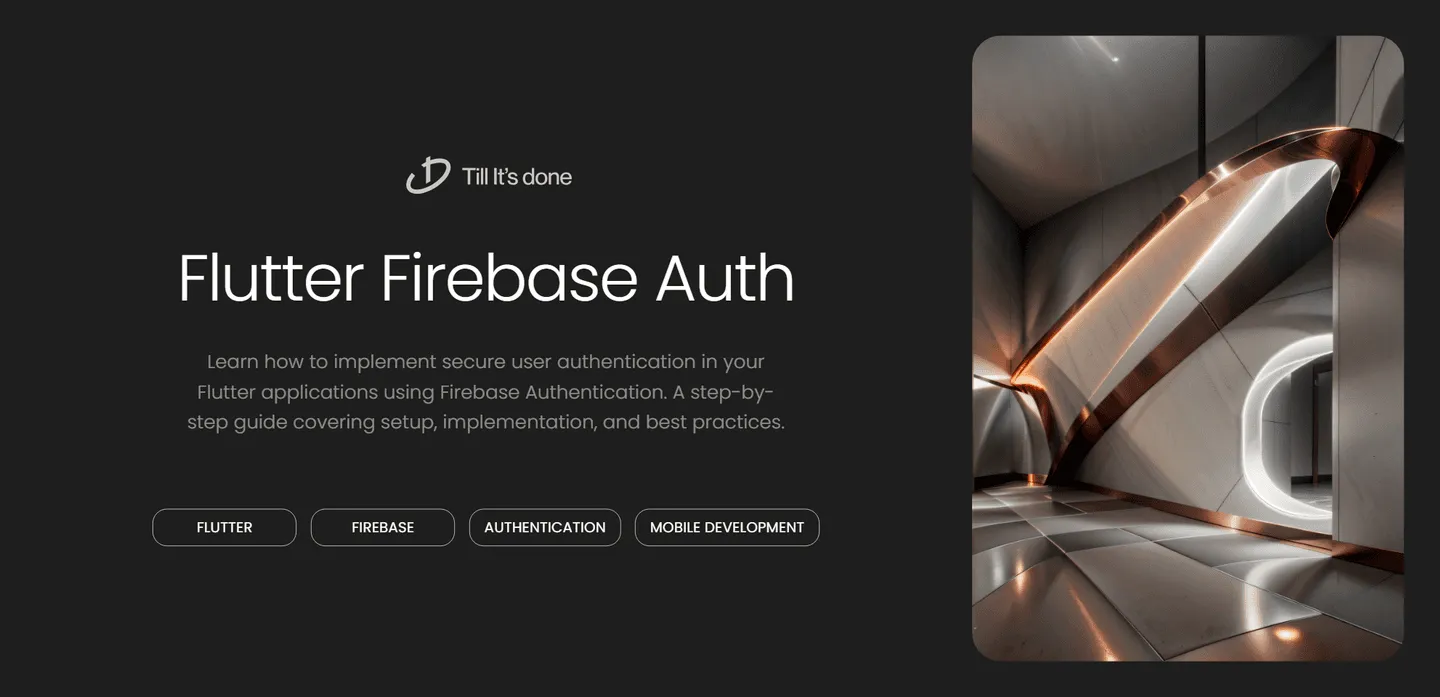
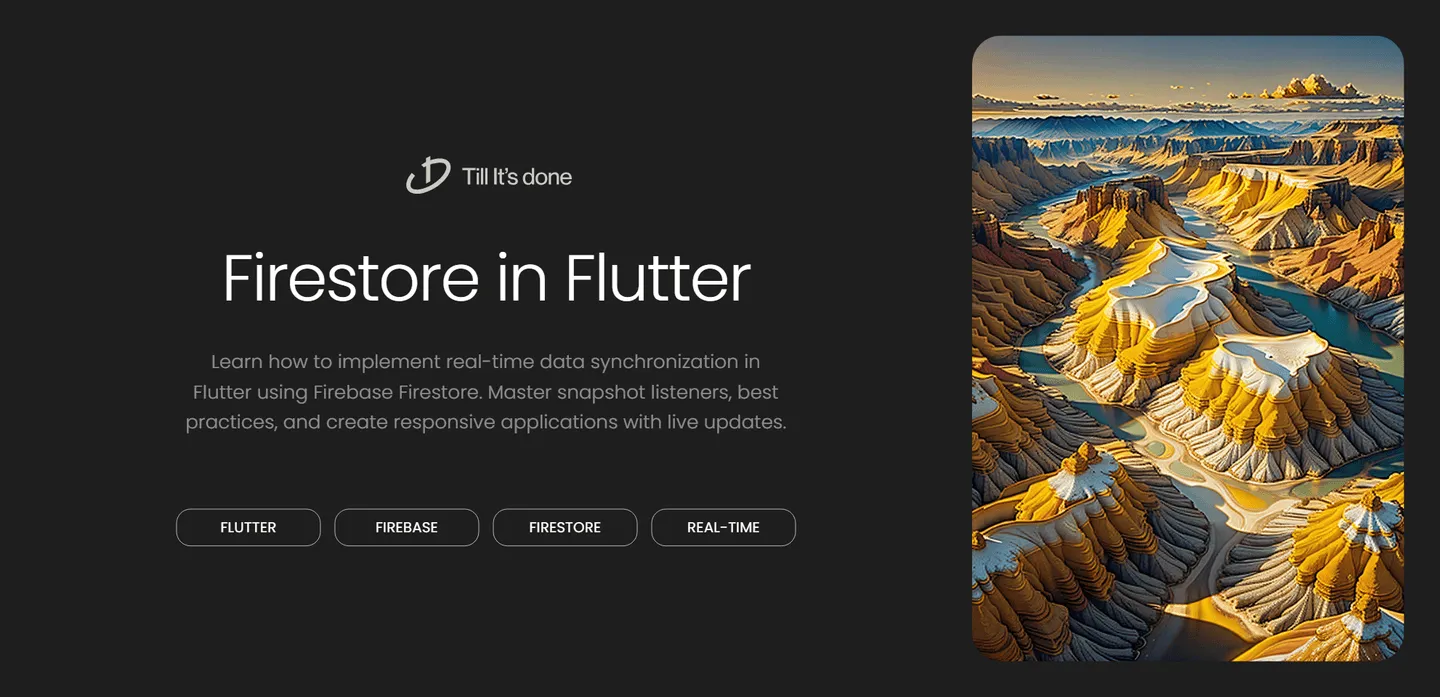
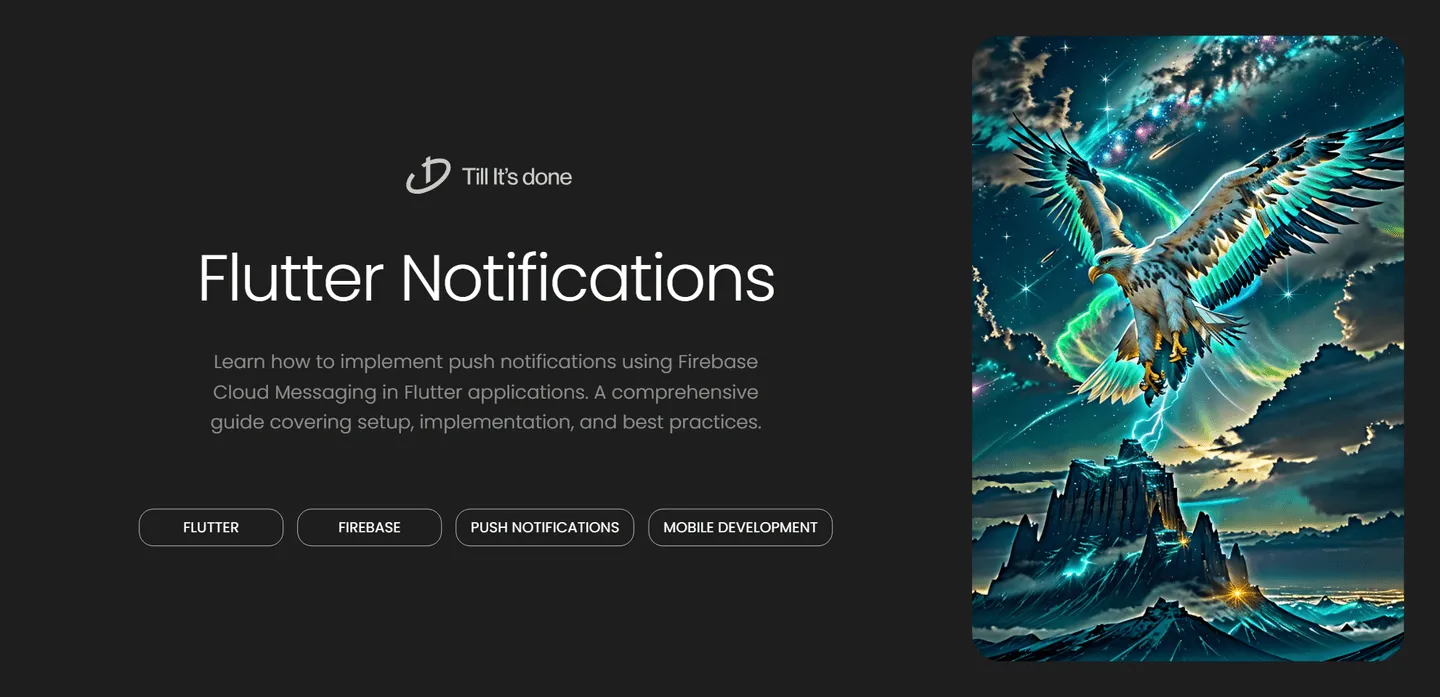
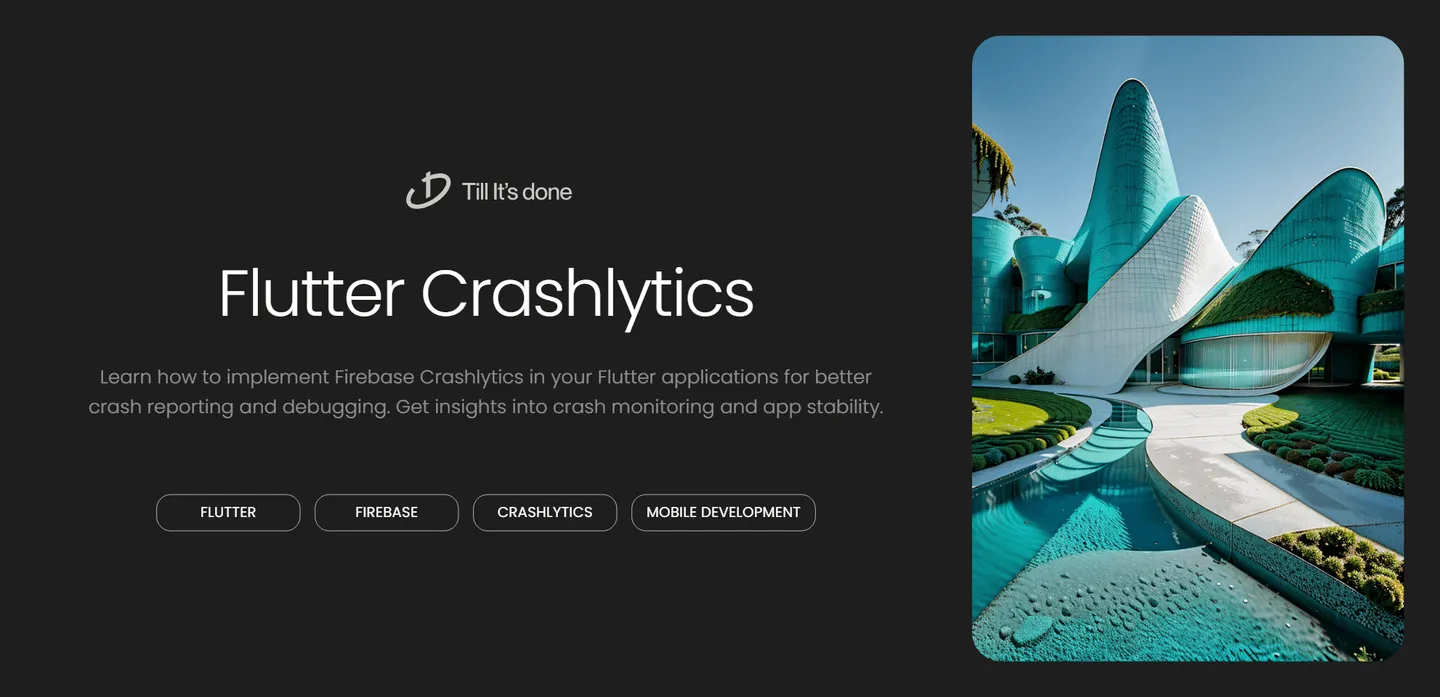
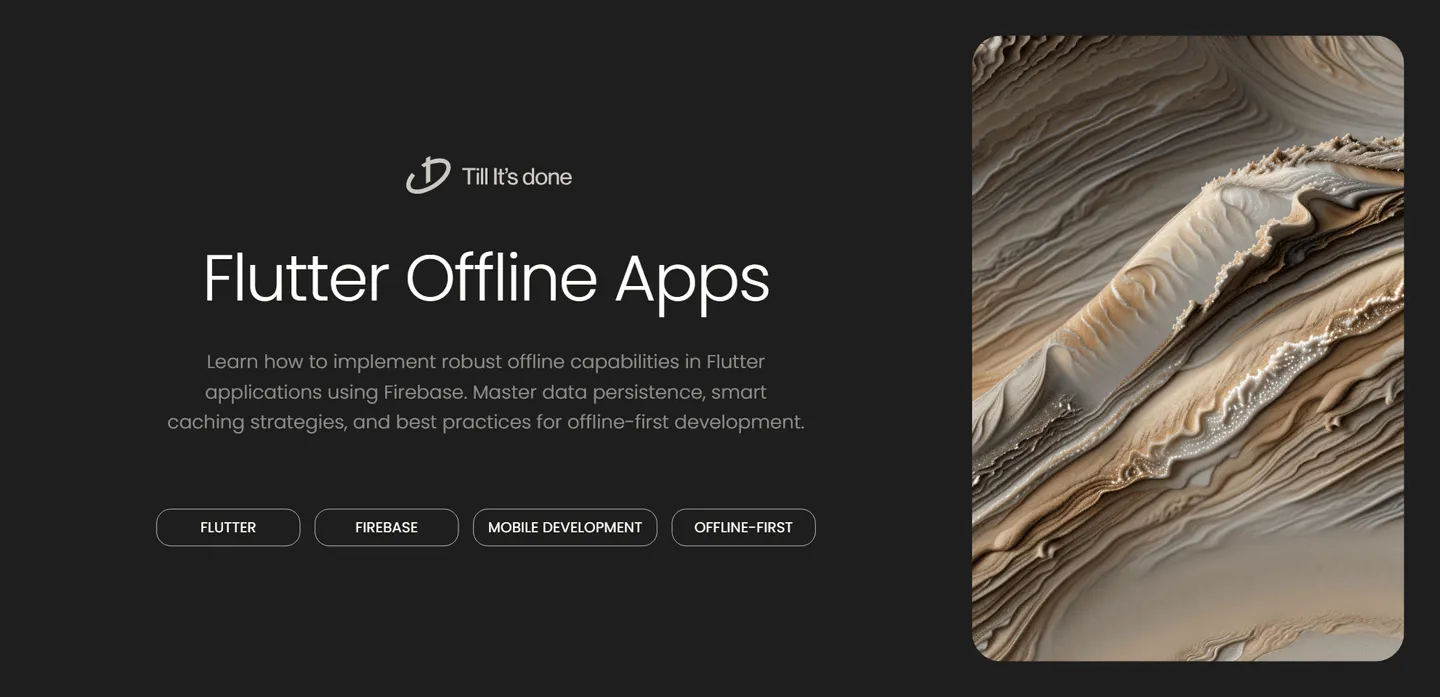
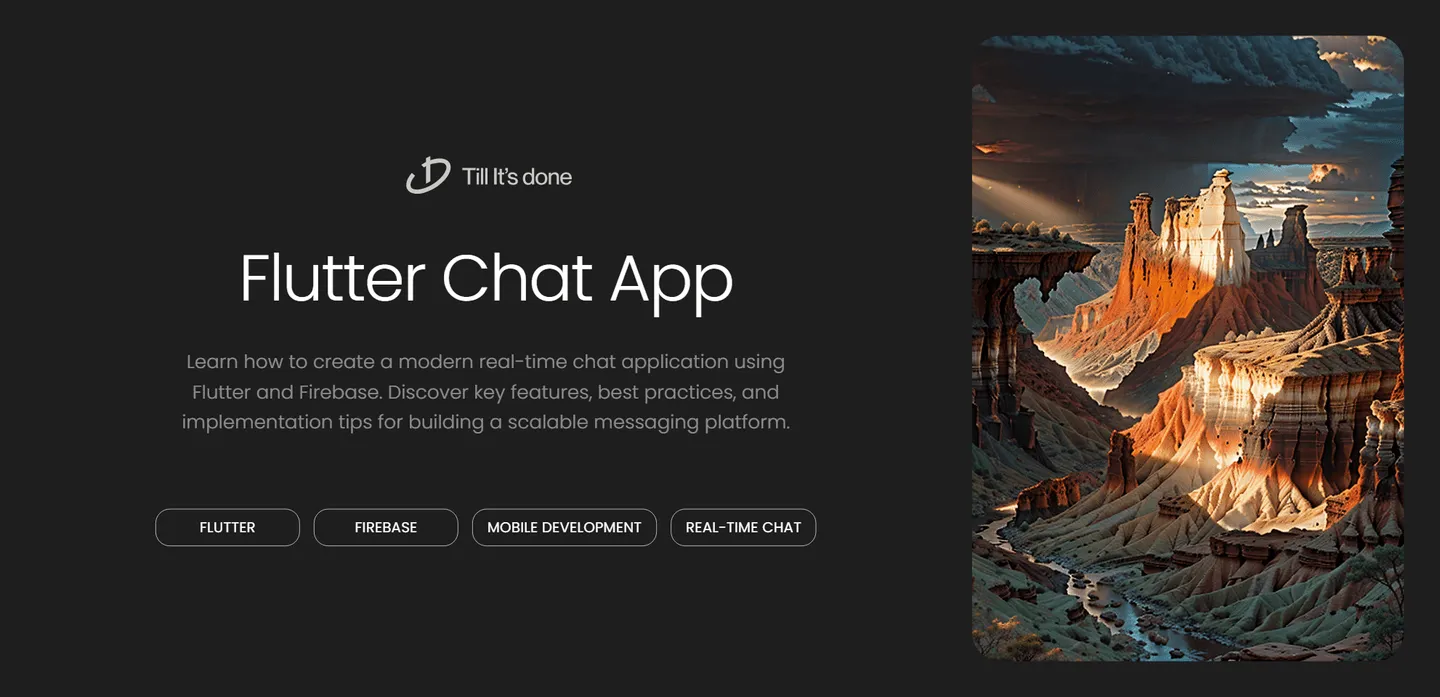
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.